Le Tutoriel de Java SequenceInputStream
1. SequenceInputStream
SequenceInputStream permet de concaténer (concatenate) deux ou plusieurs InputStream. Il lit du premier byte au dernier byte du premier InputStream, puis répète cette action avec le prochain InputStream jusqu'au dernier InputStream.
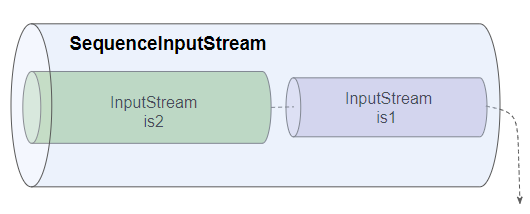
SequenceInputStream est une sous-classe d'InputStream:
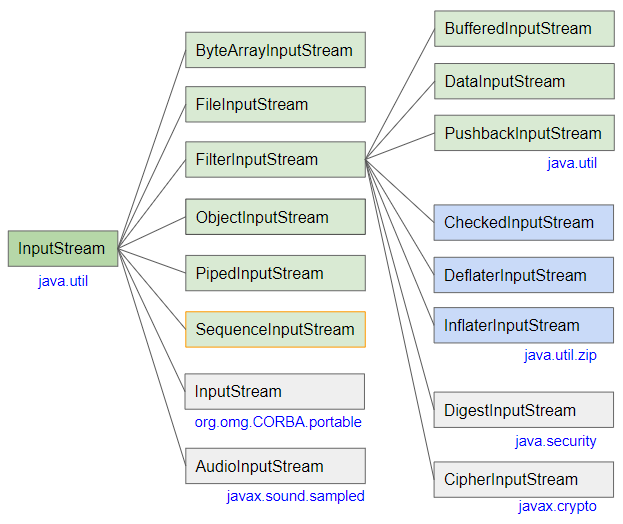
- InputStream
- ObjectInputStream
- ByteArrayInputStream
- FileInputStream
- FilterInputStream
- PipedInputStream
- AudioInputStream
- BufferedInputStream
- DataInputStream
- PushbackInputStream
- CheckedInputStream
- InflaterInputStream
- DigestInputStream
- DeflaterInputStream
- CipherInputStream
SequenceInputStream Methods
final void nextStream() throws IOException
// Methods inherited from InputStream
public int available() throws IOException
public int read() throws IOException
public int read(byte b[], int off, int len) throws IOException
public int read(byte[] b) throws IOException
public byte[] readAllBytes() throws IOException
public byte[] readNBytes(int len) throws IOException
public int readNBytes(byte[] b, int off, int len) throws IOException
public long skip(long n) throws IOException
public boolean markSupported()
public synchronized void mark(int readlimit)
public synchronized void reset() throws IOException
public void close() throws IOException
public long transferTo(OutputStream out) throws IOException
La plupart des méthodes de SequenceInputStream sont héritées d'InputStream, par conséquent, vous pouvez apprendre à utiliser ces méthodes dans l'article sur InputStream:
SequenceInputStream Constructors
public SequenceInputStream(InputStream s1, InputStream s2)
public SequenceInputStream(Enumeration<? extends InputStream> e)
2. Examples
Il existe supposément deux fichiers textes encodés de UTF-8, dont chacun contient une liste de noms de fleurs.
flowers-1.txt
# Flower names (1)
Tulip
Daffodil
Poppy
Sunflower
Bluebell
Rose
Snowdrop
Cherry blossom
flowers-2.txt
# Flower names (2)
Orchid
Iris
Peony
Chrysanthemum
Geranium
Lily
Lotus
Water lily
Dandelion
Hyacinth
Daisy
Crocus
On va lire les deux fichiers susmentionnés en utilisant SequenceInputStream, InputStreamReader et BufferedReader.
