Le Tutoriel de Java Commons Email
1. Introduction

Commons Email est une bibliothèque Java de Apache impliquant Email. En fait, pour travailler avec Java Email vous pouvez utiliser JavaMail API qui a été intégré en JDK6. Commons Email vous permet seulement de travailler avec JavaMail API plus facilement, il ne remplace pas JavaMail API.
Comme Commons IO ne remplace pas JavaIO, il ne comprend que plusieurs classes, méthodes utilitaires pour que vous travailliez avec Java IO plus facilement et que vous épargniez le temps pour votre code.
2. Bibliothèque
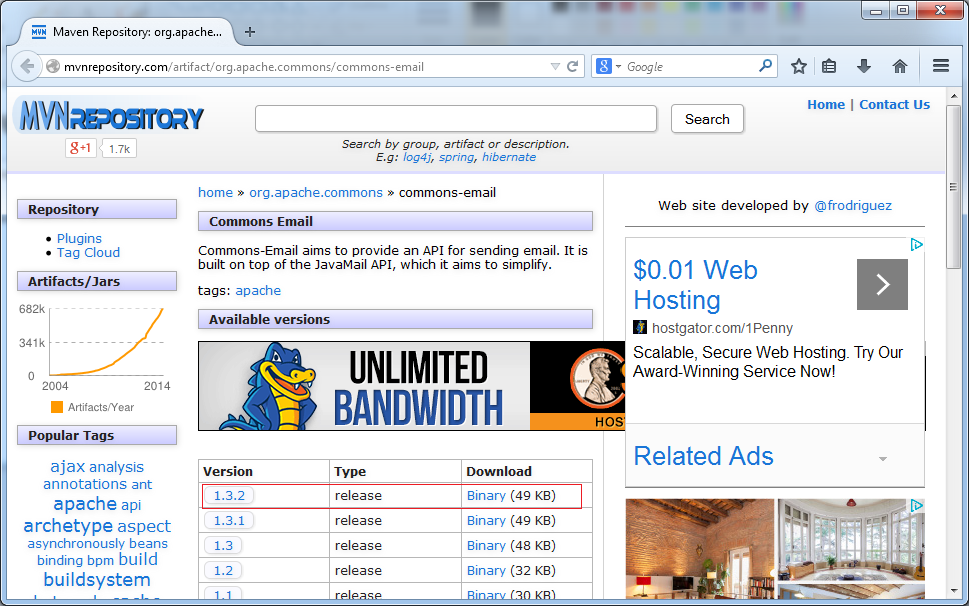
Maven:
** pom.xml **
<!-- http://mvnrepository.com/artifact/org.apache.commons/commons-email -->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-email</artifactId>
<version>1.3.2</version>
</dependency>
See Also:
3. Note pour Gmail
Si vous souhaitez envoyer l'email à partir d'une application Java utilisant Gmail, vous devez autoriser: des applications moins sécurisées:
Sur votre navigateur, connectez-vous à votre compte Gmail et accédez au lien suivant :
Vous recevrez une alerte Google :Certaines applications et périphériques utilisent une technologie de connexion moins sécurisée, ce qui rend votre compte plus vulnérable. Vous pouvez turn off l'accès à ces applications, que nous recommandons, ou activer l'accès (Turn On)si vous souhaitez les utiliser malgré les risques.
