Le Tutoriel de Android VideoView
1. Android VideoView
VideoView est un composant personnalisé disponible sur Android, c'est la combinaison de MediaPlayer et de SurfaceView qui vous aide à lire une vidéo plus facilement.
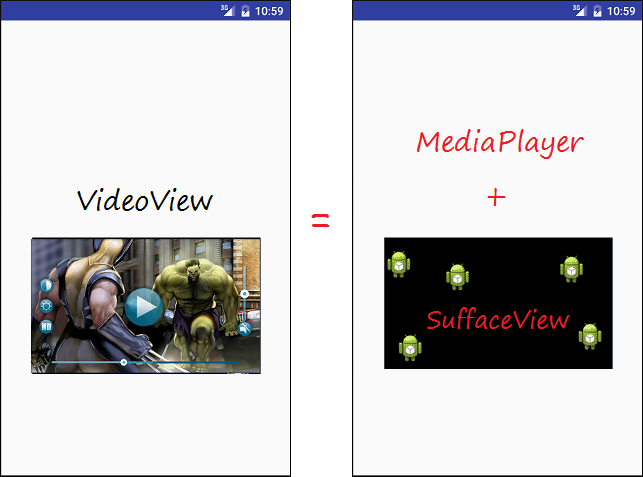
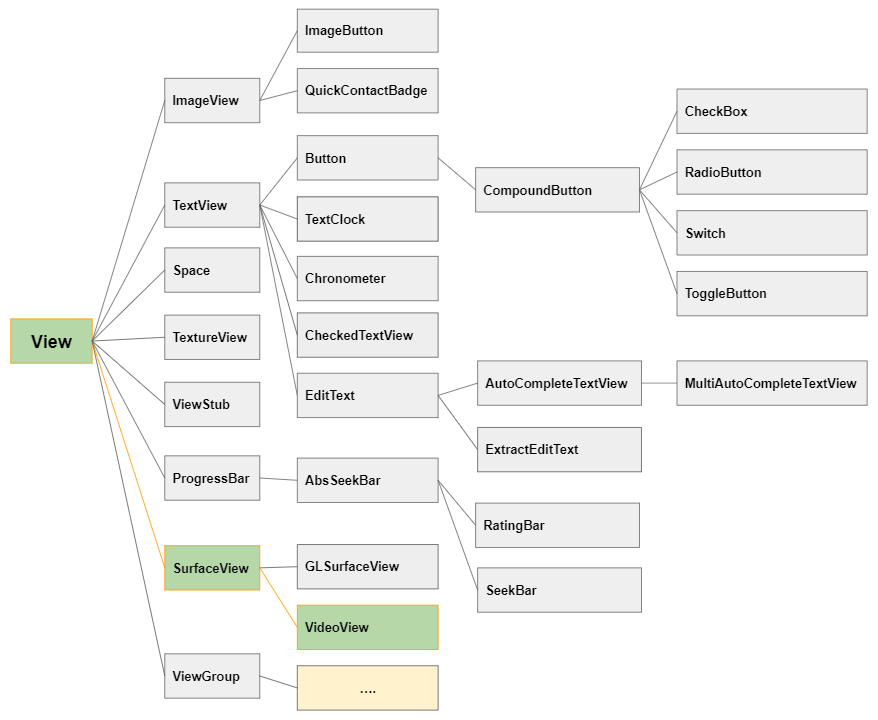
Lorsque vous utilisez VideoView, vous pouvez utiliser MediaController, cela est disponible dans Android qui sert à contrôler des médias (par exemple, le démarrage, l'arrêt, le rembobinage, la pause ...)
