Le Tutoriel de Java String, StringBuffer et StringBuilder
1. Héritage hiérarchique
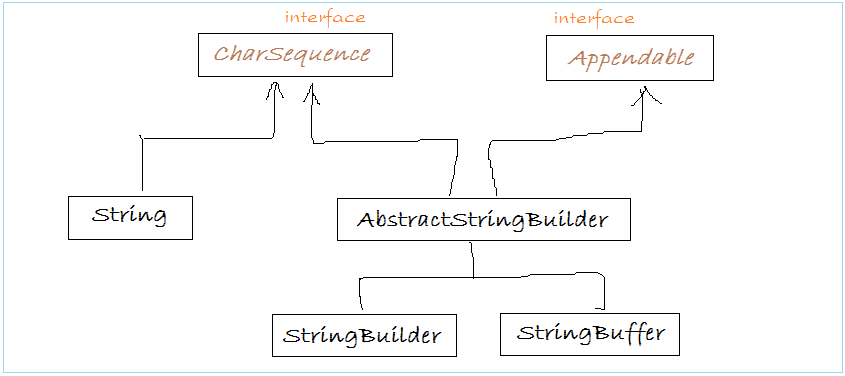
Lorsque vous travaillez avec des données textuelles, Java vous fournit trois classes String, StringBuffer et StringBuilder. Si vous travaillez avec de grandes données, vous devriez utiliser StringBuffer ou StringBuilder pour optimiser l'efficacité. Fondamentalement, trois classes ont beaucoup de similarités.
StringBuilder et StringBuffer sont semblables, sauf la situation d'utilisation liée à Multi Thread.
- String est immuable, ce concept sera discuté en détail dans le document et ne permet pas des sous-classes à exister.
- StringBuffer, StringBuilder sont muable.
StringBuilder et StringBuffer sont semblables, sauf la situation d'utilisation liée à Multi Thread.
- Lorsque vous traitez le texte de multi-thread, vous devriez utiliser StringBuffer afin d'éviter les conflits entre des threads.
- SLorsque vous traitez le texte d'un thread, vous devriez utiliser StringBuilder.
2. Concept mutable & immutable
Observez un exemple d'illustration :
// This is a class with value field and name field.
// When you create this class, you cannot reset the value and all other fields from outside.
// This class does not have methods for the purpose of resetting fields from outside.
// It means this class is immutable
public class ImmutableClassExample {
private int value;
private String name;
public ImmutableClassExample(String name, int value) {
this.value = value;
this.name= name;
}
public String getName() {
return name;
}
public int getValue() {
return value;
}
}
// This is a class owning a value field.
// After creating the object, you can reset values of the value field by calling setNewValue(int) method.
// This is a mutable class.
public class MutableClassExample {
private int value;
public MutableClassExample(int value) {
this.value= value;
}
public void setNewValue(int newValue) {
this.value = newValue;
}
}
String est une classe immuable. String contient de nombreux attributs (champs) tels que la longueur, mais des valeur dans les champs ne peuvent pas être modifiées.
3. String
String est l'une des classes très importantes en Java et toute personne qui commence par la programmation Java doit utiliser des instructions célèbres System.out.println () pour imprimer quelque chose sur la Console. Beaucoup de débutants de Java ne savent pas que String est immuable et est final (c'est-à-dire qu'il n'autorise pas de la classe à hériter), toutes des modifications apportées sur String produisent un autre objet String.
String est vraiment une classe très spéciale
En Java, String est une classe spéciale, la raison est qu'elle est régulièrement utilisée dans un programme donc elle doit contenir la performance et la flexibilité nécessaires. C'est pourquoi String a un objet et deux propriétés primitifs.
String est primitif :
Vous pouvez créer un string literal (chaîne littérale), string literal est stocké dans la pile (stack), n'a pas besoin de grand espace de stockage, et est moins coûteux à manipuler.
Les string literal sont contenus dans un réservoir (common pool). Donc les deux strings literal ont le même contenu en utilisant la même zone de mémoire sur la pile, cela permet d'économiser de la mémoire.
- String literal = "Hello World";
Les string literal sont contenus dans un réservoir (common pool). Donc les deux strings literal ont le même contenu en utilisant la même zone de mémoire sur la pile, cela permet d'économiser de la mémoire.
