Le Tutoriel de Android Networking
1. La vue d'ensemble du réseau Android
Sur Android, la programmation réseau inclut une requête au serveur et récupère les données renvoyées. En principe, vous avez deux API pour travailler avec le réseau:
Apache HttpClient:
- C'est une bibliothèque de source ouverte fournie par Apache.
HttpURLConnection
- C'est une API officielle d'Android, elle a commencé à être incluse dans la version d'Android 2.3, dans le précédent, Android utilisant ApacheHttpClient pour fonctionner avec le réseau.
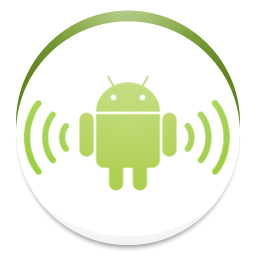
Vous devez accorder certaines autorisations à l'application si vous souhaitez travailler avec le réseau:
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
android.permission.INTERNET:
- Ajoutez cette autorisation, cela permet à votre application de pouvoir vous connecter au réseau.
android.permission.ACCESS_NETWORK_STATE:
- Permet à l'application de vérifier l'étatde la connexion de votre réseau.
Le code ci- dessous vérifie l'état de connexion du réseau:
private boolean checkInternetConnection() {
ConnectivityManager connManager =
(ConnectivityManager) this.getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = connManager.getActiveNetworkInfo();
if (networkInfo == null) {
Toast.makeText(this, "No default network is currently active", Toast.LENGTH_LONG).show();
return false;
}
if (!networkInfo.isConnected()) {
Toast.makeText(this, "Network is not connected", Toast.LENGTH_LONG).show();
return false;
}
if (!networkInfo.isAvailable()) {
Toast.makeText(this, "Network not available", Toast.LENGTH_LONG).show();
return false;
}
Toast.makeText(this, "Network OK", Toast.LENGTH_LONG).show();
return true;
}
2. NetworkOnMainThreadException
Par défaut, lorsque vous travaillez avec un réseau (network) dans Android, vous devez créer un nouveau thread pour envoyer et recevoir les données renvoyées. Si vous travaillez sur le thread principal, vous obtiendrez android.os.NetworkOnMainThreadException, c'est la politique par défaut d'Android. Cependant, vous pouvez remplacer cette politique d'Android pour pouvoir travailler avec le réseau sur le thread principal.
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
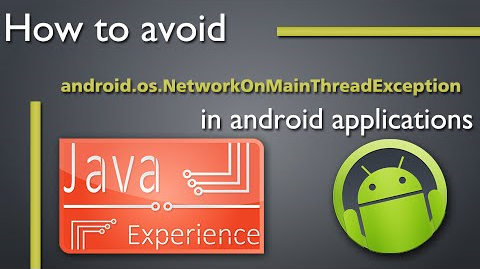
Il est recommandé de créer une classe s'étendant à partir de AsyncTask<Params, Progress, Result>, cette classe n'est pas un thread, elle s'étend d'Object, mais quand faire leur tâche (Appel la méthode AsyncTask.execute (params)), elle crée un nouveau thread pour exécuter la méthode doInBackground(params). Une fois le thread est terminé, on appellera la méthode onPostExecute(result).
Vous pouvez observer l'exemple ci- dessus:
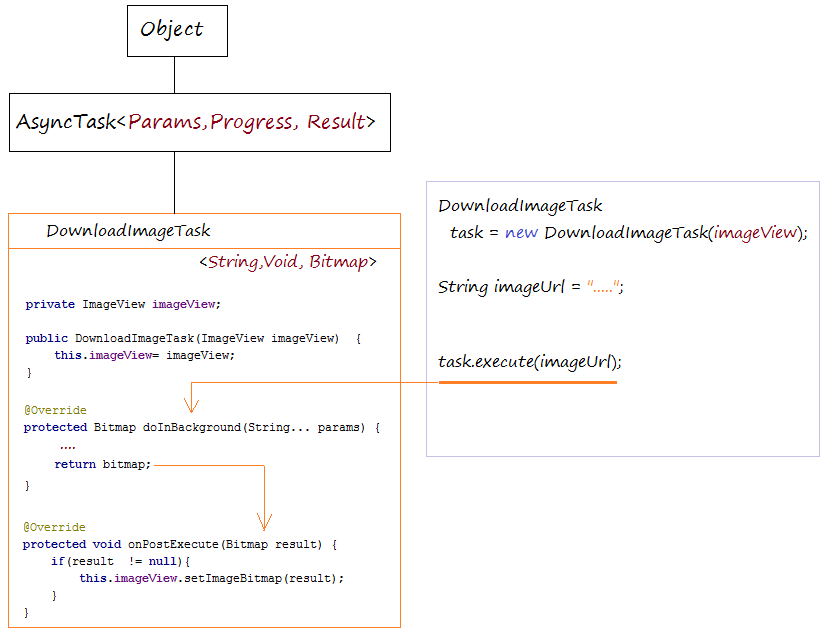
3. Exemmple de l'utilisation HttpURLConnection
Dans cet exemple, vous téléchargez l'image et Json à partir de URL et les exposer sur ImageView et TextView.
