Expression régulière en Java
1. Expression régulière (Regular expression)
Une expression régulière (Regular Expression) définit un motif (pattern) de recherche des chaînes. Elle peut être utilisé pour rechercher, modifier et manipuler le texte. Le motif qui est défini par une expression régulière peut être correspondre à une ou à plusieurs fois, ou ne correspond pas à la chaîne donnée.
L'abréviation de l'expression régulière est regex
L'abréviation de l'expression régulière est regex
Expression régulière est soutenue par la plupart langages de programmation, par exemple Java, C#, C/C++, ...malheureusement chaque langage soutient des expressions régulières légèrement différent .
Vous pourriez vous intéresser à:
2. Règle d'écriture des expressions régulières
Ordre | Expression régulière | Description |
1 | . | Correspond (match) à n'importe quel caractère |
2 | ^regex | Expression régulière doit correspondre au début de la ligne. |
3 | regex$ | Expression régulière doit correspondre à la fin de la ligne. |
4 | [abc] | Définit la définition, peut correspondre à a ou b ou c. |
5 | [abc][vz] | Définit la définition, peut correspondre à a ou b ou c suivi de v ou z |
6 | [^abc] | Lorsque le signe ^ apparaît comme le premier caractère dans les parenthèses, il nie le motif. Cela peut correspondre à tout caractère à part de a ou b ou c. |
7 | [a-d1-7] | Portée : corresponde à une chaîne de caractères entre a et d et les chiffres de 1 à 7. |
8 | X|Z | Cherche X ou Z. |
9 | XZ | Cherche X et suivi d'être Z. |
10 | $ | Vérifie la fin de la ligne. |
11 | \d | Tout chiffre, est l’abréviation de [0-9] |
12 | \D | Le caractère n'est pas un nombre, est l’abréviation de [^0-9] |
13 | \s | Le caractère est un espace blanc, est l’abréviation de [ \t\n\x0b\r\f] |
14 | \S | Le caractère n'est pas un espace blanc, est l’abréviation de [^\s] |
15 | \w | Un caractère de mot, est l’abréviation de [a-zA-Z_0-9] |
16 | \W | Un caractère n'est pas de mot, est l’abréviation de [^\w] |
17 | \S+ | Certains caractères qui ne sont pas des espaces blancs (un ou plusieurs) |
18 | \b | Caractères dans l'a-z ou A-Z ou 0-9 ou _, est l’abréviation de [a-zA-Z0-9_] . |
19 | * | Affiche 0 ou plusieurs fois, est l’abréviation de {0,} |
20 | + | Affiche 1 ou plusieurs fois, est l’abréviation de {1,} |
21 | ? | Affiche o ou 1 fois, est l’abréviation de {0,1} . |
22 | {X} | Affiche X fois, {} |
23 | {X,Y} | Affiche dans environ X à Y fois. |
24 | *? | * signifie apparaitre 0 ou plusieurs fois, ajouter ? cela signifie qu'il essaie de rechercher la correspondance la plus petite. |
3. Les caractères spéciaux dans Java Regex
Certains caractères spéciaux dans Java RegEx :
\.[{(*+?^$|
Les caractères mentionnés ci-dessus sont les caractères spéciaux. En Java Regex, si vous voulez qu'il comprenne le caractère selon la façon habituelle dont vous avez besoin d'ajouter un marquage \ à l'avant.
Ces caractères de points . java regex est interprété comme un caractère quelconque, si vous le voulez interpréter comme un caractère de point classique, ont marqué \ avant.
Ces caractères de points . java regex est interprété comme un caractère quelconque, si vous le voulez interpréter comme un caractère de point classique, ont marqué \ avant.
// Regex pattern describe any character.
String regex = ".";
// Regex pattern describe a dot character.
String regex = "\\.";
4. Utilisation de String.matches(String)
- Class String
...
// Check the entire String object matches the regex or not.
public boolean matches(String regex)
..
L'utilisation de la méthode String.matches (String regex) vous permet de vérifier entière de la String si elle correspond à regex ou non. Ceci est la manière la plus courante. Observez l'exemple ci-dessous :
StringMatches.java
package org.o7planning.tutorial.regex.stringmatches;
public class StringMatches {
public static void main(String[] args) {
String s1 = "a";
System.out.println("s1=" + s1);
// Check the entire s1
// Match any character
// Rule .
