Le Tutoriel de Android AlertDialog
1. Android AlertDialog
Android AlertDialog est une boîte de dialogue qui affiche un message et assiste 1, 2 ou 3 buttons. Elle facilite la création d'une boîte de dialogue avec quelques lignes de code.
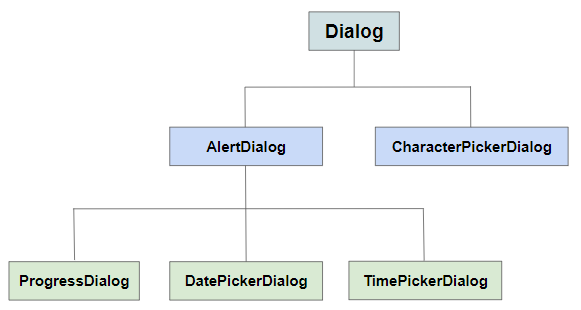
AlertDialog se compose de trois zones:
- Zone de titre (Title area)
- Zone de contenu (Content area)
- Zone des boutons (Buttons area)
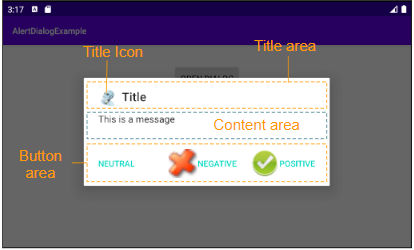
Landscape screen
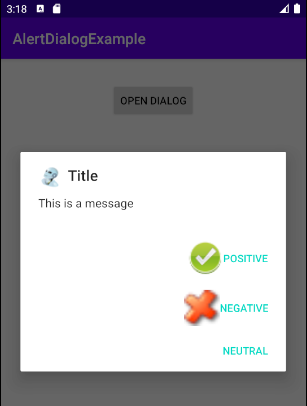
Portrait screen
Title area
Utiliser la méthode setTitle(), setIcon() pour définir le titre et l'icône d'une boîte de dialogue qui sont affichés dans Title area. Ou utilisez la méthode setCustomTitle(View) si vous voulez obtenir une zone de titre (Title area) personnalisée.
Content area
La Zone de contenu (Content area) peut afficher un message, une liste des options, etc.
Buttons area
Cette zone contient au maximum trois bouttons: Positive button, Negative button, Neutral button, dans lequels les deux premiers assistent Text & Icon, alors que Neutral button assiste uniquement Text, cependant, vous pouvez faire afficher son icône avec une petite astuce (Voir plus dans l'exemple).
2. Example d'AlertDialog (1)
Aperçu de l'exemple:
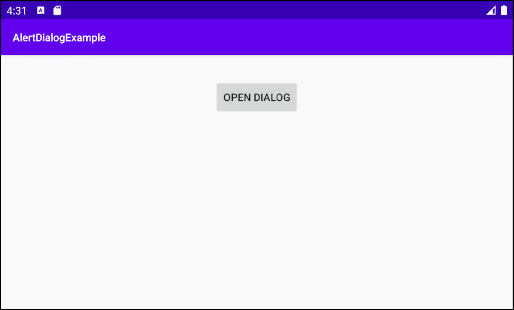
AlertButtonExample0.java
package org.o7planning.alertdialogexample;
import android.content.Context;
import android.content.DialogInterface;
import android.graphics.drawable.Drawable;
import android.widget.Toast;
import androidx.appcompat.app.AlertDialog;
public class AlertDialogExample0 {
public static void showAlertDialog(final Context context) {
final Drawable positiveIcon = context.getResources().getDrawable(R.drawable.icon_positive);
final Drawable negativeIcon = context.getResources().getDrawable(R.drawable.icon_negative);
final Drawable neutralIcon = context.getResources().getDrawable(R.drawable.icon_neutral);
AlertDialog.Builder builder = new AlertDialog.Builder(context);
// Set Title and Message:
builder.setTitle("Title").setMessage("This is a message");
//
builder.setCancelable(true);
builder.setIcon(R.drawable.icon_title);
// Create "Positive" button with OnClickListener.
builder.setPositiveButton("Positive", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(context,"You choose positive button",
Toast.LENGTH_SHORT).show();
}
});
builder.setPositiveButtonIcon(positiveIcon);
// Create "Negative" button with OnClickListener.
builder.setNegativeButton("Negative", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(context,"You choose positive button",
Toast.LENGTH_SHORT).show();
// Cancel
dialog.cancel();
}
});
builder.setNegativeButtonIcon(negativeIcon);
// Create "Neutral" button with OnClickListener.
builder.setNeutralButton("Neutral", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
// Action for 'NO' Button
Toast.makeText(context,"You choose neutral button",
Toast.LENGTH_SHORT).show();
}
});
builder.setNeutralButtonIcon(neutralIcon); // Not working!!!
