Le Tutoriel de Java DataInputStream
1. DataInputStream
DataInputStream est utilisé pour lire les données primitives à partir d'une source de données, en particulier les sources de données écrites par DataOutputStream.
public class DataInputStream extends FilterInputStream implements DataInput
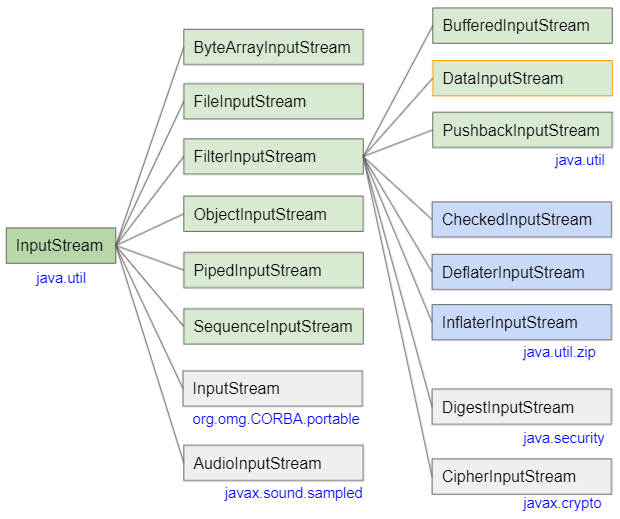
- ByteArrayInputStream
- FileInputStream
- FilterInputStream
- BufferedInputStream
- InputStream
- PushbackInputStream
- ObjectInputStream
- PipedInputStream
- SequenceInputStream
- AudioInputStream
- CheckedInputStream
- DeflaterInputStream
- InflaterInputStream
- CipherInputStream
- DigestInputStream
3. Methods
public final boolean readBoolean() throws IOException
public final byte readByte() throws IOException
public final int readUnsignedByte() throws IOException
public final short readShort() throws IOException
public final int readUnsignedShort() throws IOException
public final char readChar() throws IOException
public final int readInt() throws IOException
public final long readLong() throws IOException
public final float readFloat() throws IOException
public final double readDouble() throws IOException
public final String readUTF() throws IOException
public static final String readUTF(DataInput in) throws IOException
public final void readFully(byte[] b) throws IOException
public final void readFully(byte[] b, int off, int len) throws IOException
@Deprecated
public String readLine() throws IOException
Autres méthodes héritées des classes parentes
public int read() throws IOException
public int read(byte[] b) throws IOException
public int read(byte[] b, int off, int len) throws IOException
public byte[] readAllBytes() throws IOException
public byte[] readNBytes(int len) throws IOException
public int readNBytes(byte[] b, int off, int len) throws IOException
public long skip(long n) throws IOException
public int available() throws IOException
public void close() throws IOException
public synchronized void mark(int readlimit)
public synchronized void reset() throws IOException
public boolean markSupported()
public long transferTo(OutputStream out) throws IOException
public static InputStream nullInputStream()
4. Examples
DataInputStream est souvent utilisé pour lire les sources de données écrites par DataOutputStream. Dans cet exemple, on utilise DataOutputStream pour écrire une table de données avec une structure de type Excel dans un fichier, puis utilisons DataInputStream pour lire ce fichier.