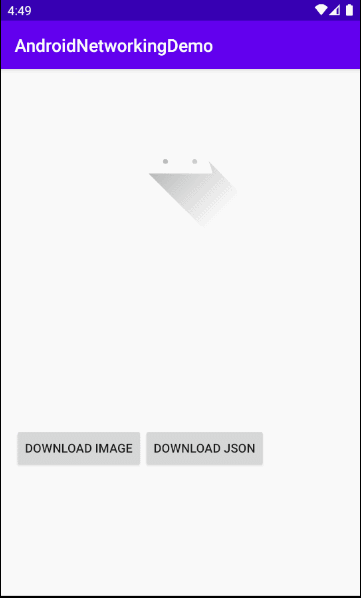
Créez le projet AndroidNetworkingDemo:
- File > New > New Project > Empty Activity
- Name: AndroidNetworkingDemo
- Package name: org.o7planning.androidnetworkingdemo
- Language: Java
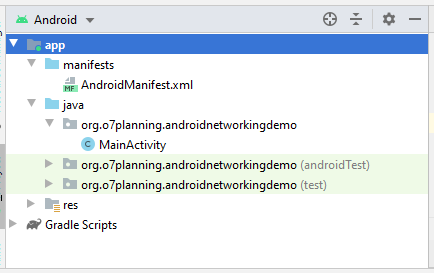
Vous devez accorder des autorisations à l'application pour accéder à Internet et l'autorisation de vérifier l'état du réseau.
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
Ajputez le code à AndroidManifest.xml:
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.o7planning.androidnetworkingdemo">
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
L'interface de l'application:
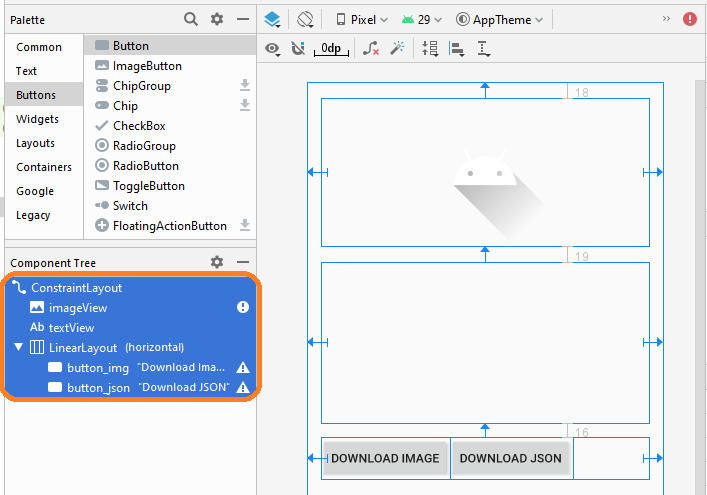
If you are interested in the steps to design this application interface, please see the appendix at the end of the article.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ImageView
android:id="@+id/imageView"
android:layout_width="0dp"
android:layout_height="171dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="18dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:contentDescription="Image"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:srcCompat="@drawable/ic_launcher_foreground" />
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="186dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="19dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView" />
<LinearLayout
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView">
<Button
android:id="@+id/button_img"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Download Image" />
<Button
android:id="@+id/button_json"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Download JSON" />
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
Code:
IOUtils.java
package org.o7planning.androidnetworkingdemo;
import java.io.InputStream;
import java.io.Reader;
public class IOUtils {
public static void closeQuietly(InputStream in) {
try {
in.close();
}catch (Exception e) {
}
}
public static void closeQuietly(Reader reader) {
try {
reader.close();
}catch (Exception e) {
}
}
}
DownloadJsonTask.java
package org.o7planning.androidnetworkingdemo;
import android.os.AsyncTask;
import android.util.Log;
import android.widget.TextView;
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
// A task with String input parameter, and returns the result as String.
