Analyser JSON dans TypeScript
1. TypeScript JSON
Comme vous le savez, TypeScript peut être considéré comme une version améliorée de JavaScript. Enfin, TypeScript est également converti en code JavaScript qui peut être exécuté dans le navigateur ou d'autres environnements de JavaScript tels que NodeJS. TypeScript prend en charge toutes les bibliothèques JavaScript et la documentation de l'API.
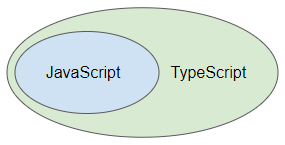
Le moyen le plus simple d'analyser (parse) les données JSON consiste à utiliser les méthodes JSON.parse(..) et JSON.stringify(..). Il n'y a aucune différence dans leur manière d'utilisation dans JavaScript et TypeScript.
JSON
// Static methods:
parse(text: string, reviver?: (this: any, key: string, value: any) => any): any;
stringify(value: any, replacer?: (this: any, key: string, value: any) => any, space?: string | number): string;
stringify(value: any, replacer?: (number | string)[] | null, space?: string | number): string;
Veuillez envisager d'utiliser l'une des bibliothèques JSON ci-dessous si vous développez un grand projet TypeScript.
2. JSON.parse(..)
parse(text: string, reviver?: (this: any, key: string, value: any) => any): any;
Convertir un texte JSON en objet TypeScript.
- text: un texte JSON.
- reviver : une fonction facultative convoquée pour chaque propriété de ce JSON. Le paramètre [key=''] correspond à l'objet JSON global. Les propriétés de niveau feuille seront exécutées en premier, les propriétés de niveau racine seront exécutées en dernier.
Exemple : Utiliser la méthode JSON.parse(..) pour convertir un texte JSON en objet.
json_parse_ex1.ts
function json_parse_ex1_test() {
let jsonString = ` {
"name": "John Smith",
"email": "john@example.com",
"contact": {
"address": "Address 1",
"phone": "12345"
}
}
`;
// Return an object.
let empObj = JSON.parse(jsonString);
console.log(empObj);
console.log(`Address: ${empObj.contact.address}`);
}
json_parse_ex1_test(); // Call the function.
Output:
{
name: 'John Smith',
email: 'john@example.com',
contact: { address: 'Address 1', phone: '12345' }
}
Address: Address 1
Exemple : Utiliser la méthode JSON.parse(..) avec la participation de la fonction reviver, pour convertir un texte JSON en objet d'une classe.
json_parse_ex2.ts
class Employee {
employeeName: string;
employeeEmail: string;
contact: Contact;
constructor(employeeName: string, employeeEmail: string, contact: Contact) {
this.employeeName = employeeName;
this.employeeEmail = employeeEmail;
this.contact = contact;
}
}
class Contact {
address: string;
phone: string;
constructor(address: string, phone: string) {
this.address = address;
this.phone = phone;
}
}
function json_parse_ex2_test() {
let jsonString = ` {
"name": "John Smith",
"email": "john@example.com",
"contact": {
"address": "Address 1",
"phone": "12345"
}
}
`;
// Return an object.
let empObj = JSON.parse(jsonString, function (key: string, value: any) {
if(key == 'name') {
return (value as string).toUpperCase(); // String.
} else if (key == 'contact') {
return new Contact(value.address, value.phone);
} else if (key == '') { // Entire JSON
return new Employee(value.name, value.email, value.contact);
}
return value;
});
console.log(empObj);
console.log(`Employee Name: ${empObj.employeeName}`);
console.log(`Employee Email: ${empObj.employeeEmail}`);
console.log(`Phone: ${empObj.contact.phone}`);
}
json_parse_ex2_test(); // Call the function.
Output:
Employee {
employeeName: 'JOHN SMITH',
employeeEmail: 'john@example.com',
contact: Contact { address: 'Address 1', phone: '12345' }
}
Employee Name: JOHN SMITH
Employee Email: john@example.com
Phone: 12345
3. JSON.stringify(..) *
stringify(value: any, replacer?: (this: any, key: string, value: any) => any, space?: string | number): string;
Convertir une valeur ou un objet en texte JSON.