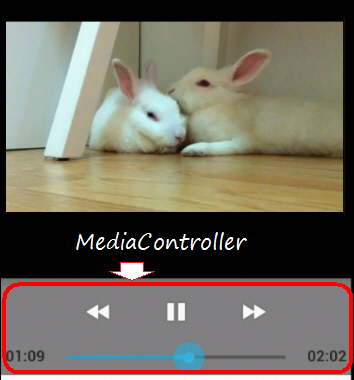
Si vous localisez VideoView et MediaController dans FrameLayout, vous pouvez obtenir l'interface comme ci- dessous:
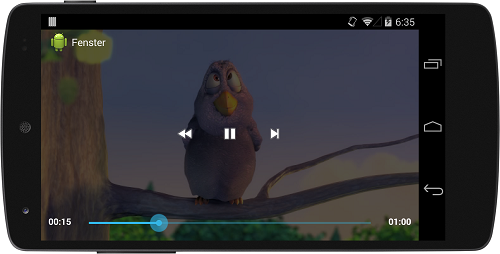
2. Exemple de lire une vidéo avec VideoView
Maintenant, nous pouvons voir un exemple avec VideoView et MediaController situé à la surface de la vidéo. Vous pouvez prévisualiser les images dans l'exemple ci- dessous:
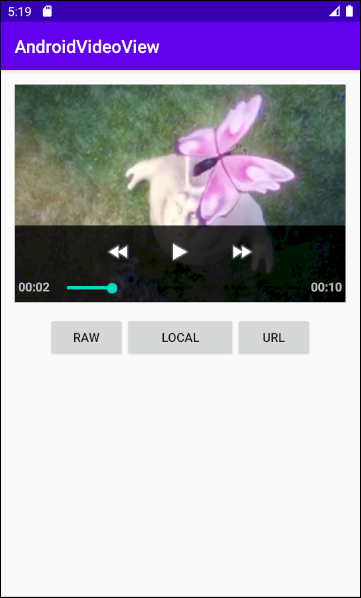
Créez un nouveau projet nommé AndroidVideoView:
- File > New > New Project > Empty Activity
- Name: AndroidVideoView
- Package name: org.o7planning.androidvideoview
- Language: Java
Créez un dossier raw afin de comprendre des fichiers video.
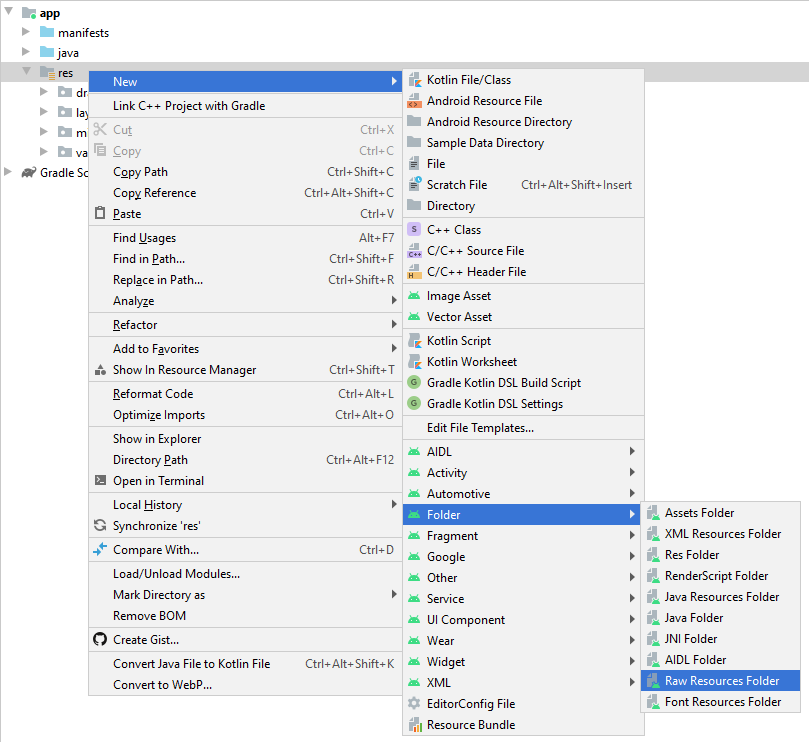
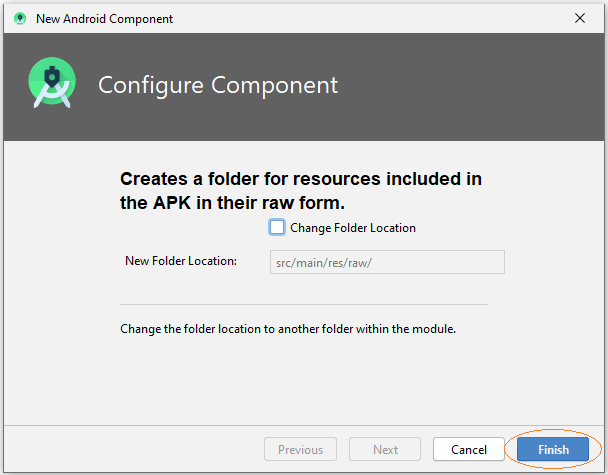
Copiez & Collez des fichiers video mp4 dans le dossier raw:
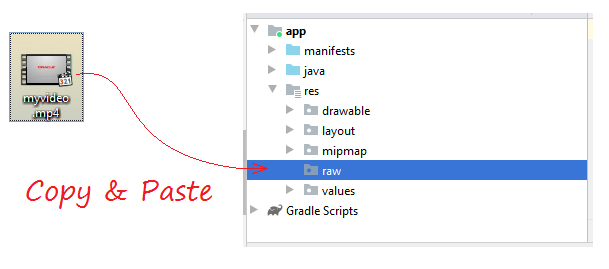
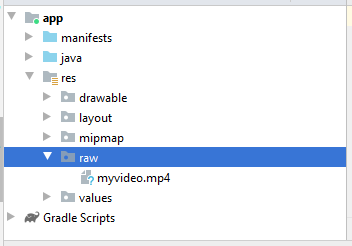
If you want to play a video from a URL you need to allow the application to use the network, add the following XML code to AndroidManifest.xml:
<uses-permission android:name="android.permission.INTERNET"/>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.o7planning.androidvideoview">
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Design the Interface:
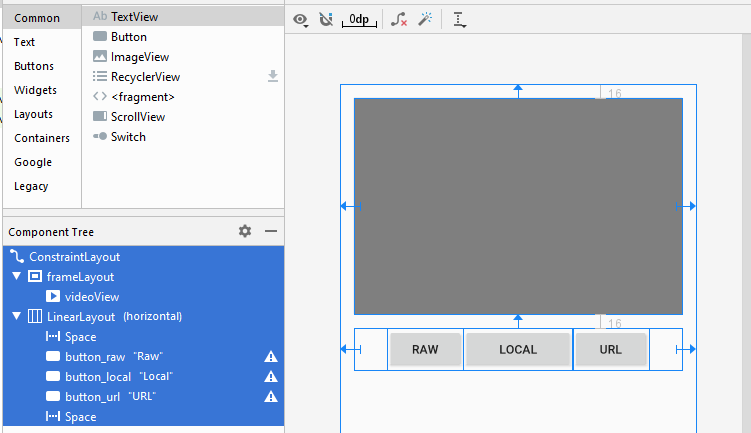
If you are interested in the steps to design the interface of this application, please see the appendix at the end of the article.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<FrameLayout
android:id="@+id/frameLayout"
android:layout_width="0dp"
android:layout_height="250dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<VideoView
android:id="@+id/videoView"
android:layout_width="wrap_content"
android:layout_height="match_parent" />
</FrameLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/frameLayout">
<Space
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1" />
<Button
android:id="@+id/button_raw"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Raw" />
<Button
android:id="@+id/button_local"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Local" />
<Button
android:id="@+id/button_url"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="URL" />
<Space
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1" />
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.androidvideoview;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.media.MediaPlayer;
import android.media.MediaPlayer.OnPreparedListener;
import android.view.View;
import android.widget.Button;
import android.widget.MediaController;
import android.widget.VideoView;
public class MainActivity extends AppCompatActivity {
private VideoView videoView;
private int position = 0;
private MediaController mediaController;
private Button buttonRaw;
private Button buttonLocal;
private Button buttonURL;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.videoView = (VideoView) findViewById(R.id.videoView);
this.buttonRaw = (Button) findViewById(R.id.button_raw);
this.buttonLocal = (Button) findViewById(R.id.button_local );
this.buttonURL = (Button) findViewById(R.id.button_url);
// Set the media controller buttons
if (this.mediaController == null) {
this.mediaController = new MediaController(MainActivity.this);
// Set the videoView that acts as the anchor for the MediaController.