OrderDate | Finished | Item | Units | UnitCost | Total |
2020-01-06 | Pencil | 95 | 1.99 | 189.05 | |
2020-01-23 | Binder | 50 | 19.99 | 999.50 | |
2020-02-09 | Pencil | 36 | 4.99 | 179.64 | |
2020-02-26 | Pen | 27 | 19.99 | 539.73 | |
2020-03-15 | Pencil | 56 | 2.99 | 167.44 |
D'abord, on écrit la classe Order qui simule les données d'une ligne de table :
Order.java
package org.o7planning.datainputstream.ex;
import java.time.LocalDate;
public class Order {
private LocalDate orderDate;
private boolean finished;
private String item;
private int units;
private float unitCost;
private float total;
public Order(LocalDate orderDate, boolean finished, //
String item, int units, float unitCost, float total) {
this.orderDate = orderDate;
this.finished = finished;
this.item = item;
this.units = units;
this.unitCost = unitCost;
this.total = total;
}
public LocalDate getOrderDate() {
return orderDate;
}
public boolean isFinished() {
return finished;
}
public String getItem() {
return item;
}
public int getUnits() {
return units;
}
public float getUnitCost() {
return unitCost;
}
public float getTotal() {
return total;
}
}
Utiliser DataOutputStream pour écrire la table de données ci-dessus dans un fichier :
WriteDataFile_example1.java
package org.o7planning.datainputstream.ex;
import java.io.DataOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.time.LocalDate;
public class WriteDataFile_example1 {
// Windows: C:/somepath/data-file.txt
private static final String filePath = "/Volumes/Data/test/data-file.txt";
public static void main(String[] args) throws IOException {
Order[] orders = new Order[] { //
new Order(LocalDate.of(2020, 1, 6), true, "Pencil", 95, 1.99f, 189.05f),
new Order(LocalDate.of(2020, 1, 23), false, "Binder", 50, 19.99f, 999.50f),
new Order(LocalDate.of(2020, 2, 9), true, "Pencil", 36, 4.99f, 179.64f),
new Order(LocalDate.of(2020, 2, 26), false, "Pen", 27, 19.99f, 539.73f),
new Order(LocalDate.of(2020, 3, 15), true, "Pencil", 56, 2.99f, 167.44f) //
};
File outFile = new File(filePath);
outFile.getParentFile().mkdirs();
OutputStream outputStream = new FileOutputStream(outFile);
DataOutputStream dataOutputStream = new DataOutputStream(outputStream);
for (Order order : orders) {
dataOutputStream.writeUTF(order.getOrderDate().toString());
dataOutputStream.writeBoolean(order.isFinished());
dataOutputStream.writeUTF(order.getItem());
dataOutputStream.writeInt(order.getUnits());
dataOutputStream.writeFloat(order.getUnitCost());
dataOutputStream.writeFloat(order.getTotal());
}
dataOutputStream.close();
}
}
Après avoir exécuté l'exemple ci-dessus, on obtient un fichier de données avec un contenu plutôt déroutant :
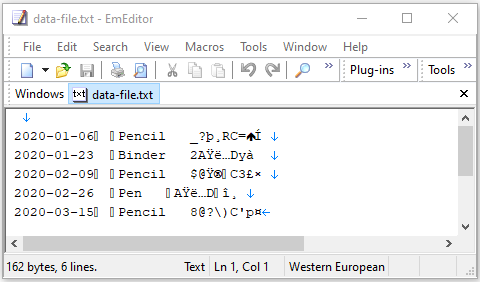
Ensuite, on utilise DataInputStream pour lire le fichier ci-dessus :
ReadDataFile_example1.java
package org.o7planning.datainputstream.ex;
import java.io.DataInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class ReadDataFile_example1 {
// Windows: C:/somepath/data-file.txt
private static final String filePath = "/Volumes/Data/test/data-file.txt";
public static void main(String[] args) throws IOException {
File file = new File(filePath);
InputStream inputStream = new FileInputStream(file);
DataInputStream dataInputStream = new DataInputStream(inputStream);
int row = 0;
System.out.printf("|%3s | %-10s | %10s | %-15s | %8s| %10s | %10s |%n", //
"No", "Order Date", "Finished?", "Item", "Units", "Unit Cost", "Total");
System.out.printf("|%3s | %-10s | %10s | %-15s | %8s| %10s | %10s |%n", //
"--", "---------", "----------", "----------", "------", "---------", "---------");
while (dataInputStream.available() > 0) {
row++;
String orderDate = dataInputStream.readUTF();
boolean finished = dataInputStream.readBoolean();
String item = dataInputStream.readUTF();
int units = dataInputStream.readInt();
float unitCost = dataInputStream.readFloat();
float total = dataInputStream.readFloat();
System.out.printf("|%3d | %-10s | %10b | %-15s | %8d| %,10.2f | %,10.2f |%n", //
row, orderDate, finished, item, units, unitCost, total);
}
dataInputStream.close();
}
}
Output:
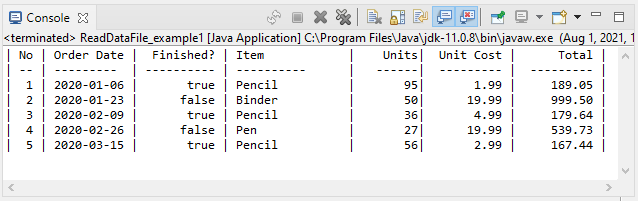
5. readUTF()
La méthode readUTF() est utilisée pour lire une chaîne encodée avec "Modified UTF-8". Les règles de lecture sont symétriques aux règles d'écriture de la méthode DataOutput.writeUTF(String).