// ==> true
boolean match = s1.matches(".");
System.out.println("-Match . " + match);
s1 = "abc";
System.out.println("s1=" + s1);
// Check the entire s1
// Match any character
// Rule .
// ==> false (Because s1 has three characters)
match = s1.matches(".");
System.out.println("-Match . " + match);
// Check the entire s1
// Match with any character 0 or more times
// Combine the rules . and *
// ==> true
match = s1.matches(".*");
System.out.println("-Match .* " + match);
String s2 = "m";
System.out.println("s2=" + s2);
// Check the entire s2
// Start by m
// Rule ^
// ==> true
match = s2.matches("^m");
System.out.println("-Match ^m " + match);
s2 = "mnp";
System.out.println("s2=" + s2);
// Check the entire s2
// Start by m
// Rule ^
// ==> false (Because s2 has three characters)
match = s2.matches("^m");
System.out.println("-Match ^m " + match);
// Start by m
// Next any character, appearing one or more times.
// Rule ^ and. and +
// ==> true
match = s2.matches("^m.+");
System.out.println("-Match ^m.+ " + match);
String s3 = "p";
System.out.println("s3=" + s3);
// Check s3 ending with p
// Rule $
// ==> true
match = s3.matches("p$");
System.out.println("-Match p$ " + match);
s3 = "2nnp";
System.out.println("s3=" + s3);
// Check the entire s3
// End of p
// ==> false (Because s3 has 4 characters)
match = s3.matches("p$");
System.out.println("-Match p$ " + match);
// Check out the entire s3
// Any character appearing once.
// Followed by n, appear one or up to three times.
// End by p: p $
// Combine the rules: . , {X, y}, $
// ==> true
match = s3.matches(".n{1,3}p$");
System.out.println("-Match .n{1,3}p$ " + match);
String s4 = "2ybcd";
System.out.println("s4=" + s4);
// Start by 2
// Next x or y or z
// Followed by any one or more times.
// Combine the rules: [abc]. , +
// ==> true
match = s4.matches("2[xyz].+");
System.out.println("-Match 2[xyz].+ " + match);
String s5 = "2bkbv";
// Start any one or more times
// Followed by a or b, or c: [abc]
// Next z or v: [zv]
// Followed by any
// ==> true
match = s5.matches(".+[abc][zv].*");
System.out.println("-Match .+[abc][zv].* " + match);
}
}
Les résultats d'exécution de l'exemple :
s1=a
-Match . true
s1=abc
-Match . false
-Match .* true
s2=m
-Match ^m true
s2=mnp
-Match ^m false
-Match ^m.+ true
s3=p
-Match p$ true
s3=2nnp
-Match p$ false
-Match .n{1,3}p$ true
s4=2ybcd
-Match 2[xyz].+ true
-Match .+[abc][zv].* true
SplitWithRegex.java
package org.o7planning.tutorial.regex.stringmatches;
public class SplitWithRegex {
public static final String TEXT = "This is my text";
public static void main(String[] args) {
System.out.println("TEXT=" + TEXT);
// White space appears one or more times.
// The whitespace characters: \t \n \x0b \r \f
// Combining rules: \ s and +
String regex = "\\s+";
String[] splitString = TEXT.split(regex);
// 4
System.out.println(splitString.length);
for (String string : splitString) {
System.out.println(string);
}
// Replace all whitespace with tabs
String newText = TEXT.replaceAll("\\s+", "\t");
System.out.println("New text=" + newText);
}
}
Les résultats d'exécution de l'exemple :
TEXT=This is my text
4
This
is
my
text
New text=This is my text
EitherOrCheck.java
package org.o7planning.tutorial.regex.stringmatches;
public class EitherOrCheck {
public static void main(String[] args) {
String s = "The film Tom and Jerry!";
// Check the whole s
// Begin by any characters appear 0 or more times
// Next Tom or Jerry
// End with any characters appear 0 or more times
// Combine the rules:., *, X | Z
// ==> true
boolean match = s.matches(".*(Tom|Jerry).*");
System.out.println("s=" + s);
System.out.println("-Match .*(Tom|Jerry).* " + match);
s = "The cat";
// ==> false
match = s.matches(".*(Tom|Jerry).*");
System.out.println("s=" + s);
System.out.println("-Match .*(Tom|Jerry).* " + match);
s = "The Tom cat";
// ==> true
match = s.matches(".*(Tom|Jerry).*");
System.out.println("s=" + s);
System.out.println("-Match .*(Tom|Jerry).* " + match);
}
}
Les résultats d'exécution de l'exemple :
s=The film Tom and Jerry!
