Méthodes Java String.format() et printf()
1. printf()
Dans la programmation Java, vous rencontrez souvent le code System.out.printf(..). En réalité, la méthode printf(..) est définie dans les classes PrintStream et PrintWriter, leur mode d'utilisation est similaire, printf signifie "Print + Format".
printf methods
// Definition of the printf methods in the PrintWriter class:
public PrintStream printf(Locale l, String format, Object... args)
public PrintStream printf(String format, Object... args)
// Definition of the printf methods in PrintWriter class:
public PrintWriter printf(Locale l, String format, Object... args)
public PrintWriter format(String format, Object... args)
System.out.printf(..) est un cas particulier, utilisé pour écrire un texte formaté sur l'écran Console, où System.out est essentiellement un objet de type PrintStream.
System class
package java.lang;
public final class System {
public static final PrintStream out = ...;
}
Avant de commencer, observer l'exemple suivant:
Printf_ex1.java
package org.o7planning.printf.ex;
public class Printf_ex1 {
public static void main(String[] args) {
String firstName = "James";
String lastName = "Smith";
int age = 20;
System.out.printf("My name is %s %s, I'm %d years old.", firstName, lastName, age);
}
}
Output:
My name is James Smith, I'm 20 years old.
2. Règles de formatage
%[flags][width][.precision]conversion-character
Les paramètres: flags, width, precision sont facultatifs.
3. conversion-character
%[flags][width][.precision]conversion-character
conversion-character | Description | Type |
d | decimal integer | byte, short, int, long |
f | floating-point number | float, double |
b | Boolean | Object |
B | will uppercase the boolean | Object |
c | Character Capital | String |
C | will uppercase the letter | String |
s | String Capital | String |
S | will uppercase all the letters in the string | String |
h | hashcode - A hashcode is like an address. This is useful for printing a reference | Object |
n | newline - Platform specific newline character - use %n instead of \n for greater compatibility |
conversion-character: n
System.out.printf("One%nTwo%nThree");
Output:
One
Two
Three
conversion-character: s
System.out.printf("My name is %s %s", "James", "Smith");
Output:
My name is James Smith
conversion-character: S
System.out.printf("My name is %S %S", "James", "Smith");
Output:
My name is JAMES SMITH
conversion-character: b
System.out.printf("%b%n", null);
System.out.printf("%b%n", false);
System.out.printf("%b%n", 5.3);
System.out.printf("%b%n", "Any text");
System.out.printf("%b%n", new Object());
Output:
false
false
true
true
true
conversion-character: B
System.out.printf("%B%n", null);
System.out.printf("%B%n", false);
System.out.printf("%B%n", 5.3);
System.out.printf("%B%n", "Any text");
System.out.printf("%B%n", new Object());
Output:
FALSE
FALSE
TRUE
TRUE
TRUE
conversion-character: d
System.out.printf("There are %d teachers and %d students in the class", 2, 25);
Output:
There are 2 teachers and 25 students in the class
conversion-character: f
System.out.printf("Exchange rate today: EUR %f = USD %f", 1.0, 1.2059);
Output:
Exchange rate today: EUR 1.000000 = USD 1.205900
conversion-character: c
char ch = 'a';
System.out.printf("Code of '%c' character is %d", ch, (int)ch);
Output:
Code of 'a' character is 97
conversion-character: C
char ch = 'a';
System.out.printf("'%C' is the upper case of '%c'", ch, ch);
Output:
'A' is the upper case of 'a'
conversion-character: h
Printf_cc_h_ex.java
package org.o7planning.printf.ex;
public class Printf_cc_h_ex {
public static void main(String[] args) {
// h (Hashcode HEX)
Object myObject = new AnyObject("Something");
System.out.println("Hashcode: " + myObject.hashCode());
System.out.println("Identity Hashcode: " + System.identityHashCode(myObject));
System.out.println("Hashcode (HEX): " + Integer.toHexString(myObject.hashCode()));
System.out.println("toString : " + String.valueOf(myObject));
System.out.printf("Printf: %h", myObject);
}
}
class AnyObject {
private String val;
public AnyObject(String val) {
this.val = val;
}
@Override
public int hashCode() {
if (this.val == null || this.val.isEmpty()) {
return 0;
}
return 1000 + (int) this.val.charAt(0);
}
}
Output:
Hashcode: 1083
Identity Hashcode: 1579572132
Hashcode (HEX): 43b
toString : org.o7planning.printf.ex.AnyObject@43b
Printf: 43b
4. width
%[flags][width][.precision]conversion-character
width - spécifie la quantité minimale d'espace (nombre de caractères) à conserver pour imprimer le contenu d'un argument correspondant.
