Tutoriel Android RatingBar
1. Android RatingBar
Sous Android, RatingBar est un contrôle d'interface utilisateur (User Interface Control) permettant de recevoir les avis des utilisateurs. RatingBar est un descendant de ProgressBar qui hérite donc les fonctions de ProgressBar. Il permet aussi d'afficher une note globale (moyenne) de tous les utilisateurs. En termes d'interface, RatingBar se compose des étoiles et les utilisateurs en sélectionnent une pour donner une note, la première étoile correspond à la note la plus faible et la dernière correspond à la note la plus élevée.
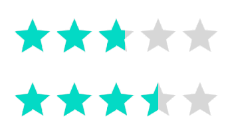
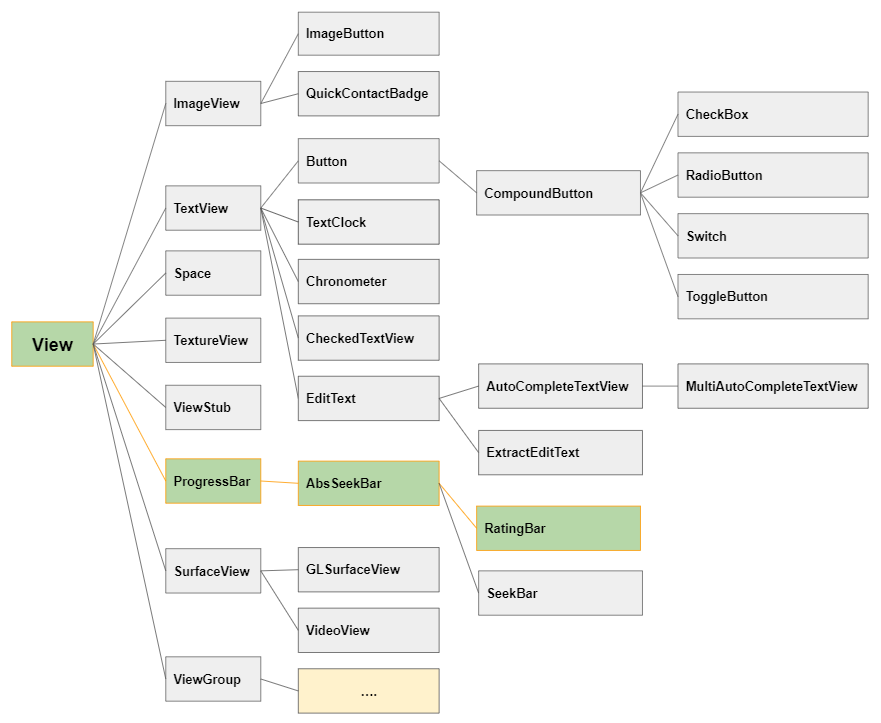
Ci-dessous la structure d'un RatingBar:
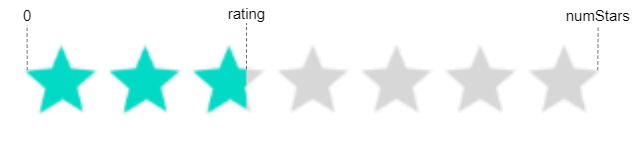
android:numStars
L'attribut android:numStars permet de définir le nombre d'étoiles affichées sur RatingBar. Sa valeur par défaut est 5.
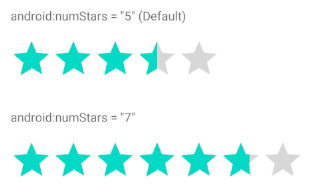
android:numStars
<!-- numStars default is 5 -->
<RatingBar
android:id="@+id/ratingBar21"
android:rating="3.5"
android:stepSize="0.1" ... />
<!-- numStars = 7 -->
<RatingBar
android:id="@+id/ratingBar22"
android:numStars="7"
android:rating="5.7"
android:stepSize="0.1" ... />
android:rating, android:stepSize
android:rating: Une valeur indiquant la note de l'utilisateur ou la valeur moyenne de toutes les évaluations des utilisateurs. Cela se situe entre (0,numStars].
