Le Tutoriel de JavaFX Alert Dialog
1. JavaFX Alert
La classe Alert (l'alerte) est une sous-classe de la classe Dialog (le dialogue) et fournit un support pour un certain nombre de types de dialog prédéfinis qui peuvent être facilement montrés aux utilisateurs pour demander une réponse. Par conséquent, pour de nombreux utilisateurs, la classe Alert est la classe la plus adaptée à leurs besoins (par opposition à l'utilisation directe de Dialog). Sinon, les utilisateurs qui souhaitent inviter un utilisateur à saisir du texte ou à sélectionner parmi une liste d'options seraient mieux servis en utilisant respectivement TextInputDialog et ChoiceDialog.
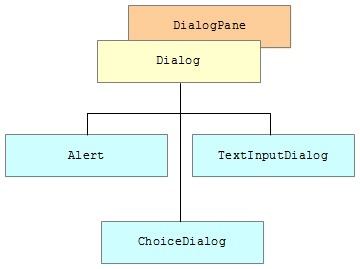
Alert par défaut est une fenêtre avec la modélisation (modelity) est Modelity.WINDOW_MODAL. Cependant, vous pouvez changer en utilisant alert.initModality(Modality).
Par défaut, n'importe quelle fenêtre qui ouvre une dialogue Alert sera la fenêtre paren (la fenêtre propriétaire) de cette ladite Alert.
Vous pouvez voir plus des explications sur Modelity dans des postes ci-dessous:
- Hướng dẫn sử dụng JavaFX Dialog
Ceci est l'illustration de la structure de fenêtre Alert:
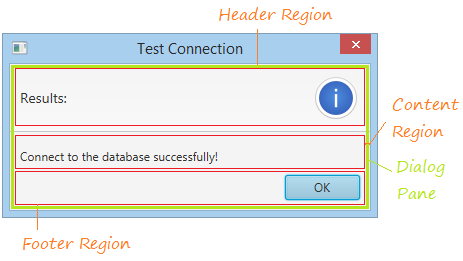
Header Region (La région Header):
Cette région est utilisée pour afficher une notification courte et une icône (icon).
Content Region (La région de contenu):
Par défaut Content Region affiche un Label que vous pouvez définir le contenu du texte de ce Label via la méthode alert.setContentText(String). Vous pouvez également afficher autres Node dans Content Region via alert.getDialogPane().setContent(Node).
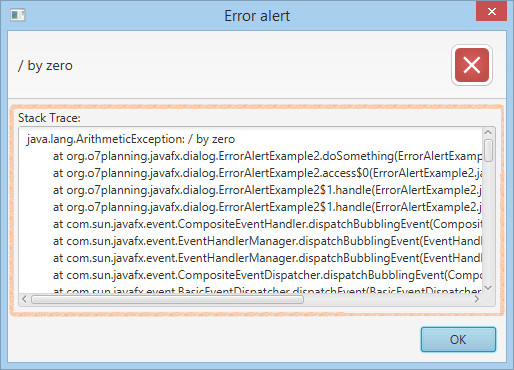
Footer Region (La région Footer):
Cette région est utilisée pour afficher les boutons (Button). Vous pouvez personnaliser les boutons affichés.
