Exemple React-Transition-Group CSSTransition (NodeJS)
1. Les objectifs de cette leçon
La bibliothèque react-transition-group vous fournit des composants pour que vous puissiez créer des effets d'animation sur l'application React. La publication ci-dessous introduit en détail de cette bibliothèque, ses composants qu'elle fournit, des API,..
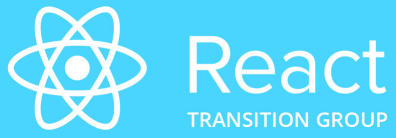
Dans cette leçon, je vais vous guider dans la création d'applications React sur l'environnement NodeJS, à l'aide du composant <CSSTransition> fourni par la bibliothèque react-transition-group.
Notez : Le composant <CSSTransition> est un cas privé qui facilite l'utilisation de la CSS Transition. En général, vous devriez travailler avec le composant <Transition>.
2. Créer un projet et installer une bibliothèque
Créez un projet React avec le nom csstransition-app en exécutant les commandes ci-dessous :
# Install 'create-react-app' tool (If it has never been installed)
npm install -g create-react-app
# Create project with 'create-react-app' tool:
create-react-app csstransition-app
Installer la bibliothèque react-transition-group :
# CD to your project
cd csstransition-app
# Install 'react-transition-group':
npm install react-transition-group --save
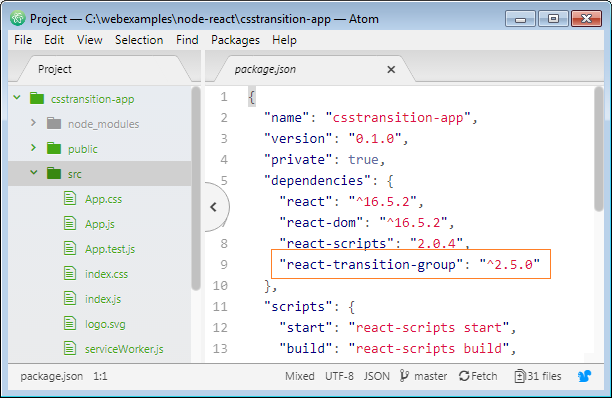
Exécutez votre application :
# Run app:
npm start
3. L'exemple de CssTransition simple
OK, ci-dessous l'avant-première de cet exemple.
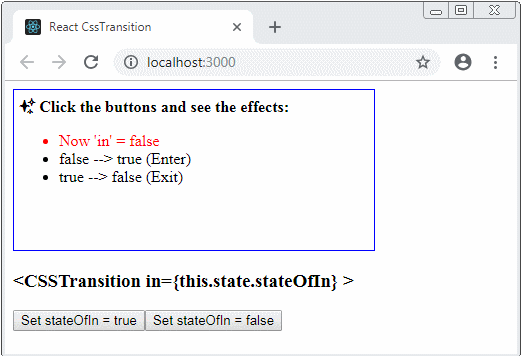
Lorsque la valeur de props: 'in' change de false à true, <CSSTransition> va changer le statut de 'entering', et garde dans ce status dans 'timeout' miliseconds, avan de le changer en statut 'entered'. Dans tel processus, les classes CSS vont appliquer aux éléments enfants de <CSSTransition>, comme l'illustration ci-dessous :
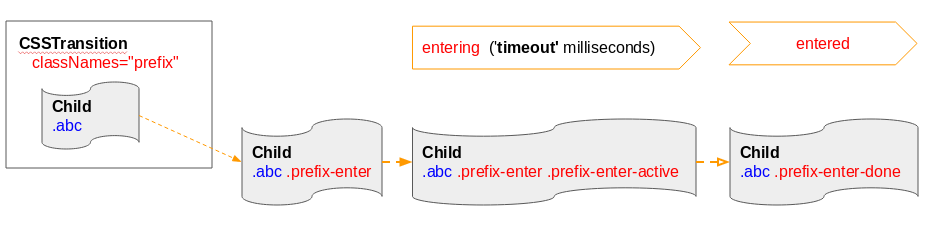
Lorsque la valeur de props: 'in' change de true à false, la <CSSTransition> va changer le statut 'exiting', et garde dans ce statut en 'timeout' milisecondes, avant de changer en statut 'exited'. Dans tel processus, les classes CSS vont appliquer aux éléments enfants de la <CSSTransition>, comme l'illustration ci-dessous :
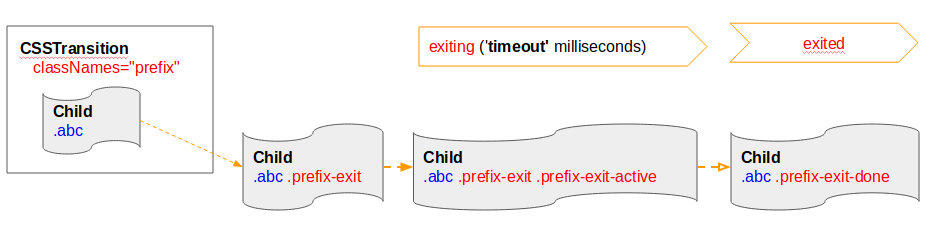
OK, Revenez au projet ci-dessus. Supprimez tout le contenu des fichiers App.js & App.css :
App.css
.example-enter {
opacity: 0.2;
transition: opacity 300ms ease-in;
background: #f9e79f;
}
.example-enter .example-enter-active {
opacity: 1;
background: #eafaf1 ;
}
.example-enter-done {
opacity: 1;
background: #eafaf1 ;
}
.example-exit {
opacity: 1;
transition: opacity 900ms ease-in;
background: #fdebd0 ;
}
.example-exit .example-exit-active {
opacity: 0.5;
background: white ;
}
.example-exit-done {
opacity: 1;
background: white;
}
.my-div {
border: 1px solid blue;
width: 350px;
height: 150px;
padding: 5px;
}
.my-message {
font-style: italic;
color: blue;
}
.my-highlight {
color: red;
}
App.js
import React, { Component } from 'react';
import logo from './logo.svg';
import './App.css';
import { TransitionGroup, CSSTransition } from "react-transition-group";
class App extends React.Component {
render() {
return (
<div>
<MyDiv></MyDiv>
</div>
);
}
}
class MyDiv extends React.Component {
constructor(props) {
super(props);
this.state = {
stateOfIn: false,
message : ""
};
}
// Begin Enter: Do anything!