String est un objet
Puisque String est une classe donc elle peut être créée par le nouvel opérateur.
- String object = new String("Hello World");
Par exemple :
// Implicit construction via string literal
String str1 = "Java is Hot";
// Explicit construction via new
String str2 = new String("I'm cool");
String Literal vs. String Object
Tel que mentionné, il y a deux façons de construction d'une chaîne (String) : construction implicite en assignant une chaîne littérale (String literal) ou en créant explicitement un String objet via le nouvel opérateur et le constructeur. Par exemple :
String s1 = "Hello"; // String literal
String s2 = "Hello"; // String literal
String s3 = s1; // same reference
String s4 = new String("Hello"); // String object
String s5 = new String("Hello"); // String object
Nous allons expliquer par l'illustration ci-dessous :
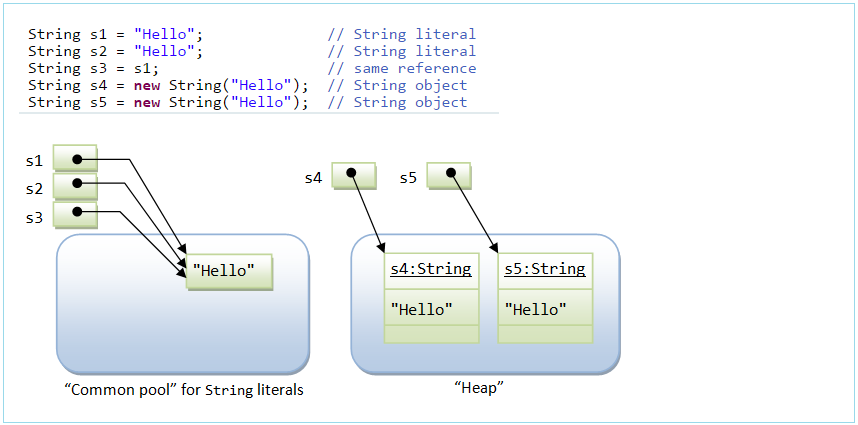
Les strings literal ont le même contenu, elles partageront le même emplacement de stockage dans le réservoir commun (common pool). Pendant ce temps, les objets String sont stockés dans le Heap et ne partagent pas les emplacements de stockage, y compris deux objets string qui ont le même contenu.
equals() vs ==
La méthode equals() sert à comparer deux objets, pour String il signifie la comparaison du contenu de deux chaines. Pour les types de référence (reference), l'opérateur == signifie la comparaison de l'adresse de la zone de stockage des objets. Observez l'exemple :
String s1 = "Hello"; // String literal
String s2 = "Hello"; // String literal
String s3 = s1; // same reference
String s4 = new String("Hello"); // String object
String s5 = new String("Hello"); // String object
s1 == s1; // true, same pointer
s1 == s2; // true, s1 and s2 share storage in common pool
s1 == s3; // true, s3 is assigned same pointer as s1
s1 == s4; // false, different pointers
s4 == s5; // false, different pointers in heap
s1.equals(s3); // true, same contents
s1.equals(s4); // true, same contents
s4.equals(s5); // true, same contents
En fait, vous devez utiliser string literal, au lieu d'utiliser l'opérateur "new" qui vous permet d'accélérer votre programme.