2. Information Alert
Information Alert (La boite d'information) est une fenêtre dialog affichant des informations. Ceci est une image d'une Information Alert standard :
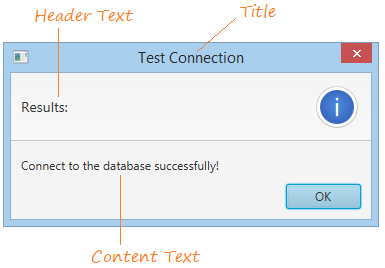
L'image d'une Information Alert avec Header Text par défaut :
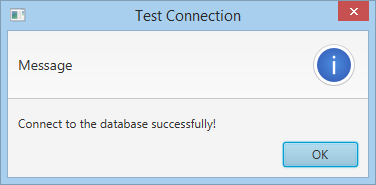
L'image d'une Information Alert sans Header Text:
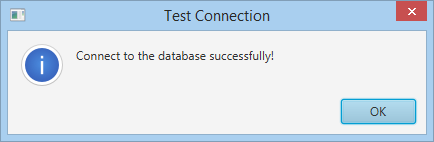
Exemple:
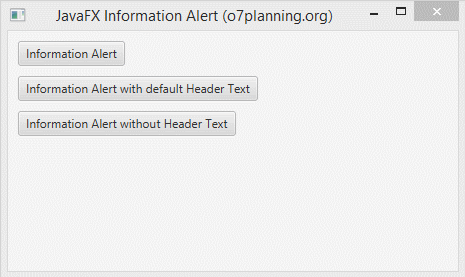
InformationAlertExample.java
package org.o7planning.javafx.alert;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class InformationAlertExample extends Application {
// Show a Information Alert with header Text
private void showAlertWithHeaderText() {
Alert alert = new Alert(AlertType.INFORMATION);
alert.setTitle("Test Connection");
alert.setHeaderText("Results:");
alert.setContentText("Connect to the database successfully!");
alert.showAndWait();
}
// Show a Information Alert with default header Text
private void showAlertWithDefaultHeaderText() {
Alert alert = new Alert(AlertType.INFORMATION);
alert.setTitle("Test Connection");
// alert.setHeaderText("Results:");
alert.setContentText("Connect to the database successfully!");
alert.showAndWait();
}
// Show a Information Alert without Header Text
private void showAlertWithoutHeaderText() {
Alert alert = new Alert(AlertType.INFORMATION);
alert.setTitle("Test Connection");
// Header Text: null
alert.setHeaderText(null);
alert.setContentText("Connect to the database successfully!");
alert.showAndWait();
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
Button button1 = new Button("Information Alert");
Button button2 = new Button("Information Alert with default Header Text");
Button button3 = new Button("Information Alert without Header Text");
button1.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithHeaderText();
}
});
button2.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithDefaultHeaderText();
}
});
button3.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithoutHeaderText();
}
});
root.getChildren().addAll(button1, button2, button3);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Information Alert (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
3. Warning Alert
Warning Alert est similaire à une Information Alert, à l'exception de son icône et de son utilisation prévue. Warning Alert est utilisée pour avertir un utilisateur des risques ou problèmes potentiels, bien que cela ne se produise probablement pas.
L'image d'une Warning Alert standard :
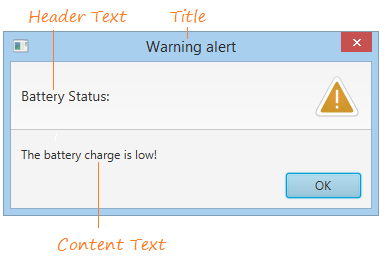
L'image d'une Warning Alert avec Header Text par défaut :
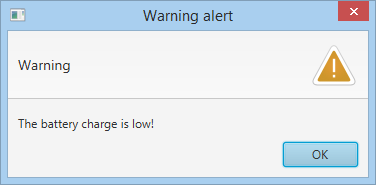
L'image d'une Warning Alert sans Header Text:
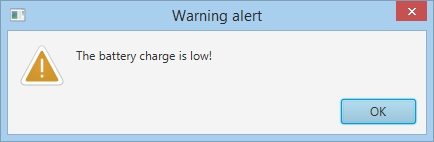
Exemple :
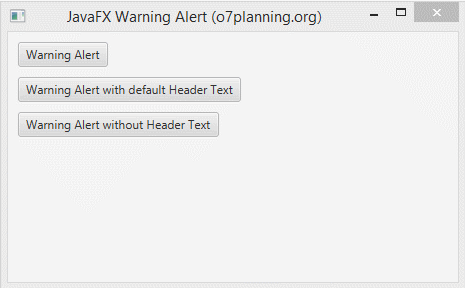
WarningAlertExample.java
package org.o7planning.javafx.alert;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class WarningAlertExample extends Application {
// Show a Warning Alert with header Text
private void showAlertWithHeaderText() {
Alert alert = new Alert(AlertType.WARNING);
