Le Tutoriel de JavaFX StackPane Layout
1. StackPane Layout
StackPane est un conteneur qui peut contenir différents composants d'interface, des sous-composants empilés à d'autres et à un moment donné, vous ne pouvez voir que le sous-composant situé sur le dessus de Stack.
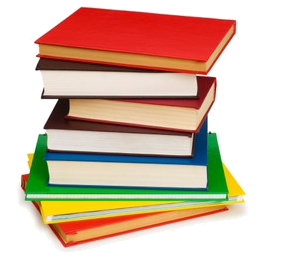
Ces sous-composants nouvellement ajoutés se situent au sommet de Stack.
// Add component to Stack.
stackPane.getChildren().add(newChild);
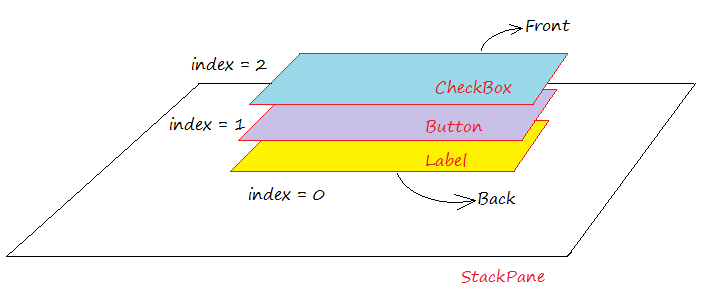
Vous pouvez mettre un certain sous-composant devant (front) Stack via la méthode nommée toFront(), ou mettre le sous-composant au bas de Stack via la méthode nommée toBack().
// All Child components of StackPane
ObservableList<Node> childs = stackPane.getChildren();
if (childs.size() > 1) {
// Top Component
Node topNode = childs.get(childs.size()-1);
topNode.toBack();
}
2. Exemple de StackPane
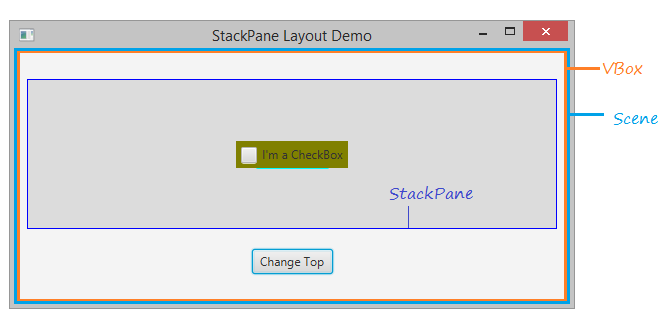
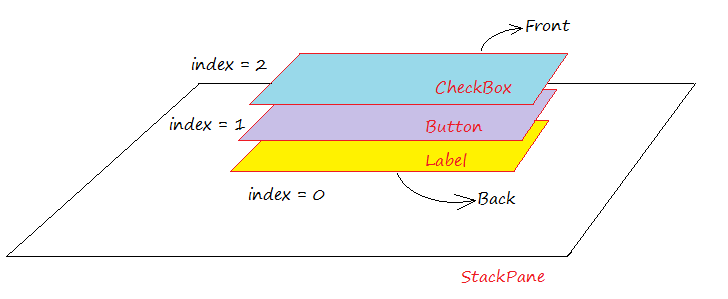
StackPaneDemo.java
package org.o7planning.javafx.stackpane;
import javafx.application.Application;
import javafx.collections.ObservableList;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Node;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.CheckBox;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class StackPaneDemo extends Application {
private StackPane stackPane;
@Override
public void start(Stage primaryStage) throws Exception {
VBox root = new VBox();
// StackPane
stackPane = new StackPane();
// Add Label to StackPane
Label label = new Label("I'm a Label");
label.setStyle("-fx-background-color:yellow");
label.setPadding(new Insets(5,5,5,5));
stackPane.getChildren().add(label);
// Add Button to StackPane
Button button = new Button("I'm a Button");
button.setStyle("-fx-background-color: cyan");
button.setPadding(new Insets(5,5,5,5));
stackPane.getChildren().add(button);
// Add CheckBox to StackPane
CheckBox checkBox = new CheckBox("I'm a CheckBox");
checkBox.setOpacity(1);
checkBox.setStyle("-fx-background-color:olive");
checkBox.setPadding(new Insets(5,5,5,5));
stackPane.getChildren().add(checkBox);
//
stackPane.setPrefSize(300, 150);
stackPane.setStyle("-fx-background-color: Gainsboro;-fx-border-color: blue;");
//