- value : Une valeur, généralement un objet ou un tableau, à convertir en texte JSON.
- replacer : Une fonction facultative, utilisée pour personnaliser les résultats.
- space: Ajouter des retraits, des espaces ou des sauts de ligne au texte JSON pour en faciliter la lecture.
Exemple : Convertir de valeurs en texte JSON :
json_stringify_ex1a.ts
console.dir(JSON.stringify(1));
console.dir(JSON.stringify(5.9));
console.dir(JSON.stringify(true));
console.dir(JSON.stringify(false));
console.dir(JSON.stringify('falcon'));
console.dir(JSON.stringify("sky"));
console.dir(JSON.stringify(null));
Output:
'1'
'5.9'
'true'
'false'
'"falcon"'
'"sky"'
'null'
Exemple : Convertir un objet en texte JSON :
json_stringify_ex1b.ts
function json_stringify_ex1b_test() {
// An Object:
let emp = {
name: 'John Smith',
email: 'john@example.com',
contact: { address: 'Address 1', phone: '12345' }
};
let jsonString = JSON.stringify(emp);
console.log(jsonString);
}
json_stringify_ex1b_test(); // Call the function.
Output:
{"name":"John Smith","email":"john@example.com","contact":{"address":"Address 1","phone":"12345"}}
Exemple : Utiliser la méthode JSON.stringify(..) avec le paramètre de replacer.
json_stringify_replacer_ex1a.ts
function json_stringify_replacer_ex1a_test() {
// An Object:
let emp = {
name: 'John Smith',
email: 'john@example.com',
contact: { address: 'Address 1', phone: '12345' }
};
let jsonString = JSON.stringify(emp, function (key: string, value: any) {
if (key == 'contact') {
return undefined;
} else if (key == 'name') {
return (value as string).toUpperCase();
}
return value;
});
console.log(jsonString);
}
json_stringify_replacer_ex1a_test(); // Call the function.
Output:
{"name":"JOHN SMITH","email":"john@example.com"}
4. JSON.stringify(..) **
stringify(value: any, replacer?: (number | string)[] | null, space?: string | number): string;
Convertir une valeur ou un objet en texte JSON.
- value: Une valeur, généralement un objet ou un tableau, à convertir en texte JSON.
- replacer: tableau facultatif de chaînes ou de nombres. En tant que liste de propriétés approuvées, elles apparaîtront dans le texte JSON renvoyé.
- space: Ajouter des retraits, des espaces ou des sauts de ligne au texte JSON pour en faciliter la lecture.
json_stringify_replacer_ex2a.ts
function json_stringify_replacer_ex2a_test() {
// An Object:
let emp = {
name: 'John Smith',
email: 'john@example.com',
contact: { address: 'Address 1', phone: '12345' }
};
let replacer = ['email', 'contact', 'address'];
let jsonString = JSON.stringify(emp, replacer, ' ');
console.log(jsonString);
}
json_stringify_replacer_ex2a_test(); // Call the function.
Output:
{
"email": "john@example.com",
"contact": {
"address": "Address 1"
}
}
Tutoriels de programmation TypeScript
- Exécutez votre premier exemple TypeScript en Visual Studio Code
- Espaces (Namespaces) de noms dans TypeScript
- Module dans TypeScript
- Opérateur typeof dans TypeScript
- Les Boucles en TypeScript
- Installer TypeScript sur Windows
- Fonctions dans TypeScript
- Le Tutoriel de TypeScript Tuples
- Interfaces dans TypeScript
- Tableaux dans TypeScript
- Opérateur instanceof dans TypeScript
- Méthodes dans TypeScript
- Fermetures dans TypeScript
- Constructeurs dans TypeScript
- Propriétés dans TypeScript
- Analyser JSON dans TypeScript
- Analyser JSON dans TypeScript avec la bibliothèque json2typescript
- Qu'est-ce que Transpiler?
Show More