Le Tutoriel de Java ObjectOutputStream
1. ObjectOutputStream
ObjectOutputStream est une sous-classe de la classe OutputStream, qui gère un objet OutputStream et fournit les méthodes susceptibles d'écrire les données primitives (primitive data) ou les objets sur OutputStream qu'il gère.
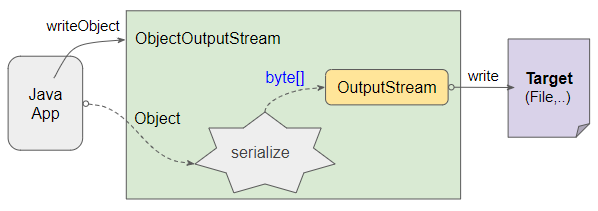
public class ObjectOutputStream
extends OutputStream implements ObjectOutput, ObjectStreamConstants
ObjectInputStream est utilisée pour lire les sources de données créées par ObjectOutputStream:
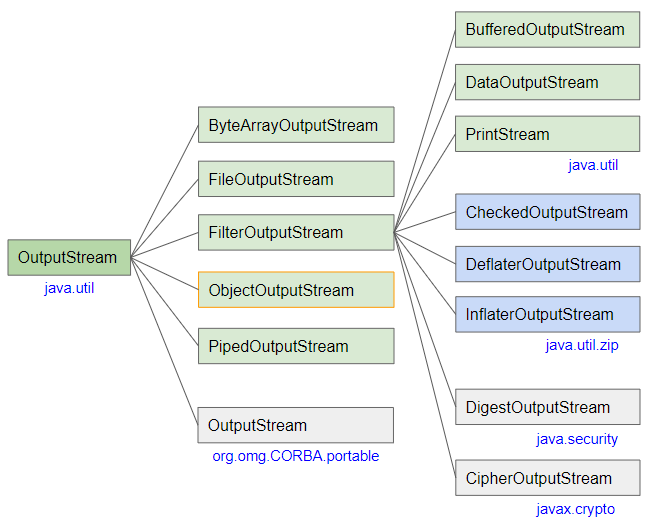
- OutputStream
- FilterOutputStream
- ByteArrayOutputStream
- PrintStream
- PipedOutputStream
- BufferedOutputStream
- DataOutputStream
- FileInputStream
- CheckedOutputStream
- CheckedOutputStream
- CipherOutputStream
- DeflaterOutputStream
- DigestOutputStream
- InflaterOutputStream
ObjectOutputStream Methods
public final void writeObject(Object obj) throws IOException
public void writeBoolean(boolean val) throws IOException
public void writeByte(int val) throws IOException
public void writeShort(int val) throws IOException
public void writeChar(int val) throws IOException
public void writeInt(int val) throws IOException
public void writeLong(long val) throws IOException
public void writeFloat(float val) throws IOException
public void writeDouble(double val) throws IOException
public void writeBytes(String str) throws IOException
public void writeChars(String str) throws IOException
public void writeUTF(String str) throws IOException
public void writeUnshared(Object obj) throws IOException
public void writeFields() throws IOException
public void defaultWriteObject() throws IOException
public void useProtocolVersion(int version) throws IOException
public ObjectOutputStream.PutField putFields() throws IOException
public void reset() throws IOException
protected void writeObjectOverride(Object obj) throws IOException
protected void annotateClass(Class<?> cl) throws IOException
protected void annotateProxyClass(Class<?> cl) throws IOException
protected Object replaceObject(Object obj) throws IOException
protected boolean enableReplaceObject(boolean enable) throws SecurityException
protected void writeStreamHeader() throws IOException
protected void writeClassDescriptor(ObjectStreamClass desc) throws IOException
protected void drain() throws IOException
int getProtocolVersion()
void writeTypeString(String str) throws IOException
// Methods Inherited from OutputStream
public void write(int val) throws IOException
public void write(byte[] buf) throws IOException
public void write(byte[] buf, int off, int len) throws IOException
public void flush() throws IOException
public void close() throws IOException
ObjectOutputStream Constructors
ObjectOutputStream(OutputStream out)
Les objets doivent être sérialisés (serialized) avant d'être écrits sur ObjectOutputStream. Ces objets doivent être implémentés une interface Serializable.