// Create AlertDialog:
AlertDialog alert = builder.create();
alert.show();
}
}
3. Exemple d'AlertDialog (2)
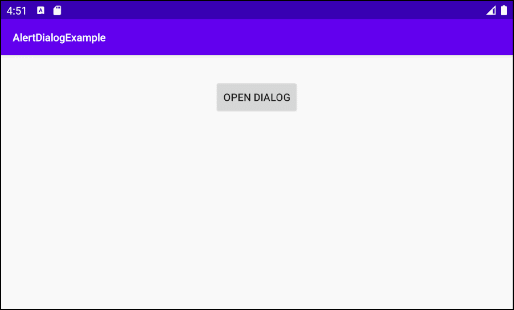
AlertDialogExample2.java
package org.o7planning.alertdialogexample;
import android.app.Activity;
import android.content.Context;
import android.content.DialogInterface;
import android.graphics.drawable.Drawable;
import android.widget.Toast;
import androidx.appcompat.app.AlertDialog;
public class AlertDialogExample2 {
public static void showAlertDialog(final Context context) {
final Drawable positiveIcon = context.getResources().getDrawable(R.drawable.icon_positive);
final Drawable negativeIcon = context.getResources().getDrawable(R.drawable.icon_negative);
AlertDialog.Builder builder = new AlertDialog.Builder(context);
// Set Title and Message:
builder.setTitle("Confirmation").setMessage("Do you want to close this app?");
//
builder.setCancelable(true);
builder.setIcon(R.drawable.icon_title);
// Create "Yes" button with OnClickListener.
builder.setPositiveButton("Yes", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(context,"You choose Yes button",
Toast.LENGTH_SHORT).show();
Activity activity = (Activity) context;
activity.finish();
}
});
builder.setPositiveButtonIcon(positiveIcon);
// Create "No" button with OnClickListener.
builder.setNegativeButton("No", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(context,"You choose No button",
Toast.LENGTH_SHORT).show();
// Cancel
dialog.cancel();
}
});
builder.setNegativeButtonIcon(negativeIcon);
// Create AlertDialog:
AlertDialog alert = builder.create();
alert.show();
}
}
4. Exemple d'AlertDialog (List)
Voici un exemple d'AlertDialog avec une liste d'options pour les utilisateurs:
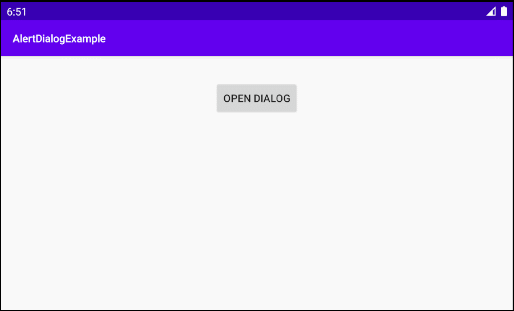
AlertDialogListExample.java
package org.o7planning.alertdialogexample;
import android.app.Activity;
import android.content.Context;
import android.content.DialogInterface;
import android.graphics.drawable.Drawable;
import android.widget.Toast;
import androidx.appcompat.app.AlertDialog;
public class AlertDialogListExample {
public static void showAlertDialog(final Activity activity) {
final Drawable negativeIcon = activity.getResources().getDrawable(R.drawable.icon_negative);
AlertDialog.Builder builder = new AlertDialog.Builder(activity);
// Set Title.
builder.setTitle("Select an Animal");
// Add a list
final String[] animals = {"Horse", "Cow", "Camel", "Sheep", "Goat"};
builder.setItems(animals, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
String animal = animals[which];
dialog.dismiss(); // Close Dialog
// Do some thing....
// For example: Call method of MainActivity.
