Exemple Upload file avec Spring Boot
1. Créer le projet Spring Boot
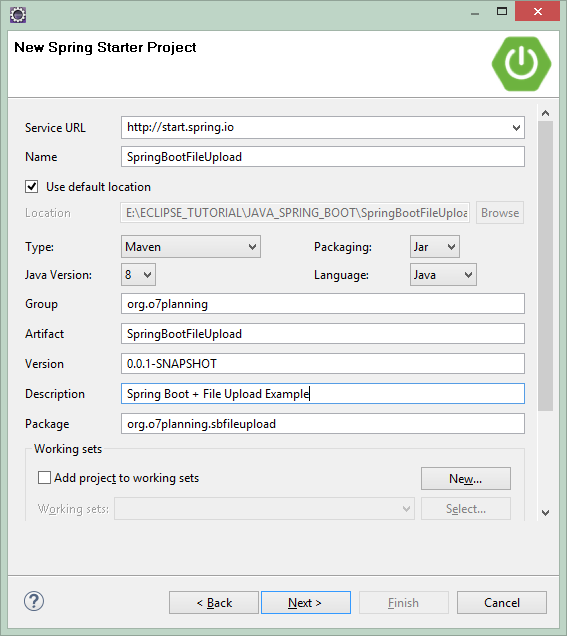
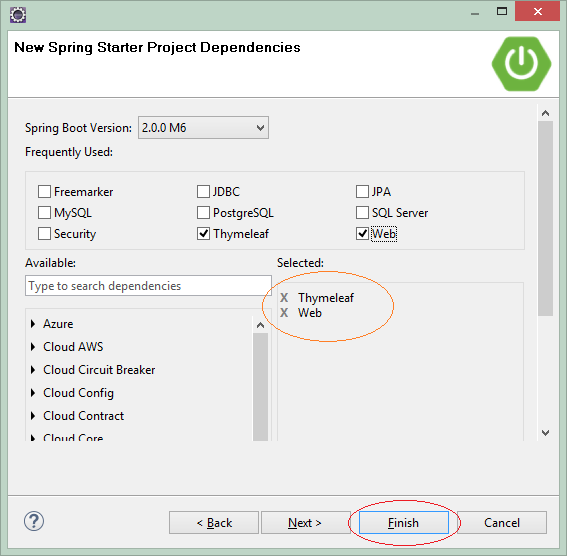
OK. Project has been created.
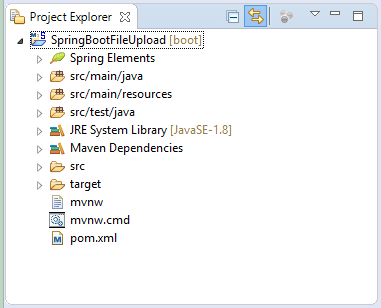
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootFileUpload</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootFileUpload</name>
<description>Spring Boot + File Upload Example</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
SpringBootFileUploadApplication.java
package org.o7planning.sbfileupload;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringBootFileUploadApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootFileUploadApplication.class, args);
}
}
2. Configurer la taille du fichier
Spring Boot a automatiquement configuré les bibliothèques nécessaires pourque vous puissiez construire la fonction de téléchargement de fichier. La taille par défaut du fichier est limitée à 128KB, vous devez donc configurer afin de modifier la valeur de ce paramètre. Ajoutez les propriétés suivantes au fichier application.properties.
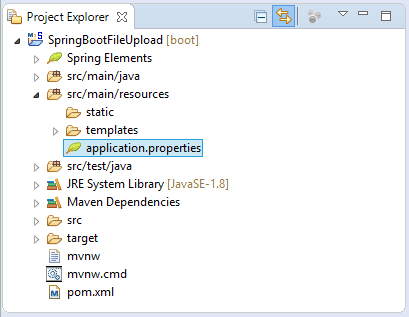
application.properties
spring.servlet.multipart.max-file-size=5MB
spring.servlet.multipart.max-request-size=5MB
La taille maximale du fichier est 5GB.
3. Form & Controller
MyUploadForm.java
package org.o7planning.sbfileupload.form;
import org.springframework.web.multipart.MultipartFile;
public class MyUploadForm {
private String description;
// Upload files.
private MultipartFile[] fileDatas;
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public MultipartFile[] getFileDatas() {
return fileDatas;
}
public void setFileDatas(MultipartFile[] fileDatas) {
this.fileDatas = fileDatas;
}
}
MyFileUploadController.java
package org.o7planning.sbfileupload.controller;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import org.o7planning.sbfileupload.form.MyUploadForm;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.multipart.MultipartFile;
@Controller
public class MyFileUploadController {
@RequestMapping(value = "/")
public String homePage() {
return "index";
}
// GET: Show upload form page.
