Le Tutorial de stockage interne Android (Internal Storage)
1. Android Internal Storage
Android Internal Storage: Un lieu de stockage de données privées de chaque application, ces données sont stockées et utilisées pour leur propre application. D'autres applications ne peuvent y accéder. Normalement, lorsque l'application est supprimée dans des appareils Android, le fichier de données associé est également supprimé.
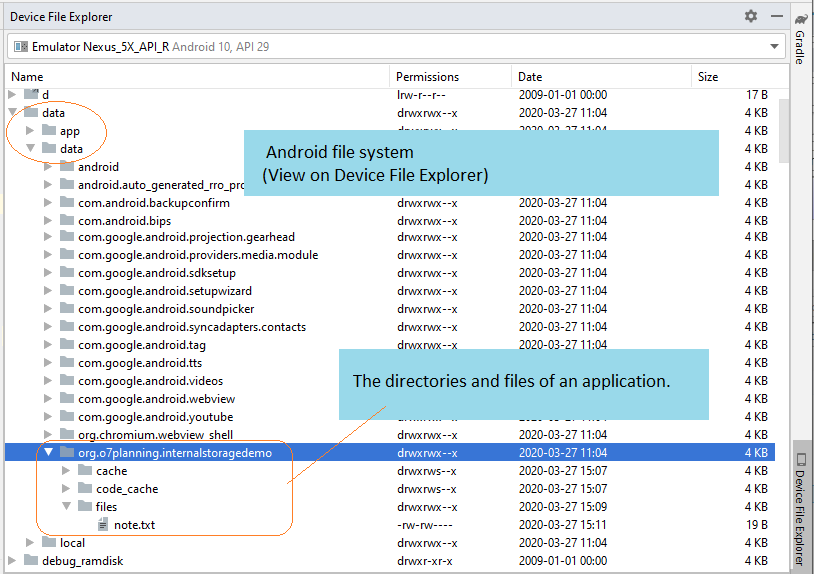
Une autre fonctionnalité lorsque vous travaillez avec des fichiers dans le stockage interne est que vous ne pouvez travailler avec un nom de fichier simple et ne pouvez pas travailler avec un nom de fichier qui a le chemin.
Ouvrir le fichier pour écire des données:
// Is a simple file name.
// Note!! Do not allow the path.
String simpleFileName ="note.txt";
// Open Stream to write file.
FileOutputStream out = openFileOutput(simpleFileName, MODE_PRIVATE);
Vous avez 4 manières d'écriture:
Mode de création de fichier | Description |
MODE_PRIVATE | Le mode par défaut, où le fichier créé ne peut être consulté que par l'application appelante (ou toutes les applications partageant la même ID utilisateur). |
MODE_APPEND | Le mode des données ajoutées au fichier s'il existe déjà. |
MODE_ENABLE_WRITE_AHEAD_LOGGING | |
MODE_WORLD_READABLE | Ces modes sont très dangereux, c'est comme un trou de sécurité dans Android, il est préférable de ne pas utiliser, vous pouvez utiliser des techniques alternatives telles que:
|
MODE_WORLD_WRITEABLE | Ces modes sont très dangereux, c'est comme un trou de sécurité dans Android, il est préférable de ne pas utiliser, vous pouvez utiliser des techniques alternatives telles que:
|
MODE_MULTI_PROCESS | Ce mode permet de créer plusieurs processus dans le fichier. Cependant, il est recommandé de ne pas utiliser ce mode car il ne fonctionne pas sur certaines versions d'Android. Vous pouvez utiliser d'autres techniques, par exemple:
|
Ouvriez le fichier pour lire des données:
// Is a simple file name.
// Note!! Do not allow the path.
String simpleFileName = "note.txt";
// Open stream to read file.
FileInputStream in = this.openFileInput(simpleFileName);
2. La lecture et l'écriture des données dans l'exemple de stockage interne
Maintenant, vous pouvez faire un exemple d'écriture de données sur les fichiers stockés dans le stockage interne ( Internal Storage) et lire les données de ce fichier.
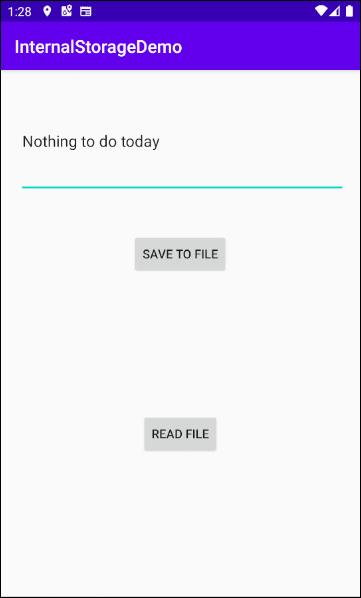
Créez un projet nommé InternalStorageDemo.