alert.setTitle("Warning alert");
alert.setHeaderText("Battery Status:");
alert.setContentText("The battery charge is low!");
alert.showAndWait();
}
// Show a Warning Alert with default header Text
private void showAlertWithDefaultHeaderText() {
Alert alert = new Alert(AlertType.WARNING);
alert.setTitle("Warning alert");
// alert.setHeaderText("Battery Status:");
alert.setContentText("The battery charge is low!");
alert.showAndWait();
}
// Show a Warning Alert without Header Text
private void showAlertWithoutHeaderText() {
Alert alert = new Alert(AlertType.WARNING);
alert.setTitle("Warning alert");
// Header Text: null
alert.setHeaderText(null);
alert.setContentText("The battery charge is low!");
alert.showAndWait();
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
Button button1 = new Button("Warning Alert");
Button button2 = new Button("Warning Alert with default Header Text");
Button button3 = new Button("Warning Alert without Header Text");
button1.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithHeaderText();
}
});
button2.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithDefaultHeaderText();
}
});
button3.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithoutHeaderText();
}
});
root.getChildren().addAll(button1, button2, button3);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Warning Alert (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
4. Error Alert
Error Alert est la même que Informtion Alert et Warning Alert, sauf pour l'icône (icon) et l'utilisation prévue. Error Alert est utilisée pour notifier l'occurrence d'une affaire sérieuse.
Pour créer une Error Alert vous devez utiliser AlertType.ERROR.
* Error Alert *
Alert alert = new Alert(AlertType.ERROR);
alert.setTitle("Error alert");
alert.setHeaderText("Can not add user");
alert.setContentText("The 'abc' user does not exists!");
alert.showAndWait();
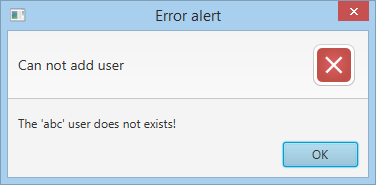
En effet, lors qu'une erreur se produit, vous affichez une Error Alert pour que des utilisateurs sachent. Il comprend des informations courtes et probablement des détails d'erreur, dans ce cas vous devez définir un contenu de personnalisation pour Content Region.
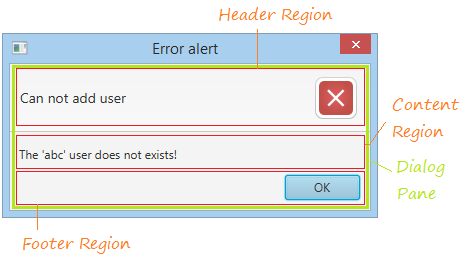
Exemple:
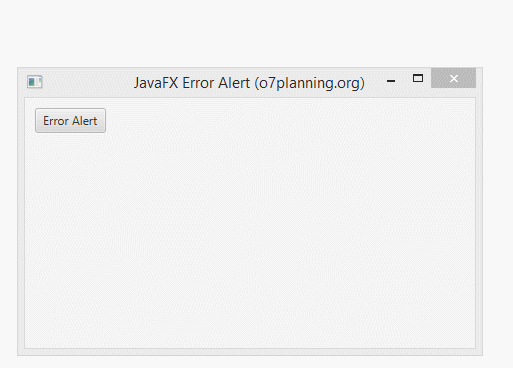
ErrorAlertExample2.java
package org.o7planning.javafx.alert;
import java.io.PrintWriter;
import java.io.StringWriter;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextArea;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ErrorAlertExample2 extends Application {
private void showError(Exception e) {
Alert alert = new Alert(AlertType.ERROR);
alert.setTitle("Error alert");
alert.setHeaderText(e.getMessage());
VBox dialogPaneContent = new VBox();
Label label = new Label("Stack Trace:");
String stackTrace = this.getStackTrace(e);
TextArea textArea = new TextArea();
textArea.setText(stackTrace);
dialogPaneContent.getChildren().addAll(label, textArea);
// Set content for Dialog Pane
alert.getDialogPane().setContent(dialogPaneContent);
alert.showAndWait();
}
private void doSomething() {
try {
// An error has occurred here.