Les méthodes de String
Voici les méthodes de String :
SN | Methods | Description |
1 | char charAt(int index) | Returns the character at the specified index. |
2 | int compareTo(Object o) | Compares this String to another Object. |
3 | int compareTo(String anotherString) | Compares two strings lexicographically. |
4 | int compareToIgnoreCase(String str) | Compares two strings lexicographically, ignoring case differences. |
5 | String concat(String str) | Concatenates the specified string to the end of this string. |
6 | boolean contentEquals(StringBuffer sb) | Returns true if and only if this String represents the same sequence of characters as the specified StringBuffer. |
7 | static String copyValueOf(char[] data) | Returns a String that represents the character sequence in the array specified. |
8 | static String copyValueOf(char[] data, int offset, int count) | Returns a String that represents the character sequence in the array specified. |
9 | boolean endsWith(String suffix) | Tests if this string ends with the specified suffix. |
10 | boolean equals(Object anObject) | Compares this string to the specified object. |
11 | boolean equalsIgnoreCase(String anotherString) | Compares this String to another String, ignoring case considerations. |
12 | byte getBytes() | Encodes this String into a sequence of bytes using the platform's default charset, storing the result into a new byte array. |
13 | byte[] getBytes(String charsetName) | Encodes this String into a sequence of bytes using the named charset, storing the result into a new byte array. |
14 | void getChars(int srcBegin, int srcEnd, char[] dst, int dstBegin) | Copies characters from this string into the destination character array. |
15 | int hashCode() | Returns a hash code for this string. |
16 | int indexOf(int ch) | Returns the index within this string of the first occurrence of the specified character. |
17 | int indexOf(int ch, int fromIndex) | Returns the index within this string of the first occurrence of the specified character, starting the search at the specified index. |
18 | int indexOf(String str) | Returns the index within this string of the first occurrence of the specified substring. |
19 | int indexOf(String str, int fromIndex) | Returns the index within this string of the first occurrence of the specified substring, starting at the specified index. |
20 | String intern() | Returns a canonical representation for the string object. |
21 | int lastIndexOf(int ch) | Returns the index within this string of the last occurrence of the specified character. |
22 | int lastIndexOf(int ch, int fromIndex) | Returns the index within this string of the last occurrence of the specified character, searching backward starting at the specified index. |
23 | int lastIndexOf(String str) | Returns the index within this string of the rightmost occurrence of the specified substring. |
24 | int lastIndexOf(String str, int fromIndex) | Returns the index within this string of the last occurrence of the specified substring, searching backward starting at the specified index. |
25 | int length() | Returns the length of this string. |
26 | boolean matches(String regex) | Tells whether or not this string matches the given regular expression. |
27 | boolean regionMatches(boolean ignoreCase, int toffset, String other, int ooffset, int len) | Tests if two string regions are equal. |
28 | boolean regionMatches(int toffset, String other, int ooffset, int len) | Tests if two string regions are equal. |
29 | String replace(char oldChar, char newChar) | Returns a new string resulting from replacing all occurrences of oldChar in this string with newChar. |
30 | String replaceAll(String regex, String replacement) | Replaces each substring of this string that matches the given regular expression with the given replacement. |
31 | String replaceFirst(String regex, String replacement) | Replaces the first substring of this string that matches the given regular expression with the given replacement. |
32 | String[] split(String regex) | Splits this string around matches of the given regular expression. |
33 | String[] split(String regex, int limit) | Splits this string around matches of the given regular expression. |
34 | boolean startsWith(String prefix) | Tests if this string starts with the specified prefix. |
35 | boolean startsWith(String prefix, int toffset) | Tests if this string starts with the specified prefix beginning a specified index. |
36 | CharSequence subSequence(int beginIndex, int endIndex) | Returns a new character sequence that is a subsequence of this sequence. |
37 | String substring(int beginIndex) | Returns a new string that is a substring of this string. |
38 | String substring(int beginIndex, int endIndex) | Returns a new string that is a substring of this string. |
39 | char[] toCharArray() | Converts this string to a new character array. |
40 | String toLowerCase() | Converts all of the characters in this String to lower case using the rules of the default locale. |
41 | String toLowerCase(Locale locale) | Converts all of the characters in this String to lower case using the rules of the given Locale. |
42 | String toString() | This object (which is already a string!) is itself returned. |
43 | String toUpperCase() | Converts all of the characters in this String to upper case using the rules of the default locale. |
44 | String toUpperCase(Locale locale) | Converts all of the characters in this String to upper case using the rules of the given Locale. |
45 | String trim() | Returns a copy of the string, with leading and trailing whitespace omitted. |
46 | static String valueOf(primitive data type x) | Returns the string representation of the passed data type argument. |
4. StringBuffer vs StringBuilder
StringBuilder et StringBuffer sont très similaires, la différence est que toutes les méthodes StringBuffer sont synchronisées, il est approprié lorsque vous travaillez avec une application multi-thread, car plusieurs threads peuvent accéder à un objet StringBuffer en même temps. Pendant ce temps, StringBuilder a des méthodes similaires, mais elles ne sont pas synchronisées, mais comme ses performances sont plus élevées, vous devriez utiliser StringBuilder dans des applications à thread unique ou l'utiliser comme variable locale dans une méthode.