- File > New > New Project > Empty Activity
- Name: InternalStorageDemo
- Package name: org.o7planning.internalstoragedemo
- Language: Java
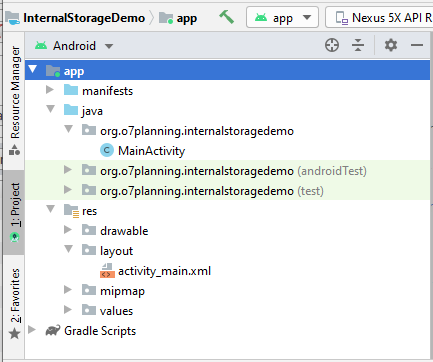
L'interface de l'application:
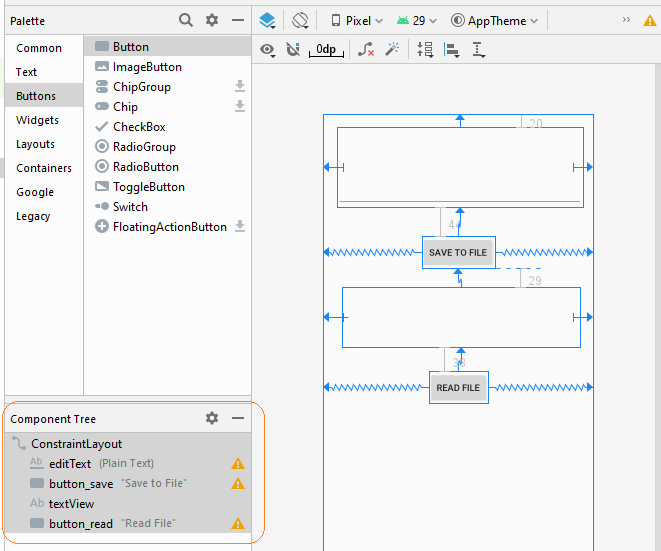
The interface of this application is very simple, if you are interested in the steps to create it, see the appendix at the end of the article.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editText"
android:layout_width="0dp"
android:layout_height="122dp"
android:layout_marginStart="21dp"
android:layout_marginLeft="21dp"
android:layout_marginTop="20dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="textPersonName"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button_save"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="44dp"
android:text="Save to File"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText" />
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="91dp"
android:layout_marginStart="29dp"
android:layout_marginLeft="29dp"
android:layout_marginTop="29dp"
android:layout_marginEnd="21dp"
android:layout_marginRight="21dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_save" />
<Button
android:id="@+id/button_read"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="38dp"
android:text="Read File"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.internalstoragedemo;
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.InputStreamReader;
public class MainActivity extends AppCompatActivity {
private Button saveButton;
private Button readButton;
private TextView textView;
private EditText editText;
// Is a simple file name.
// Note!! Do not allow the path.
private String simpleFileName = "note.txt";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.saveButton = (Button) this.findViewById(R.id.button_save);
this.readButton = (Button) this.findViewById(R.id.button_read);
this.textView = (TextView) this.findViewById(R.id.textView);
this.editText = (EditText) this.findViewById(R.id.editText);
this.saveButton.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
saveData();
}
});
this.readButton.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
readData();
}
});
}
private void saveData() {
String data = this.editText.getText().toString();
try {
// Open Stream to write file.
FileOutputStream out = this.openFileOutput(simpleFileName, MODE_PRIVATE);
// Ghi dữ liệu.
out.write(data.getBytes());
out.close();
Toast.makeText(this,"File saved!",Toast.LENGTH_SHORT).show();
} catch (Exception e) {
Toast.makeText(this,"Error:"+ e.getMessage(),Toast.LENGTH_SHORT).show();
}
}
private void readData() {
try {
// Open stream to read file.
FileInputStream in = this.openFileInput(simpleFileName);
BufferedReader br= new BufferedReader(new InputStreamReader(in));
StringBuilder sb= new StringBuilder();
String s= null;
while((s= br.readLine())!= null) {
sb.append(s).append("\n");
}
this.textView.setText(sb.toString());
} catch (Exception e) {
Toast.makeText(this,"Error:"+ e.getMessage(),Toast.LENGTH_SHORT).show();
}
}
}
Utlisez "Android Device Manager" vous pouvez voir les fichiers qui sont créés sur le système.
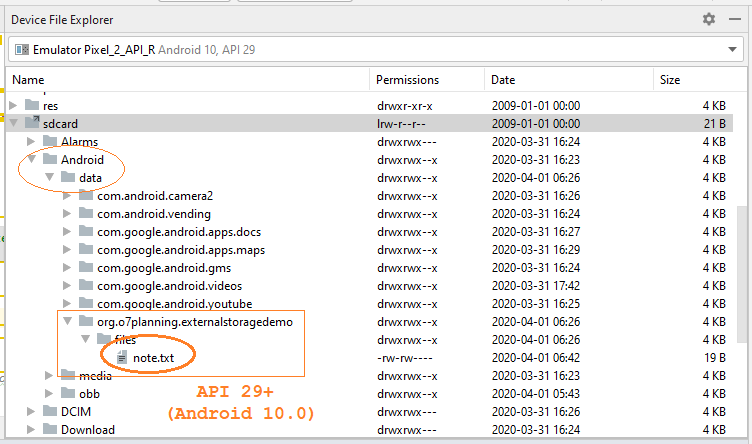
See more about "Device File Explorer":
Tutoriels de programmation Android
- De quoi avez-vous besoin pour démarrer avec Android?