Toast.makeText(activity,"You select: " + animal,
Toast.LENGTH_SHORT).show();
// activity.someMethod(animal);
}
});
//
builder.setCancelable(true);
builder.setIcon(R.drawable.icon_title);
// Create "Cancel" button with OnClickListener.
builder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(activity,"You choose No button",
Toast.LENGTH_SHORT).show();
// Cancel
dialog.cancel();
}
});
builder.setNegativeButtonIcon(negativeIcon);
// Create AlertDialog:
AlertDialog alert = builder.create();
alert.show();
}
}
5. Exemple d'AlertDialog (Choix unique)
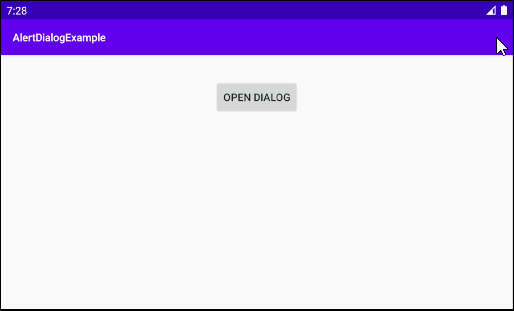
AlertDialogSingleChoiceExample.java
package org.o7planning.alertdialogexample;
import android.app.Activity;
import android.content.DialogInterface;
import android.widget.Toast;
import androidx.appcompat.app.AlertDialog;
import java.util.HashSet;
import java.util.Set;
public class AlertDialogSingleChoiceExample {
public static void showAlertDialog(final Activity activity) {
AlertDialog.Builder builder = new AlertDialog.Builder(activity);
// Set Title.
builder.setTitle("Select an Animal");
// Add a list
final String[] animals = {"Horse", "Cow", "Camel", "Sheep", "Goat"};
int checkedItem = 3; // Sheep
final Set<String> selectedItems = new HashSet<String>();
selectedItems.add(animals[checkedItem]);
builder.setSingleChoiceItems(animals, checkedItem, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// Do Something...
selectedItems.clear();
selectedItems.add(animals[which]);
}
});
//
builder.setCancelable(true);
builder.setIcon(R.drawable.icon_title);
// Create "Yes" button with OnClickListener.
builder.setPositiveButton("OK", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
if(selectedItems.isEmpty()) {
return;
}
String animal = selectedItems.iterator().next();
// Close Dialog
dialog.dismiss();
// Do something, for example: Call a method of Activity...
Toast.makeText(activity,"You select " + animal,
Toast.LENGTH_SHORT).show();
}
});
// Create "Cancel" button with OnClickListener.
builder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(activity,"You choose Cancel button",
Toast.LENGTH_SHORT).show();
// Cancel
dialog.cancel();
}
});
// Create AlertDialog:
AlertDialog alert = builder.create();
alert.show();
}
}
6. Exemple d'AlertDialog (Choix multiple)
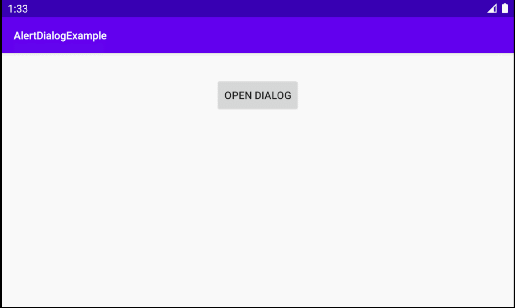
AlertDialogMultiChoiceExample.java
package org.o7planning.alertdialogexample;
import android.app.Activity;
import android.content.DialogInterface;
import android.widget.Toast;
import androidx.appcompat.app.AlertDialog;
import java.util.HashSet;
import java.util.Set;
public class AlertDialogMultiChoiceExample {
public static void showAlertDialog(final Activity activity) {
AlertDialog.Builder builder = new AlertDialog.Builder(activity);
// Set Title.
builder.setTitle("Select an Animal");
// Add a list
final String[] animals = {"Horse", "Cow", "Camel", "Sheep", "Goat"};
final boolean[] checkedInfos = new boolean[]{false, false, false, true, false}; // Sheep
builder.setMultiChoiceItems(animals, checkedInfos, new DialogInterface.OnMultiChoiceClickListener() {
@Override
public void onClick(DialogInterface dialog, int which, boolean isChecked) {
checkedInfos[which] = isChecked;
}
});
//
builder.setCancelable(true);
builder.setIcon(R.drawable.icon_title);
// Create "Yes" button with OnClickListener.
builder.setPositiveButton("OK", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
// Close Dialog
dialog.dismiss();
String s= null;
for(int i=0; i< animals.length;i++) {
if(checkedInfos[i]) {
if(s == null) {
s = animals[i];
} else {
s+= ", " + animals[i];
}
}
}
s = s == null? "":s;
// Do something, for example: Call a method of Activity...