public final String readUTF() throws IOException
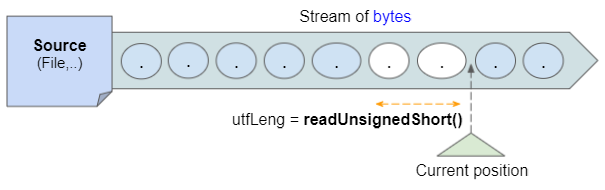
À la position du curseur (cursor) sur le flux de bytes (stream of bytes), le DataInputStream lira les 2 bytes suivants à l'aide de la méthode readUnsignedShort() pour déterminer le nombre de bytes utilisés pour stocker la chaîne UTF-8 et obtenir le résultat est utfLeng.
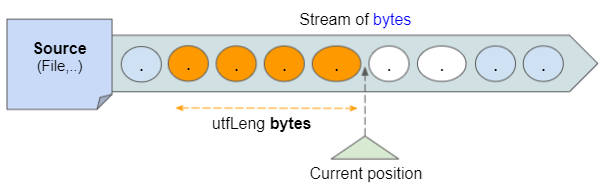
Ensuite, le DataInputStream lit les utfLeng bytes suivants et les convertit en une chaîne UTF-8.
- UTF-8
- DataOutputStream
6. readUTF(DataInput in)
La méthode statique readUTF(DataInput) est utilisée pour lire une chaîne "Modified UTF-8" à partir d'un objet DataInput spécifié.
public static final String readUTF(DataInput in) throws IOException
7. readFully(byte[] buffer)
Lire autant de bytes que possible à partir de ce DataInputStream et l'affecte au tableau de buffer spécifié.
public final void readFully(byte[] buffer) throws IOException
Cette méthode se bloque jusqu'à ce que l'une des conditions suivantes se produise :
- Lire buffer.lengthbytes.
- La fin du flux est atteinte.
- Une IOException se produit (Différent d'EOFException).
8. readFully(byte[] buffer, int off, int len)
Lire autant de bytes que possible à partir de ce DataInputStream et l'affecte au tableau de buffer spécifié de l'index off à l'index off+len-1.
public final void readFully(byte[] buffer, int off, int len) throws IOException
Cette méthode se bloque jusqu'à ce que l'une des conditions suivantes se produise :
- Lire buffer.lengthbytes.
- La fin du flux est atteinte.
- Une IOException se produit (Différent d'EOFException).
9. readBoolean()
La méthode readBoolean() lit un byte à partir de ce DataInputStream. Si le résultat n'est pas nul, la méthode renvoie true, sinon renvoie false.
public final boolean readBoolean() throws IOException
10. readByte()
La méthode readByte() lit un byte de ce DataInputStream, la valeur renvoyée est comprise entre -128 et 127.
