Le Tutoriel de Java BiFunction
1. BiFunction
Dans Java 8, BiFunction est une functional interface qui représente un opérateur lequel accepte deux valeurs d'entrée et en renvoie une.
Le code source de l'interface BiFunction:
BiFunction
@FunctionalInterface
public interface BiFunction<T, U, R> {
R apply(T t, U u);
default <V> BiFunction<T, U, V> andThen(Function<? super R, ? extends V> after) {
Objects.requireNonNull(after);
return (T t, U u) -> after.apply(apply(t, u));
}
}
Voir aussi: Function est une functional interface similaire à BiFunction qui accepte un paramètre d'entrée et renvoie une valeur.
Exemple: Créer une BiFunction qui accepte 2 paramètres d'entrée de type String et renvoie une String.
BiFunctionEx1.java
package org.o7planning.bifunction.ex;
import java.util.function.BiFunction;
public class BiFunctionEx1 {
public static void main(String[] args) {
// Create a BiFunction object directly.
// Accepts 2 input parameters of type String and return a String.
BiFunction<String, String, String> namer //
= (firstName, lastName) -> firstName + " " + lastName;
String fullName = namer.apply("James", "Smith");
System.out.println(fullName);
}
}
Output:
James Smith
Ci-dessous une liste de méthodes dans le package java.util utilisant BiFunction:
V | HashMap.compute(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) |
V | Hashtable.compute(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) |
default V | Map.compute(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) |
V | TreeMap.compute(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) |
V | HashMap.computeIfPresent(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) |
V | Hashtable.computeIfPresent(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) |
default V | Map.computeIfPresent(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) |
V | TreeMap.computeIfPresent(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) |
V | HashMap.merge(K key, V value, BiFunction<? super V,? super V,? extends V> remappingFunction) |
V | Hashtable.merge(K key, V value, BiFunction<? super V,? super V,? extends V> remappingFunction) |
default V | Map.merge(K key, V value, BiFunction<? super V,? super V,? extends V> remappingFunction) |
V | TreeMap.merge(K key, V value, BiFunction<? super V,? super V,? extends V> remappingFunction) |
default void | Map.replaceAll(BiFunction<? super K,? super V,? extends V> function) |
Exemple: Un objet Map contient des mappages entre le nom du pays et le coût de location de l'appartement dans un mois. On met à jour une augmentation de 10% à l'exception du Vietnam.
BiFunctionEx2.java
package org.o7planning.bifunction.ex;
import java.util.HashMap;
import java.util.Map;
import java.util.function.BiFunction;
public class BiFunctionEx2 {
public static void main(String[] args) {
// Data in 2021.
// String country --> Float price to rent an apartment per month ($).
Map<String, Float> pricingMap = new HashMap<String, Float>();
pricingMap.put("Singapore", 2147f);
pricingMap.put("Sweden", 890f);
pricingMap.put("Japan", 770f);
pricingMap.put("Mexico", 345f);
pricingMap.put("Vietnam", 305f);
pricingMap.put("India", 156f);
// Pricing Map
System.out.println(pricingMap);
System.out.println();
// (String,Float) --> Float.
BiFunction<String, Float, Float> biFunction = (country, price) -> {
if (country.equals("Vietnam")) {
return price;
}
return price * 1.1f;
};
pricingMap.replaceAll(biFunction);
// After replacement.
System.out.println("After replacement:\n");
System.out.println(pricingMap);
}
}
Output:
{Sweden=890.0, Vietnam=305.0, Singapore=2147.0, Japan=770.0, Mexico=345.0, India=156.0}
After replacement:
{Sweden=979.0, Vietnam=305.0, Singapore=2361.7, Japan=847.0, Mexico=379.5, India=171.6}
2. andThen(Function after)
La méthode andThen(after) renvoie une fonction BiFunction combinée. Tout d'abord, BiFunction.apply est convoqué pour transformer (T,U) en (R). Ensuite, after.apply est convoqué pour transformer (R) en (V). Si une erreur se produit dans l'une des 2 étapes ci-dessus, l'erreur est transférée à l'appelant. Si une erreur se produit au niveau de la fonction BiFunction actuelle, alors after est ignoré.
