Le Tutoriel de Java BiPredicate
1. BiPredicate
Dans Java 8, BiPredicate est une functional interface, qui représente un opérateur lequel accepte deux paramètres d'entrée et renvoie une valeur boolean.
BiPredicate interface
@FunctionalInterface
public interface BiPredicate<T, U> {
boolean test(T t, U u);
default BiPredicate<T, U> and(BiPredicate<? super T, ? super U> other) {
Objects.requireNonNull(other);
return (T t, U u) -> test(t, u) && other.test(t, u);
}
default BiPredicate<T, U> negate() {
return (T t, U u) -> !test(t, u);
}
default BiPredicate<T, U> or(BiPredicate<? super T, ? super U> other) {
Objects.requireNonNull(other);
return (T t, U u) -> test(t, u) || other.test(t, u);
}
}
Voir aussi: Predicate est une functional interface similaire à BiPredicate, qui n'accepte qu'un seul paramètre:
Exemple: Créer un objet BiPredicate pour vérifier si la longueur d'une String correspond à un nombre donné.
BiPredicateEx1.java
package org.o7planning.bipredicate.ex;
import java.util.function.BiPredicate;
public class BiPredicateEx1 {
public static void main(String[] args) {
// Create a BiPredicate.
BiPredicate<String,Integer> tester = (aString, aNumber) -> {
return aString.length() == aNumber;
};
// Test:
boolean testResult = tester.test("o7planning.org", 14);
System.out.println("Test Result: " + testResult);
}
}
Output:
Test Result: true
Consulter l'exemple ci-dessus. Un objet Map<String,Integer> contient des mappages entre une String et sa longueur. Cependant, il existe des mappages incorrects. Imprimer les mappages corrects.
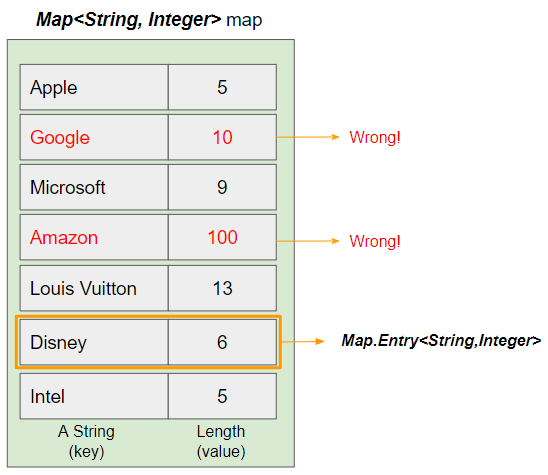
BiPredicateEx2.java
package org.o7planning.bipredicate.ex;
import java.util.HashMap;
import java.util.Map;
import java.util.function.BiPredicate;
public class BiPredicateEx2 {
public static void main(String[] args) {
// Create a BiPredicate.
BiPredicate<String,Integer> tester = (aString, aNumber) -> {
return aString.length() == aNumber;
};
// String aString --> Integer length.
Map<String,Integer> map = new HashMap<String,Integer>();
map.put("Apple", 5);
map.put("Google", 10); // Wrong
map.put("Microsoft", 9);
map.put("Amazon", 100); // Wrong
map.put("Louis Vuitton", 13);
map.put("Disney", 6);
map.put("Intel", 5);
map.entrySet() // Set<Map.Entry<String,Integer>>
.stream() // Stream<Map.Entry<String,Integer>>
// filter(Predicate<Map.Entry<String,Integer>>)
.filter(entry -> tester.test(entry.getKey(), entry.getValue())) // Stream<Map.Entry<String,Integer>>
.forEach(entry -> System.out.println(entry.getKey() +" --> " + entry.getValue()));
}
}
Output:
Apple --> 5
Disney --> 6
Louis Vuitton --> 13
Microsoft --> 9
Intel --> 5
3. BiPredicate + Method reference
Si une méthode statique prend 2 paramètres et renvoie un boolean, alors sa référence est considérée comme un BiPredicate:
Par exemple:
BiPredicate_mRef_ex1.java
package org.o7planning.bipredicate.ex;
import java.util.function.BiPredicate;
public class BiPredicate_mRef_ex1 {
public static void main(String[] args) {
// Create a BiPredicate from a Method reference.