root.getChildren().add(stackPane);
Button controlButton = new Button("Change Top");
root.getChildren().add(controlButton);
root.setAlignment(Pos.CENTER);
VBox.setMargin(stackPane, new Insets(10, 10, 10, 10));
VBox.setMargin(controlButton, new Insets(10, 10, 10, 10));
//
Scene scene = new Scene(root, 550, 250);
primaryStage.setTitle("StackPane Layout Demo");
primaryStage.setScene(scene);
controlButton.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
changeTop();
}
});
primaryStage.show();
}
private void changeTop() {
ObservableList<Node> childs = this.stackPane.getChildren();
if (childs.size() > 1) {
//
Node topNode = childs.get(childs.size()-1);
topNode.toBack();
}
}
public static void main(String[] args) {
launch(args);
}
}
L'exécution de l'exemple:
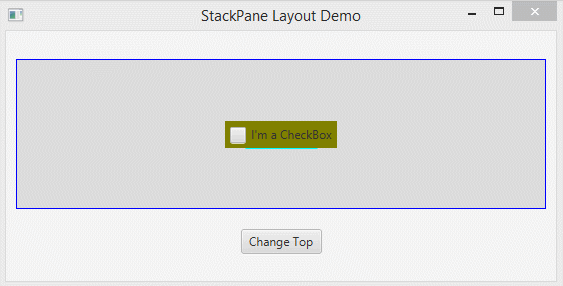
Normalement, vous n'indiquez que le sous-composant sur le dessus de StackPane et cachez d'autres sous-composants. Utilisation de la méthode Node.setVisible(visible). Voyons l'exemple illustratif:
StackPaneDemo2.java
package org.o7planning.javafx.stackpane;
import javafx.application.Application;
import javafx.collections.ObservableList;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Node;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.CheckBox;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class StackPaneDemo2 extends Application {
private StackPane stackPane;
@Override
public void start(Stage primaryStage) throws Exception {
VBox root = new VBox();
// StackPane
stackPane = new StackPane();
// Add Label to StackPane
Label label = new Label("I'm a Label");
label.setStyle("-fx-background-color:yellow");
label.setPadding(new Insets(5,5,5,5));
// Hide this label.
label.setVisible(false);
stackPane.getChildren().add(label);
// Add Button to StackPane
Button button = new Button("I'm a Button");
button.setStyle("-fx-background-color: cyan");
button.setPadding(new Insets(5,5,5,5));
// Hide this button.
button.setVisible(false);
stackPane.getChildren().add(button);
// Add CheckBox to StackPane
CheckBox checkBox = new CheckBox("I'm a CheckBox");
checkBox.setOpacity(1);
checkBox.setStyle("-fx-background-color:olive");
checkBox.setPadding(new Insets(5,5,5,5));
// Show this checkBox (default).
