Le Tutoriel de Flutter LinearProgressIndicator
1. LinearProgressIndicator
LinearProgressIndicator est une sous-classe de ProgressIndicator. Il crée un indicateur de barre de progression horizontale, mais il sera également possible de créer un indicateur de barre de progression verticale s'il est placé à l'intérieur d'un RotatedBox tournant de 90 degrés.
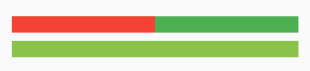
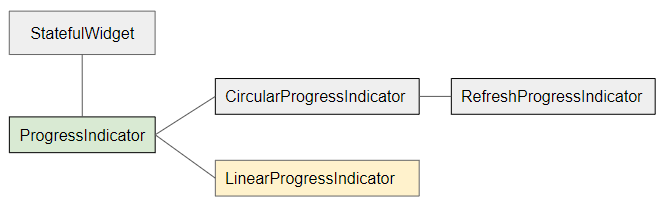
- CircularProgressIndicator
- ProgressIndicator
LinearProgressIndicator est divisé en deux types:
Determinate
Determinate (Déterminé): un indicateur de progression qui montre à l'utilisateur le pourcentage de travail achevé en fonction de la valeur de la propriété value (dans la fourchette de 0 et 1).
Indeterminate
Indeterminate (Indéterminé) : un indicateur de progrès qui n'identifie ni le pourcentage de travail achevé ni l'heure de fin.
LinearProgressIndicator Constructor:
LinearProgressIndicator constructor
const LinearProgressIndicator(
{Key key,
double value,
Color backgroundColor,
Animation<Color> valueColor,
double minHeight,
String semanticsLabel,
String semanticsValue}
)
2. Example: Indeterminate
À commencer par un exemple simple, un LinearProgressIndicator simule un processus actif mais ne connaît pas le pourcentage de travail qui a été terminé et ne sait pas quand terminer.
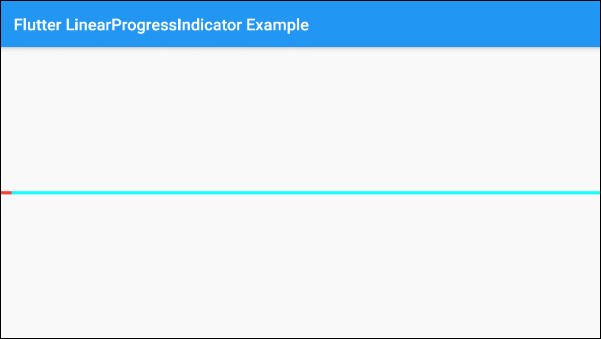
main.dart (ex 1)
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning.org',
debugShowCheckedModeBanner: false,
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter LinearProgressIndicator Example'),
),
body: Center(
child: LinearProgressIndicator(
backgroundColor: Colors.cyan[100],
valueColor: new AlwaysStoppedAnimation<Color>(Colors.green),
),
),
);
}
}
Par défaut, LinearProgressIndicator a une taille assez petite, mais si vous voulez personnaliser sa taille, il faut le mettre dans un SizedBox.
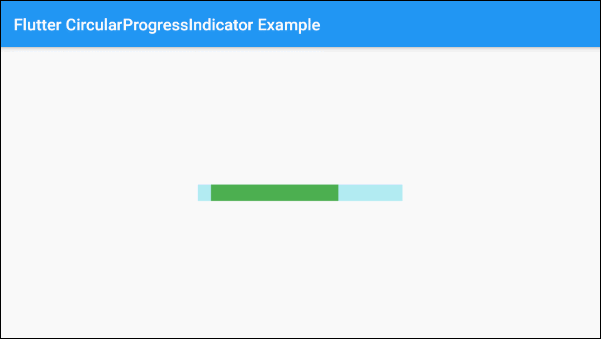
SizedBox(
width: 250,
height: 20,
child: LinearProgressIndicator(
backgroundColor: Colors.cyan[100],
valueColor: new AlwaysStoppedAnimation<Color>(Colors.green),
),
)
Le paramètre valueColor est utilisé pour spécifier une animation de couleur à la progression de LinearProgressIndicator, par exemple:
valueColor: new AlwaysStoppedAnimation<Color>(Colors.red)
AlwaysStoppedAnimation<Color> arrêtera toujours l'animation de LinearProgressIndicator si le paramètre value est une valeur spécifique et non une valeur null.