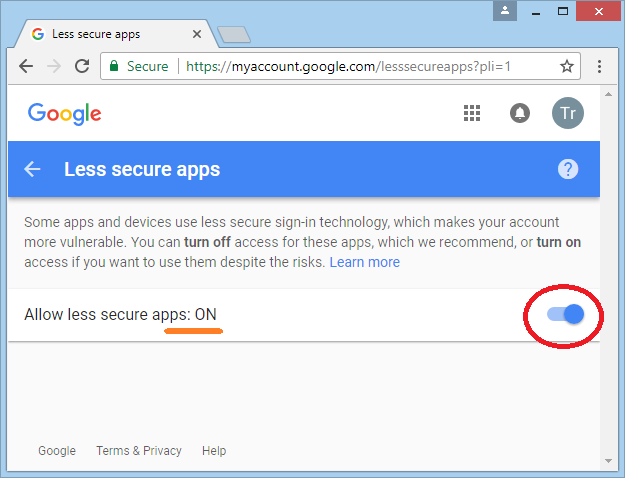
4. Exemple d'envoie un email simple
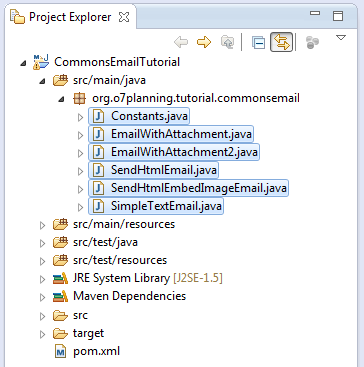
Tout d'abord, un exemple envoie un simple e-mail avec un Commons-Email
Constants.java
package org.o7planning.tutorial.commonsemail;
public class Constants {
public static final String MY_EMAIL = "yourEmail@gmail.com";
public static final String MY_PASSWORD ="your password";
public static final String FRIEND_EMAIL = "friendEmail@gmail.com";
}
SimpleTextEmail.java
package org.o7planning.tutorial.commonsemail;
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.Email;
import org.apache.commons.mail.SimpleEmail;
public class SimpleTextEmail {
public static void main(String[] args) {
try {
Email email = new SimpleEmail();
// Configuration
email.setHostName("smtp.googlemail.com");
email.setSmtpPort(465);
email.setAuthenticator(new DefaultAuthenticator(Constants.MY_EMAIL,
Constants.MY_PASSWORD));
// Required for gmail
email.setSSLOnConnect(true);
// Sender
email.setFrom(Constants.MY_EMAIL);
// Email title
email.setSubject("Test Email");
// Email message.
email.setMsg("This is a test mail ... :-)");
// Receiver
email.addTo(Constants.FRIEND_EMAIL);
email.send();
System.out.println("Sent!!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Exécutez l'exemple :
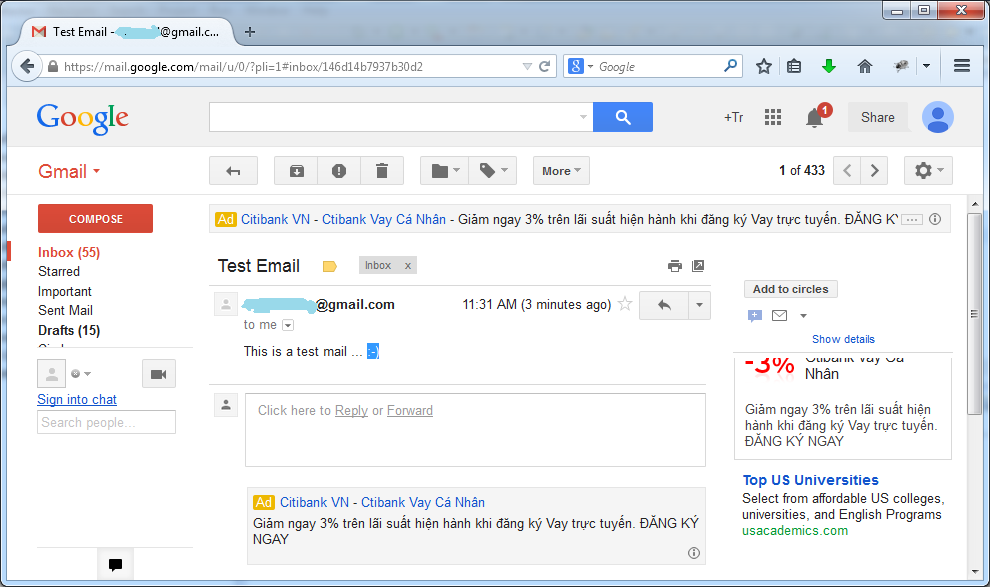
5. Envoyer un E-mail avec des pièces jointes
Par exemple, l'envoie l'email avec un fichier joint sur le disque dur.