public class DownloadJsonTask
// AsyncTask<Params, Progress, Result>
extends AsyncTask<String, Void, String> {
private TextView textView;
public DownloadJsonTask(TextView textView) {
this.textView= textView;
}
@Override
protected String doInBackground(String... params) {
String textUrl = params[0];
InputStream in = null;
BufferedReader br= null;
try {
URL url = new URL(textUrl);
HttpURLConnection httpConn = (HttpURLConnection) url.openConnection();
httpConn.setAllowUserInteraction(false);
httpConn.setInstanceFollowRedirects(true);
httpConn.setRequestMethod("GET");
httpConn.connect();
int resCode = httpConn.getResponseCode();
if (resCode == HttpURLConnection.HTTP_OK) {
in = httpConn.getInputStream();
br= new BufferedReader(new InputStreamReader(in));
StringBuilder sb= new StringBuilder();
String s= null;
while((s= br.readLine())!= null) {
sb.append(s);
sb.append("\n");
}
return sb.toString();
} else {
return null;
}
} catch (Exception e) {
e.printStackTrace();
} finally {
IOUtils.closeQuietly(in);
IOUtils.closeQuietly(br);
}
return null;
}
// When the task is completed, this method will be called
// Download complete. Lets update UI
@Override
protected void onPostExecute(String result) {
if(result != null){
this.textView.setText(result);
} else{
Log.e("MyMessage", "Failed to fetch data!");
}
}
}
DownloadImageTask.java
package org.o7planning.androidnetworkingdemo;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.AsyncTask;
import android.util.Log;
import android.widget.ImageView;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
// A task with String input parameter, and returns the result as bitmap.
public class DownloadImageTask
// AsyncTask<Params, Progress, Result>
extends AsyncTask<String, Void, Bitmap> {
private ImageView imageView;
public DownloadImageTask(ImageView imageView) {
this.imageView= imageView;
}
@Override
protected Bitmap doInBackground(String... params) {
String imageUrl = params[0];
InputStream in = null;
try {
URL url = new URL(imageUrl);
HttpURLConnection httpConn = (HttpURLConnection) url.openConnection();
httpConn.setAllowUserInteraction(false);
httpConn.setInstanceFollowRedirects(true);
httpConn.setRequestMethod("GET");
httpConn.connect();
int resCode = httpConn.getResponseCode();
if (resCode == HttpURLConnection.HTTP_OK) {
in = httpConn.getInputStream();
} else {
return null;
}
Bitmap bitmap = BitmapFactory.decodeStream(in);
return bitmap;
} catch (Exception e) {
e.printStackTrace();
} finally {
IOUtils.closeQuietly(in);
}
return null;
}
// When the task is completed, this method will be called
// Download complete. Lets update UI
@Override
protected void onPostExecute(Bitmap result) {
if(result != null){
this.imageView.setImageBitmap(result);
} else{
Log.e("MyMessage", "Failed to fetch data!");
}
}
}
MainActivity.java
package org.o7planning.androidnetworkingdemo;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.content.Context;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private ImageView imageView;
private TextView textView;
private Button buttonImg;
private Button buttonJson;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.imageView = (ImageView) this.findViewById(R.id.imageView);
this.textView = (TextView) this.findViewById(R.id.textView);
this.buttonImg = (Button) this.findViewById(R.id.button_img);
this.buttonJson = (Button) this.findViewById(R.id.button_json);
this.buttonImg.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
downloadAndShowImage(v);
}
});
this.buttonJson.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
downloadAndShowJson(v);
}
});
}
private boolean checkInternetConnection() {
// Get Connectivity Manager
ConnectivityManager connManager =
(ConnectivityManager) this.getSystemService(Context.CONNECTIVITY_SERVICE);
// Details about the currently active default data network
NetworkInfo networkInfo = connManager.getActiveNetworkInfo();
if (networkInfo == null) {
Toast.makeText(this, "No default network is currently active", Toast.LENGTH_LONG).show();
return false;
}
if (!networkInfo.isConnected()) {
Toast.makeText(this, "Network is not connected", Toast.LENGTH_LONG).show();
return false;
}
if (!networkInfo.isAvailable()) {
Toast.makeText(this, "Network not available", Toast.LENGTH_LONG).show();
return false;
}
Toast.makeText(this, "Network OK", Toast.LENGTH_LONG).show();
return true;
}
// When user click on the "Download Image".
public void downloadAndShowImage(View view) {
boolean networkOK = this.checkInternetConnection();
if (!networkOK) {
return;
}
String imageUrl = "https://o7planning.org/download/static/default/demo-data/logo.png";
// Create a task to download and display image.
DownloadImageTask task = new DownloadImageTask(this.imageView);
// Execute task (Pass imageUrl).
task.execute(imageUrl);
}
// When user click on the "Download Json".
public void downloadAndShowJson(View view) {
boolean networkOK = this.checkInternetConnection();
if (!networkOK) {
return;
}
String jsonUrl = "https://o7planning.org/download/static/default/demo-data/company.json";
// Create a task to download and display json content.