int a = 100 / 0;
} catch (Exception e) {
showError(e);
}
}
private String getStackTrace(Exception e) {
StringWriter sw = new StringWriter();
PrintWriter pw = new PrintWriter(sw);
e.printStackTrace(pw);
String s = sw.toString();
return s;
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
Button button1 = new Button("Error Alert");
button1.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
doSomething();
}
});
root.getChildren().addAll(button1);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Error Alert (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
5. Confirmation Alert
Confirmation Alert s'affiche pour demander aux utilisateurs de confirmer qu'une action sera effectuée ou non. Il est par défaut que Confirmation Alert aura 2 options pour les utilisateurs telles que OK ou Cancel.
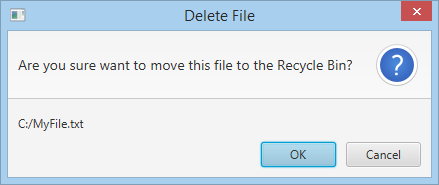
Exemple:
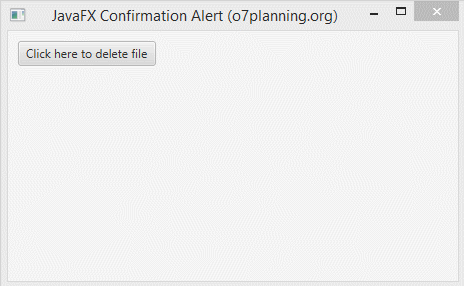
ConfirmationAlertExample.java
package org.o7planning.javafx.alert;
import java.util.Optional;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.control.ButtonType;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ConfirmationAlertExample extends Application {
private Label label;
private void showConfirmation() {
Alert alert = new Alert(AlertType.CONFIRMATION);
alert.setTitle("Delete File");
alert.setHeaderText("Are you sure want to move this file to the Recycle Bin?");
alert.setContentText("C:/MyFile.txt");
// option != null.
Optional<ButtonType> option = alert.showAndWait();
if (option.get() == null) {
this.label.setText("No selection!");
} else if (option.get() == ButtonType.OK) {
this.label.setText("File deleted!");
} else if (option.get() == ButtonType.CANCEL) {
this.label.setText("Cancelled!");
} else {
this.label.setText("-");
}
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
this.label = new Label();
Button button = new Button("Click here to delete file");
button.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showConfirmation();
}
});
root.getChildren().addAll(button, label);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Confirmation Alert (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
6. Personnaliser des Button
La dialogue Alert vous permet de personnaliser des boutons qui seront affiché sur la Footer Region, ceci est un exemple :
Exemple:
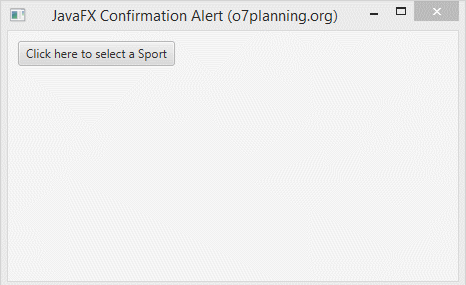
ConfirmationAlertExample2.java
package org.o7planning.javafx.alert;
import java.util.Optional;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.control.ButtonType;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ConfirmationAlertExample2 extends Application {
private Label label;
private void showConfirmation() {
Alert alert = new Alert(AlertType.CONFIRMATION);
alert.setTitle("Select");
alert.setHeaderText("Choose the sport you like:");
ButtonType football = new ButtonType("Football");
ButtonType badminton = new ButtonType("Badminton");
ButtonType volleyball = new ButtonType("Volleyball");
// Remove default ButtonTypes
alert.getButtonTypes().clear();
alert.getButtonTypes().addAll(football, badminton, volleyball);
// option != null.