this.mediaController.setAnchorView(videoView);
// Set MediaController for VideoView
this.videoView.setMediaController(mediaController);
}
// When the video file ready for playback.
this.videoView.setOnPreparedListener(new OnPreparedListener() {
public void onPrepared(MediaPlayer mediaPlayer) {
videoView.seekTo(position);
if (position == 0) {
videoView.start();
}
// When video Screen change size.
mediaPlayer.setOnVideoSizeChangedListener(new MediaPlayer.OnVideoSizeChangedListener() {
@Override
public void onVideoSizeChanged(MediaPlayer mp, int width, int height) {
// Re-Set the videoView that acts as the anchor for the MediaController
mediaController.setAnchorView(videoView);
}
});
}
});
this.buttonRaw.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// "myvideo.mp4" in directory "raw".
String resName = VideoViewUtils.RAW_VIDEO_SAMPLE;
VideoViewUtils.playRawVideo(MainActivity.this, videoView, resName);
}
});
this.buttonLocal.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String localPath = VideoViewUtils.LOCAL_VIDEO_SAMPLE;
VideoViewUtils.playLocalVideo(MainActivity.this, videoView, localPath);
}
});
this.buttonURL.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String videoURL = VideoViewUtils.URL_VIDEO_SAMPLE;
VideoViewUtils.playURLVideo(MainActivity.this, videoView, videoURL);
}
});
}
// When you change direction of phone, this method will be called.
// It store the state of video (Current position)
@Override
public void onSaveInstanceState(Bundle savedInstanceState) {
super.onSaveInstanceState(savedInstanceState);
// Store current position.
savedInstanceState.putInt("CurrentPosition", videoView.getCurrentPosition());
videoView.pause();
}
// After rotating the phone. This method is called.
@Override
public void onRestoreInstanceState(Bundle savedInstanceState) {
super.onRestoreInstanceState(savedInstanceState);
// Get saved position.
position = savedInstanceState.getInt("CurrentPosition");
videoView.seekTo(position);
}
}
VideoViewUtils.java
package org.o7planning.androidvideoview;
import android.content.Context;
import android.net.Uri;
import android.util.Log;
import android.widget.Toast;
import android.widget.VideoView;
public class VideoViewUtils {
// "myvideo.mp4" in directory "raw".
public static final String RAW_VIDEO_SAMPLE = "myvideo";
public static final String LOCAL_VIDEO_SAMPLE ="/storage/emulated/0/DCIM/Camera/VID_20180212_195520.mp4";
public static final String URL_VIDEO_SAMPLE = "https://ex1.o7planning.com/_testdatas_/mov_bbb.mp4";
public static final String LOG_TAG= "AndroidVideoView";
// Play a video in directory RAW.
// Video name = "myvideo.mp4" ==> resName = "myvideo".
public static void playRawVideo(Context context, VideoView videoView, String resName) {
try {
// ID of video file.
int id = VideoViewUtils.getRawResIdByName( context, resName);
Uri uri = Uri.parse("android.resource://" + context.getPackageName() + "/" + id);
Log.i(LOG_TAG, "Video URI: "+ uri);
videoView.setVideoURI(uri);
videoView.requestFocus();
} catch (Exception e) {
Log.e(LOG_TAG, "Error Play Raw Video: "+e.getMessage());
Toast.makeText(context,"Error Play Raw Video: "+ e.getMessage(),Toast.LENGTH_SHORT).show();
e.printStackTrace();
}
}
// @localPath = "/storage/emulated/0/DCIM/Camera/VID_20180212_195520.mp4"; (For example).