EmailWithAttachment.java
package org.o7planning.tutorial.commonsemail;
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.EmailAttachment;
import org.apache.commons.mail.MultiPartEmail;
public class EmailWithAttachment {
public static void main(String[] args) {
try {
// Create the attachment
EmailAttachment attachment = new EmailAttachment();
attachment.setPath("C:/mypictures/map-vietnam.png");
attachment.setDisposition(EmailAttachment.ATTACHMENT);
attachment.setDescription("Vietnam Map");
attachment.setName("Map");
// Create the email message
MultiPartEmail email = new MultiPartEmail();
// Configuration
email.setHostName("smtp.googlemail.com");
email.setSmtpPort(465);
email.setSSLOnConnect(true);
email.setAuthenticator(new DefaultAuthenticator(Constants.MY_EMAIL,
Constants.MY_PASSWORD));
email.setFrom(Constants.MY_EMAIL, "TRAN");
email.addTo(Constants.FRIEND_EMAIL, "Hong");
email.setSubject("The Map");
email.setMsg("Here is the map you wanted");
// Add the attachment
email.attach(attachment);
// Send the email
email.send();
System.out.println("Sent!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Des résultats :
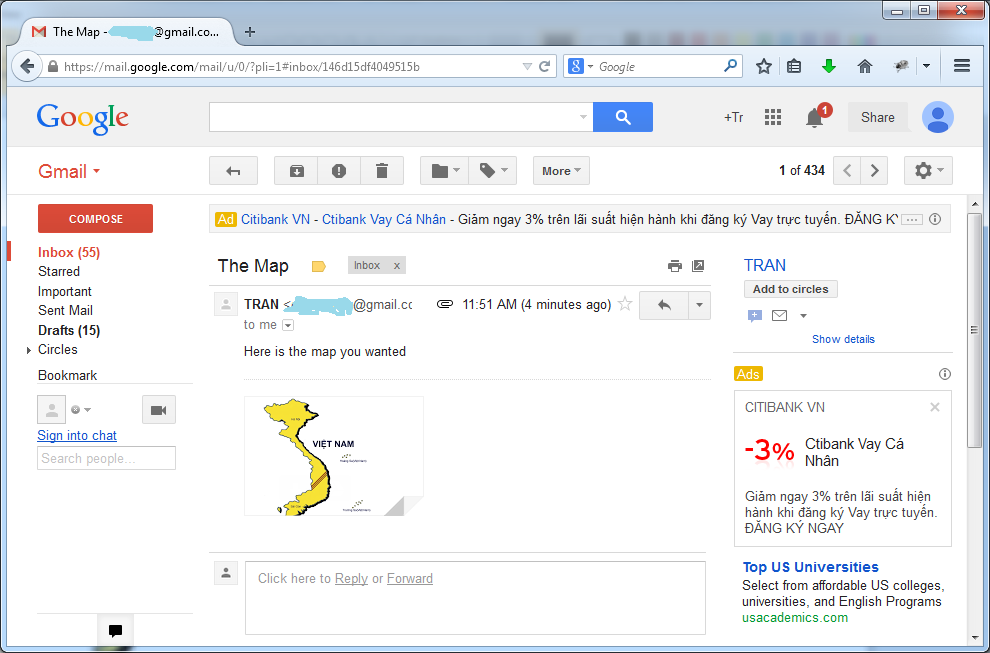
Un autre exemple d'envoyer un email avec pièces jointes à partir d'un lien (lien)
EmailWithAttachment2.java
package org.o7planning.tutorial.commonsemail;
import java.net.URL;
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.EmailAttachment;
import org.apache.commons.mail.MultiPartEmail;
public class EmailWithAttachment2 {
public static void main(String[] args) {
try {
// Create the attachment
EmailAttachment attachment = new EmailAttachment();
attachment.setURL(new URL(
"http://www.apache.org/images/asf_logo_wide.gif"));
attachment.setDisposition(EmailAttachment.ATTACHMENT);
attachment.setDescription("Apache logo");
attachment.setName("Apache logo");
// Create the email message
MultiPartEmail email = new MultiPartEmail();
// Configuration
email.setHostName("smtp.googlemail.com");
email.setSmtpPort(465);
email.setSSLOnConnect(true);
email.setAuthenticator(new DefaultAuthenticator(
Constants.MY_EMAIL, Constants.MY_PASSWORD));
email.setFrom(Constants.MY_EMAIL, "TRAN");
email.addTo(Constants.FRIEND_EMAIL, "Hong");
email.setSubject("The logo");
email.setMsg("Here is Apache's logo");
// Add the attachment