Optional<ButtonType> option = alert.showAndWait();
if (option.get() == null) {
this.label.setText("No selection!");
} else if (option.get() == football) {
this.label.setText("You like Football");
} else if (option.get() == badminton) {
this.label.setText("You like Badminton");
} else if (option.get() == volleyball) {
this.label.setText("You like Volleyball");
} else {
this.label.setText("-");
}
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
this.label = new Label();
Button button = new Button("Click here to select a Sport");
button.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showConfirmation();
}
});
root.getChildren().addAll(button, label);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Confirmation Alert (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
Tutoriels de JavaFX
- Ouvrir une nouvelle fenêtre (Window) dans JavaFX
- Le Tutoriel de JavaFX ChoiceDialog
- Le Tutoriel de JavaFX Alert Dialog
- Le Tutoriel de JavaFX TextInputDialog
- Installer e(fx)clipse pour Eclipse (Outillage JavaFX)
- Installer JavaFX Scene Builder pour Eclipse
- Tutoriel JavaFX pour débutant - Hello JavaFX
- Le Tutoriel de JavaFX FlowPane Layout
- Le Tutoriel de JavaFX TilePane Layout
- Le Tutoriel de JavaFX HBox et VBox Layout
- Le Tutoriel de JavaFX BorderPane Layout
- Le Tutoriel de JavaFX AnchorPane Layout
- Le Tutoriel de JavaFX TitledPane
- Le Tutoriel de JavaFX Accordion
- Le Tutoriel de JavaFX ListView
- Le Tutoriel de JavaFX Group
- Le Tutoriel de JavaFX ComboBox
- Transformations dans JavaFX
- Les effets (effects) dans JavaFX
- Le Tutoriel de JavaFX GridPane Layout
- Le Tutoriel de JavaFX StackPane Layout
- Le Tutoriel de JavaFX ScrollPane
- Le Tutoriel de JavaFX WebView et WebEngine
- Le Tutoriel de JavaFX HTMLEditor
- Le Tutoriel de JavaFX TableView
- Le Tutoriel de JavaFX TreeView
- Le Tutoriel de JavaFX TreeTableView
- Le Tutoriel de JavaFX Menu
- Le Tutoriel de JavaFX ContextMenu
- Le Tutoriel de JavaFX Image et ImageView
- Le Tutoriel de JavaFX Label
- Le Tutoriel de JavaFX Hyperlink
- Le Tutoriel de JavaFX Button
- Le Tutoriel de JavaFX ToggleButton
- Le Tutoriel de JavaFX RadioButton
- Le Tutoriel de JavaFX MenuButton et SplitMenuButton
- Le Tutoriel de JavaFX TextField
- Le Tutoriel de JavaFX PasswordField
- Le Tutoriel de JavaFX TextArea
- Le Tutoriel de JavaFX Slider
- Le Tutoriel de JavaFX Spinner
- Le Tutoriel de JavaFX ProgressBar et ProgressIndicator
- Le Tutoriel de JavaFX ChoiceBox
- Le Tutoriel de JavaFX Tooltip
- Le Tutoriel de JavaFX DatePicker
- Le Tutoriel de JavaFX ColorPicker
- Le Tutoriel de JavaFX FileChooser et DirectoryChooser
- Le Tutoriel de JavaFX PieChart
- Le Tutoriel de JavaFX AreaChart et StackedAreaChart
- Le Tutoriel de JavaFX BarChart et StackedBarChart
- Le Tutoriel de JavaFX Line
- Le Tutoriel de JavaFX Rectangle et Ellipse
Show More