-Match .*(Tom|Jerry).* true
s=The cat
-Match .*(Tom|Jerry).* false
s=The Tom cat
-Match .*(Tom|Jerry).* true
5. Utilisation de Pattern et Matcher
1. Objet Pattern est la version qui a été compilée par une expression régulière. Il n'a pas de constructeur publique et nous utilisons sa méthode statique compile(String) pour créer les modèles de l'objet avec le paramètre d'une expression régulière.
2. Matcher est l'objet moteur de regex qui correspond au motif de la chaîne d'entrée avec le modèle d'objet créé ci-dessus. Cette classe n'a pas de constructeur public et nous prenons un objet Matcher en utilisant de la méthode matcher (String) de l'objet Pattern. Avec le paramètre d'entrée de la chaîne est le texte à vérifier.
3. PatternSyntaxException sera jeté dehors si la grammaire de l'expression régulière est incorrecte.
2. Matcher est l'objet moteur de regex qui correspond au motif de la chaîne d'entrée avec le modèle d'objet créé ci-dessus. Cette classe n'a pas de constructeur public et nous prenons un objet Matcher en utilisant de la méthode matcher (String) de l'objet Pattern. Avec le paramètre d'entrée de la chaîne est le texte à vérifier.
3. PatternSyntaxException sera jeté dehors si la grammaire de l'expression régulière est incorrecte.
String regex= ".xx.";
// Create a Pattern object through a static method.
Pattern pattern = Pattern.compile(regex);
// Get a Matcher object
Matcher matcher = pattern.matcher("MxxY");
boolean match = matcher.matches();
System.out.println("Match "+ match);
- Class Pattern:
public static Pattern compile(String regex, int flags) ;
public static Pattern compile(String regex);
public Matcher matcher(CharSequence input);
public static boolean matches(String regex, CharSequence input);
- Class Matcher:
public int start()
public int start(int group)
public int end()
public int end(int group)
public String group()
public String group(int group)
public String group(String name)
public int groupCount()
public boolean matches()
public boolean lookingAt()
public boolean find()
Voici un exemple d'utilisation Matcher et la méthode find() pour chercher une sous-chaîne correspondant à une expression régulière.
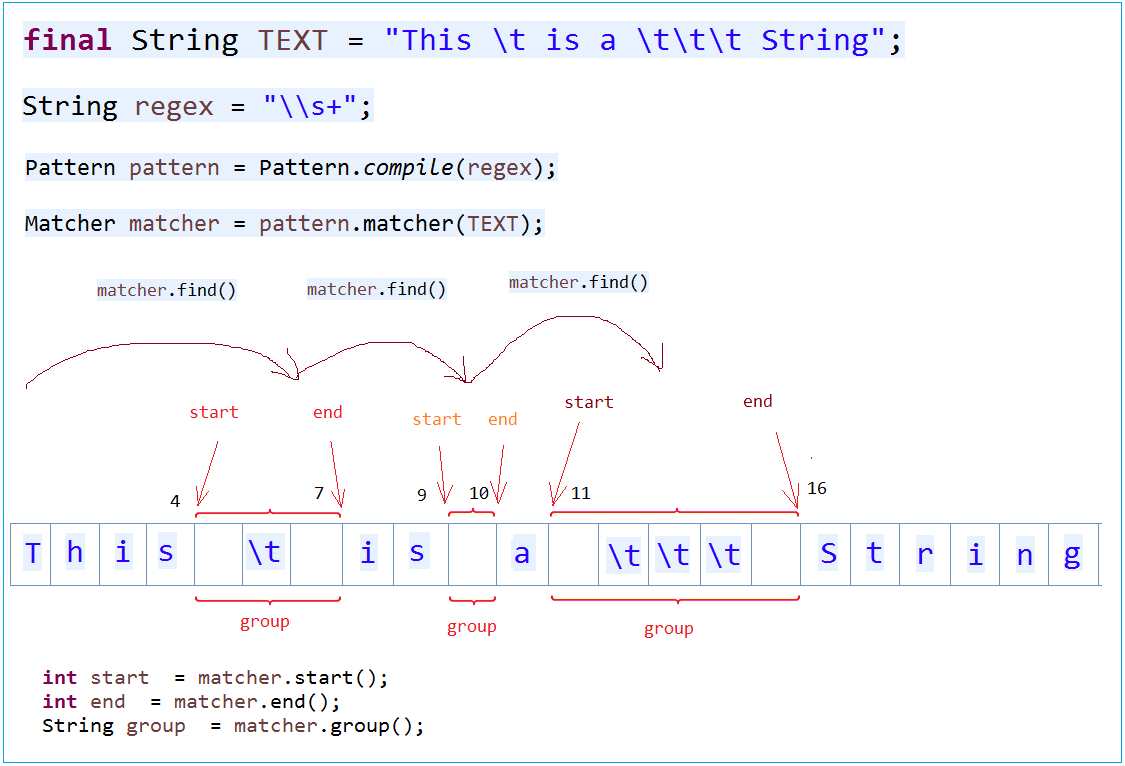
MatcherFind.java
package org.o7planning.tutorial.regex;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class MatcherFind {
public static void main(String[] args) {
final String TEXT = "This \t is a \t\t\t String";
// Spaces appears one or more time.
String regex = "\\s+";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(TEXT);
int i = 0;
while (matcher.find()) {
System.out.print("start" + i + " = " + matcher.start());
System.out.print(" end" + i + " = " + matcher.end());
System.out.println(" group" + i + " = " + matcher.group());
i++;
}
}
}
Les résultats d'exécution de l'exemple :
start = 4 end = 7 group =