@RequestMapping(value = "/uploadOneFile", method = RequestMethod.GET)
public String uploadOneFileHandler(Model model) {
MyUploadForm myUploadForm = new MyUploadForm();
model.addAttribute("myUploadForm", myUploadForm);
return "uploadOneFile";
}
// POST: Do Upload
@RequestMapping(value = "/uploadOneFile", method = RequestMethod.POST)
public String uploadOneFileHandlerPOST(HttpServletRequest request, //
Model model, //
@ModelAttribute("myUploadForm") MyUploadForm myUploadForm) {
return this.doUpload(request, model, myUploadForm);
}
// GET: Show upload form page.
@RequestMapping(value = "/uploadMultiFile", method = RequestMethod.GET)
public String uploadMultiFileHandler(Model model) {
MyUploadForm myUploadForm = new MyUploadForm();
model.addAttribute("myUploadForm", myUploadForm);
return "uploadMultiFile";
}
// POST: Do Upload
@RequestMapping(value = "/uploadMultiFile", method = RequestMethod.POST)
public String uploadMultiFileHandlerPOST(HttpServletRequest request, //
Model model, //
@ModelAttribute("myUploadForm") MyUploadForm myUploadForm) {
return this.doUpload(request, model, myUploadForm);
}
private String doUpload(HttpServletRequest request, Model model, //
MyUploadForm myUploadForm) {
String description = myUploadForm.getDescription();
System.out.println("Description: " + description);
// Root Directory.
String uploadRootPath = request.getServletContext().getRealPath("upload");
System.out.println("uploadRootPath=" + uploadRootPath);
File uploadRootDir = new File(uploadRootPath);
// Create directory if it not exists.
if (!uploadRootDir.exists()) {
uploadRootDir.mkdirs();
}
MultipartFile[] fileDatas = myUploadForm.getFileDatas();
//
List<File> uploadedFiles = new ArrayList<File>();
List<String> failedFiles = new ArrayList<String>();
for (MultipartFile fileData : fileDatas) {
// Client File Name
String name = fileData.getOriginalFilename();
System.out.println("Client File Name = " + name);
if (name != null && name.length() > 0) {
try {
// Create the file at server
File serverFile = new File(uploadRootDir.getAbsolutePath() + File.separator + name);
BufferedOutputStream stream = new BufferedOutputStream(new FileOutputStream(serverFile));
stream.write(fileData.getBytes());
stream.close();
//
uploadedFiles.add(serverFile);
System.out.println("Write file: " + serverFile);
} catch (Exception e) {
System.out.println("Error Write file: " + name);
failedFiles.add(name);
}
}
}
model.addAttribute("description", description);
model.addAttribute("uploadedFiles", uploadedFiles);
model.addAttribute("failedFiles", failedFiles);
return "uploadResult";
}
}
4. Thymeleaf Templates
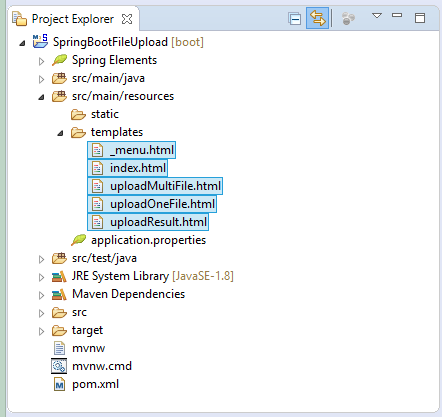
_menu.html
<div xmlns:th="http://www.thymeleaf.org"
style="border:1px solid #ccc;padding:5px;">
<a th:href="@{/uploadOneFile}">Upload One File</a>
|
<a th:href="@{/uploadMultiFile}">Upload Multi File</a>
</div>
index.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Spring Boot File Upload Example</title>
</head>
<body>
<th:block th:include="/_menu"></th:block>
<h3>Spring Boot File Upload Example</h3>
<ul>
<li><a th:href="@{/uploadOneFile}">Upload One File Example</a></li>
<li><a th:href="@{/uploadMultiFile}">Upload Multiple Files Example</a></li>
</ul>
</body>
</html>
uploadOneFile.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Upload One File</title>
</head>
<body>
<th:block th:include="/_menu"></th:block>
<h3>Upload One File:</h3>
<!-- MyUploadForm -->
<form th:object="${myUploadForm}" method="POST"
action="" enctype="multipart/form-data">
Description:
<br>
<input th:field="*{description}" style="width:300px;"/>
<br/><br/>
File to upload: <input th:field="*{fileDatas}" type="file"/>
<br />
<input type="submit" value="Upload">
</form>