SizedBox(
width: 250,
height: 20,
child: LinearProgressIndicator(
value: 0.3,
backgroundColor: Colors.cyan[100],
valueColor: new AlwaysStoppedAnimation<Color>(Colors.green),
),
)
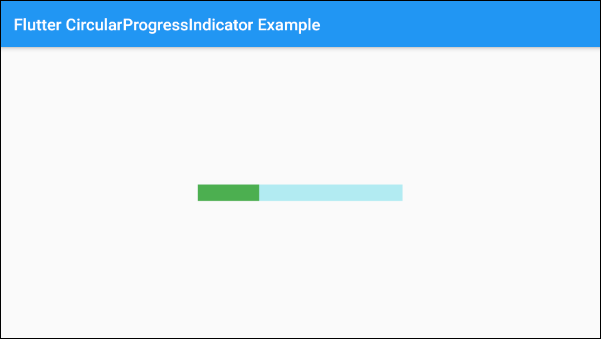
En se basant sur des caractéristiques susmentionnées des paramètres value et valueColor, vous pouvez contrôler le comportement de LinearProgressIndicator. Ci-dessous un exemple:
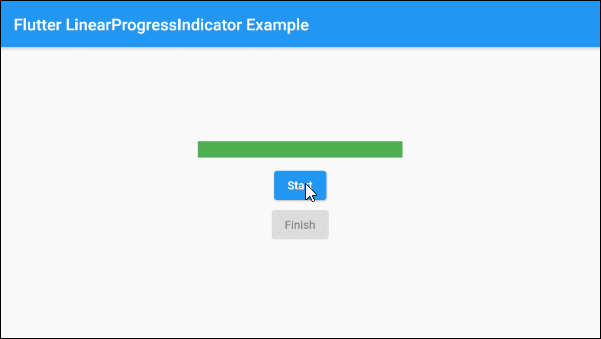
main.dart (ex 1d)
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning.org',
debugShowCheckedModeBanner: false,
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
@override
State<StatefulWidget> createState() {
return _HomePageState();
}
}
class _HomePageState extends State<HomePage> {
bool _working = false;
void startWorking() async {
this.setState(() {
this._working = true;
});
}
void finishWorking() {
this.setState(() {
this._working = false;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter LinearProgressIndicator Example'),
),
body: Center(
child: Column (
mainAxisAlignment: MainAxisAlignment.center,
children: [
SizedBox(
width: 250,
height: 20,
child: LinearProgressIndicator(
value: this._working? null: 1,
backgroundColor: Colors.cyan[100],
valueColor: new AlwaysStoppedAnimation<Color>(Colors.green),
),
),
SizedBox(width:10, height:10),
ElevatedButton(
child: Text("Start"),
onPressed: this._working? null: () {
this.startWorking();
}
),
ElevatedButton(
child: Text("Finish"),
onPressed: !this._working? null: () {
this.finishWorking();
}
)
]
)
),
);
}
}
3. Example: Determinate
Dans l'exemple suivant, on utilise LinearProgressIndicator pour présenter une progression avec le pourcentage de travail terminé. Le paramètre value a une valeur de 0 à 1
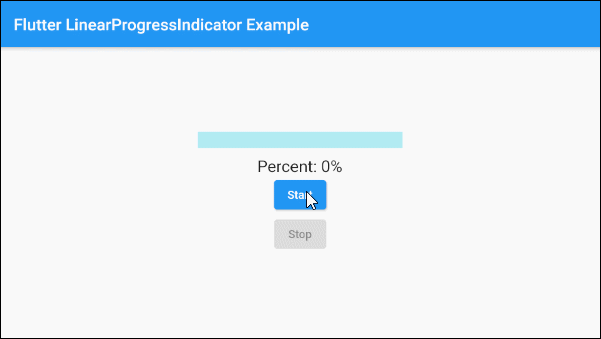
main.dart (ex 2)
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning.org',
debugShowCheckedModeBanner: false,
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
@override
State<StatefulWidget> createState() {
return _HomePageState();
}
}
class _HomePageState extends State<HomePage> {