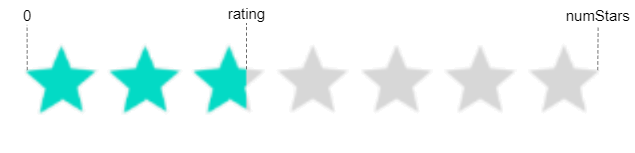
La valeur par défaut de l'attribut android:stepSize est 0.5, ce qui signifie que RatingBar n'affiche que les notes (ou les avis d'utilisateurs) avec les valeurs 0, 0.5, 1, 1.5, 2, etc. Quand la valeur de l'attribut android:stepSite est modifié en 0.1, RatingBar peut afficher des valeurs 0, 0.1, 0.2, 0.3, 0.4, 0.5, etc.
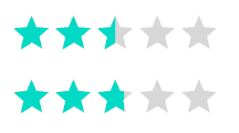
android:stepSize
<!-- android.stepSize = "0.5" (Default) -->
<RatingBar
android:id="@+id/ratingBar31"
android:rating="2.7" ... />
<!-- android.stepSize = "0.1" -->
<RatingBar
android:id="@+id/ratingBar32"
android:rating="2.7"
android:stepSize="0.1" ... />
android:isIndicator
L'attribut android:isIndicator = "true" est utilisé quand vous ne voulez pas permettre aux utilisateurs de laisser une note avec RatingBar. Par exemple, vous prévoyez d'avoir une RatingBar pour afficher uniquement une note globale (moyenne). Par défaut, la valeur de l'attribut est false.
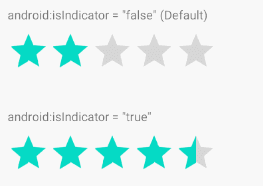
<!-- android:isIndicator = "false" (Default) -->
<RatingBar
android:id="@+id/ratingBar51"
android:rating="2" ... />
<!-- android:isIndicator = "true" (Readonly RatingBar) -->
<RatingBar
android:id="@+id/ratingBar52"
android:isIndicator="true"
android:rating="4.5" ... />
2. RatingBar Styles
L'attribut style est une option de RatingBar permettant de définir le style pour RatingBar. Vous pouvez utiliser certains styles disponibles dans la bibliothèque d'Android.
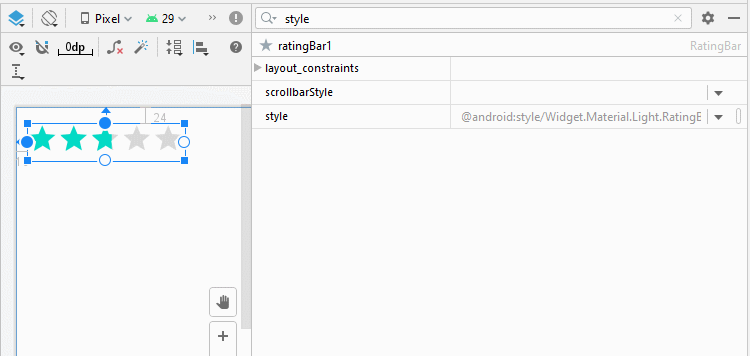
La bibliothèque d'Android fournit certains styles pour RatingBar, pourtant, beaucoup d'entre eux se ressemblent. Ainsi, il est difficile de faire la différence. Ci-dessous un exemple avec certains styles sympas:
- style="@style/Widget.AppCompat.RatingBar.Indicator"
- style="@android:style/Widget.DeviceDefault.Light.RatingBar.Small"
- style="@android:style/Widget.RatingBar"
- style="@android:style/Widget.Holo.RatingBar"
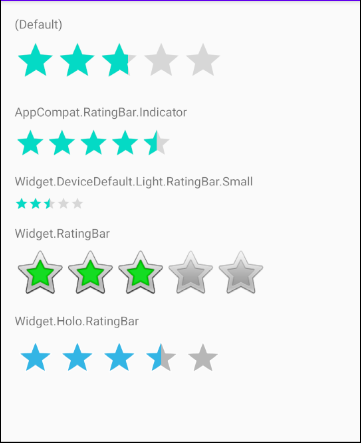
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<TextView
android:id="@+id/textView41"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="(Default)"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<RatingBar
android:id="@+id/ratingBar41"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:rating="2.7"
android:stepSize="0.1"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView41" />
<TextView
android:id="@+id/textView42"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="AppCompat.RatingBar.Indicator"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ratingBar41" />
<RatingBar
android:id="@+id/ratingBar42"
style="@style/Widget.AppCompat.RatingBar.Indicator"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:rating="4.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView42" />
<TextView
android:id="@+id/textView43"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.DeviceDefault.Light.RatingBar.Small"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ratingBar42" />
<RatingBar
android:id="@+id/ratingBar43"
style="@android:style/Widget.DeviceDefault.Light.RatingBar.Small"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:rating="2.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView43" />
<TextView