email.attach(attachment);
// Send the email
email.send();
System.out.println("Sent!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Exécutez l'exemple :
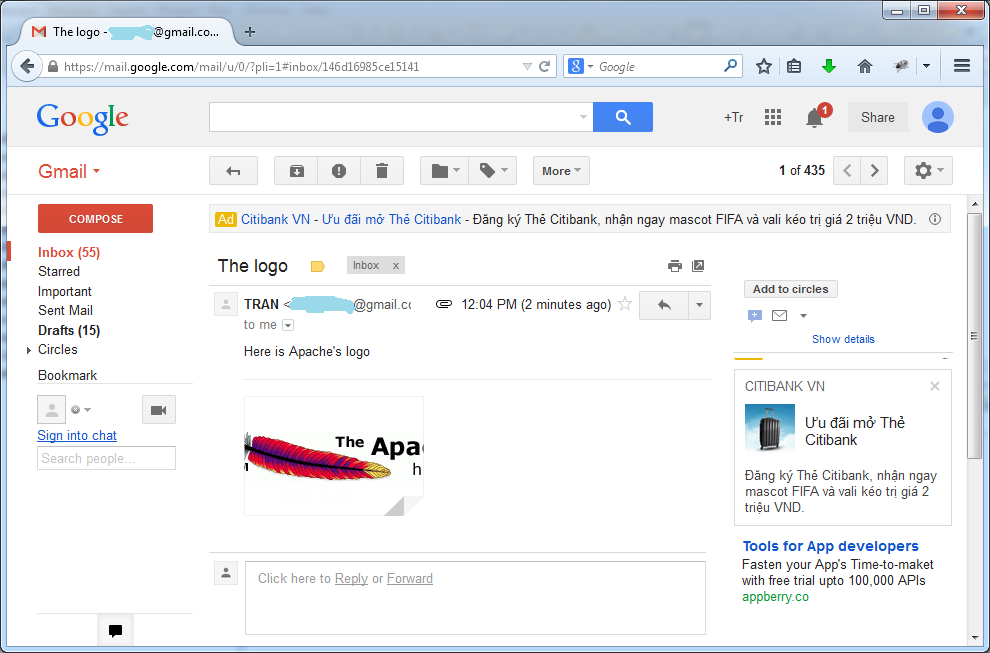
6. Envoyer un email au format HTML
SendHtmlEmail.java
package org.o7planning.tutorial.commonsemail;
import java.net.URL;
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.HtmlEmail;
public class SendHtmlEmail {
public static void main(String[] args) {
try {
// Create the email message
HtmlEmail email = new HtmlEmail();
// Configuration
email.setHostName("smtp.googlemail.com");
email.setSmtpPort(465);
email.setAuthenticator(new DefaultAuthenticator(
Constants.MY_EMAIL,Constants.MY_PASSWORD));
email.setSSLOnConnect(true);
email.setFrom(Constants.MY_EMAIL, "TRAN");
// Receiver
email.addTo(Constants.FRIEND_EMAIL);
// Title
email.setSubject("Test Sending HTML formatted email");
// Embed the image and get the content id
URL url = new URL("http://www.apache.org/images/asf_logo_wide.gif");
String cid = email.embed(url, "Apache logo");
// Set the html message
email.setHtmlMsg("<html><h2>The apache logo</h2> <img src=\"cid:"
+ cid + "\"></html>");
