Exemple de Android Intent implicite, ouvrez une URL, envoyez un email
2. L'exemple simple avec Intent implicite
Cet exemple simple, lorsque vous cliquez sur un Button qui nécessite de voir le site Web par URL, vous créez Intent implicite, une Intent a été envoyée au système Android pour décider quels composants seront ouverts, peut-être que votre équipement installe de nombreux navigateurs différents (Firefox, Chrome, ...), l'appareil l'ouvrira dans votre navigateur par défaut ou votre navigateur préféré.
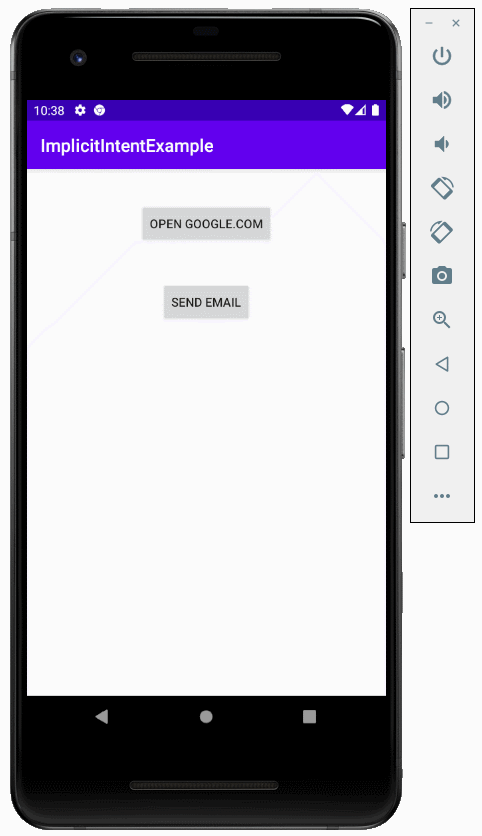
En plus, les exemples incluent également:
- Envoy un e-mail
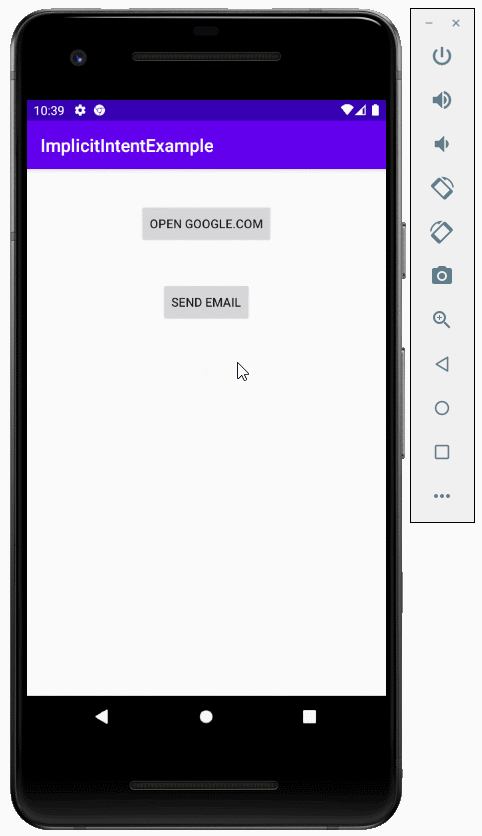
Note: The Android system will try to find its best application to send email. On the emulator, there may not be a good emailing application.
Créez un nouveau projet Android nommé "ImplicitIntentExample".