Les méthodes de StringBuffer (StringBuilder similaire)
// Constructors
StringBuffer() // an initially-empty StringBuffer
StringBuffer(int size) // with the specified initial size
StringBuffer(String s) // with the specified initial content
// Length
int length()
// Methods for building up the content
// type could be primitives, char[], String, StringBuffer, etc
StringBuffer append(type arg) // ==> note above!
StringBuffer insert(int offset, type arg) // ==> note above!
// Methods for manipulating the content
StringBuffer delete(int start, int end)
StringBuffer deleteCharAt(int index)
void setLength(int newSize)
void setCharAt(int index, char newChar)
StringBuffer replace(int start, int end, String s)
StringBuffer reverse()
// Methods for extracting whole/part of the content
char charAt(int index)
String substring(int start)
String substring(int start, int end)
String toString()
// Methods for searching
int indexOf(String searchKey)
int indexOf(String searchKey, int fromIndex)
int lastIndexOf(String searchKey)
int lastIndexOf(String searchKey, int fromIndex)
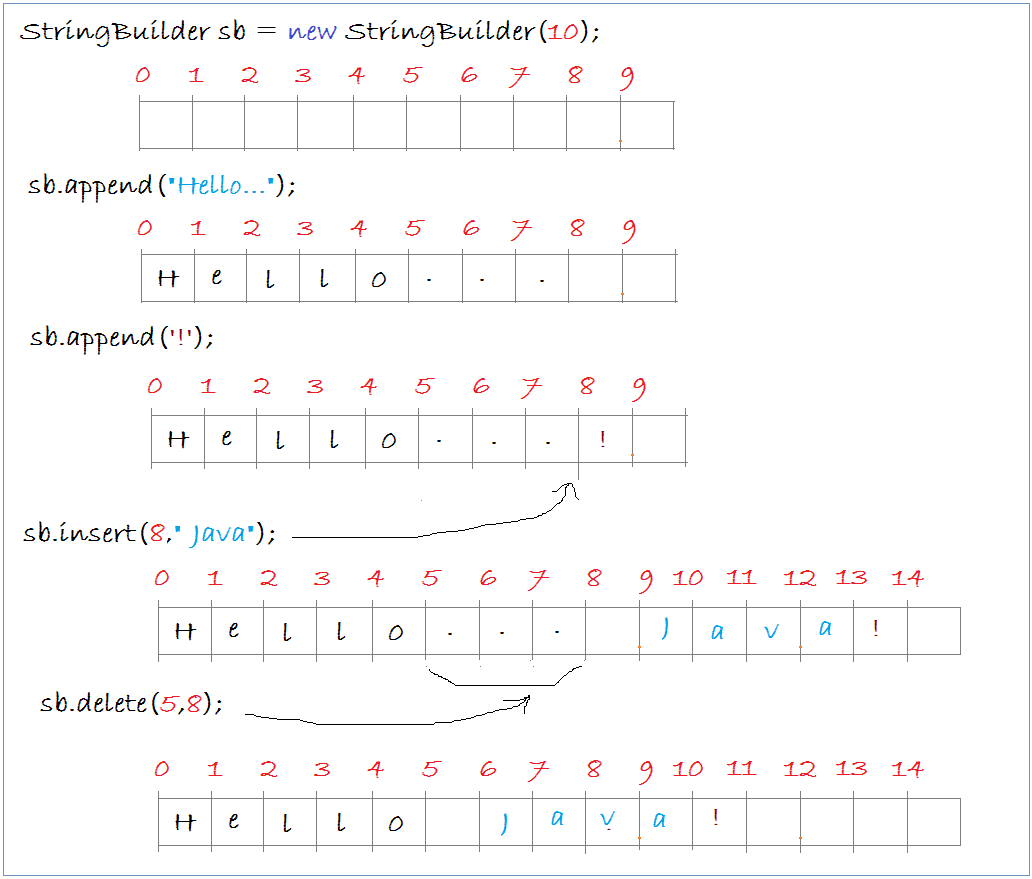
StringBuilderDemo.java
package org.o7planning.tutorial.strbb;
public class StringBuilderDemo {
public static void main(String[] args) {
// Create StringBuilder object
// with no characters in it and
// an initial capacity specified by the capacity argument
StringBuilder sb = new StringBuilder(10);
// Append the string Hello ... on sb.
sb.append("Hello...");
System.out.println("- sb after appends a string: " + sb);
// append a character
char c = '!';
sb.append(c);
System.out.println("- sb after appending a char: " + sb);
// Insert a string at index 5
sb.insert(8, " Java");
System.out.println("- sb after insert string: " + sb);
// Delete substring at index 5 to 8
sb.delete(5,8);
System.out.println("- sb after delete: " + sb);
}
}
Les résultats de l'exécution d'exemple :
- sb after appends a string: Hello...