2. Example 1
La classe Employee implémente l'interface Serializable, ce qui est nécessaire pour qu'elle puisse être écrite sur ObjectOutputStream.
Employee.java
package org.o7planning.beans;
import java.io.Serializable;
public class Employee implements Serializable {
private static final long serialVersionUID = 1L;
private String fullName;
private float salary;
public Employee(String fullName, float salary) {
this.fullName = fullName;
this.salary = salary;
}
public String getFullName() {
return fullName;
}
public void setFullName(String firstName) {
this.fullName = firstName;
}
public float getSalary() {
return salary;
}
public void setSalary(float lastName) {
this.salary = lastName;
}
}
Par exemple: Utiliser ObjectOutputStream pour écrire les objets Employee sur un fichier.
WriteEmployeeDataEx.java
package org.o7planning.objectoutputstream.ex;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import java.io.OutputStream;
import java.util.Date;
import org.o7planning.beans.Employee;
public class WriteEmployeeDataEx {
// Windows: C:/Data/test/employees.data
private static String file_path = "/Volumes/Data/test/employees.data";
public static void main(String[] args) throws IOException {
File outFile = new File(file_path);
outFile.getParentFile().mkdirs();
Employee e1 = new Employee("Tom", 1000f);
Employee e2 = new Employee("Jerry", 2000f);
Employee e3 = new Employee("Donald", 1200f);
Employee[] employees = new Employee[] { e1, e2, e3 };
OutputStream os = new FileOutputStream(outFile);
ObjectOutputStream oos = new ObjectOutputStream(os);
System.out.println("Writing file: " + outFile.getAbsolutePath());
oos.writeObject(new Date());
oos.writeUTF("Employee data"); // Some informations.
oos.writeInt(employees.length); // Number of Employees
for (Employee e : employees) {
oos.writeObject(e);
}
oos.close();
System.out.println("Finished!");
}
}
Après avoir exécuté la classe WriteEmployeeDataEx, on obtient un fichier avec un contenu déroutant. Pour lire son contenu, vous devez utiliser la classe ObjectInputStream.
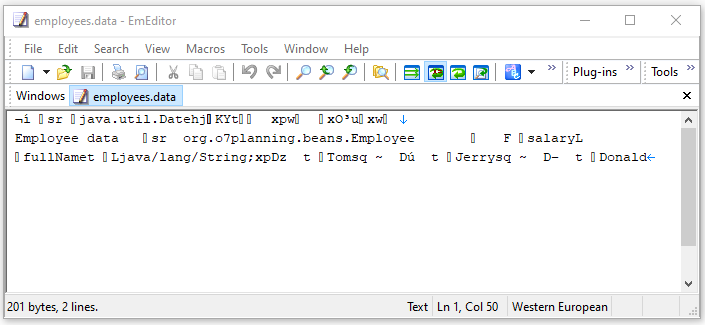
OK, lire le fichier écrit dans l'étape précédente avec ObjectInputStream.
ReadEmployeeDataEx.java
package org.o7planning.objectoutputstream.ex;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.ObjectInputStream;
import java.util.Date;
import org.o7planning.beans.Employee;
public class ReadEmployeeDataEx {
// Windows: C:/Data/test/employees.data
private static String file_path = "/Volumes/Data/test/employees.data";
public static void main(String[] args) throws IOException, ClassNotFoundException {
File inFile = new File(file_path);
InputStream is = new FileInputStream(inFile);
ObjectInputStream ois = new ObjectInputStream(is);
System.out.println("Reading file: " + inFile.getAbsolutePath());
System.out.println();
Date date = (Date) ois.readObject();
String info = ois.readUTF();
System.out.println(date);
System.out.println(info);
System.out.println();
int employeeCount = ois.readInt();
for(int i=0; i< employeeCount; i++) {
Employee e = (Employee) ois.readObject();
System.out.println("Employee Name: " + e.getFullName() +" / Salary: " + e.getSalary());
}
ois.close();
}
}
Output:
Reading file: /Volumes/Data/test/employees.data
Sat Mar 20 18:54:24 KGT 2021
Employee data
Employee Name: Tom / Salary: 1000.0
Employee Name: Jerry / Salary: 2000.0
Employee Name: Donald / Salary: 1200.0
3. Example 2
La plupart des classes de Java Collection Framework implémentent une interface Serializable, telle que ArrayList, LinkedList, HashMap, LinkedHashMap, TreeMap, ... de sorte que leur objet est accessible en écriture sur ObjectOutputStream.