double _value = 0;
bool _working = false;
void startWorking() async {
this.setState(() {
this._working = true;
this._value = 0;
});
for(int i = 0; i< 10; i++) {
if(!this._working) {
break;
}
await Future.delayed(Duration(seconds: 1));
this.setState(() {
this._value += 0.1;
});
}
this.setState(() {
this._working = false;
});
}
void stopWorking() {
this.setState(() {
this._working = false;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter LinearProgressIndicator Example'),
),
body: Center(
child: Column (
mainAxisAlignment: MainAxisAlignment.center,
children: [
SizedBox(
width: 250,
height: 20,
child: LinearProgressIndicator(
value: this._value,
backgroundColor: Colors.cyan[100],
valueColor: new AlwaysStoppedAnimation<Color>(Colors.green),
),
),
SizedBox(width:10, height: 10),
Text(
"Percent: " + (this._value * 100).round().toString()+ "%",
style: TextStyle(fontSize: 20),
),
ElevatedButton(
child: Text("Start"),
onPressed: this._working? null: () {
this.startWorking();
}
),
ElevatedButton(
child: Text("Stop"),
onPressed: !this._working? null: () {
this.stopWorking();
}
)
]
)
),
);
}
}
4. Example: Vertical Progress
LinearProgressIndicator ne soutient pas la direction verticale. Cependant, si vous souhaitez avoir un indicateur de barre de progression verticale, vous pouvez le placer dans une RotatedBox avec une rotation de 90 degrés.
Par exemple:
RotatedBox(
quarterTurns: -1,
child: SizedBox(
width: 250,
height: 25,
child : LinearProgressIndicator(
backgroundColor: Colors.cyan[100],
valueColor: new AlwaysStoppedAnimation<Color>(Colors.green),
)
)
)
- Le paramètre quarterTurns = 1 signifie une rotation de 90 degrés dans le sens des aiguilles d'une montre.
- Sinon, quarterTurns = -1 signifie une rotation de 90 degrés dans le sens antihoraire.
5. backgroundColor
backgroundColor est utilisé pour définir la couleur d'arrière-plan de LinearProgressIndicator.
Color backgroundColor
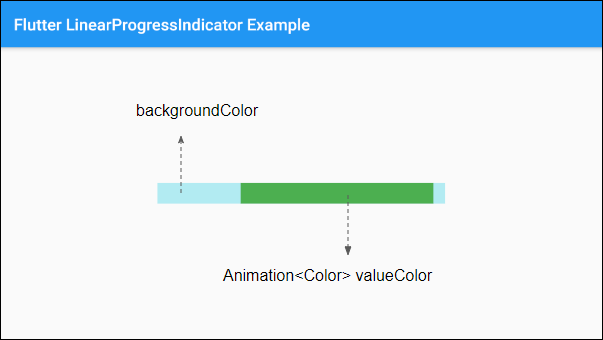
7. valueColor
valueColor est utilisé pour spécifier une animation de couleur à la progression.
