Le Tutoriel de Java ByteArrayOutputStream
1. ByteArrayOutputStream
ByteArrayOutputStream est une sous-classe d'OutputStream. Comme son nom l'indique, ByteArrayOutputStream est utilisé pour écrire des bytes dans un tableau bytes qui gère à la manière d'un OutputStream.
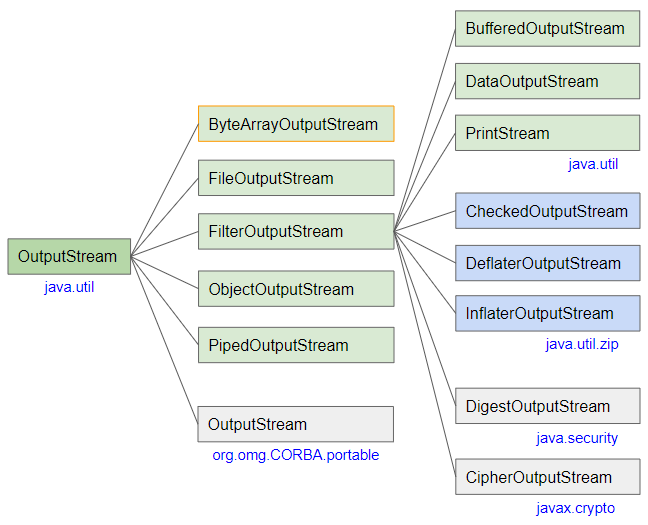
- OutputStream
- BufferedOutputStream
- ObjectOutputStream
- PrintStream
- FileOutputStream
- FilterOutputStream
- PipedOutputStream
- DataOutputStream
- InflaterOutputStream
- DigestOutputStream
- DeflaterOutputStream
- CipherOutputStream
- CheckedOutputStream
Les bytes écrits dans ByteArrayOutputStream seront assignés aux éléments du tableau byte que gère ByteArrayOutputStream.
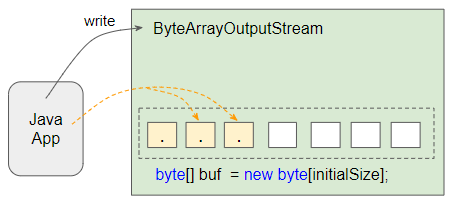
Lorsque le nombre de bytes écrits dans ByteArrayOutputStream est supérieur à la longueur du tableau, ByteArrayOutputStream crée un nouveau tableau de plus grande longueur et copie les bytes de l'ancien tableau.
2. Constructors
Constructors
public ByteArrayOutputStream()
public ByteArrayOutputStream(int initialSize)
- Le constructeur ByteArrayOutputStream(int) crée un objet ByteArrayOutputStream avec le tableau byte dont la taille est initialement spéficiée.
- Le constructeur ByteArrayOutputStream() crée un objet ByteArrayOutputStream avec le tableau byte ayant la taille par défaut (32).
3. Methods
Toutes les méthodes de ByteArrayOutputStream sont héritées de sa classe parent - OutputStream.
void close()
void reset()
int size()
byte[] toByteArray()
String toString()
String toString(String charsetName)
String toString(Charset charset)
void write(byte[] b, int off, int len)
void write(int b)
void writeBytes(byte[] b)
void writeTo(OutputStream out)
Voir l'article OutputStream pour plus d'exemples sur ces méthodes.
4. Examples
ByteArrayOutputStreamEx1.java
package org.o7planning.bytearrayoutputstream.ex;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
public class ByteArrayOutputStreamEx1 {
public static void main(String[] args) throws IOException {
ByteArrayOutputStream baos = new ByteArrayOutputStream(1024);
byte[] bytes1 = new byte[] {'H', 'e'};
baos.write(bytes1);
baos.write(108); // 'l'
baos.write('l'); // code: 108
baos.write('o'); // code: 111
byte[] buffer = baos.toByteArray();
for(byte b: buffer) {
System.out.println(b + " --> " + (char)b);
}
}
}
Output:
72 --> H
101 --> e
108 --> l
108 --> l
111 --> o
Par exemple: additionner 2 tableaux byte pour en créer un nouveau.
ByteArrayOutputStreamEx3.java
package org.o7planning.bytearrayoutputstream.ex;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
public class ByteArrayOutputStreamEx3 {
public static void main(String[] args) throws IOException {
byte[] arr1 = "Hello ".getBytes();
byte[] arr2 = new byte[] {'W', 'o', 'r', 'l', 'd', '!'};
byte[] result = add(arr1, arr2);
for(byte b: result) {
System.out.println(b + " " + (char)b);
}
}
public static byte[] add(byte[] arr1, byte[] arr2) {
if (arr1 == null) {
return arr2;
}
if (arr2 == null) {
return arr1;
}
ByteArrayOutputStream baos = new ByteArrayOutputStream();
try {
baos.write(arr1);
baos.write(arr2);
} catch (Exception e) {
// Never happened!
}
return baos.toByteArray();
}
}
Output:
72 H
101 e
108 l
108 l
111 o
32
87 W
111 o
114 r
108 l
100 d
33 !
En règle générale, la plupart des méthodes de ByteArrayOutputStream sont héritées d'OutputStream. Vous pouver trouver plus d'exemples sur la manière d'utilisation de ces méthodes dans l'article ci-dessous:
Tutoriels Java IO
- Le Tutoriel de Java CharArrayWriter
- Le Tutoriel de Java FilterReader
- Le Tutoriel de Java FilterWriter
- Le Tutoriel de Java PrintStream
- Le Tutoriel de Java BufferedReader
- Le Tutoriel de Java BufferedWriter
- Le Tutoriel de Java StringReader
- Le Tutoriel de Java StringWriter
- Le Tutoriel de Java PipedReader
- Le Tutoriel de Java LineNumberReader
- Le Tutoriel de Java PushbackReader
- Le Tutoriel de Java PrintWriter
- Tutoriel sur les flux binaires Java IO
- Le Tutoriel de Java IO Character Streams
- Le Tutoriel de Java BufferedOutputStream
- Le Tutoriel de Java ByteArrayOutputStream
- Le Tutoriel de Java DataOutputStream
- Le Tutoriel de Java PipedInputStream
- Le Tutoriel de Java OutputStream
- Le Tutoriel de Java ObjectOutputStream
- Le Tutoriel de Java PushbackInputStream
- Le Tutoriel de Java SequenceInputStream
- Le Tutoriel de Java BufferedInputStream
- Le Tutoriel de Java Reader
- Le Tutoriel de Java Writer
- Le Tutoriel de Java FileReader
- Le Tutoriel de Java FileWriter
- Le Tutoriel de Java CharArrayReader
- Le Tutoriel de Java ByteArrayInputStream
- Le Tutoriel de Java DataInputStream
- Le Tutoriel de Java ObjectInputStream
- Le Tutoriel de Java InputStreamReader
- Le Tutoriel de Java OutputStreamWriter
- Le Tutoriel de Java InputStream
- Le Tutoriel de Java FileInputStream
Show More