SequenceInputStreamEx1.java
package org.o7planning.sequenceinputstream.ex;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.SequenceInputStream;
public class SequenceInputStreamEx1 {
// Windows: C:/Data/test/flowers-1.txt
private static String file_path1 = "/Volumes/Data/test/flowers-1.txt";
private static String file_path2 = "/Volumes/Data/test/flowers-2.txt";
public static void main(String[] args) throws IOException {
InputStream is1 = new FileInputStream(file_path1);
InputStream is2 = new FileInputStream(file_path2);
// Create SequenceInputStream:
InputStream is = new SequenceInputStream(is1, is2);
InputStreamReader isr = new InputStreamReader(is, "UTF-8");
BufferedReader br = new BufferedReader(isr);
String line;
while((line = br.readLine())!= null) {
System.out.println(line);
}
br.close();
}
}
Output:
# Flower names (1)
Tulip
Daffodil
Poppy
Sunflower
Bluebell
Rose
Snowdrop
Cherry blossom
# Flower names (2)
Orchid
Iris
Peony
Chrysanthemum
Geranium
Lily
Lotus
Water lily
Dandelion
Hyacinth
Daisy
Crocus
En améliorant l'exemple ci-dessus, imprimer uniquement la ligne qui n'est pas vide et non une ligne de commentaire (Commencer par "#").
SequenceInputStreamEx2.java
package org.o7planning.sequenceinputstream.ex;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.SequenceInputStream;
public class SequenceInputStreamEx2 {
// Windows: C:/Data/test/flowers-1.txt
private static String file_path1 = "/Volumes/Data/test/flowers-1.txt";
private static String file_path2 = "/Volumes/Data/test/flowers-2.txt";
public static void main(String[] args) throws IOException {
InputStream is1 = new FileInputStream(file_path1);
InputStream is2 = new FileInputStream(file_path2);
// Create SequenceInputStream:
InputStream is = new SequenceInputStream(is1, is2);
InputStreamReader isr = new InputStreamReader(is, "UTF-8");
BufferedReader br = new BufferedReader(isr);
br.lines() // Stream
.filter(line -> !line.isBlank()) // Not blank
.filter(line -> !line.startsWith("#")) // Not start with "#"
.forEach(System.out::println);
br.close();
}
}
Output:
Tulip
Daffodil
Poppy
Sunflower
Bluebell
Rose
Snowdrop
Cherry blossom
Orchid
Iris
Peony
Chrysanthemum
Geranium
Lily
Lotus
Water lily
Dandelion
Hyacinth
Daisy
Crocus
Tutoriels Java IO
- Le Tutoriel de Java CharArrayWriter
- Le Tutoriel de Java FilterReader
- Le Tutoriel de Java FilterWriter
- Le Tutoriel de Java PrintStream
- Le Tutoriel de Java BufferedReader
- Le Tutoriel de Java BufferedWriter
- Le Tutoriel de Java StringReader
- Le Tutoriel de Java StringWriter
- Le Tutoriel de Java PipedReader
- Le Tutoriel de Java LineNumberReader
- Le Tutoriel de Java PushbackReader
- Le Tutoriel de Java PrintWriter
- Tutoriel sur les flux binaires Java IO
- Le Tutoriel de Java IO Character Streams
- Le Tutoriel de Java BufferedOutputStream
- Le Tutoriel de Java ByteArrayOutputStream
- Le Tutoriel de Java DataOutputStream
- Le Tutoriel de Java PipedInputStream
- Le Tutoriel de Java OutputStream
- Le Tutoriel de Java ObjectOutputStream
- Le Tutoriel de Java PushbackInputStream
- Le Tutoriel de Java SequenceInputStream
- Le Tutoriel de Java BufferedInputStream
- Le Tutoriel de Java Reader
- Le Tutoriel de Java Writer
- Le Tutoriel de Java FileReader
- Le Tutoriel de Java FileWriter
- Le Tutoriel de Java CharArrayReader
- Le Tutoriel de Java ByteArrayInputStream
- Le Tutoriel de Java DataInputStream
- Le Tutoriel de Java ObjectInputStream
- Le Tutoriel de Java InputStreamReader
- Le Tutoriel de Java OutputStreamWriter
- Le Tutoriel de Java InputStream
- Le Tutoriel de Java FileInputStream
Show More