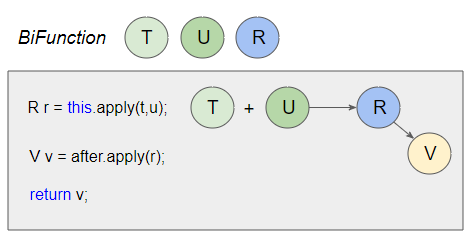
Le code source de la méthode andThen(after) peut être un peu déroutant:
@FunctionalInterface
public interface BiFunction<T, U, R> {
R apply(T t, U u);
default <V> BiFunction<T, U, V> andThen(Function<? super R, ? extends V> after) {
Objects.requireNonNull(after);
return (T t, U u) -> after.apply(apply(t, u));
}
}
Et on peut interpréter le code source ci-dessus de manière plus simple:
BiFunction (Simpler)
@FunctionalInterface
public interface BiFunction<T, U, R> {
// (T,U) -> R
R apply(T t, U u);
// (T,U) -> V
default <V> BiFunction<T, U, V> andThen(Function<? super R, ? extends V> after) {
Objects.requireNonNull(after);
BiFunction<T, U, V> ret = (T t, U u) -> {
// (T,U) -> R
R r = this.apply(t, u);
// R -> V
V v = after.apply(r);
return v;
};
return ret;
}
}
Par exemple:
BiFunction_andThen_ex1.java
package org.o7planning.bifunction.ex;
import java.util.function.Function;
public class BiFunction_andThen_ex1 {
public static void main(String[] args) {
// BiFunction: (char,int) --> (String)
// Example: ('0', 3) --> "000"
BiFunction<Character, Integer, String> bif = (ch, n) -> {
String s="";
for(int i=0;i< n;i++) {
s+=ch;
}
return s;
};
// Function: (String) --> (String)
// Example: "abc" --> "ABC".
Function<String,String> after = s -> s.toUpperCase();
// Test:
String result = bif.andThen(after).apply('a', 3);
System.out.println("Result: " + result);
}
}
Output:
Result: AAA
3. BiFunction + Method reference
Si une méthode prend deux paramètres et renvoie une valeur, sa référence peut être considérée comme une fonction BiFunction.
Exemple: La méthode statique Math.max(int,int) accepte 2 paramètres et renvoie un Integer. Donc sa référence est Math::max peut être considérée comme une BiFunction.
BiFunction_mRef_ex1.java
package org.o7planning.bifunction.ex;
public class BiFunction_mRef_ex1 {
public static void main(String[] args) {
// Create a BiFunction from method reference.
// (Integer,Integer) --> (Integer)
BiFunction<Integer, Integer, Integer> biFunc = Math::max;
int max = biFunc.apply(10, 20); // 20
System.out.println("Max: " + max); // 20
}
}
Output:
Max: 20
Exemple: BiFunction + la référence de méthode non statique:
BiFunction_mRef_ex2.java
package org.o7planning.bifunction.ex;
public class BiFunction_mRef_ex2 {
public static void main(String[] args) {
CardTemplate template = new CardTemplate("Designed by Tom");
// Create a BiFunction from method reference.
// (String,String) --> (String)
BiFunction<String, String, String> biFunc = template::getContent;
String cardContent = biFunc.apply("Eli", "Smith");
System.out.println(cardContent);
}
}
class CardTemplate {
private String someInfo;
public CardTemplate(String someInfo) {
this.someInfo = someInfo;
}
public String getContent(String firstName, String lastName) {
return "----- ~~~~~ -----\n" //
+ "First name: " + firstName + "\n" //
+ "Last name: " + lastName + "\n" //
+ this.someInfo;
}
}
Output:
----- ~~~~~ -----
First name: Eli
Last name: Smith
Designed by Tom
4. BiFunction + Constructor reference
Comme on le sait tous, un constructeur est une méthode spéciale qui renvoie un objet. Ainsi, si un constructeur dispose de 2 paramètres, sa référence sera considérée comme une BiFunction.
BiFunction_cRef_ex1.java
package org.o7planning.bifunction.ex;
public class BiFunction_cRef_ex1 {
public static void main(String[] args) {
// Create a BiFunction from constructor reference.