onEnterHandler() {
this.setState({message: 'Begin Enter...'});
}
onEnteredHandler () {
this.setState({message: 'OK Entered!'});
}
onEnteringHandler() {
this.setState({message: 'Entering... (Wait timeout!)'});
}
// Begin Exit: Do anything!
onExitHandler() {
this.setState({message: 'Begin Exit...'});
}
onExitingHandler() {
this.setState({message: 'Exiting... (Wait timeout!)'});
}
onExitedHandler() {
this.setState({message: 'OK Exited!'});
}
render() {
return (
<div>
<CSSTransition
classNames="example"
in={this.state.stateOfIn}
timeout={1500}
onEnter = {() => this.onEnterHandler()}
onEntering = {() => this.onEnteringHandler()}
onEntered={() => this.onEnteredHandler()}
onExit={() => this.onExitHandler()}
onExiting={() => this.onExitingHandler()}
onExited={() => this.onExitedHandler()}
>
<div className ="my-div">
<b>{"\u2728"} Click the buttons and see the effects:</b>
<ul>
<li className ="my-highlight">Now 'in' = {String(this.state.stateOfIn)}</li>
<li> false --> true (Enter)</li>
<li> true --> false (Exit)</li>
</ul>
<div className="my-message">{this.state.message}</div>
</div>
</CSSTransition>
<h3><CSSTransition in={'{this.state.stateOfIn}'} ></h3>
<button onClick={() => {this.setState({ stateOfIn: true });}}>Set stateOfIn = true</button>
<button onClick={() => {this.setState({ stateOfIn: false });}}>Set stateOfIn = false</button>
</div>
);
}
}
export default App;
Il n'est pas nécessaire de changer les contenus du fichier index.js & index.html :
index.js
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import * as serviceWorker from './serviceWorker';
ReactDOM.render(<App />, document.getElementById('root'));
// If you want your app to work offline and load faster, you can change
// unregister() to register() below. Note this comes with some pitfalls.
// Learn more about service workers: http://bit.ly/CRA-PWA
serviceWorker.unregister();
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<link rel="shortcut icon" href="%PUBLIC_URL%/favicon.ico">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="theme-color" content="#000000">
<link rel="manifest" href="%PUBLIC_URL%/manifest.json">
<title>React CssTransition</title>
</head>
<body>
<noscript>
You need to enable JavaScript to run this app.
</noscript>
<div id="root"></div>
</body>
</html>
Tutoriels de programmation ReactJS
- Le Tutoriel de ReactJS props et state
- Le Tutoriel de ReactJS Event
- Le Tutoriel de ReactJS Component API
- Méthodes dans le cycle de vie ReactJS Component
- Le Tutoriel de ReactJS Refs
- Le Tutoriel de ReactJS Lists et Keys
- Le Tutoriel de ReactJS Form
- Comprendre ReactJS Router avec exemple côté client
- Introduction à Redux
- Exemple simple avec React et Redux côté client
- Le Tutoriel de React-Transition-Group API
- Démarrage rapide avec ReactJS dans l'environnement NodeJS
- Comprendre le ReactJS-Router avec un exemple basique (NodeJS)
- Exemple React-Transition-Group Transition (NodeJS)
- Exemple React-Transition-Group CSSTransition (NodeJS)
- Introduction à ReactJS
- Installation du plugin React pour l'éditeur Atom
- Créer un HTTPServer simple avec NodeJS
- Démarrage rapide avec ReactJS - Hello ReactJS
Show More