Par exemple: Ecrire un objet ArrayList sur le fichier.
Remarque: Tous les éléments d'ArrayList doit être de type Serializable.
WriteListEx1.java
package org.o7planning.objectoutputstream.ex;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
public class WriteListEx1 {
// Windows: C:/Data/test/flowers.data
private static String file_path = "/Volumes/Data/test/flowers.data";
public static void main(String[] args) throws IOException, ClassNotFoundException {
writeFile();
readFile();
}
private static void writeFile() throws IOException {
ArrayList<String> flowers = new ArrayList<String>();
flowers.add("Tulip");
flowers.add("Daffodil");
flowers.add("Poppy");
flowers.add("Sunflower");
flowers.add("Bluebell");
File file = new File(file_path);
file.getParentFile().mkdirs();
OutputStream os = new FileOutputStream(file);
ObjectOutputStream oos = new ObjectOutputStream(os);
// Write a String
oos.writeUTF("A list of flowers");
// Write an Object
oos.writeObject(flowers);
oos.close();
}
@SuppressWarnings("unchecked")
private static void readFile() throws IOException, ClassNotFoundException {
File file = new File(file_path);
file.getParentFile().mkdirs();
InputStream is = new FileInputStream(file);
ObjectInputStream ois = new ObjectInputStream(is);
// Read a String
String info = ois.readUTF();
// Read an Object
List<String> flowers = (List<String>) ois.readObject();
System.out.println(info);
System.out.println();
for (String s : flowers) {
System.out.println(s);
}
ois.close();
}
}
Output:
A list of flowers
Tulip
Daffodil
Poppy
Sunflower
Bluebell
4. writeFields()
Supposons que vous ayez un objet GameSetting et que vous vouliez l'écrire dans ObjectOutputStream, mais pas dans tous ses champs (field).
GameSetting.java
package org.o7planning.beans;
import java.io.IOException;
import java.io.ObjectOutputStream;
public class GameSetting implements java.io.Serializable {
private static final long serialVersionUID = 1L;
private int sound;
private int bightness;
private String difficultyLevel;
private String userNote;
public GameSetting(int sound, int bightness, String difficultyLevel, String userNote) {
this.sound = sound;
this.bightness = bightness;
this.difficultyLevel = difficultyLevel;
this.userNote = userNote;
}
public int getSound() {
return sound;
}
public int getBightness() {
return bightness;
}
public String getDifficultyLevel() {
return difficultyLevel;
}
public String getUserNote() {
return userNote;
}
// Do not change name and parameter of this method.
private void writeObject(ObjectOutputStream out) throws IOException {
ObjectOutputStream.PutField fields = out.putFields();
// Write this object with custom fields
fields.put("sound", this.sound < 20 ? 20 : this.sound);
fields.put("bightness", this.bightness < 30 ? 30 : this.bightness);
fields.put("difficultyLevel", this.difficultyLevel);
// Do not write "userNote".
// fields.put("userNote", this.userNote);
out.writeFields();
}
}
ObjectOutputStream_writeFields.java
package org.o7planning.objectoutputstream.ex;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.OutputStream;
import java.util.Date;
import org.o7planning.beans.GameSetting;
public class ObjectOutputStream_writeFields {
// Windows: C:/Data/test/game_setting.data
private static String file_path = "/Volumes/Data/test/game_setting.data";
public static void main(String[] args) throws IOException, ClassNotFoundException {
GameSetting setting = new GameSetting(10, 80, "Hard", "Try game again!");
writeGameSetting(setting);
readGameSetting();
}
private static void writeGameSetting(GameSetting setting) throws IOException {
File file = new File(file_path);
file.getParentFile().mkdirs();
OutputStream os = new FileOutputStream(file);
ObjectOutputStream oos = new ObjectOutputStream(os);
// Write a String
oos.writeUTF("Game Settings, Save at " + new Date());
// Write Object
oos.writeObject(setting);
oos.close();
}
private static void readGameSetting() throws IOException, ClassNotFoundException {
File file = new File(file_path);
file.getParentFile().mkdirs();
InputStream is = new FileInputStream(file);
ObjectInputStream ois = new ObjectInputStream(is);
// Read a String
String info = ois.readUTF();
// Read fields
GameSetting setting = (GameSetting) ois.readObject();
System.out.println("sound: " + setting.getSound());
System.out.println("bightness: " + setting.getBightness());
System.out.println("difficultyLevel: " + setting.getDifficultyLevel());
System.out.println("userNote: " + setting.getUserNote()); // null.