public static void playLocalVideo(Context context, VideoView videoView, String localPath) {
try {
} catch(Exception e) {
Log.e(LOG_TAG, "Error Play Local Video: "+ e.getMessage());
Toast.makeText(context,"Error Play Local Video: "+ e.getMessage(),Toast.LENGTH_SHORT).show();
e.printStackTrace();
}
}
// String videoURL = "https://ex1.o7planning.com/_testdatas_/mov_bbb.mp4";
// String videoURL = "https://www.radiantmediaplayer.com/media/bbb-360p.mp4";
public static void playURLVideo(Context context, VideoView videoView, String videoURL) {
try {
Log.i(LOG_TAG, "Video URL: "+ videoURL);
Uri uri= Uri.parse( videoURL );
videoView.setVideoURI(uri);
videoView.requestFocus();
} catch(Exception e) {
Log.e(LOG_TAG, "Error Play URL Video: "+ e.getMessage());
Toast.makeText(context,"Error Play URL Video: "+ e.getMessage(),Toast.LENGTH_SHORT).show();
e.printStackTrace();
}
}
// Find ID corresponding to the name of the resource (in the directory RAW).
public static int getRawResIdByName(Context context, String resName) {
String pkgName = context.getPackageName();
// Return 0 if not found.
int resID = context.getResources().getIdentifier(resName, "raw", pkgName);
Log.i(LOG_TAG, "Res Name: " + resName + "==> Res ID = " + resID);
return resID;
}
}
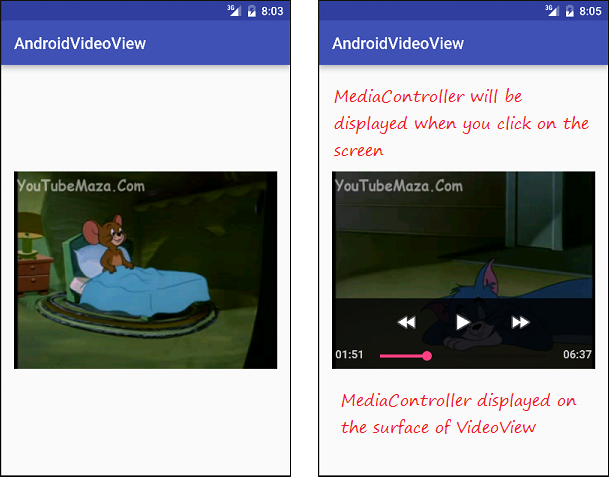
Quelques remarques de l'utilisation du code:
Imaginez quand vous utilisez setAnchorView() pour attacher MediaController à VideoView:
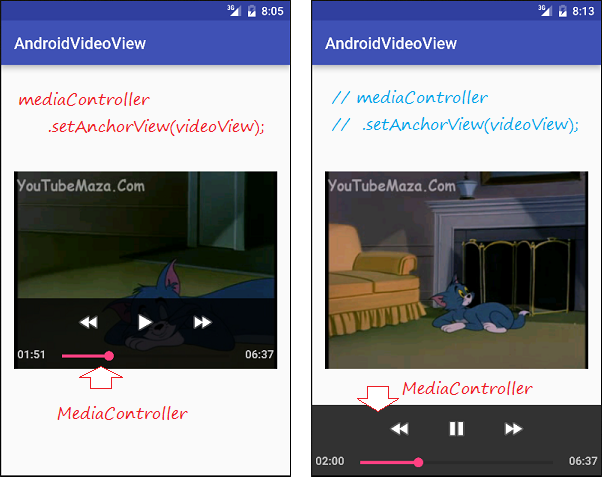
Tutoriels de programmation Android
- Configurer Android Emulator en Android Studio
- Le Tutoriel de Android ToggleButton
- Créer un File Finder Dialog simple dans Android
- Le Tutoriel de Android TimePickerDialog
- Le Tutoriel de Android DatePickerDialog
- De quoi avez-vous besoin pour démarrer avec Android?
- Installer Android Studio sur Windows
- Installer Intel® HAXM pour Android Studio
- Le Tutoriel de Android AsyncTask
- Le Tutoriel de Android AsyncTaskLoader
- Tutoriel Android pour débutant - Exemples de base
- Comment connaître le numéro de téléphone d'Android Emulator et le changer?