start = 9 end = 10 group =
start = 11 end = 16 group =
Method Matcher.lookingAt()
MatcherLookingAt.java
package org.o7planning.tutorial.regex;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class MatcherLookingAt {
public static void main(String[] args) {
String country1 = "iran";
String country2 = "Iraq";
// Start by I followed by any character.
// Following is the letter a or e.
String regex = "^I.[ae]";
Pattern pattern = Pattern.compile(regex, Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(country1);
// lookingAt () searches that match the first part.
System.out.println("lookingAt = " + matcher.lookingAt());
// matches() must be matching the entire
System.out.println("matches = " + matcher.matches());
// Reset matcher with new text: country2
matcher.reset(country2);
System.out.println("lookingAt = " + matcher.lookingAt());
System.out.println("matches = " + matcher.matches());
}
}
6. Groupe (Group)
Une expression régulière que vous pouvez diviser en groupes (group) :
// A regular expression
String regex = "\\s+=\\d+";
// Writing as three group, by marking ()
String regex2 = "(\\s+)(=)(\\d+)";
// Two group
String regex3 = "(\\s+)(=\\d+)";
Le groupe peut être imbriqué et donc besoin d'une règle d'indexation du groupe. Le modèle entier est défini comme le groupe 0. Le groupe restant a décrit une illustration similaire ci-dessous :

Remarque : Utilisez les ( ?:Pattern) pour informer à Java de ne pas considérer cela comme un groupe (aucune capture) (None-capturing group)
Depuis Java 7, vous pouvez définir un groupe nommé (?<name>pattern), et vous pouvez accéder à le contenu qui correspond à Matcher.groupe(String name). Ce Regex est plus long, mais ce code est plus significatif lorsqu'il précise ce que vous êtes en train de correspondre à ou d'extraire avec le regex.
Le groupe nommé peut également être consulté via Matcher.group (groupe int) avec le schéma de numérotation similaire.
En interne, l'implémentation de Java ne fait que mapper du nom au numéro de groupe. Par conséquent, vous ne pouvez pas utiliser le même nom pour 2 groupes de capture différents.
Le groupe nommé peut également être consulté via Matcher.group (groupe int) avec le schéma de numérotation similaire.
En interne, l'implémentation de Java ne fait que mapper du nom au numéro de groupe. Par conséquent, vous ne pouvez pas utiliser le même nom pour 2 groupes de capture différents.
-
Voyez un exemple qui utilise le nom pour le groupe (Java >=7)
NamedGroup.java
package org.o7planning.tutorial.regex;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class NamedGroup {
public static void main(String[] args) {
final String TEXT = " int a = 100;float b= 130;float c= 110 ; ";
// Use (?<groupName>pattern) to define a group named: groupName
// Defined group named declare: using (?<declare>...)