- Installer Android Studio sur Windows
- Installer Intel® HAXM pour Android Studio
- Configurer Android Emulator en Android Studio
- Le Tutoriel Android pour débutant - Hello Android
- Tutoriel Android pour débutant - Exemples de base
- Utilisation des Image assets et des Icon assets d'Android Studio
- Utiliser Android Device File Explorer
- Activer USB Debugging sur un appareil Android
- Configuration de la Carte SD pour Android Emulator
- Comment connaître le numéro de téléphone d'Android Emulator et le changer?
- Comment ajouter des bibliothèques externes à Android Project dans Android Studio?
- Comment désactiver les autorisations déjà accordées à l'application Android?
- Comment supprimer des applications de Android Emulator?
- Le Tutoriel de Android UI Layouts
- Le Tutoriel de Android LinearLayout
- Le Tutoriel de Android TableLayout
- Le Tutoriel de Android FrameLayout
- Le Tutoriel de Android Button
- Le Tutoriel de Android ToggleButton
- Le Tutoriel de Android Switch
- Le Tutoriel de Android ImageButton
- Le Tutoriel de Android FloatingActionButton
- Le Tutoriel de Android CheckBox
- Le Tutoriel de Android RadioGroup et RadioButton
- Le Tutoriel de Android Chip et ChipGroup
- Exemple ChipGroup et Chip Entry
- Le Tutoriel de Android QuickContactBadge
- Le Tutoriel de Android Space
- Le Tutoriel de Android Toast
- Créer un Android Toast personnalisé
- Le Tutoriel de Android SnackBar
- Le Tutoriel de Android TextView
- Le Tutoriel de Android TextClock
- Le Tutoriel de Android EditText
- Le Tutoriel de Android TextInputLayout
- Le Tutoriel de Android TextWatcher
- Formater le numéro de carte de crédit avec Android TextWatcher
- Le Tutoriel de Android Clipboard
- Créer un File Chooser simple dans Android
- Créer un File Finder Dialog simple dans Android
- Le Tutoriel de Android AutoCompleteTextView et MultiAutoCompleteTextView
- Le Tutoriel de Android ImageView
- Le Tutoriel de Android ImageSwitcher
- Le Tutoriel de Android ScrollView et HorizontalScrollView
- Le Tutoriel de Android WebView
- Le Tutoriel de Android SeekBar
- Le Tutoriel de Android Dialog
- Le Tutoriel de Android AlertDialog
- Le Tutoriel de Android CharacterPickerDialog
- Le Tutoriel de Android DialogFragment
- Le Tutoriel de Android DatePicker
- Le Tutoriel de Android TimePicker
- Le Tutoriel de Android TimePickerDialog
- Le Tutoriel de Android DatePickerDialog
- Le Tutoriel de Android Chronometer
- Tutoriel Android RatingBar
- Le Tutoriel de Android ProgressBar
- Le Tutoriel de Android Spinner
- Le Tutoriel de Android OptionMenu
- Le Tutoriel de Android ContextMenu
- Le Tutoriel de Android PopupMenu
- Le Tutoriel de Android Fragment
- Le Tutoriel de Android ListView
- Android ListView avec Checkbox en utilisant ArrayAdapter
- Le Tutoriel de Android GridView
- Le Tutoriel de Android CardView
- Le Tutoriel de Android ViewPager2
- Le Tutoriel de Android StackView
- Le Tutoriel de Android Camera
- Le Tutoriel de Android MediaPlayer
- Le Tutoriel de Android VideoView
- Jouer des effets sonores dans Android avec SoundPool
- Le Tutoriel de Android Networking
- Analyser JSON dans Android
- Le Tutoriel de Android SharedPreferences
- Le Tutorial de stockage interne Android (Internal Storage)
- Le Tutoriel de Android External Storage
- Le Tutoriel de Android Intents
- Exemple d'une Android Intent explicite, appelant une autre Intent
- Exemple de Android Intent implicite, ouvrez une URL, envoyez un email
- Le Tutoriel de Android Service
- Le Tutoriel Android Notifications
- Le Tutoriel de Android et SQLite Database
- Le Tutoriel de Google Maps Android API
- Le Tutoriel de texte pour parler dans Android
- Le Tutoriel de Android AsyncTask
- Le Tutoriel de Android AsyncTaskLoader
- Obtenir un numéro de téléphone dans Android à l'aide de TelephonyManager
- Le Tutoriel de Android SMS
- Le Tutoriel de Android Phone Call
- Le Tutoriel de Android Wifi Scanning
- Le Tutoriel de programmation de jeux Android 2D pour débutant
Show More