Toast.makeText(activity,"You select " + s,
Toast.LENGTH_SHORT).show();
}
});
// Create "Cancel" button with OnClickListener.
builder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(activity,"You choose Cancel button",
Toast.LENGTH_SHORT).show();
// Cancel
dialog.cancel();
}
});
// Create AlertDialog:
AlertDialog alert = builder.create();
alert.show();
}
}
7. Exemple de Title Area personnalisé
En appliquant la méthode setCustomTitle(View) vous pouvez personnaliser la zone de titre (Title area) d'AlertDialog:
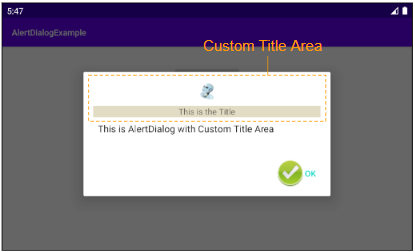
Et probablement utiliser Android Resource File pour créer l'interface pour la zone de titre (Title area).
AlertDialog.Builder builder = new AlertDialog.Builder(context);
// Custom Title Area.
LayoutInflater inflater = context.getLayoutInflater();
View view = inflater.inflate(R.layout.layout_custom_title, null);
builder.setCustomTitle(view);
...
// Create AlertDialog:
AlertDialog alert = builder.create();
alert.show();
OK, créer un Layout Resource File:
- File > New > Android Resource File
- File Name: layout_custom_title.xml
- Resource type: Layout
- Directory name: layout
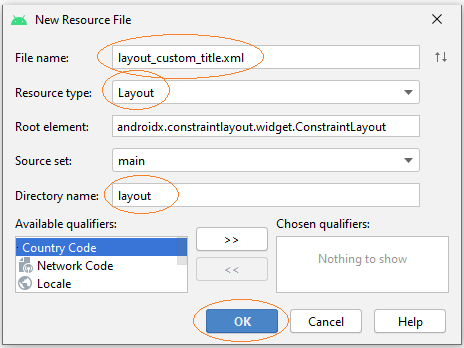
Concevoir l'interface pour la zone de titre (Title area):
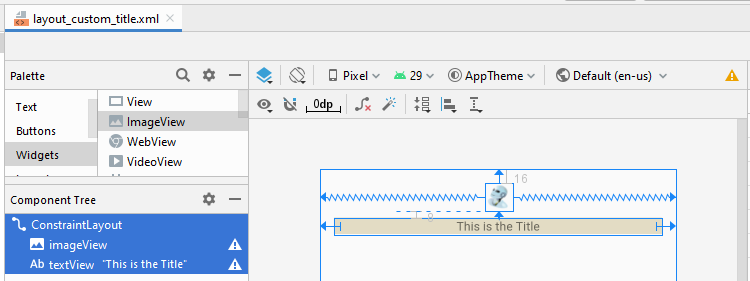
layout_custom_title.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:srcCompat="@drawable/icon_title" />
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:background="#E3DCC4"
android:gravity="center"
android:text="This is the Title"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView" />
</androidx.constraintlayout.widget.ConstraintLayout>
AlertDialogCustomTitleExample.java
package org.o7planning.alertdialogexample;
import android.app.Activity;
import android.content.Context;
import android.content.DialogInterface;
import android.graphics.drawable.Drawable;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.Toast;
import androidx.appcompat.app.AlertDialog;
public class AlertDialogCustomTitleExample {
public static void showAlertDialog(final Activity context) {
final Drawable positiveIcon = context.getResources().getDrawable(R.drawable.icon_positive);
AlertDialog.Builder builder = new AlertDialog.Builder(context);
// Custom Title Area.
LayoutInflater inflater = context.getLayoutInflater();
View view = inflater.inflate(R.layout.layout_custom_title, null);
builder.setCustomTitle(view);
// Message.
builder.setMessage("This is AlertDialog with Custom Title Area");
//
builder.setCancelable(true);
builder.setIcon(R.drawable.icon_title);
// Create "OK" button with OnClickListener.
builder.setPositiveButton("OK", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
}
});
builder.setPositiveButtonIcon(positiveIcon);
// Create AlertDialog:
AlertDialog alert = builder.create();
alert.show();
}
}
Tutoriels de programmation Android
- Configurer Android Emulator en Android Studio
- Le Tutoriel de Android ToggleButton
- Créer un File Finder Dialog simple dans Android
- Le Tutoriel de Android TimePickerDialog
- Le Tutoriel de Android DatePickerDialog
- De quoi avez-vous besoin pour démarrer avec Android?