// Set the alternative message
email.setTextMsg("Your email client does not support HTML messages");
// Send email
email.send();
System.out.println("Sent!!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
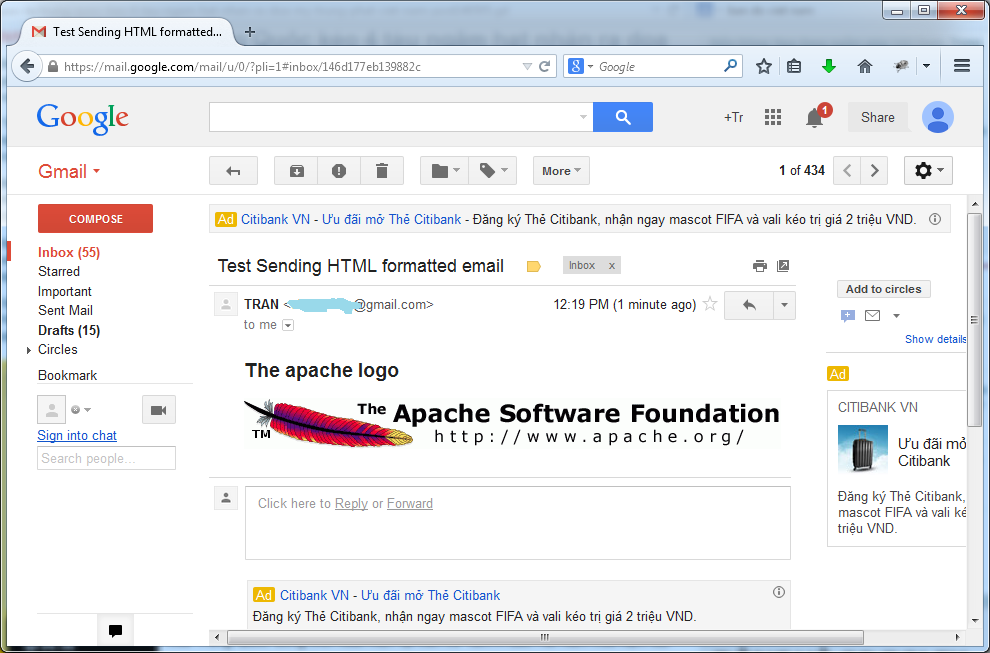
7. Envoyer un email au format HTML, intégré des photos
SendHtmlEmbedImageEmail.java
package org.o7planning.tutorial.commonsemail;
import java.net.URL;
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.ImageHtmlEmail;
import org.apache.commons.mail.resolver.DataSourceUrlResolver;
public class SendHtmlEmbedImageEmail {
public static void main(String[] args) {
try {
// Load your HTML email template
// Here, Img has relative location (**)
String htmlEmailTemplate = "<h2>Hello!</h2>"
+"This is Apache Logo <br/>"
+"<img src='proper/commons-email/images/commons-logo.png'/>";
// Create the email message
ImageHtmlEmail email = new ImageHtmlEmail();
// Configuration
email.setHostName("smtp.googlemail.com");
email.setSmtpPort(465);
email.setAuthenticator(new DefaultAuthenticator(
Constants.MY_EMAIL, Constants.MY_PASSWORD));
email.setSSLOnConnect(true);
email.setFrom(Constants.MY_EMAIL, "TRAN");
