Boucles dans JavaScript
1. Vue d'ensemble des boucles en ECMAScript
Dans ECMAScript des instructions (statement) sont exécutées de haut en bas. Mais parfois lorsque vous voulez exécuter une séquence de instructions, vous pouvez utiliser la boucle (loop).
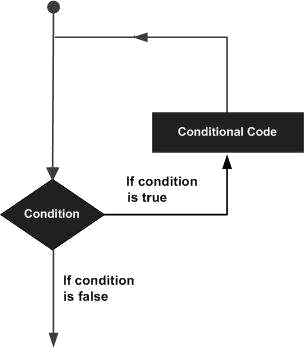
ECMAScript vous fournit 3 types de boucle :
- while loop
- for loop
- do .. while loop
Des instructions (statement) peuvent être utilisées à l'intérieur de boucle :
- continue
- break
Instruction de Contrôle | Description |
break | Termine l'instruction de la boucle |
continue | Cause la boucle pour passer le reste du corps de son bloc (block), et réessayer immédiatement ses conditions avant la répétition. |
2. La boucle while
La syntaxe de la boucle while :
while (condition) {
// Do something here
// ....
}
Exemple :
while-loop-example.js
console.log("While loop example");
// Declare a variable, and assign value of 2.
let x = 2;
// Condition is x < 10
// If x < 10 is true then run block
while (x < 10) {
console.log("Value of x = ", x);
x = x + 3;
}
// This statment is out of while block.
console.log("Finish");
Output:
While loop example
Value of x = 2
Value of x = 5
Value of x = 8
Finish
3. La boucle for
La boucle for standard a la syntaxe comme ci-dessous :
for(InitialValues; condition; updateValues )
{
// Statements
}
- InitialValues: Initialise des valeurs pour les variables liées dans la boucle.
- condition: définit les conditions d'exécution des blocs de commande.
- updateValues: met à jour de nouvelles valeurs pour les variables.
for-loop-example.js
console.log("For loop example");
for( let i = 0; i < 10; i= i + 3) {
console.log("i= "+ i);
}
Output:
For loop example
i= 0
i= 3
i= 6
i= 9
for-loop-example2.js
console.log("For loop example");
for(let i = 0, j = 0; i + j < 10; i = i+1, j = j+2) {
console.log("i = " + i +", j = " + j);
}
Output:
For loop example
i = 0, j = 0
i = 1, j = 2
i = 2, j = 4
i = 3, j = 6
L'utilisation de la boucle for peut vous permettre de traverser les éléments du tableau.
for-loop-example3.js
console.log("For loop example");
// Array
let names =["Tom","Jerry", "Donald"];
for (let i = 0; i < names.length; i++ ) {
console.log("Name = ", names[i]);
}
console.log("End of example");
Output:
For loop example
Name = Tom
Name = Jerry
Name = Donald
End of example
4. La boucle for .. in
la boucle for..in vous permet de parcourir les property d'un objet.
for (prop in object) {
// Do something
}
Exemple :
for-in-loop-example.js
// An object has 4 properties (name, age, gender,greeing)
var myObject = {
name : "John",
age: 25,
gender: "Male",
greeting : function() {
return "Hello";
}
};
for(myProp in myObject) {
console.log(myProp);
}
Output:
name
age
gender
greeting
5. La boucle for .. of
La boucle for..of vous permet de parcourir une Collection, par exemple, Map, Set.
Exemple :
for-of-example.js
// Create a Set from an Array
var fruits = new Set( ["Apple","Banana","Papaya"] );
for(let fruit of fruits) {
console.log(fruit);
}
Output:
Apple
Banana
Papaya
for-of-example2.js
// Create a Map object.
var myContacts = new Map();
myContacts.set("0100-1111", "Tom");
myContacts.set("0100-5555", "Jerry");
myContacts.set("0100-2222", "Donald");
for( let arr of myContacts) {
console.log(arr);
console.log(" - Phone: " + arr[0]);
console.log(" - Name: " + arr[1]);
}
Output:
[ '0100-1111', 'Tom' ]
- Phone: 0100-1111
- Name: Tom
[ '0100-5555', 'Jerry' ]
- Phone: 0100-5555
- Name: Jerry
[ '0100-2222', 'Donald' ]
- Phone: 0100-2222
- Name: Donald
6. La boucle do .. while
The do-while loop is used to execute a section of program many times.The characteristics of the do-while is that the block of statement is always executed at least once. After each iteration, the program will check the condition, if the condition is still correct, the statement block will be executed again.
do {
// Do something
}
while (expression);
Exemple :
do-while-loop-example.js
let value = 3;
do {
console.log("Value = " + value);
value = value + 3;
} while (value < 10);
Output:
Value = 3
Value = 6
Value = 9
7. Utiliser l'instruction break dans la boucle
break est une instruction qui peut se trouver dans une boucle. Cette instruction termine la boucle inconditionnellement.