checkBox.setVisible(true);
stackPane.getChildren().add(checkBox);
//
stackPane.setPrefSize(300, 150);
stackPane.setStyle("-fx-background-color: Gainsboro;-fx-border-color: blue;");
//
root.getChildren().add(stackPane);
Button controlButton = new Button("Change Top");
root.getChildren().add(controlButton);
root.setAlignment(Pos.CENTER);
VBox.setMargin(stackPane, new Insets(10, 10, 10, 10));
VBox.setMargin(controlButton, new Insets(10, 10, 10, 10));
//
Scene scene = new Scene(root, 550, 250);
primaryStage.setTitle("StackPane Layout Demo 2");
primaryStage.setScene(scene);
controlButton.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
changeTop();
}
});
primaryStage.show();
}
private void changeTop() {
ObservableList<Node> childs = this.stackPane.getChildren();
if (childs.size() > 1) {
//
Node topNode = childs.get(childs.size()-1);
// This node will be brought to the front
Node newTopNode = childs.get(childs.size()-2);
topNode.setVisible(false);
topNode.toBack();
newTopNode.setVisible(true);
}
}
public static void main(String[] args) {
launch(args);
}
}
L'exécution de l'exemple:
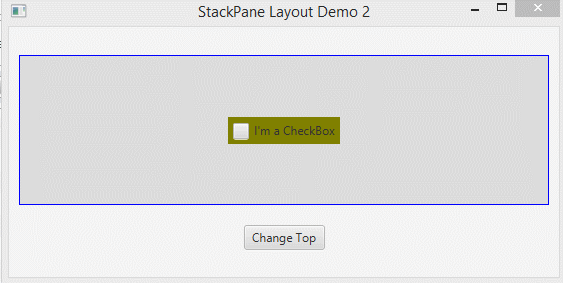
Tutoriels de JavaFX
- Ouvrir une nouvelle fenêtre (Window) dans JavaFX
- Le Tutoriel de JavaFX ChoiceDialog
- Le Tutoriel de JavaFX Alert Dialog
- Le Tutoriel de JavaFX TextInputDialog
- Installer e(fx)clipse pour Eclipse (Outillage JavaFX)
- Installer JavaFX Scene Builder pour Eclipse
- Tutoriel JavaFX pour débutant - Hello JavaFX
- Le Tutoriel de JavaFX FlowPane Layout
- Le Tutoriel de JavaFX TilePane Layout
- Le Tutoriel de JavaFX HBox et VBox Layout
- Le Tutoriel de JavaFX BorderPane Layout
- Le Tutoriel de JavaFX AnchorPane Layout
- Le Tutoriel de JavaFX TitledPane
- Le Tutoriel de JavaFX Accordion
- Le Tutoriel de JavaFX ListView
- Le Tutoriel de JavaFX Group
- Le Tutoriel de JavaFX ComboBox
- Transformations dans JavaFX
- Les effets (effects) dans JavaFX
- Le Tutoriel de JavaFX GridPane Layout
- Le Tutoriel de JavaFX StackPane Layout
- Le Tutoriel de JavaFX ScrollPane
- Le Tutoriel de JavaFX WebView et WebEngine
- Le Tutoriel de JavaFX HTMLEditor
- Le Tutoriel de JavaFX TableView
- Le Tutoriel de JavaFX TreeView
- Le Tutoriel de JavaFX TreeTableView
- Le Tutoriel de JavaFX Menu
- Le Tutoriel de JavaFX ContextMenu
- Le Tutoriel de JavaFX Image et ImageView
- Le Tutoriel de JavaFX Label
- Le Tutoriel de JavaFX Hyperlink
- Le Tutoriel de JavaFX Button
- Le Tutoriel de JavaFX ToggleButton
- Le Tutoriel de JavaFX RadioButton
- Le Tutoriel de JavaFX MenuButton et SplitMenuButton
- Le Tutoriel de JavaFX TextField
- Le Tutoriel de JavaFX PasswordField
- Le Tutoriel de JavaFX TextArea
- Le Tutoriel de JavaFX Slider
- Le Tutoriel de JavaFX Spinner
- Le Tutoriel de JavaFX ProgressBar et ProgressIndicator
- Le Tutoriel de JavaFX ChoiceBox
- Le Tutoriel de JavaFX Tooltip
- Le Tutoriel de JavaFX DatePicker
- Le Tutoriel de JavaFX ColorPicker
- Le Tutoriel de JavaFX FileChooser et DirectoryChooser
- Le Tutoriel de JavaFX PieChart
- Le Tutoriel de JavaFX AreaChart et StackedAreaChart
- Le Tutoriel de JavaFX BarChart et StackedBarChart
- Le Tutoriel de JavaFX Line
- Le Tutoriel de JavaFX Rectangle et Ellipse
Show More