android:id="@+id/textView44"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.RatingBar"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ratingBar43" />
<RatingBar
android:id="@+id/ratingBar44"
style="@android:style/Widget.RatingBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:rating="3"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView44" />
<TextView
android:id="@+id/textView45"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.Holo.RatingBar"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ratingBar44" />
<RatingBar
android:id="@+id/ratingBa45"
style="@android:style/Widget.Holo.RatingBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:rating="3.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView45" />
</androidx.constraintlayout.widget.ConstraintLayout>
3. RatingBar Events
Il y a certains évènements relatifs à une RatingBar, ci-dessous ceux les plus utilisés:
- setOnClickListener(View.OnClickListener);
- setOnRatingBarChangeListener(RatingBar.OnRatingBarChangeListener);
On Click Event:
L'évènement se produit quand l'utilisateur clique (click) sur RatingBar. Cela donne le même effet quand l'utilisateur clique sur un Button.
ratingBar.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
RatingBar rb = (RatingBar) v;
float rating = rb.getRating();
// Your code ...
}
});
On RatingBar Changed Event
L'évènement se produit quand la valeur de rating change en raison d'une action de l'utilisateur ou sous l'effet de la méthode ratingBar.setRating(newRating), etc.
ratingBar.setOnRatingBarChangeListener(new RatingBar.OnRatingBarChangeListener() {
@Override
public void onRatingChanged(RatingBar ratingBar, float newRating, boolean fromUser) {
// Your code
}
});
4. Exemple de RatingBar
OK, prendre un exemple simple en utilisant RatingBar. Dans cet exemple, la première RatingBar reçoit les avis d'utilisateurs. La seconde RatingBar affiche une note globale (moyenne) de tous les utilisateurs.
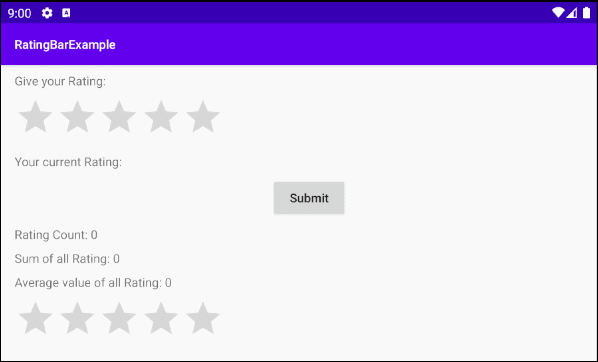
Dans Android Studio, créer un nouveau projet:
- File > New > New Project > Empty Activity
- Name: RatingBarExample
- Package name: org.o7planning.ratingbarexample
- Language: Java
Voici l'interface de l'application:
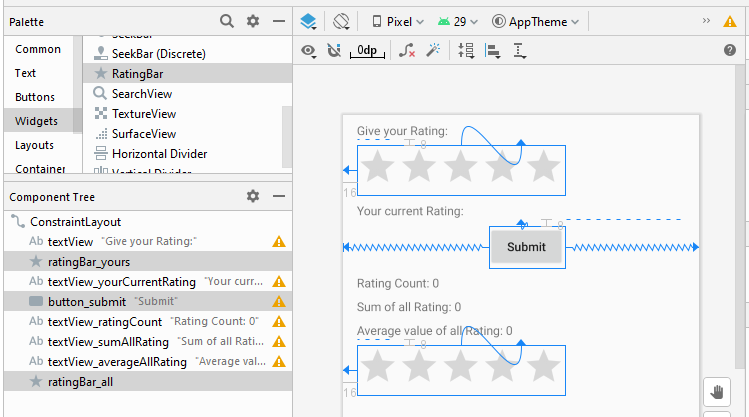
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Give your Rating:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<RatingBar
android:id="@+id/ratingBar_yours"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<TextView
android:id="@+id/textView_yourCurrentRating"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Your current Rating:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ratingBar_yours" />
<Button
android:id="@+id/button_submit"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:text="Submit"
android:textAllCaps="false"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.52"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_yourCurrentRating" />
<TextView
android:id="@+id/textView_ratingCount"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Rating Count: 0"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_submit" />
<TextView
android:id="@+id/textView_sumAllRating"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Sum of all Rating: 0"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_ratingCount" />