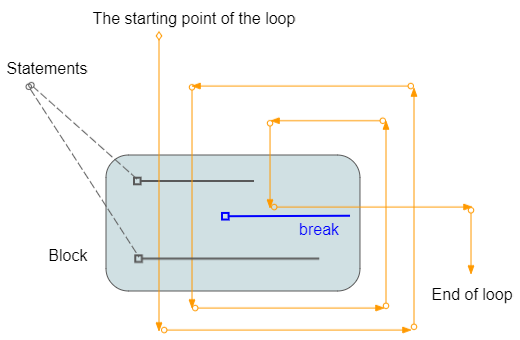
loop-break-example.js
console.log("Break example");
// Declare a variable and assign value of 2.
let x = 2;
while (x < 15) {
console.log("----------------------");
console.log("x = ", x);
// If x = 5 then exit the loop.
if (x == 5) {
break;
}
// Increase value of x by 1
x = x + 1;
console.log("x after + 1 = ", x);
}
console.log("End of example");
Output:
Break example
----------------------
x = 2
x after + 1 = 3
----------------------
x = 3
x after + 1 = 4
----------------------
x = 4
x after + 1 = 5
----------------------
x = 5
End of example
8. Utiliser l'instruction continue dans la boucle
continue est une instruction qui peut se trouver dans une boucle. Lorsque le programme attrape l'instruction continue, il va ignorer les lignes de commandes dans le bloc, dessous de continue et commence une nouvelle boucle.
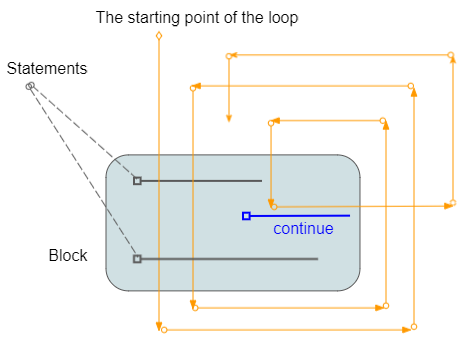
loop-continue-example.js
console.log("Continue example");
// Declare a variable and assign value of 2
x = 2
while (x < 7) {
console.log("----------------------")
console.log("x = ", x)
// % is used for calculating remainder
// If x is even, then ignore the command line below of continue
// and start new iteration.
if (x % 2 == 0) {
// Increase x by 1.
x = x + 1;
continue;
}
else {
// Increase x by 1.
x = x + 1
console.log("x after + 1 =", x)
}
}
console.log("End of example");
Output:
Continue example
----------------------
x = 2
----------------------
x = 3
x after + 1 = 4
----------------------
x = 4
----------------------
x = 5
x after + 1 = 6
----------------------
x = 6
End of example
9. La boucle Labelled (Labelled Loop)
The ECMAScript allows you to stick a label to a loop. It is like you name a loop, which is useful when you use multiple nested loops in a program.
- You can use break labelX statement;to break a loop is attached labelX.
- You can use continue labelX statement; to continue a loop is attached labelX.
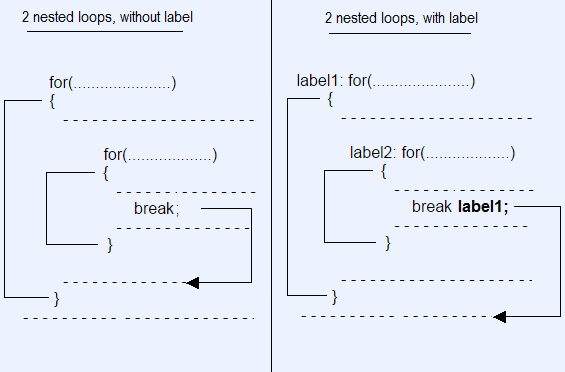
Syntaxe :
// for loop with Label.