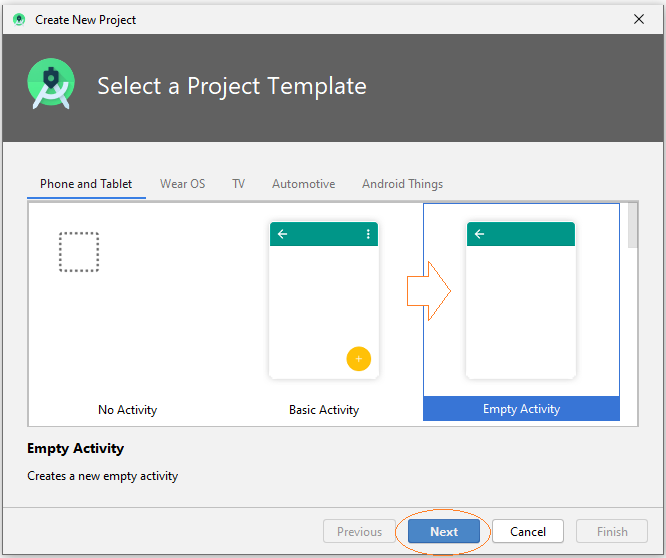
- Name: ImplicitIntentExample
- Package name: org.o7planning.implicitintentexample
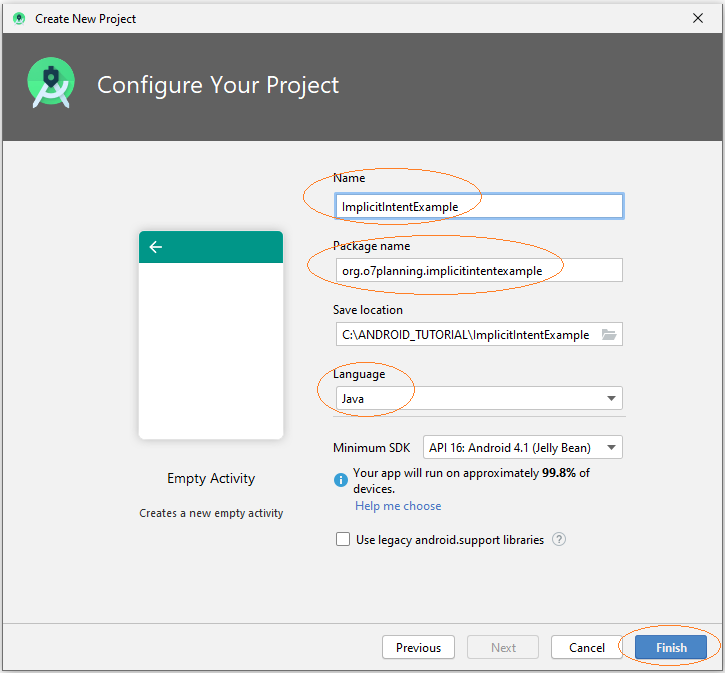
The interface of the application:
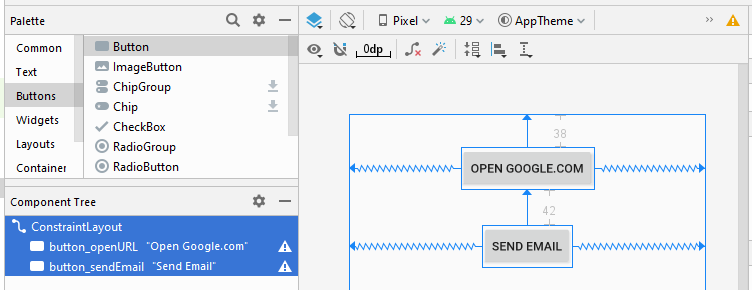
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button_openURL"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="38dp"
android:text="Open Google.com"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button_sendEmail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="42dp"
android:text="Send Email"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_openURL" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.implicitintentexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.content.Intent;
import android.net.Uri;
import android.view.View;
import android.widget.Button;
public class MainActivity extends AppCompatActivity {
private Button buttonOpenURL;
private Button buttonSendEmail;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.buttonOpenURL = (Button) this.findViewById(R.id.button_openURL);
this.buttonSendEmail = (Button) this.findViewById(R.id.button_sendEmail);
this.buttonOpenURL.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
openURL();
}
});
this.buttonSendEmail.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
sendEmail();
}
});
}
// The method is called when the user clicks on "Open google.com" button.
public void openURL( ) {
String url="https://google.com";
// An implicit intent, request a URL.
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse(url));
this.startActivity(intent);
}
// The method is called when the user clicks on "Send Email" button.
public void sendEmail( ) {
// List of recipients
String[] recipients=new String[]{"friendemail@gmail.com"};
String subject="Hi, how are you!";
String content ="This is my test email";
Intent intentEmail = new Intent(Intent.ACTION_SEND, Uri.parse("mailto:"));
intentEmail.putExtra(Intent.EXTRA_EMAIL, recipients);
intentEmail.putExtra(Intent.EXTRA_SUBJECT, subject);
intentEmail.putExtra(Intent.EXTRA_TEXT, content);
intentEmail.setType("text/plain");
startActivity(Intent.createChooser(intentEmail, "Choose an email client from..."));
}
}
Tutoriels de programmation Android
- Configurer Android Emulator en Android Studio
- Le Tutoriel de Android ToggleButton
- Créer un File Finder Dialog simple dans Android
- Le Tutoriel de Android TimePickerDialog
- Le Tutoriel de Android DatePickerDialog
- De quoi avez-vous besoin pour démarrer avec Android?
