Le Tutoriel de Android CheckBox
1. Android CheckBox
Sous Android, la CheckBox est un bouton avec deux états checked (cochés) et unchecked (non cochés), c'est un composant de base et est très souvent utilisé dans les applications Android.
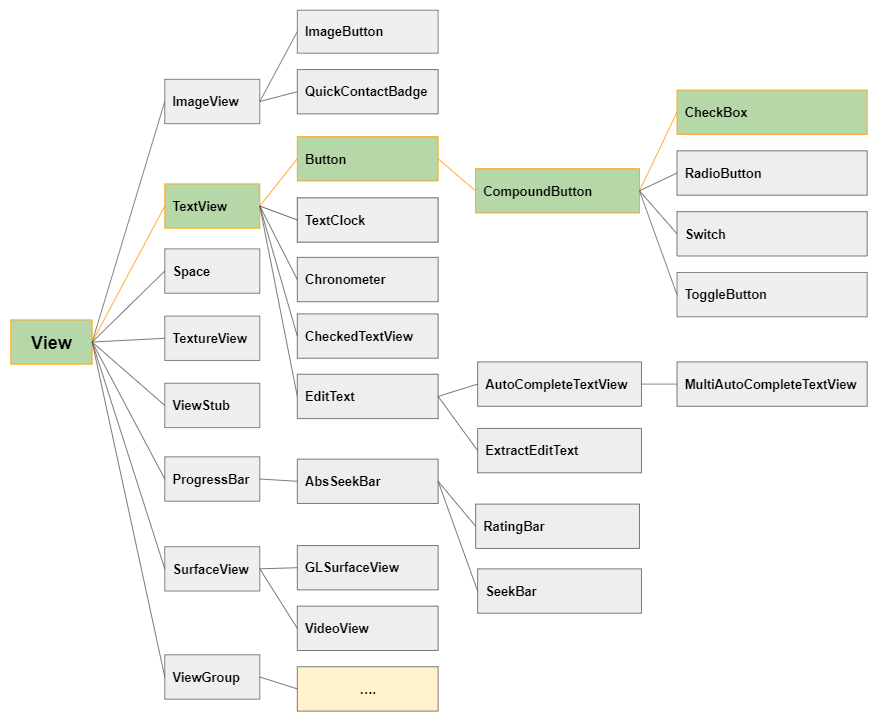
Fondamentalement, vous pouvez utiliser plusieurs CheckBox dans l'application pour permettre aux utilisateurs de sélectionner une ou plusieurs options dans un ensemble de valeurs.
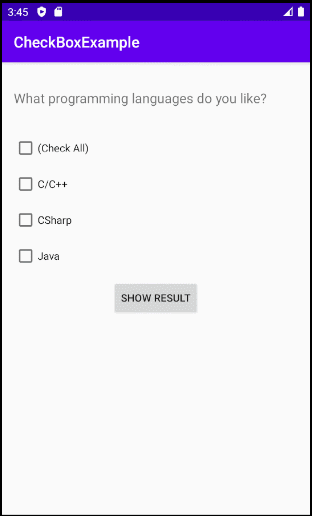
Par défaut, la CheckBox a un état unchecked, vous pouvez changer son état via la propriété android:checked.
<CheckBox
android:id="@+id/someId"
android:checked="true"
... />
Quelques propriétés importantes de CheckBox:
android:checked | Spécifiez l'état actuel de la CheckBox. |
android:gravity | Alignez (align) le texte de la CheckBox. Les valeurs possibles sont left, right, center, top, ... |
android:text | Définissez le contenu du text pour CheckBox. |
android:textColor | Définissez la couleur de police du texte. |
android:textSize | Définissez la taille de la police du texte. |
android:textStyle | Définir le style du texte (bold, italic, bolditalic). |
android:background | Définissez la couleur d'arrière-plan pour CheckBox. |
android:padding | Définir le padding pour CheckBox. |
android:onClick | Le nom de la méthode sera appelé lorsque l'utilisateur cliquera dessus CheckBox. |
toggle()
All 4 classes of ToggleButton, CheckBox, RadioButton, Switch are subclasses of CompoundButton, so they inherit the toggle() method, which is the commonly used method to change their state from Checked (ON) to Unchecked (OFF), and vice versa.
CompoundButton button = (CheckBox) findViewById(R.id.checkBox);
button.toggle();
2. CheckBox Events
Il existe un certain nombre d'événements liés à un CheckBox, mais les deux événements suivants sont utilisés le plus souvent:
- checkBox.setOnClickListener(View.OnClickListener)
- checkBox.setOnCheckedChangeListener(CompoundButton.OnCheckedChangeListener)
On Click Event:
L'événement se produit lorsque l'utilisateur clique (click) sur la CheckBox, tout comme l'action de l'utilisateur lorsqu'il clique sur un Bouton.