- Le Tutoriel de Android TextInputLayout
- Le Tutoriel de Android CardView
- Le Tutoriel de Android ViewPager2
- Obtenir un numéro de téléphone dans Android à l'aide de TelephonyManager
- Le Tutoriel de Android Phone Call
- Le Tutoriel de Android Wifi Scanning
- Le Tutoriel de programmation de jeux Android 2D pour débutant
- Le Tutoriel de Android DialogFragment
- Le Tutoriel de Android CharacterPickerDialog
- Le Tutoriel Android pour débutant - Hello Android
- Utiliser Android Device File Explorer
- Activer USB Debugging sur un appareil Android
- Le Tutoriel de Android UI Layouts
- Le Tutoriel de Android SMS
- Le Tutoriel de Android et SQLite Database
- Le Tutoriel de Google Maps Android API
- Le Tutoriel de texte pour parler dans Android
- Le Tutoriel de Android Space
- Le Tutoriel de Android Toast
- Créer un Android Toast personnalisé
- Le Tutoriel de Android SnackBar
- Le Tutoriel de Android TextView
- Le Tutoriel de Android TextClock
- Le Tutoriel de Android EditText
- Le Tutoriel de Android TextWatcher
- Formater le numéro de carte de crédit avec Android TextWatcher
- Le Tutoriel de Android Clipboard
- Créer un File Chooser simple dans Android
- Le Tutoriel de Android AutoCompleteTextView et MultiAutoCompleteTextView
- Le Tutoriel de Android ImageView
- Le Tutoriel de Android ImageSwitcher
- Le Tutoriel de Android ScrollView et HorizontalScrollView
- Le Tutoriel de Android WebView
- Le Tutoriel de Android SeekBar
- Le Tutoriel de Android Dialog
- Le Tutoriel de Android AlertDialog
- Tutoriel Android RatingBar
- Le Tutoriel de Android ProgressBar
- Le Tutoriel de Android Spinner
- Le Tutoriel de Android Button
- Le Tutoriel de Android Switch
- Le Tutoriel de Android ImageButton
- Le Tutoriel de Android FloatingActionButton
- Le Tutoriel de Android CheckBox
- Le Tutoriel de Android RadioGroup et RadioButton
- Le Tutoriel de Android Chip et ChipGroup
- Utilisation des Image assets et des Icon assets d'Android Studio
- Configuration de la Carte SD pour Android Emulator
- Exemple ChipGroup et Chip Entry
- Comment ajouter des bibliothèques externes à Android Project dans Android Studio?
- Comment désactiver les autorisations déjà accordées à l'application Android?
- Comment supprimer des applications de Android Emulator?
- Le Tutoriel de Android LinearLayout
- Le Tutoriel de Android TableLayout
- Le Tutoriel de Android FrameLayout
- Le Tutoriel de Android QuickContactBadge
- Le Tutoriel de Android StackView
- Le Tutoriel de Android Camera
- Le Tutoriel de Android MediaPlayer
- Le Tutoriel de Android VideoView
- Jouer des effets sonores dans Android avec SoundPool
- Le Tutoriel de Android Networking
- Analyser JSON dans Android
- Le Tutoriel de Android SharedPreferences
- Le Tutorial de stockage interne Android (Internal Storage)
- Le Tutoriel de Android External Storage
- Le Tutoriel de Android Intents
- Exemple d'une Android Intent explicite, appelant une autre Intent
- Exemple de Android Intent implicite, ouvrez une URL, envoyez un email
- Le Tutoriel de Android Service
- Le Tutoriel Android Notifications
- Le Tutoriel de Android DatePicker
- Le Tutoriel de Android TimePicker
- Le Tutoriel de Android Chronometer
- Le Tutoriel de Android OptionMenu
- Le Tutoriel de Android ContextMenu
- Le Tutoriel de Android PopupMenu
- Le Tutoriel de Android Fragment
- Le Tutoriel de Android ListView
- Android ListView avec Checkbox en utilisant ArrayAdapter
- Le Tutoriel de Android GridView
Show More