email.addTo(Constants.FRIEND_EMAIL);
email.setSubject("Sending HTML formatted email with embedded images");
// Define you base URL to resolve relative resource locations
// (Example - Img you see above ** )
URL url = new URL("http://commons.apache.org");
email.setDataSourceResolver(new DataSourceUrlResolver(url) );
// Set the html message
email.setHtmlMsg(htmlEmailTemplate);
// Set the alternative message
email.setTextMsg("Your email client does not support HTML messages");
// Send the email
email.send();
System.out.println("Sent!!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
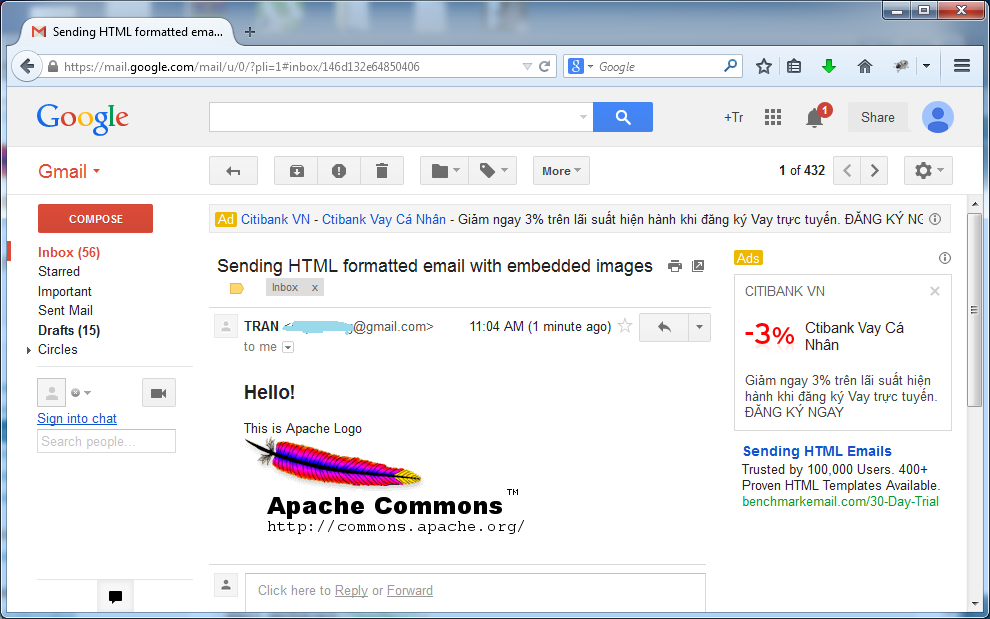
8. Annexe : Télécharger la bibliothèque Commons Email
Si vous utilisez Commons Email dans un projet courant, vous devrez télécharger les bibliothèques nécessaires. Parce que Commons Email est basé sur l'API Email, vous devez télécharger 2 bibliothèques :
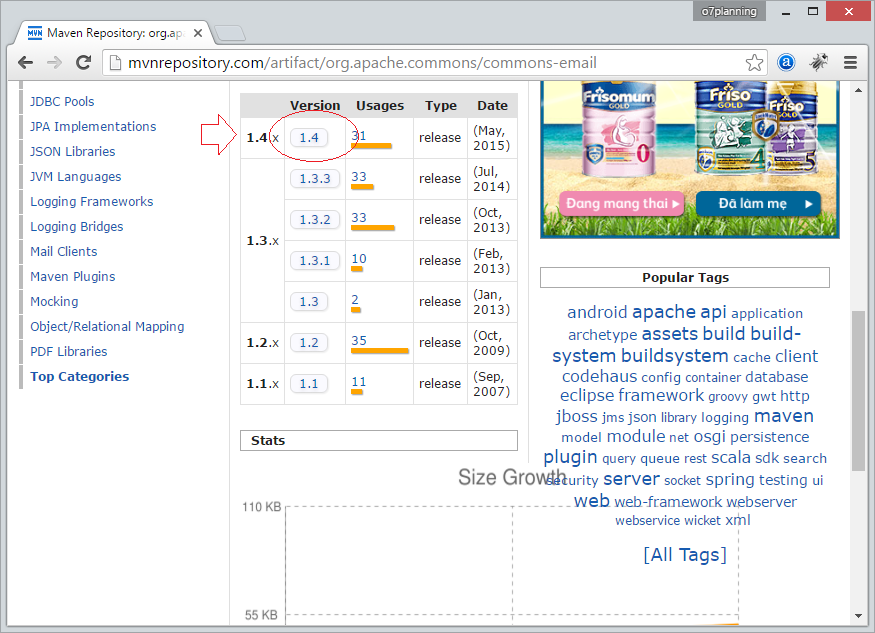
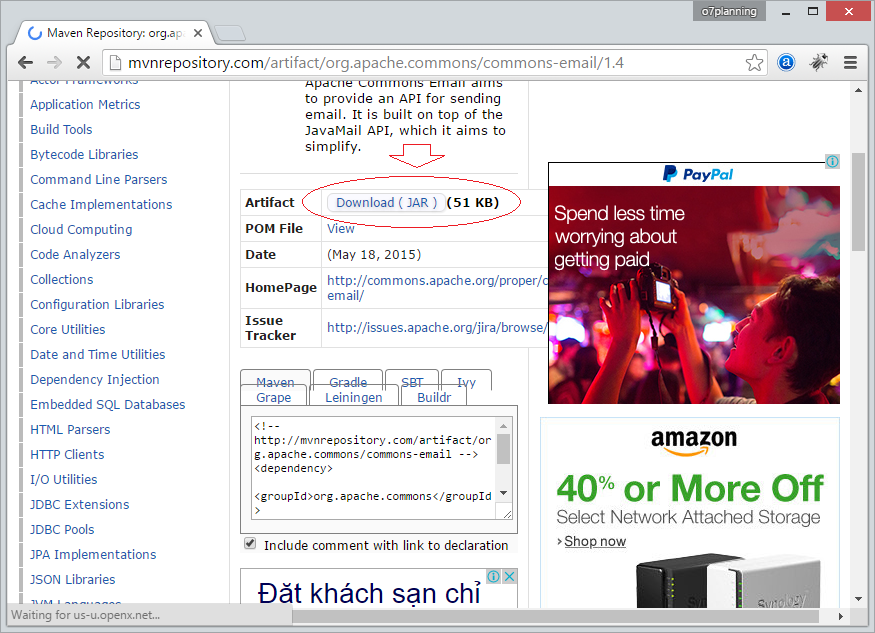
Ensuite téléchargez la bibliothèque Email API :
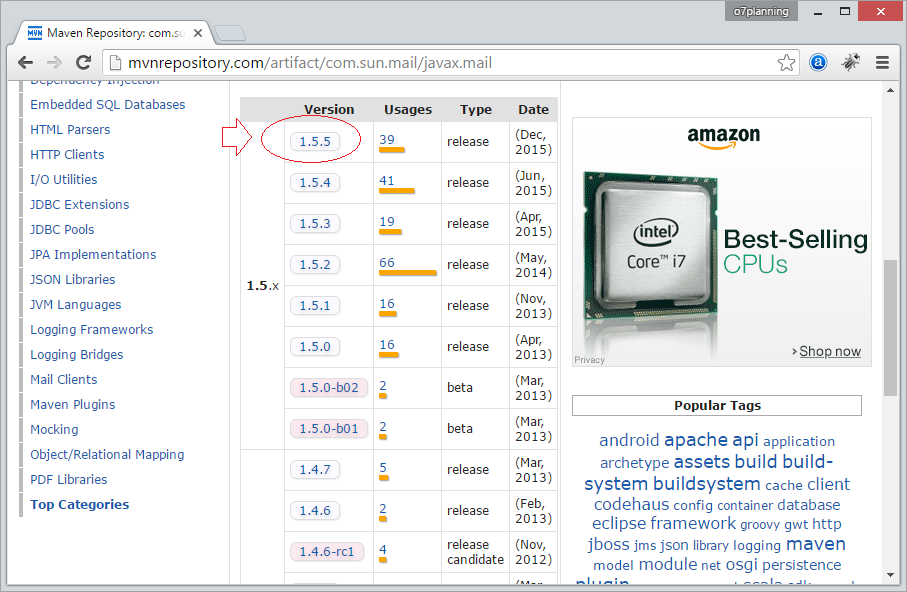
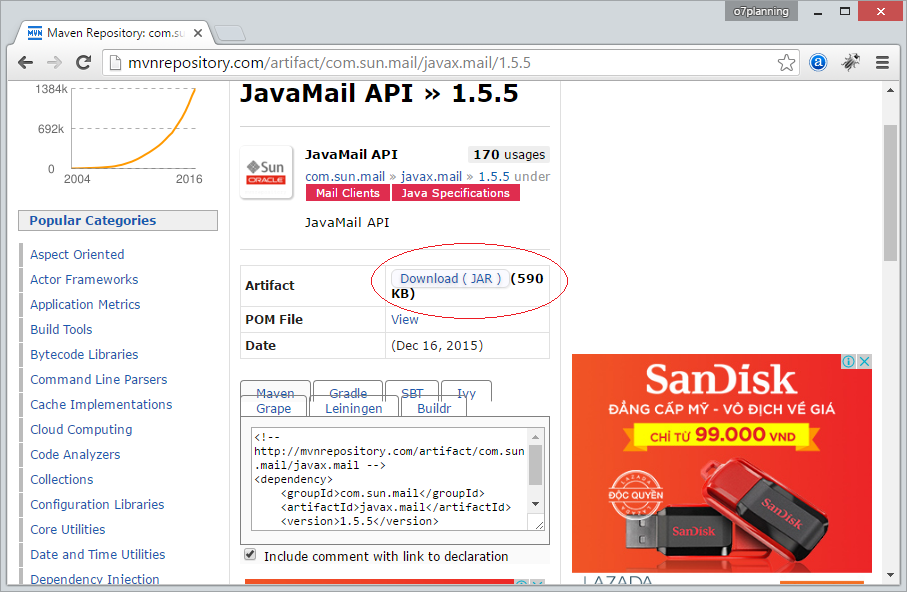
Enfin, vous téléchargez 2 fichiers jar.