// (String,Integer) --> (Staff)
BiFunction<String, Integer, Staff> biFunc = Staff::new;
Staff tom = biFunc.apply("Tom", 1000);
Staff jerry = biFunc.apply("Jerry", 2000);
tom.showInfo();
jerry.showInfo();
}
public static class Staff {
private String fullName;
private int salary;
public Staff(String fullName,int salary) {
this.fullName= fullName;
this.salary = salary;
}
public String getFullName() {
return fullName;
}
public int getSalary() {
return salary;
}
public void showInfo() {
System.out.println("Full Name: " + this.fullName +", Salary: " + this.salary);
}
}
}
Output:
Full Name: Tom, Salary: 1000
Full Name: Jerry, Salary: 2000
Java Basic
- Qu'est-ce qui est nécessaire pour commencer avec Java?
- L'histoire de Java et la différence entre Oracle JDK et OpenJDK
- Installer Java sur Windows
- Installer Java sur Ubuntu
- Installer OpenJDK sur Ubuntu
- Installer Eclipse
- Installer Eclipse sur Ubuntu
- Configuration d'Eclipse pour utiliser le JDK au lieu de JRE
- Le Tutoriel Java pour débutant
- Histoire des bits et des bytes en informatique
- Types de données dans Java
- Opérations sur les bits
- Le Tutoriel de instruction Java If else
- Le Tutoriel de instruction Java Switch
- Les Boucles en Java
- Les Tableaux (Array) en Java
- JDK Javadoc au format CHM
- Héritage et polymorphisme en Java
- Classe abstraite et interface en Java
- Modificateurs d'accès en Java
- Le Tutoriel de Java Enum
- Le Tutoriel de Java Annotation
- Personnaliser le compilateur Java pour traiter votre annotation (Annotation Processing Tool)
- Comparer et trier en Java
- Le Tutoriel de Java String, StringBuffer et StringBuilder
- Méthodes Java String.format() et printf()
- Tutoriel de gestion des exceptions Java
- Le Tutoriel de Java Generics
- Comprendre Java System.identityHashCode, Object.hashCode et Object.equals
- Le Tutoriel de Java WeakReference
- Le Tutoriel de Java SoftReference
- Le Tutoriel de Java PhantomReference
- Manipulation de fichiers et de répertoires en Java
- Syntaxe et nouvelles fonctionnalités de Java 8
- Expression régulière en Java
- Tutoriel de programmation Java multithreading
- Bibliothèques de pilotes JDBC pour différents types de bases de données en Java
- Tutoriel Java JDBC
- Obtenir des valeurs de colonne automatiquement incrémentées lors de l'insertion d'un enregistrement à l'aide de JDBC
- Tutoriel sur la compression et la décompression Java
- Le Tutoriel de Java Reflection
- Invocation de méthode à distance en Java
- Le Tutoriel de Java Socket
- Le Tutoriel de Java Stream
- Le Tutoriel de Java Functional Interface
- Le Tutoriel de Java Predicate
- Le Tutoriel de Java BiPredicate
- Le Tutoriel de Java Consumer
- Le Tutoriel de Java BiConsumer
- Le Tutoriel de Java Supplier
- Le Tutoriel de Java Function
- Le Tutoriel de Java BiFunction
- Programmation orientée aspect Java avec AspectJ (AOP)
- Programmation Java pour l'équipe utilisant Eclipse et SVN
- Quelle plate-forme devez-vous choisir pour développer des applications de bureau Java?
- Exemple de Java encoding et decoding utilisant Apache Base64
- Le Tutoriel de Java Commons IO
- Le Tutoriel de Java Commons Email
- Le Tutoriel de Java Commons Logging
- Introduction à Raspberry Pi
Show More
- Tutoriels de programmation Java Servlet/JSP
- Tutoriels de Java Collections Framework
- Tutoriels Java API pour HTML & XML
- Tutoriels Java IO
- Tutoriels Java Date Time
- Tutoriels Spring Boot
- Tutoriels Maven
- Tutoriels Gradle
- Tutoriels Java Web Service
- Tutoriels de programmation Java SWT
- Tutoriels de JavaFX
- Tutoriels Java Oracle ADF
- Tutoriels Struts2
- Tutoriels Spring Cloud