BiPredicate<Staff,Integer> tester = StaffUtils::higher;
Staff tom = new Staff("Tom", 3000);
boolean testResult = tester.test(tom, 2000);
System.out.println("Test Result: " + testResult);
}
}
class StaffUtils {
// A static method take 2 input parameters and return boolean type.
public static boolean higher(Staff staff, int salary) {
return staff.getSalary() > salary;
}
}
class Staff {
private String fullName;
private int salary;
public Staff(String fullName, int salary) {
this.fullName = fullName;
this.salary = salary;
}
public String getFullName() {
return fullName;
}
public int getSalary() {
return salary;
}
}
Output:
Test Result: true
4. negate()
negate() renvoie un nouvel objet BiPredicate dont l'évaluation est négative du BiPredicate actuel.
default BiPredicate<T, U> negate() {
return (T t, U u) -> !test(t, u);
}
Exemple: Un BiPredicate<Integer,Integer> qui vérifie 2 chiffres pour vérifier si le premier est supérieur au deuxième.
BiPredicate_negate_ex1.java
package org.o7planning.bipredicate.ex;
import java.util.function.BiPredicate;
public class BiPredicate_negate_ex1 {
public static void main(String[] args) {
// Create a BiPredicate.
// Check if anInteger1 > anInteger2
BiPredicate<Integer, Integer> tester = (anInteger1, anInteger2) -> {
return anInteger1 > anInteger2;
};
boolean test1 = tester.test(100, 10);
boolean test2 = tester.negate().test(100, 10);
System.out.println("Test1: " + test1);
System.out.println("Test2: " + test2);
}
}
Output:
Test1: true
Test2: false
Exemple: Un objet Map<String,Integer> contient des mappages entre une String et sa longueur. Cependant, il existe des mappages incorrects. Imprimer ces mappages incorrects.
BiPredicate_negate_ex2.java
package org.o7planning.bipredicate.ex;
import java.util.HashMap;
import java.util.Map;
import java.util.function.BiPredicate;
public class BiPredicate_negate_ex2 {
public static void main(String[] args) {
// Create a BiPredicate.
// Check if length of a String equals to aNumber.
BiPredicate<String,Integer> tester = (aString, aNumber) -> {
return aString.length() == aNumber;
};
// String aString --> Integer length.
Map<String,Integer> map = new HashMap<String,Integer>();
map.put("Apple", 5);
map.put("Google", 10); // Wrong
map.put("Microsoft", 9);
map.put("Amazon", 100); // Wrong
map.put("Louis Vuitton", 13);
map.put("Disney", 6);
map.put("Intel", 5);
map.entrySet() // Set<Map.Entry<String,Integer>>
.stream() // Stream<Map.Entry<String,Integer>>
// filter(Predicate<Map.Entry<String,Integer>>)
.filter(entry -> tester.negate().test(entry.getKey(), entry.getValue())) // Stream<Map.Entry<String,Integer>>
.forEach(entry -> System.out.println(entry.getKey() +" --> " + entry.getValue()));
}
}
Output:
Google --> 10
Amazon --> 100
5. and(BiPredicate other)
La méthode and(other) crée un nouvel objet BiPredicate en combinant le BiPredicate actuel et other. Il est évalué comme true si le BiPredicate actuel et other sont évalués comme true.
default BiPredicate<T, U> and(BiPredicate<? super T, ? super U> other) {
Objects.requireNonNull(other);
return (T t, U u) -> test(t, u) && other.test(t, u);
}
Par exemple:
BiPredicate_and_ex1.java
package org.o7planning.bipredicate.ex;
import java.util.function.BiPredicate;
public class BiPredicate_and_ex1 {
public static void main(String[] args) {
// Test if anInteger1 > anInteger2
BiPredicate<Integer, Integer> tester1 = (anInteger1, anInteger2) -> {
return anInteger1 > anInteger2;
};
// Test if anInteger1 + anInteger2 > 100
BiPredicate<Integer, Integer> tester2 = (anInteger1, anInteger2) -> {
return anInteger1 + anInteger2 > 100;
};
// Test if number1 > number2 and number1 + number2 > 100.
boolean testResult1 = tester1.and(tester2).test(70, 45);
boolean testResult2 = tester1.and(tester2).test(45, 70);
System.out.println("Test Result 1: " + testResult1);
System.out.println("Test Result 2: " + testResult2);
}
}
Output:
Test Result 1: true
Test Result 2: false
6. or(BiPredicate other)
La méthode or(other) renvoie un nouvel objet BiPredicate en combinant le BiPredicate actuel et other. Il est évalué comme true si l'un ou l'autre, le BiPredicate actuel ou other est évalué comme true.