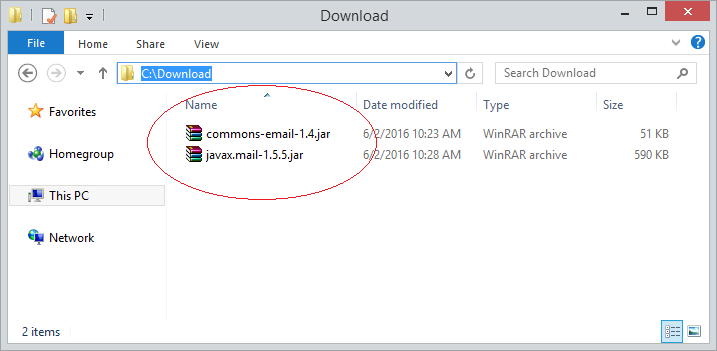
Java Basic
- Personnaliser le compilateur Java pour traiter votre annotation (Annotation Processing Tool)
- Programmation Java pour l'équipe utilisant Eclipse et SVN
- Le Tutoriel de Java WeakReference
- Le Tutoriel de Java PhantomReference
- Tutoriel sur la compression et la décompression Java
- Configuration d'Eclipse pour utiliser le JDK au lieu de JRE
- Méthodes Java String.format() et printf()
- Syntaxe et nouvelles fonctionnalités de Java 8
- Expression régulière en Java
- Tutoriel de programmation Java multithreading
- Bibliothèques de pilotes JDBC pour différents types de bases de données en Java
- Tutoriel Java JDBC
- Obtenir des valeurs de colonne automatiquement incrémentées lors de l'insertion d'un enregistrement à l'aide de JDBC
- Le Tutoriel de Java Stream
- Le Tutoriel de Java Functional Interface
- Introduction à Raspberry Pi
- Le Tutoriel de Java Predicate
- Classe abstraite et interface en Java
- Modificateurs d'accès en Java
- Le Tutoriel de Java Enum
- Le Tutoriel de Java Annotation
- Comparer et trier en Java
- Le Tutoriel de Java String, StringBuffer et StringBuilder
- Tutoriel de gestion des exceptions Java
- Le Tutoriel de Java Generics
- Manipulation de fichiers et de répertoires en Java
- Le Tutoriel de Java BiPredicate
- Le Tutoriel de Java Consumer
- Le Tutoriel de Java BiConsumer
- Qu'est-ce qui est nécessaire pour commencer avec Java?
- L'histoire de Java et la différence entre Oracle JDK et OpenJDK
- Installer Java sur Windows
- Installer Java sur Ubuntu
- Installer OpenJDK sur Ubuntu
- Installer Eclipse
- Installer Eclipse sur Ubuntu
- Le Tutoriel Java pour débutant
- Histoire des bits et des bytes en informatique
- Types de données dans Java
- Opérations sur les bits
- Le Tutoriel de instruction Java If else
- Le Tutoriel de instruction Java Switch
- Les Boucles en Java
- Les Tableaux (Array) en Java
- JDK Javadoc au format CHM
- Héritage et polymorphisme en Java
- Le Tutoriel de Java Function
- Le Tutoriel de Java BiFunction
- Exemple de Java encoding et decoding utilisant Apache Base64
- Le Tutoriel de Java Reflection
- Invocation de méthode à distance en Java
- Le Tutoriel de Java Socket
- Quelle plate-forme devez-vous choisir pour développer des applications de bureau Java?
- Le Tutoriel de Java Commons IO
- Le Tutoriel de Java Commons Email
- Le Tutoriel de Java Commons Logging
- Comprendre Java System.identityHashCode, Object.hashCode et Object.equals
- Le Tutoriel de Java SoftReference
- Le Tutoriel de Java Supplier
- Programmation orientée aspect Java avec AspectJ (AOP)
Show More
- Tutoriels de programmation Java Servlet/JSP
- Tutoriels de Java Collections Framework
- Tutoriels Java API pour HTML & XML
- Tutoriels Java IO
- Tutoriels Java Date Time
- Tutoriels Spring Boot
- Tutoriels Maven
- Tutoriels Gradle
- Tutoriels Java Web Service
- Tutoriels de programmation Java SWT
- Tutoriels de JavaFX
- Tutoriels Java Oracle ADF
- Tutoriels Struts2
- Tutoriels Spring Cloud