// And a group named value: use: (?<value>..)
String regex = "(?<declare>\\s*(int|float)\\s+[a-z]\\s*)=(?<value>\\s*\\d+\\s*);";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(TEXT);
while (matcher.find()) {
String group = matcher.group();
System.out.println(group);
System.out.println("declare: " + matcher.group("declare"));
System.out.println("value: " + matcher.group("value"));
System.out.println("------------------------------");
}
}
}
Les résultats d'exécution de l’exemple :
int a = 100;
declare: int a
value: 100
------------------------------
float b= 130;
declare: float b
value: 130
------------------------------
float c= 110 ;
declare: float c
value: 110
------------------------------
Pour mieux comprendre vous pouvez voir les illustrations ci-dessous :
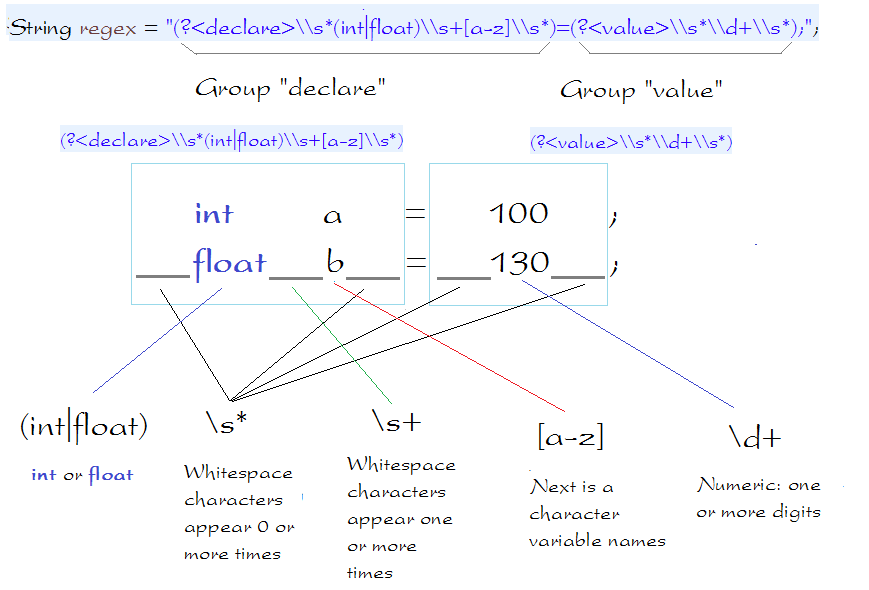
7. Utilisation de Pattern, Matcher, Group et *?
Dans certaines situations *? très important, s'il vous plaît voyez l'exemple suivant :
// This is a regex
// any characters appear 0 or more times,
// followed by ' and >
String regex = ".*'>";
// TEXT1 match the regex.
String TEXT1 = "FILE1'>";
// And TEXT2 match the regex
String TEXT2 = "FILE1'> <a href='http://HOST/file/FILE2'>";
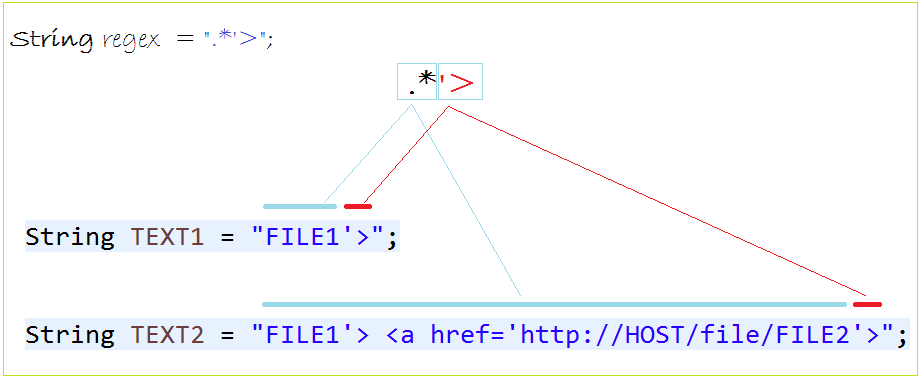
*? trouveront une minimum correspondance appropriée. Nous voyons l'exemple suivant :
NamedGroup2.java
package org.o7planning.tutorial.regex;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class NamedGroup2 {
public static void main(String[] args) {
String TEXT = "<a href='http://HOST/file/FILE1'>File 1</a>"
+ "<a href='http://HOST/file/FILE2'>File 2</a>";
// Java >= 7.