CheckBox chk = (CheckBox) findViewById(R.id.chk1);
chk.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
boolean checked = ((CheckBox) v).isChecked();
// Check which checkbox was clicked
if (checked){
// Your code
}
else{
// Your code
}
}
});
On Checked Change Event:
L'événement se produit lorsque la CheckBox change d'état, soit par l'action de l'utilisateur, soit par l'effet d'un appel de méthode checkBox.setChecked(newState), ..
CheckBox chk = (CheckBox) findViewById(R.id.chk1);
chk.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if(isChecked) {
// Your code
} else {
// Your code
}
}
});
3. Example de CheckBox
Exemple d'aperçu:
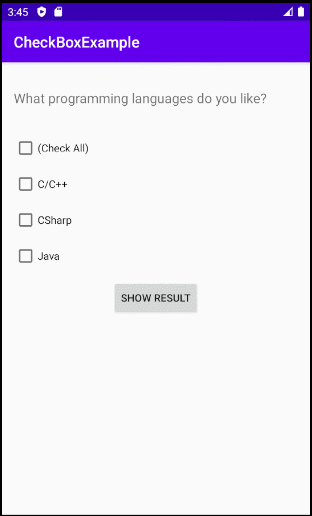
L'interface de l'exemple d'application:
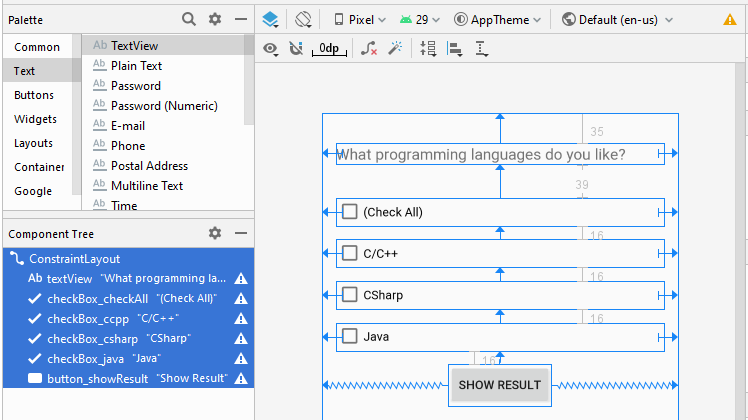
Remarque: Si vous êtes intéressé par les étapes de conception de l'interface de cette application, veuillez consulter l'annexe à la fin de l'article.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="35dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="What programming languages do you like?"
android:textSize="18sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<CheckBox
android:id="@+id/checkBox_checkAll"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="39dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="(Check All)"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<CheckBox
android:id="@+id/checkBox_ccpp"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="C/C++"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_checkAll" />
<CheckBox
android:id="@+id/checkBox_csharp"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="CSharp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_ccpp" />
<CheckBox
android:id="@+id/checkBox_java"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Java"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_csharp" />
<Button
android:id="@+id/button_showResult"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Show Result"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_java" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.checkboxexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.CompoundButton;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private CheckBox checkBoxCheckAll;
private CheckBox checkBoxCcpp;
private CheckBox checkBoxCsharp;
private CheckBox checkBoxJava;
private Button buttonShowResult;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.checkBoxCheckAll = (CheckBox) this.findViewById(R.id.checkBox_checkAll);
this.checkBoxCcpp = (CheckBox) this.findViewById(R.id.checkBox_ccpp);
this.checkBoxCsharp = (CheckBox) this.findViewById(R.id.checkBox_csharp);
this.checkBoxJava = (CheckBox) this.findViewById(R.id.checkBox_java);
this.buttonShowResult = (Button) this.findViewById(R.id.button_showResult);
this.buttonShowResult.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showResult();
}
});
this.checkBoxCheckAll.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
checkAllCheckedChange(isChecked);
}
});
}
private void showResult() {
String message = null;
if(this.checkBoxCcpp.isChecked()) {
message = this.checkBoxCcpp.getText().toString();
}
if(this.checkBoxCsharp.isChecked()) {
if(message== null) {
message = this.checkBoxCsharp.getText().toString();
} else {
message += ", " + this.checkBoxCsharp.getText().toString();
}
}
if(this.checkBoxJava.isChecked()) {
if(message== null) {
message = this.checkBoxJava.getText().toString();
} else {
message += ", " + this.checkBoxJava.getText().toString();
}
}
message = message == null? "You select nothing": "You select: " + message;
Toast.makeText(this, message, Toast.LENGTH_LONG).show();
}
// When "Check All" change state.
private void checkAllCheckedChange(boolean isChecked) {
this.checkBoxCsharp.setChecked(isChecked);
this.checkBoxCcpp.setChecked(isChecked);
this.checkBoxJava.setChecked(isChecked);
}
}
Tutoriels de programmation Android
- Configurer Android Emulator en Android Studio
- Le Tutoriel de Android ToggleButton
- Créer un File Finder Dialog simple dans Android
- Le Tutoriel de Android TimePickerDialog
- Le Tutoriel de Android DatePickerDialog
- De quoi avez-vous besoin pour démarrer avec Android?