- Installer Android Studio sur Windows
- Installer Intel® HAXM pour Android Studio
- Le Tutoriel de Android AsyncTask
- Le Tutoriel de Android AsyncTaskLoader
- Tutoriel Android pour débutant - Exemples de base
- Comment connaître le numéro de téléphone d'Android Emulator et le changer?
- Le Tutoriel de Android TextInputLayout
- Le Tutoriel de Android CardView
- Le Tutoriel de Android ViewPager2
- Obtenir un numéro de téléphone dans Android à l'aide de TelephonyManager
- Le Tutoriel de Android Phone Call
- Le Tutoriel de Android Wifi Scanning
- Le Tutoriel de programmation de jeux Android 2D pour débutant
- Le Tutoriel de Android DialogFragment
- Le Tutoriel de Android CharacterPickerDialog
- Le Tutoriel Android pour débutant - Hello Android
- Utiliser Android Device File Explorer
- Activer USB Debugging sur un appareil Android
- Le Tutoriel de Android UI Layouts
- Le Tutoriel de Android SMS
- Le Tutoriel de Android et SQLite Database
- Le Tutoriel de Google Maps Android API
- Le Tutoriel de texte pour parler dans Android
- Le Tutoriel de Android Space
- Le Tutoriel de Android Toast
- Créer un Android Toast personnalisé
- Le Tutoriel de Android SnackBar
- Le Tutoriel de Android TextView
- Le Tutoriel de Android TextClock
- Le Tutoriel de Android EditText
- Le Tutoriel de Android TextWatcher
- Formater le numéro de carte de crédit avec Android TextWatcher
- Le Tutoriel de Android Clipboard
- Créer un File Chooser simple dans Android
- Le Tutoriel de Android AutoCompleteTextView et MultiAutoCompleteTextView
- Le Tutoriel de Android ImageView
- Le Tutoriel de Android ImageSwitcher
- Le Tutoriel de Android ScrollView et HorizontalScrollView
- Le Tutoriel de Android WebView
- Le Tutoriel de Android SeekBar
- Le Tutoriel de Android Dialog
- Le Tutoriel de Android AlertDialog
- Tutoriel Android RatingBar
- Le Tutoriel de Android ProgressBar
- Le Tutoriel de Android Spinner
- Le Tutoriel de Android Button
- Le Tutoriel de Android Switch
- Le Tutoriel de Android ImageButton
- Le Tutoriel de Android FloatingActionButton
- Le Tutoriel de Android CheckBox
- Le Tutoriel de Android RadioGroup et RadioButton
- Le Tutoriel de Android Chip et ChipGroup
- Utilisation des Image assets et des Icon assets d'Android Studio
- Configuration de la Carte SD pour Android Emulator
- Exemple ChipGroup et Chip Entry
- Comment ajouter des bibliothèques externes à Android Project dans Android Studio?
- Comment désactiver les autorisations déjà accordées à l'application Android?
- Comment supprimer des applications de Android Emulator?
- Le Tutoriel de Android LinearLayout
- Le Tutoriel de Android TableLayout
- Le Tutoriel de Android FrameLayout
- Le Tutoriel de Android QuickContactBadge
- Le Tutoriel de Android StackView
- Le Tutoriel de Android Camera
- Le Tutoriel de Android MediaPlayer
- Le Tutoriel de Android VideoView
- Jouer des effets sonores dans Android avec SoundPool
- Le Tutoriel de Android Networking
- Analyser JSON dans Android
- Le Tutoriel de Android SharedPreferences
- Le Tutorial de stockage interne Android (Internal Storage)
- Le Tutoriel de Android External Storage
- Le Tutoriel de Android Intents
- Exemple d'une Android Intent explicite, appelant une autre Intent
- Exemple de Android Intent implicite, ouvrez une URL, envoyez un email
- Le Tutoriel de Android Service
- Le Tutoriel Android Notifications
- Le Tutoriel de Android DatePicker
- Le Tutoriel de Android TimePicker
- Le Tutoriel de Android Chronometer
- Le Tutoriel de Android OptionMenu
- Le Tutoriel de Android ContextMenu
- Le Tutoriel de Android PopupMenu
- Le Tutoriel de Android Fragment
- Le Tutoriel de Android ListView
- Android ListView avec Checkbox en utilisant ArrayAdapter
- Le Tutoriel de Android GridView
Show More