<TextView
android:id="@+id/textView_averageAllRating"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Average value of all Rating: 0"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_sumAllRating" />
<RatingBar
android:id="@+id/ratingBar_all"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:isIndicator="true"
android:stepSize="0.1"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_averageAllRating" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.ratingbarexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.RatingBar;
import android.widget.TextView;
import java.util.ArrayList;
import java.util.List;
public class MainActivity extends AppCompatActivity {
private RatingBar ratingBarYours;
private RatingBar ratingBarAll;
private Button buttonSubmit;
private TextView textViewYourCurrentRating;
private TextView textViewRatingCount;
private TextView textViewSumAllRating;
private TextView textViewAverageAllRating;
private List<Float> allRatings = new ArrayList<Float>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//
this.buttonSubmit = (Button) this.findViewById(R.id.button_submit);
this.ratingBarYours = (RatingBar) this.findViewById(R.id.ratingBar_yours);
this.ratingBarAll = (RatingBar) this.findViewById(R.id.ratingBar_all);
this.textViewYourCurrentRating = (TextView) this.findViewById(R.id.textView_yourCurrentRating);
this.textViewRatingCount= (TextView) this.findViewById(R.id.textView_ratingCount);
this.textViewSumAllRating= (TextView) this.findViewById(R.id.textView_sumAllRating);
this.textViewAverageAllRating= (TextView) this.findViewById(R.id.textView_averageAllRating);
this.ratingBarYours.setOnRatingBarChangeListener(new RatingBar.OnRatingBarChangeListener() {
@Override
public void onRatingChanged(RatingBar ratingBar, float rating, boolean fromUser) {
textViewYourCurrentRating.setText("Your current Rating: " + rating);
}
});
this.buttonSubmit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
doSubmit();
}
});
}
private void doSubmit() {
float rating = this.ratingBarYours.getRating();
this.allRatings.add(rating);
int ratingCount = this.allRatings.size();
float ratingSum = 0f;
for(Float r: this.allRatings) {
ratingSum += r;
}
float averageRating = ratingSum / ratingCount;
this.textViewRatingCount.setText("Rating Count: " + ratingCount);
this.textViewSumAllRating.setText("Sum off all Rating: " + ratingSum);
this.textViewAverageAllRating.setText("Average value off all Rating: " + averageRating);
float ratingAll = this.ratingBarAll.getNumStars() * averageRating / this.ratingBarYours.getNumStars() ;
this.ratingBarAll.setRating(ratingAll);
}
}
Tutoriels de programmation Android
- Configurer Android Emulator en Android Studio
- Le Tutoriel de Android ToggleButton
- Créer un File Finder Dialog simple dans Android
- Le Tutoriel de Android TimePickerDialog
- Le Tutoriel de Android DatePickerDialog
- De quoi avez-vous besoin pour démarrer avec Android?
- Installer Android Studio sur Windows
- Installer Intel® HAXM pour Android Studio
- Le Tutoriel de Android AsyncTask
- Le Tutoriel de Android AsyncTaskLoader
- Tutoriel Android pour débutant - Exemples de base
- Comment connaître le numéro de téléphone d'Android Emulator et le changer?