printf(format, arg1, arg2,.., argN)
5. flags
%[flags][width][.precision]conversion-character
flag | Description |
- | left-justify (default is to right-justify ) |
+ | output a plus ( + ) or minus ( - ) sign for a numerical value |
0 | forces numerical values to be zero-padded (default is blank padding ) |
, | comma grouping separator (for numbers > 1000) |
space will display a minus sign if the number is negative or a space if it is positive |
flag: -
String[] fullNames = new String[] {"Tom", "Jerry", "Donald"};
float[] salaries = new float[] {1000, 1500, 1200};
// s
System.out.printf("|%-10s | %-30s | %-15s |%n", "No", "Full Name", "Salary");
for(int i=0; i< fullNames.length; i++) {
System.out.printf("|%-10d | %-30s | %15f |%n", (i+1), fullNames[i], salaries[i]);
}
Output:
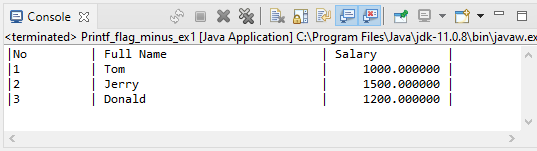
flag: +
// flag: +
System.out.printf("The world's economy increased by %+f percent in 2020. %n", -3.3);
System.out.printf("China's economy increased by %+f percent in 2020. %n", 2.3);
System.out.println();
// without flag: +
System.out.printf("The world's economy increased by %f percent in 2020. %n", -3.3);
System.out.printf("China's economy increased by %f percent in 2020. %n", 2.3);
Output:
The world's economy increased by -3.300000 percent in 2020.
China's economy increased by +2.300000 percent in 2020.
The world's economy increased by -3.300000 percent in 2020.
China's economy increased by 2.300000 percent in 2020.
flag: 0 (zero)
// flag: 0 & with: 20
System.out.printf("|%020f|%n", -3.1);
// without flag & with: 20
System.out.printf("|%20f|%n", -3.1);
// flag: - (left align) & with: 20
System.out.printf("|%-20f
Output:
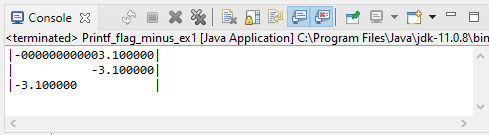
flag: , (comma)
// flag: ,
System.out.printf("%,f %n", 12345678.9);
// flag: ,
System.out.printf("%,f %n", -12345678.9);
Output:
12,345,678.900000
-12,345,678.900000
flag: (space)
// flag: (space)
System.out.printf("% f %n", 12345678.9);
System.out.printf("% f %n", -12345678.9);
System.out.println();
// flags: , (space + comma)
System.out.printf("% ,f %n", 12345678.9);
System.out.printf("% ,
Output:
12345678.900000
-12345678.900000
12,345,678.900000
-12,345,678.900000
6. precision
%[flags][width][.precision]conversion-character
System.out.printf("%f %n", 12345678.911);
System.out.printf("%f %n", -12345678.911);
System.out.println();
// flag: , (comma)
System.out.printf("%,f %n", 12345678.911);
// flag: , (comma)
System.out.printf("%,f %n", -12345678.911);
System.out.println();
// flags: , (space + comma)
System.out.printf("% ,f %n", 12345678.911);
// flags: , (space + comma)
System.out.printf("% ,f %n", -12345678.911);
System.out.println();
// flag: , (comma) & precision: .2
System.out.printf("%,.2f %n", 12345678.911);
// flag: , (comma) & precision: .3
System.out.printf("%,.3f %n", -12345678.911);
System.out.println();
// flags: , (space + comma) & precision: .2
System.out.printf("% ,.2f %n", 12345678.911);
// flags: , (space + comma) & precision: .3
System.out.printf("% ,.3f %n", -12345678.911);
Output:
12345678.911000
-12345678.911000
12,345,678.911000