// Define group named fileName.
// *? ==> ? after a quantifier makes it a reluctant quantifier.
// It tries to find the smallest match.
String regex = "/file/(?<fileName>.*?)'>";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(TEXT);
while (matcher.find()) {
System.out.println("File Name = " + matcher.group("fileName"));
}
}
}
Les résultats d'exécution de l’exemple :
File Name = FILE1
File Name = FILE2
Java Basic
- Personnaliser le compilateur Java pour traiter votre annotation (Annotation Processing Tool)
- Programmation Java pour l'équipe utilisant Eclipse et SVN
- Le Tutoriel de Java WeakReference
- Le Tutoriel de Java PhantomReference
- Tutoriel sur la compression et la décompression Java
- Configuration d'Eclipse pour utiliser le JDK au lieu de JRE
- Méthodes Java String.format() et printf()
- Syntaxe et nouvelles fonctionnalités de Java 8
- Expression régulière en Java
- Tutoriel de programmation Java multithreading
- Bibliothèques de pilotes JDBC pour différents types de bases de données en Java
- Tutoriel Java JDBC
- Obtenir des valeurs de colonne automatiquement incrémentées lors de l'insertion d'un enregistrement à l'aide de JDBC
- Le Tutoriel de Java Stream
- Le Tutoriel de Java Functional Interface
- Introduction à Raspberry Pi
- Le Tutoriel de Java Predicate
- Classe abstraite et interface en Java
- Modificateurs d'accès en Java
- Le Tutoriel de Java Enum
- Le Tutoriel de Java Annotation
- Comparer et trier en Java
- Le Tutoriel de Java String, StringBuffer et StringBuilder
- Tutoriel de gestion des exceptions Java
- Le Tutoriel de Java Generics
- Manipulation de fichiers et de répertoires en Java
- Le Tutoriel de Java BiPredicate
- Le Tutoriel de Java Consumer
- Le Tutoriel de Java BiConsumer
- Qu'est-ce qui est nécessaire pour commencer avec Java?
- L'histoire de Java et la différence entre Oracle JDK et OpenJDK
- Installer Java sur Windows
- Installer Java sur Ubuntu
- Installer OpenJDK sur Ubuntu
- Installer Eclipse
- Installer Eclipse sur Ubuntu
- Le Tutoriel Java pour débutant
- Histoire des bits et des bytes en informatique
- Types de données dans Java
- Opérations sur les bits
- Le Tutoriel de instruction Java If else
- Le Tutoriel de instruction Java Switch
- Les Boucles en Java
- Les Tableaux (Array) en Java
- JDK Javadoc au format CHM
- Héritage et polymorphisme en Java
- Le Tutoriel de Java Function
- Le Tutoriel de Java BiFunction
- Exemple de Java encoding et decoding utilisant Apache Base64
- Le Tutoriel de Java Reflection
- Invocation de méthode à distance en Java
- Le Tutoriel de Java Socket
- Quelle plate-forme devez-vous choisir pour développer des applications de bureau Java?
- Le Tutoriel de Java Commons IO
- Le Tutoriel de Java Commons Email
- Le Tutoriel de Java Commons Logging
- Comprendre Java System.identityHashCode, Object.hashCode et Object.equals
- Le Tutoriel de Java SoftReference
- Le Tutoriel de Java Supplier
- Programmation orientée aspect Java avec AspectJ (AOP)
Show More
- Tutoriels de programmation Java Servlet/JSP
- Tutoriels de Java Collections Framework
- Tutoriels Java API pour HTML & XML
- Tutoriels Java IO
- Tutoriels Java Date Time
- Tutoriels Spring Boot
- Tutoriels Maven
- Tutoriels Gradle
- Tutoriels Java Web Service
- Tutoriels de programmation Java SWT
- Tutoriels de JavaFX
- Tutoriels Java Oracle ADF
- Tutoriels Struts2
- Tutoriels Spring Cloud