label1: for( ... ) {
}
// while loop with Label.
label2: while ( ... ) {
}
// do-while loop with Label.
label3: do {
} while ( ... );
Example of using nested loops, labelled and labelled break statement.
labelled-loop-break-example.js
console.log("Labelled Loop Break example");
let i = 0;
label1: while (i < 5) {
console.log("----------------------\n");
console.log("i = " + i);
i++;
label2: for (let j = 0; j < 3; j++) {
console.log(" --> " + j);
if (j > 0) {
// Exit the loop with label1.
break label1;
}
}
}
console.log("Done!");
Output:
Labelled Loop Break example
----------------------
i = 0
--> 0
--> 1
Done!
Example of using nested labelled loops and labelled continue statement.
labelled-loop-continue-example.js
let i = 0;
label1: while (i < 5) {
console.log("outer i= " + i);
i++;
label2: for (let j = 0; j < 3; j++) {
if (j > 0) {
continue label2;
}
if (i > 1) {
continue label1;
}
console.log("inner i= " + i + ", j= " + j);
}
}
Output:
outer i= 0
inner i= 1, j= 0
outer i= 1
outer i= 2
outer i= 3
outer i= 4
Tutoriels de programmation ECMAScript, Javascript
- Introduction à Javascript et ECMAScript
- Démarrage rapide avec Javascript
- Boîte de dialogue Alert, Confirm, Prompt en Javascript
- Démarrage rapide avec JavaScript
- Variables dans JavaScript
- Opérations sur les bits
- Les Tableaux (Array) en JavaScript
- Boucles dans JavaScript
- Le Tutoriel de JavaScript Function
- Le Tutoriel de JavaScript Number
- Le Tutoriel de JavaScript Boolean
- Le Tutoriel de JavaScript String
- Le Tutoriel de instruction JavaScript if/else
- Le Tutoriel de instruction JavaScript switch
- Tutoriel de gestion des erreurs JavaScript
- Le Tutoriel de JavaScript Date
- Le Tutoriel de JavaScript Module
- L'histoire des modules en JavaScript
- Fonctions setTimeout et setInterval dans JavaScript
- Le Tutoriel de Javascript Form Validation
- Le Tutoriel de JavaScript Web Cookie
- Mot clé Void dans JavaScript
- Classes et objets dans JavaScript
- Techniques de simulation classe et héritage en JavaScript
- Héritage et polymorphisme dans JavaScript
- Comprendre Duck Typing dans JavaScript
- Le Tutoriel de JavaScript Symbol
- Le Tutoriel de JavaScript Set Collection
- Le Tutoriel de JavaScript Map Collection
- Comprendre JavaScript Iterable et Iterator
- Expression régulière en JavaScript
- Le Tutoriel de JavaScript Promise, Async Await
- Le Tutoriel de Javascript Window
- Le Tutoriel de Javascript Console
- Le Tutoriel de Javascript Screen
- Le Tutoriel de Javascript Navigator
- Le Tutoriel de Javascript Geolocation API
- Le Tutoriel de Javascript Location
- Le Tutoriel de Javascript History API
- Le Tutoriel de Javascript Statusbar
- Le Tutoriel de Javascript Locationbar
- Le Tutoriel de Javascript Scrollbars
- Le Tutoriel de Javascript Menubar
- Le Tutoriel de Javascript JSON
- La gestion des événements en JavaScript
- Le Tutoriel de Javascript MouseEvent
- Le Tutoriel de Javascript WheelEvent
- Le Tutoriel de Javascript KeyboardEvent
- Le Tutoriel de Javascript FocusEvent
- Le Tutoriel de Javascript InputEvent
- Le Tutoriel de Javascript ChangeEvent
- Le Tutoriel de Javascript DragEvent
- Le Tutoriel de Javascript HashChangeEvent
- Le Tutoriel de Javascript URL Encoding
- Le Tutoriel de Javascript FileReader
- Le Tutoriel de Javascript XMLHttpRequest
- Le Tutoriel de Javascript Fetch API
- Analyser XML en Javascript avec DOMParser
- Introduction à Javascript HTML5 Canvas API
- Mettre en évidence le code avec la bibliothèque Javascript de SyntaxHighlighter
- Que sont les polyfills en science de la programmation?
Show More