-12,345,678.911000
12,345,678.911000
-12,345,678.911000
12,345,678.91
-12,345,678.911
12,345,678.91
-12,345,678.911
Java Basic
- Personnaliser le compilateur Java pour traiter votre annotation (Annotation Processing Tool)
- Programmation Java pour l'équipe utilisant Eclipse et SVN
- Le Tutoriel de Java WeakReference
- Le Tutoriel de Java PhantomReference
- Tutoriel sur la compression et la décompression Java
- Configuration d'Eclipse pour utiliser le JDK au lieu de JRE
- Méthodes Java String.format() et printf()
- Syntaxe et nouvelles fonctionnalités de Java 8
- Expression régulière en Java
- Tutoriel de programmation Java multithreading
- Bibliothèques de pilotes JDBC pour différents types de bases de données en Java
- Tutoriel Java JDBC
- Obtenir des valeurs de colonne automatiquement incrémentées lors de l'insertion d'un enregistrement à l'aide de JDBC
- Le Tutoriel de Java Stream
- Le Tutoriel de Java Functional Interface
- Introduction à Raspberry Pi
- Le Tutoriel de Java Predicate
- Classe abstraite et interface en Java
- Modificateurs d'accès en Java
- Le Tutoriel de Java Enum
- Le Tutoriel de Java Annotation
- Comparer et trier en Java
- Le Tutoriel de Java String, StringBuffer et StringBuilder
- Tutoriel de gestion des exceptions Java
- Le Tutoriel de Java Generics
- Manipulation de fichiers et de répertoires en Java
- Le Tutoriel de Java BiPredicate
- Le Tutoriel de Java Consumer
- Le Tutoriel de Java BiConsumer
- Qu'est-ce qui est nécessaire pour commencer avec Java?
- L'histoire de Java et la différence entre Oracle JDK et OpenJDK
- Installer Java sur Windows
- Installer Java sur Ubuntu
- Installer OpenJDK sur Ubuntu
- Installer Eclipse
- Installer Eclipse sur Ubuntu
- Le Tutoriel Java pour débutant
- Histoire des bits et des bytes en informatique
- Types de données dans Java
- Opérations sur les bits
- Le Tutoriel de instruction Java If else
- Le Tutoriel de instruction Java Switch
- Les Boucles en Java
- Les Tableaux (Array) en Java
- JDK Javadoc au format CHM
- Héritage et polymorphisme en Java
- Le Tutoriel de Java Function
- Le Tutoriel de Java BiFunction
- Exemple de Java encoding et decoding utilisant Apache Base64
- Le Tutoriel de Java Reflection
- Invocation de méthode à distance en Java
- Le Tutoriel de Java Socket
- Quelle plate-forme devez-vous choisir pour développer des applications de bureau Java?
- Le Tutoriel de Java Commons IO
- Le Tutoriel de Java Commons Email
- Le Tutoriel de Java Commons Logging
- Comprendre Java System.identityHashCode, Object.hashCode et Object.equals
- Le Tutoriel de Java SoftReference
- Le Tutoriel de Java Supplier
- Programmation orientée aspect Java avec AspectJ (AOP)
Show More
- Tutoriels de programmation Java Servlet/JSP
- Tutoriels de Java Collections Framework
- Tutoriels Java API pour HTML & XML
- Tutoriels Java IO
- Tutoriels Java Date Time
- Tutoriels Spring Boot
- Tutoriels Maven
- Tutoriels Gradle
- Tutoriels Java Web Service
- Tutoriels de programmation Java SWT
- Tutoriels de JavaFX
- Tutoriels Java Oracle ADF
- Tutoriels Struts2
- Tutoriels Spring Cloud