- Installer Android Studio sur Windows
- Installer Intel® HAXM pour Android Studio
- Le Tutoriel de Android AsyncTask
- Le Tutoriel de Android AsyncTaskLoader
- Tutoriel Android pour débutant - Exemples de base
- Comment connaître le numéro de téléphone d'Android Emulator et le changer?
- Le Tutoriel de Android TextInputLayout
- Le Tutoriel de Android CardView
- Le Tutoriel de Android ViewPager2
- Obtenir un numéro de téléphone dans Android à l'aide de TelephonyManager
- Le Tutoriel de Android Phone Call
- Le Tutoriel de Android Wifi Scanning
- Le Tutoriel de programmation de jeux Android 2D pour débutant
- Le Tutoriel de Android DialogFragment
- Le Tutoriel de Android CharacterPickerDialog
- Le Tutoriel Android pour débutant - Hello Android
- Utiliser Android Device File Explorer
- Activer USB Debugging sur un appareil Android
- Le Tutoriel de Android UI Layouts
- Le Tutoriel de Android SMS
- Le Tutoriel de Android et SQLite Database
- Le Tutoriel de Google Maps Android API
- Le Tutoriel de texte pour parler dans Android
- Le Tutoriel de Android Space
- Le Tutoriel de Android Toast
- Créer un Android Toast personnalisé
- Le Tutoriel de Android SnackBar
- Le Tutoriel de Android TextView
- Le Tutoriel de Android TextClock
- Le Tutoriel de Android EditText
- Le Tutoriel de Android TextWatcher
- Formater le numéro de carte de crédit avec Android TextWatcher
- Le Tutoriel de Android Clipboard
- Créer un File Chooser simple dans Android
- Le Tutoriel de Android AutoCompleteTextView et MultiAutoCompleteTextView
- Le Tutoriel de Android ImageView
- Le Tutoriel de Android ImageSwitcher
- Le Tutoriel de Android ScrollView et HorizontalScrollView
- Le Tutoriel de Android WebView
- Le Tutoriel de Android SeekBar
- Le Tutoriel de Android Dialog
- Le Tutoriel de Android AlertDialog
- Tutoriel Android RatingBar
- Le Tutoriel de Android ProgressBar
- Le Tutoriel de Android Spinner
- Le Tutoriel de Android Button
- Le Tutoriel de Android Switch
- Le Tutoriel de Android ImageButton
- Le Tutoriel de Android FloatingActionButton
- Le Tutoriel de Android CheckBox
- Le Tutoriel de Android RadioGroup et RadioButton
- Le Tutoriel de Android Chip et ChipGroup
- Utilisation des Image assets et des Icon assets d'Android Studio
- Configuration de la Carte SD pour Android Emulator
- Exemple ChipGroup et Chip Entry
- Comment ajouter des bibliothèques externes à Android Project dans Android Studio?
- Comment désactiver les autorisations déjà accordées à l'application Android?
- Comment supprimer des applications de Android Emulator?
- Le Tutoriel de Android LinearLayout
- Le Tutoriel de Android TableLayout
- Le Tutoriel de Android FrameLayout
- Le Tutoriel de Android QuickContactBadge
- Le Tutoriel de Android StackView
- Le Tutoriel de Android Camera
- Le Tutoriel de Android MediaPlayer
- Le Tutoriel de Android VideoView
- Jouer des effets sonores dans Android avec SoundPool
- Le Tutoriel de Android Networking
- Analyser JSON dans Android
- Le Tutoriel de Android SharedPreferences
- Le Tutorial de stockage interne Android (Internal Storage)
- Le Tutoriel de Android External Storage
- Le Tutoriel de Android Intents
- Exemple d'une Android Intent explicite, appelant une autre Intent
- Exemple de Android Intent implicite, ouvrez une URL, envoyez un email
- Le Tutoriel de Android Service
- Le Tutoriel Android Notifications
- Le Tutoriel de Android DatePicker
- Le Tutoriel de Android TimePicker
- Le Tutoriel de Android Chronometer
- Le Tutoriel de Android OptionMenu
- Le Tutoriel de Android ContextMenu
- Le Tutoriel de Android PopupMenu
- Le Tutoriel de Android Fragment
- Le Tutoriel de Android ListView
- Android ListView avec Checkbox en utilisant ArrayAdapter
- Le Tutoriel de Android GridView
Show More