- sb after appending a char: Hello...!
- sb after insert string: Hello... Java!
- sb after delete: Hello Java!
Java Basic
- Personnaliser le compilateur Java pour traiter votre annotation (Annotation Processing Tool)
- Programmation Java pour l'équipe utilisant Eclipse et SVN
- Le Tutoriel de Java WeakReference
- Le Tutoriel de Java PhantomReference
- Tutoriel sur la compression et la décompression Java
- Configuration d'Eclipse pour utiliser le JDK au lieu de JRE
- Méthodes Java String.format() et printf()
- Syntaxe et nouvelles fonctionnalités de Java 8
- Expression régulière en Java
- Tutoriel de programmation Java multithreading
- Bibliothèques de pilotes JDBC pour différents types de bases de données en Java
- Tutoriel Java JDBC
- Obtenir des valeurs de colonne automatiquement incrémentées lors de l'insertion d'un enregistrement à l'aide de JDBC
- Le Tutoriel de Java Stream
- Le Tutoriel de Java Functional Interface
- Introduction à Raspberry Pi
- Le Tutoriel de Java Predicate
- Classe abstraite et interface en Java
- Modificateurs d'accès en Java
- Le Tutoriel de Java Enum
- Le Tutoriel de Java Annotation
- Comparer et trier en Java
- Le Tutoriel de Java String, StringBuffer et StringBuilder
- Tutoriel de gestion des exceptions Java
- Le Tutoriel de Java Generics
- Manipulation de fichiers et de répertoires en Java
- Le Tutoriel de Java BiPredicate
- Le Tutoriel de Java Consumer
- Le Tutoriel de Java BiConsumer
- Qu'est-ce qui est nécessaire pour commencer avec Java?
- L'histoire de Java et la différence entre Oracle JDK et OpenJDK
- Installer Java sur Windows
- Installer Java sur Ubuntu
- Installer OpenJDK sur Ubuntu
- Installer Eclipse
- Installer Eclipse sur Ubuntu
- Le Tutoriel Java pour débutant
- Histoire des bits et des bytes en informatique
- Types de données dans Java
- Opérations sur les bits
- Le Tutoriel de instruction Java If else
- Le Tutoriel de instruction Java Switch
- Les Boucles en Java
- Les Tableaux (Array) en Java
- JDK Javadoc au format CHM
- Héritage et polymorphisme en Java
- Le Tutoriel de Java Function
- Le Tutoriel de Java BiFunction
- Exemple de Java encoding et decoding utilisant Apache Base64
- Le Tutoriel de Java Reflection
- Invocation de méthode à distance en Java
- Le Tutoriel de Java Socket
- Quelle plate-forme devez-vous choisir pour développer des applications de bureau Java?
- Le Tutoriel de Java Commons IO
- Le Tutoriel de Java Commons Email
- Le Tutoriel de Java Commons Logging
- Comprendre Java System.identityHashCode, Object.hashCode et Object.equals
- Le Tutoriel de Java SoftReference
- Le Tutoriel de Java Supplier
- Programmation orientée aspect Java avec AspectJ (AOP)
Show More
- Tutoriels de programmation Java Servlet/JSP
- Tutoriels de Java Collections Framework
- Tutoriels Java API pour HTML & XML
- Tutoriels Java IO
- Tutoriels Java Date Time
- Tutoriels Spring Boot
- Tutoriels Maven
- Tutoriels Gradle
- Tutoriels Java Web Service
- Tutoriels de programmation Java SWT
- Tutoriels de JavaFX
- Tutoriels Java Oracle ADF
- Tutoriels Struts2
- Tutoriels Spring Cloud