Animation<Color> valueColor
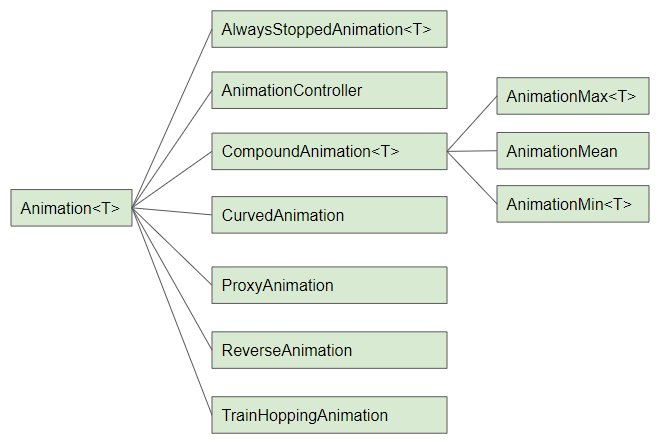
Par exemple:
LinearProgressIndicator(
backgroundColor: Colors.cyanAccent,
valueColor: new AlwaysStoppedAnimation<Color>(Colors.red),
)
- Le Tutoriel de Flutter Animation
8. minHeight
minHeight est utilisé pour définir la hauteur minimale de LinearProgressIndicator.
double minHeight
9. semanticsLabel
semanticsLabel est utilisé pour décrire l'utilisation prévue de LinearProgressIndicator, qui est entièrement caché dans l'interface et déterminé pour les lecteurs d'écran, tels que les lecteurs d'écran pour les malvoyants.
String semanticsLabel
10. semanticsValue
semanticsValue est complètement masquée à l'écran. Son objectif est de fournir des informations sur la progression actuelle des lecteurs d'écran.
Par défaut, la valeur de la semanticsValue est le pourcentage du travail terminé, par exemple, la valeur de la propriété value est 0,3, ce qui signifie que la valeur sémantique est "30%".
String semanticsValue
Example:
LinearProgressIndicator(
value: this._value,
backgroundColor: Colors.cyanAccent,
valueColor: new AlwaysStoppedAnimation<Color>(Colors.red),
semanticsLabel: "Downloading file abc.mp3",
semanticsValue: "Percent " + (this._value * 100).toString() + "%",
)
Tutoriels de programmation Flutter
- Installer Flutter SDK sur Windows
- Installer Flutter Plugin pour Android Studio
- Créez votre première application Flutter - Hello Flutter
- Le Tutoriel de Flutter Scaffold
- Le Tutoriel de Flutter AppBar
- Le Tutoriel de Flutter BottomAppBar
- Le Tutoriel de Flutter TabBar
- Le Tutoriel de Flutter Banner
- Le Tutoriel de Flutter SplashScreen
- Le Tutoriel de Flutter BottomNavigationBar
- Le Tutoriel de Flutter FancyBottomNavigation
- Le Tutoriel de CircularProgressIndicator
- Le Tutoriel de Flutter LinearProgressIndicator
- Le Tutoriel de Flutter Container
- Le Tutoriel de Flutter Center
- Le Tutoriel de Flutter Align
- Le Tutoriel de Flutter Row
- Le Tutoriel de Flutter Column
- Le Tutoriel de Flutter Stack
- Le Tutoriel de Flutter IndexedStack
- Le Tutoriel de Flutter Spacer
- Le Tutoriel de Flutter Expanded
- Le Tutoriel de Flutter SizedBox
- Le Tutoriel de Flutter RotatedBox
- Le Tutoriel de Flutter Card
- Le Tutoriel de Flutter CircleAvatar
- Le Tutoriel de Flutter IconButton
- Le Tutoriel de Flutter FlatButton
- Le Tutoriel de Flutter TextButton
- Le Tutoriel de Flutter ElevatedButton
- Le Tutoriel de Flutter SnackBar
- Le Tutoriel de Flutter Tween
- Le Tutoriel de Flutter SimpleDialog
- Le Tutoriel de Flutter AlertDialog
- Navigation et Routing dans Flutter
- Le Tutoriel de Flutter Border
- Le Tutoriel de Flutter ContinuousRectangleBorder
- Le Tutoriel de Flutter RoundedRectangleBorder
- Le Tutoriel de Flutter CircleBorder
- Le Tutoriel de Flutter StadiumBorder
- Le Tutoriel de Flutter EdgeInsetsGeometry
- Le Tutoriel de Flutter EdgeInsets
- Le Tutoriel de Flutter Alignment
- Le Tutoriel de Flutter Positioned
Show More