- Installer Android Studio sur Windows
- Installer Intel® HAXM pour Android Studio
- Le Tutoriel de Android AsyncTask
- Le Tutoriel de Android AsyncTaskLoader
- Tutoriel Android pour débutant - Exemples de base
- Comment connaître le numéro de téléphone d'Android Emulator et le changer?
- Le Tutoriel de Android TextInputLayout
- Le Tutoriel de Android CardView
- Le Tutoriel de Android ViewPager2
- Obtenir un numéro de téléphone dans Android à l'aide de TelephonyManager
- Le Tutoriel de Android Phone Call
- Le Tutoriel de Android Wifi Scanning
- Le Tutoriel de programmation de jeux Android 2D pour débutant
- Le Tutoriel de Android DialogFragment
- Le Tutoriel de Android CharacterPickerDialog
- Le Tutoriel Android pour débutant - Hello Android
- Utiliser Android Device File Explorer
- Activer USB Debugging sur un appareil Android
- Le Tutoriel de Android UI Layouts
- Le Tutoriel de Android SMS
- Le Tutoriel de Android et SQLite Database
- Le Tutoriel de Google Maps Android API
- Le Tutoriel de texte pour parler dans Android
- Le Tutoriel de Android Space
- Le Tutoriel de Android Toast
- Créer un Android Toast personnalisé
- Le Tutoriel de Android SnackBar
- Le Tutoriel de Android TextView
- Le Tutoriel de Android TextClock
- Le Tutoriel de Android EditText
- Le Tutoriel de Android TextWatcher
- Formater le numéro de carte de crédit avec Android TextWatcher
- Le Tutoriel de Android Clipboard
- Créer un File Chooser simple dans Android
- Le Tutoriel de Android AutoCompleteTextView et MultiAutoCompleteTextView
- Le Tutoriel de Android ImageView
- Le Tutoriel de Android ImageSwitcher
- Le Tutoriel de Android ScrollView et HorizontalScrollView
- Le Tutoriel de Android WebView
- Le Tutoriel de Android SeekBar
- Le Tutoriel de Android Dialog
- Le Tutoriel de Android AlertDialog
- Tutoriel Android RatingBar
- Le Tutoriel de Android ProgressBar
- Le Tutoriel de Android Spinner
- Le Tutoriel de Android Button
- Le Tutoriel de Android Switch
- Le Tutoriel de Android ImageButton
- Le Tutoriel de Android FloatingActionButton
- Le Tutoriel de Android CheckBox
- Le Tutoriel de Android RadioGroup et RadioButton
- Le Tutoriel de Android Chip et ChipGroup
- Utilisation des Image assets et des Icon assets d'Android Studio
- Configuration de la Carte SD pour Android Emulator
- Exemple ChipGroup et Chip Entry
- Comment ajouter des bibliothèques externes à Android Project dans Android Studio?
- Comment désactiver les autorisations déjà accordées à l'application Android?
- Comment supprimer des applications de Android Emulator?
- Le Tutoriel de Android LinearLayout
- Le Tutoriel de Android TableLayout
- Le Tutoriel de Android FrameLayout
- Le Tutoriel de Android QuickContactBadge
- Le Tutoriel de Android StackView
- Le Tutoriel de Android Camera
- Le Tutoriel de Android MediaPlayer
- Le Tutoriel de Android VideoView
- Jouer des effets sonores dans Android avec SoundPool
- Le Tutoriel de Android Networking
- Analyser JSON dans Android
- Le Tutoriel de Android SharedPreferences
- Le Tutorial de stockage interne Android (Internal Storage)
- Le Tutoriel de Android External Storage
- Le Tutoriel de Android Intents
- Exemple d'une Android Intent explicite, appelant une autre Intent
- Exemple de Android Intent implicite, ouvrez une URL, envoyez un email
- Le Tutoriel de Android Service
- Le Tutoriel Android Notifications
- Le Tutoriel de Android DatePicker
- Le Tutoriel de Android TimePicker
- Le Tutoriel de Android Chronometer
- Le Tutoriel de Android OptionMenu
- Le Tutoriel de Android ContextMenu
- Le Tutoriel de Android PopupMenu
- Le Tutoriel de Android Fragment
- Le Tutoriel de Android ListView
- Android ListView avec Checkbox en utilisant ArrayAdapter
- Le Tutoriel de Android GridView
Show More