Il convient à la lecture de données écrites par la méthode DataOutput.writeByte(byte).
public final byte readByte() throws IOException
11. readUnsignedByte()
La méthode readUnsignedShort() lit 1 byte à partir de ce DataInputStream et le convertit en un nombre entier non signé de 4 bytes, valeurs de 0 à 255.
public final int readUnsignedByte() throws IOException
12. readShort()
La méthode readShort() lit 2 bytes à partir de ce DataInputStream et le convertit en un type de données short.
public final short readShort() throws IOException
La règle de conversion :
return (short)((firstByte << 8) | (secondByte & 0xff));
13. readUnsignedShort()
La méthode readUnsignedShort() lit 2 bytes à partir de ce DataInputStream et le convertit en un nombre entier non signé de 4 bytes, valeurs comprises entre 0 et 65535.
public final int readUnsignedShort() throws IOException
La règle de conversion :
return (((firstByte & 0xff) << 8) | (secondByte & 0xff))
14. readChar()
La méthode readChar() lit 2 bytes à partir de ce DataInputStream et le convertit en type de données char.
public final char readChar() throws IOException
La règle de conversion :
return (char)((firstByte << 8) | (secondByte & 0xff));
15. readInt()
La méthode readInt() lit 4 bytes à partir de ce DataInputStream et le convertit en type de données int.
public final int readInt() throws IOException
La règle de conversion :
return (((firstByte & 0xff) << 24) | ((secondByte & 0xff) << 16)
| ((thirdByte & 0xff) << 8) | (fourthByte & 0xff));
16. readLong()
La méthode readLong() lit 8 bytes à partir de ce DataInputStream et le convertit en un type de données long.
public final long readLong() throws IOException
La règle de conversion :
return (((long)(byte1 & 0xff) << 56) |
((long)(byte2 & 0xff) << 48) |
((long)(byte3 & 0xff) << 40) |
((long)(byte4 & 0xff) << 32) |
((long)(byte5 & 0xff) << 24) |
((long)(byte6 & 0xff) << 16) |
((long)(byte7 & 0xff) << 8) |
((long)(byte8 & 0xff)));
Tutoriels Java IO
- Le Tutoriel de Java CharArrayWriter
- Le Tutoriel de Java FilterReader
- Le Tutoriel de Java FilterWriter
- Le Tutoriel de Java PrintStream
- Le Tutoriel de Java BufferedReader
- Le Tutoriel de Java BufferedWriter
- Le Tutoriel de Java StringReader
- Le Tutoriel de Java StringWriter
- Le Tutoriel de Java PipedReader
- Le Tutoriel de Java LineNumberReader
- Le Tutoriel de Java PushbackReader
- Le Tutoriel de Java PrintWriter
- Tutoriel sur les flux binaires Java IO
- Le Tutoriel de Java IO Character Streams
- Le Tutoriel de Java BufferedOutputStream
- Le Tutoriel de Java ByteArrayOutputStream
- Le Tutoriel de Java DataOutputStream
- Le Tutoriel de Java PipedInputStream
- Le Tutoriel de Java OutputStream
- Le Tutoriel de Java ObjectOutputStream
- Le Tutoriel de Java PushbackInputStream
- Le Tutoriel de Java SequenceInputStream
- Le Tutoriel de Java BufferedInputStream
- Le Tutoriel de Java Reader
- Le Tutoriel de Java Writer
- Le Tutoriel de Java FileReader
- Le Tutoriel de Java FileWriter
- Le Tutoriel de Java CharArrayReader
- Le Tutoriel de Java ByteArrayInputStream
- Le Tutoriel de Java DataInputStream
- Le Tutoriel de Java ObjectInputStream
- Le Tutoriel de Java InputStreamReader
- Le Tutoriel de Java OutputStreamWriter
- Le Tutoriel de Java InputStream
- Le Tutoriel de Java FileInputStream
Show More