DownloadJsonTask task = new DownloadJsonTask(this.textView);
// Execute task (Pass jsonUrl).
task.execute(jsonUrl);
}
}
Tutoriels de programmation Android
- Configurer Android Emulator en Android Studio
- Le Tutoriel de Android ToggleButton
- Créer un File Finder Dialog simple dans Android
- Le Tutoriel de Android TimePickerDialog
- Le Tutoriel de Android DatePickerDialog
- De quoi avez-vous besoin pour démarrer avec Android?
- Installer Android Studio sur Windows
- Installer Intel® HAXM pour Android Studio
- Le Tutoriel de Android AsyncTask
- Le Tutoriel de Android AsyncTaskLoader
- Tutoriel Android pour débutant - Exemples de base
- Comment connaître le numéro de téléphone d'Android Emulator et le changer?
- Le Tutoriel de Android TextInputLayout
- Le Tutoriel de Android CardView
- Le Tutoriel de Android ViewPager2
- Obtenir un numéro de téléphone dans Android à l'aide de TelephonyManager
- Le Tutoriel de Android Phone Call
- Le Tutoriel de Android Wifi Scanning
- Le Tutoriel de programmation de jeux Android 2D pour débutant
- Le Tutoriel de Android DialogFragment
- Le Tutoriel de Android CharacterPickerDialog
- Le Tutoriel Android pour débutant - Hello Android
- Utiliser Android Device File Explorer
- Activer USB Debugging sur un appareil Android
- Le Tutoriel de Android UI Layouts
- Le Tutoriel de Android SMS
- Le Tutoriel de Android et SQLite Database
- Le Tutoriel de Google Maps Android API
- Le Tutoriel de texte pour parler dans Android
- Le Tutoriel de Android Space
- Le Tutoriel de Android Toast
- Créer un Android Toast personnalisé
- Le Tutoriel de Android SnackBar
- Le Tutoriel de Android TextView
- Le Tutoriel de Android TextClock
- Le Tutoriel de Android EditText
- Le Tutoriel de Android TextWatcher
- Formater le numéro de carte de crédit avec Android TextWatcher
- Le Tutoriel de Android Clipboard
- Créer un File Chooser simple dans Android
- Le Tutoriel de Android AutoCompleteTextView et MultiAutoCompleteTextView
- Le Tutoriel de Android ImageView
- Le Tutoriel de Android ImageSwitcher
- Le Tutoriel de Android ScrollView et HorizontalScrollView
- Le Tutoriel de Android WebView
- Le Tutoriel de Android SeekBar
- Le Tutoriel de Android Dialog
- Le Tutoriel de Android AlertDialog
- Tutoriel Android RatingBar
- Le Tutoriel de Android ProgressBar
- Le Tutoriel de Android Spinner
- Le Tutoriel de Android Button
- Le Tutoriel de Android Switch
- Le Tutoriel de Android ImageButton
- Le Tutoriel de Android FloatingActionButton
- Le Tutoriel de Android CheckBox
- Le Tutoriel de Android RadioGroup et RadioButton
- Le Tutoriel de Android Chip et ChipGroup
- Utilisation des Image assets et des Icon assets d'Android Studio
- Configuration de la Carte SD pour Android Emulator
- Exemple ChipGroup et Chip Entry
- Comment ajouter des bibliothèques externes à Android Project dans Android Studio?
- Comment désactiver les autorisations déjà accordées à l'application Android?
- Comment supprimer des applications de Android Emulator?
- Le Tutoriel de Android LinearLayout
- Le Tutoriel de Android TableLayout
- Le Tutoriel de Android FrameLayout
- Le Tutoriel de Android QuickContactBadge
- Le Tutoriel de Android StackView
- Le Tutoriel de Android Camera
- Le Tutoriel de Android MediaPlayer
- Le Tutoriel de Android VideoView
- Jouer des effets sonores dans Android avec SoundPool
- Le Tutoriel de Android Networking
- Analyser JSON dans Android
- Le Tutoriel de Android SharedPreferences
- Le Tutorial de stockage interne Android (Internal Storage)
- Le Tutoriel de Android External Storage
- Le Tutoriel de Android Intents
- Exemple d'une Android Intent explicite, appelant une autre Intent
- Exemple de Android Intent implicite, ouvrez une URL, envoyez un email
- Le Tutoriel de Android Service
- Le Tutoriel Android Notifications
- Le Tutoriel de Android DatePicker
- Le Tutoriel de Android TimePicker
- Le Tutoriel de Android Chronometer
- Le Tutoriel de Android OptionMenu
- Le Tutoriel de Android ContextMenu
- Le Tutoriel de Android PopupMenu
- Le Tutoriel de Android Fragment
- Le Tutoriel de Android ListView
- Android ListView avec Checkbox en utilisant ArrayAdapter
- Le Tutoriel de Android GridView
Show More