</body>
</html>
uploadMultiFile.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Upload Multi File</title>
</head>
<body>
<th:block th:include="/_menu"></th:block>
<h3>Upload Multiple File:</h3>
<!-- MyUploadForm -->
<form th:object="${myUploadForm}" method="POST"
action="" enctype="multipart/form-data">
Description:
<br>
<input th:field="*{description}" style="width:300px;"/>
<br/><br/>
File to upload (1): <input th:field="*{fileDatas}" type="file"/><br />
File to upload (2): <input th:field="*{fileDatas}" type="file"/><br />
File to upload (3): <input th:field="*{fileDatas}" type="file"/><br />
File to upload (4): <input th:field="*{fileDatas}" type="file"/><br />
File to upload (5): <input th:field="*{fileDatas}" type="file"/><br />
<input type="submit" value="Upload">
</form>
</body>
</html>
uploadResult.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Upload Result</title>
</head>
<body>
<th:block th:include="/_menu"></th:block>
Description: <span th:utext="${description}"></span>
<br/>
<h3>Uploaded Files:</h3>
<ul th:each="file : ${uploadedFiles}">
<li th:utext="${file}"></li>
</ul>
<h3>Failed:</h3>
<ul th:each="file : ${failedFiles}">
<li style="color:red;" th:utext="${file}"></li>
</ul>
</body>
</html>
Tutoriels Spring Boot
- Installer Spring Tool Suite pour Eclipse
- Le Tutoriel de Spring pour débutant
- Le Tutoriel de Spring Boot pour débutant
- Propriétés communes de Spring Boot
- Le Tutoriel de Spring Boot et Thymeleaf
- Le Tutoriel de Spring Boot et FreeMarker
- Le Tutoriel de Spring Boot et Groovy
- Le Tutoriel de Spring Boot et Mustache
- Le Tutoriel de Spring Boot et JSP
- Le Tutoriel de Spring Boot, Apache Tiles, JSP
- Utiliser Logging dans Spring Boot
- Surveillance des applications avec Spring Boot Actuator
- Créer une application Web multilingue avec Spring Boot
- Utiliser plusieurs ViewResolvers dans Spring Boot
- Utiliser Twitter Bootstrap dans Spring Boot
- Le Tutoriel de Spring Boot Interceptor
- Le Tutoriel de Spring Boot, Spring JDBC et Spring Transaction
- Le Tutoriel de Spring JDBC
- Le Tutoriel de Spring Boot, JPA et Spring Transaction
- Le Tutoriel de Spring Boot et Spring Data JPA
- Le Tutoriel de Spring Boot, Hibernate et Spring Transaction
- Intégration de Spring Spring, JPA et H2 Database
- Le Tutoriel de Spring Boot et MongoDB
- Utiliser plusieurs DataSources avec Spring Boot et JPA
- Utiliser plusieurs DataSources avec Spring Boot et RoutingDataSource
- Créer une application de connexion avec Spring Boot, Spring Security, Spring JDBC
- Créer une application de connexion avec Spring Boot, Spring Security, JPA
- Créer une application d'enregistrement d'utilisateur avec Spring Boot, Spring Form Validation
- Exemple de OAuth2 Social Login dans Spring Boot
- Exécuter des tâches planifiées en arrière-plan dans Spring
- Exemple CRUD Restful WebService avec Spring Boot
- Exemple Spring Boot Restful Client avec RestTemplate
- Exemple CRUD avec Spring Boot, REST et AngularJS
- Sécurité Spring RESTful Service utilisant Basic Authentication
- Sécuriser Spring Boot RESTful Service en utilisant Auth0 JWT
- Exemple Upload file avec Spring Boot
- Le exemple de Download file avec Spring Boot
- Le exemple de Upload file avec Spring Boot et jQuery Ajax
- Le exemple de Upload file avec Spring Boot et AngularJS
- Créer une application Web Panier avec Spring Boot, Hibernate
- Le Tutoriel de Spring Email
- Créer une application Chat simple avec Spring Boot et Websocket
- Déployer le application Spring Boot sur Tomcat Server
- Déployer le application Spring Boot sur Oracle WebLogic Server
- Installer un certificat SSL gratuit Let's Encrypt pour Spring Boot
- Configurer Spring Boot pour rediriger HTTP vers HTTPS
Show More