default BiPredicate<T, U> or(BiPredicate<? super T, ? super U> other) {
Objects.requireNonNull(other);
return (T t, U u) -> test(t, u) || other.test(t, u);
}
Par exemple:
BiPredicate_or_ex1.java
package org.o7planning.bipredicate.ex;
import java.util.function.BiPredicate;
public class BiPredicate_or_ex1 {
public static void main(String[] args) {
// Test if anInteger1 > anInteger2
BiPredicate<Integer, Integer> tester1 = (anInteger1, anInteger2) -> {
return anInteger1 > anInteger2;
};
// Test if anInteger1 + anInteger2 > 100
BiPredicate<Integer, Integer> tester2 = (anInteger1, anInteger2) -> {
return anInteger1 + anInteger2 > 100;
};
// Test if number1 > number2 or number1 + number2 > 100.
boolean testResult1 = tester1.or(tester2).test(70, 45);
boolean testResult2 = tester1.or(tester2).test(45, 70);
System.out.println("Test Result 1: " + testResult1);
System.out.println("Test Result 2: " + testResult2);
}
}
Output:
Test Result 1: true
Test Result 2: true
Java Basic
- Personnaliser le compilateur Java pour traiter votre annotation (Annotation Processing Tool)
- Programmation Java pour l'équipe utilisant Eclipse et SVN
- Le Tutoriel de Java WeakReference
- Le Tutoriel de Java PhantomReference
- Tutoriel sur la compression et la décompression Java
- Configuration d'Eclipse pour utiliser le JDK au lieu de JRE
- Méthodes Java String.format() et printf()
- Syntaxe et nouvelles fonctionnalités de Java 8
- Expression régulière en Java
- Tutoriel de programmation Java multithreading
- Bibliothèques de pilotes JDBC pour différents types de bases de données en Java
- Tutoriel Java JDBC
- Obtenir des valeurs de colonne automatiquement incrémentées lors de l'insertion d'un enregistrement à l'aide de JDBC
- Le Tutoriel de Java Stream
- Le Tutoriel de Java Functional Interface
- Introduction à Raspberry Pi
- Le Tutoriel de Java Predicate
- Classe abstraite et interface en Java
- Modificateurs d'accès en Java
- Le Tutoriel de Java Enum
- Le Tutoriel de Java Annotation
- Comparer et trier en Java
- Le Tutoriel de Java String, StringBuffer et StringBuilder
- Tutoriel de gestion des exceptions Java
- Le Tutoriel de Java Generics
- Manipulation de fichiers et de répertoires en Java
- Le Tutoriel de Java BiPredicate
- Le Tutoriel de Java Consumer
- Le Tutoriel de Java BiConsumer
- Qu'est-ce qui est nécessaire pour commencer avec Java?
- L'histoire de Java et la différence entre Oracle JDK et OpenJDK
- Installer Java sur Windows
- Installer Java sur Ubuntu
- Installer OpenJDK sur Ubuntu
- Installer Eclipse
- Installer Eclipse sur Ubuntu
- Le Tutoriel Java pour débutant
- Histoire des bits et des bytes en informatique
- Types de données dans Java
- Opérations sur les bits
- Le Tutoriel de instruction Java If else
- Le Tutoriel de instruction Java Switch
- Les Boucles en Java
- Les Tableaux (Array) en Java
- JDK Javadoc au format CHM
- Héritage et polymorphisme en Java
- Le Tutoriel de Java Function
- Le Tutoriel de Java BiFunction
- Exemple de Java encoding et decoding utilisant Apache Base64
- Le Tutoriel de Java Reflection
- Invocation de méthode à distance en Java
- Le Tutoriel de Java Socket
- Quelle plate-forme devez-vous choisir pour développer des applications de bureau Java?
- Le Tutoriel de Java Commons IO
- Le Tutoriel de Java Commons Email
- Le Tutoriel de Java Commons Logging
- Comprendre Java System.identityHashCode, Object.hashCode et Object.equals
- Le Tutoriel de Java SoftReference
- Le Tutoriel de Java Supplier
- Programmation orientée aspect Java avec AspectJ (AOP)
Show More
- Tutoriels de programmation Java Servlet/JSP
- Tutoriels de Java Collections Framework
- Tutoriels Java API pour HTML & XML
- Tutoriels Java IO
- Tutoriels Java Date Time
- Tutoriels Spring Boot
- Tutoriels Maven
- Tutoriels Gradle
- Tutoriels Java Web Service
- Tutoriels de programmation Java SWT
- Tutoriels de JavaFX
- Tutoriels Java Oracle ADF
- Tutoriels Struts2
- Tutoriels Spring Cloud