- Le Tutoriel de Android TextInputLayout
- Le Tutoriel de Android CardView
- Le Tutoriel de Android ViewPager2
- Obtenir un numéro de téléphone dans Android à l'aide de TelephonyManager
- Le Tutoriel de Android Phone Call
- Le Tutoriel de Android Wifi Scanning
- Le Tutoriel de programmation de jeux Android 2D pour débutant
- Le Tutoriel de Android DialogFragment
- Le Tutoriel de Android CharacterPickerDialog
- Le Tutoriel Android pour débutant - Hello Android
- Utiliser Android Device File Explorer
- Activer USB Debugging sur un appareil Android
- Le Tutoriel de Android UI Layouts
- Le Tutoriel de Android SMS
- Le Tutoriel de Android et SQLite Database
- Le Tutoriel de Google Maps Android API
- Le Tutoriel de texte pour parler dans Android
- Le Tutoriel de Android Space
- Le Tutoriel de Android Toast
- Créer un Android Toast personnalisé
- Le Tutoriel de Android SnackBar
- Le Tutoriel de Android TextView
- Le Tutoriel de Android TextClock
- Le Tutoriel de Android EditText
- Le Tutoriel de Android TextWatcher
- Formater le numéro de carte de crédit avec Android TextWatcher
- Le Tutoriel de Android Clipboard
- Créer un File Chooser simple dans Android
- Le Tutoriel de Android AutoCompleteTextView et MultiAutoCompleteTextView
- Le Tutoriel de Android ImageView
- Le Tutoriel de Android ImageSwitcher
- Le Tutoriel de Android ScrollView et HorizontalScrollView
- Le Tutoriel de Android WebView
- Le Tutoriel de Android SeekBar
- Le Tutoriel de Android Dialog
- Le Tutoriel de Android AlertDialog
- Tutoriel Android RatingBar
- Le Tutoriel de Android ProgressBar
- Le Tutoriel de Android Spinner
- Le Tutoriel de Android Button
- Le Tutoriel de Android Switch
- Le Tutoriel de Android ImageButton
- Le Tutoriel de Android FloatingActionButton
- Le Tutoriel de Android CheckBox
- Le Tutoriel de Android RadioGroup et RadioButton
- Le Tutoriel de Android Chip et ChipGroup
- Utilisation des Image assets et des Icon assets d'Android Studio
- Configuration de la Carte SD pour Android Emulator
- Exemple ChipGroup et Chip Entry
- Comment ajouter des bibliothèques externes à Android Project dans Android Studio?
- Comment désactiver les autorisations déjà accordées à l'application Android?
- Comment supprimer des applications de Android Emulator?
- Le Tutoriel de Android LinearLayout
- Le Tutoriel de Android TableLayout
- Le Tutoriel de Android FrameLayout
- Le Tutoriel de Android QuickContactBadge
- Le Tutoriel de Android StackView
- Le Tutoriel de Android Camera
- Le Tutoriel de Android MediaPlayer
- Le Tutoriel de Android VideoView
- Jouer des effets sonores dans Android avec SoundPool
- Le Tutoriel de Android Networking
- Analyser JSON dans Android
- Le Tutoriel de Android SharedPreferences
- Le Tutorial de stockage interne Android (Internal Storage)
- Le Tutoriel de Android External Storage
- Le Tutoriel de Android Intents
- Exemple d'une Android Intent explicite, appelant une autre Intent
- Exemple de Android Intent implicite, ouvrez une URL, envoyez un email
- Le Tutoriel de Android Service
- Le Tutoriel Android Notifications
- Le Tutoriel de Android DatePicker
- Le Tutoriel de Android TimePicker
- Le Tutoriel de Android Chronometer
- Le Tutoriel de Android OptionMenu
- Le Tutoriel de Android ContextMenu
- Le Tutoriel de Android PopupMenu
- Le Tutoriel de Android Fragment
- Le Tutoriel de Android ListView
- Android ListView avec Checkbox en utilisant ArrayAdapter
- Le Tutoriel de Android GridView
Show More