ois.close();
}
}
Output:
sound: 20
bightness: 80
difficultyLevel: Hard
userNote: null
Voir plus sur la méthode ObjectInputStream.readFields():
5. writeUnshared(Object)
La méthode writeUnshared(Object) fonctionne de manière similaire à la méthode writeObject(Object) sauf dans la situation suivante:
Supposons que vous souhaitiez écrire un objet "X" à deux reprises dans un ObjectOutputStream, que se passera-t-il?
|
|
|
|
Par exemple, utiliser la méthode writeObject pour écrire à deux reprises un objet ArrayList dans un fichier; et utiliser la méthode writeUnshared pour écrire à deux reprises un objet ArrayList dans un autre fichier. Le deuxième fichier aura une taille plus grande.
ObjectOutputStream_writeUnshared.java
package org.o7planning.objectoutputstream.ex;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import java.io.OutputStream;
import java.util.ArrayList;
public class ObjectOutputStream_writeUnshared {
// Windows: C:/Data/test/test1.data
private static String file_path1 = "/Volumes/Data/test/test1.data";
private static String file_path2 = "/Volumes/Data/test/test2.data";
public static void main(String[] args) throws IOException, ClassNotFoundException {
writeObjectTest();
writeUnsharedTest();
}
private static void writeObjectTest() throws IOException {
File file = new File(file_path1);
file.getParentFile().mkdirs();
ArrayList<String> list = new ArrayList<String>();
list.add("One");
list.add("Two");
OutputStream os = new FileOutputStream(file);
ObjectOutputStream oos = new ObjectOutputStream(os);
oos.writeObject(list); // Write the first time
oos.writeObject(list); // Write the second time
oos.close();
}
private static void writeUnsharedTest() throws IOException {
File file = new File(file_path2);
file.getParentFile().mkdirs();
ArrayList<String> list = new ArrayList<String>();
list.add("One");
list.add("Two");
OutputStream os = new FileOutputStream(file);
ObjectOutputStream oos = new ObjectOutputStream(os);
oos.writeUnshared(list); // Write the first time
oos.writeUnshared(list); // Write the second time
oos.close();
}
}
Output:
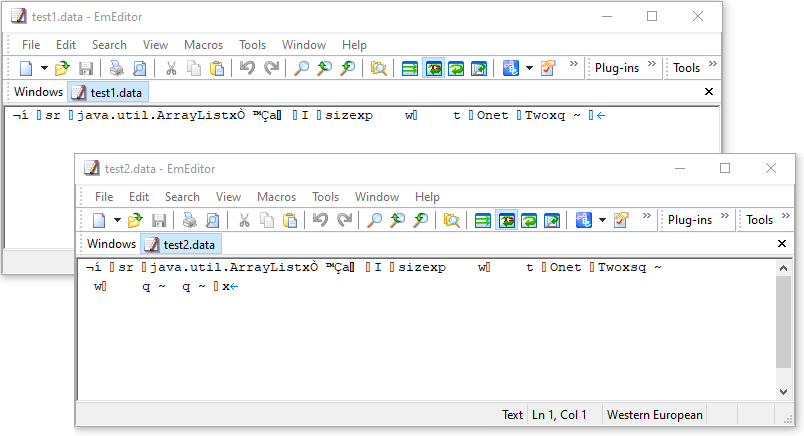
Tutoriels Java IO
- Le Tutoriel de Java CharArrayWriter
- Le Tutoriel de Java FilterReader
- Le Tutoriel de Java FilterWriter
- Le Tutoriel de Java PrintStream
- Le Tutoriel de Java BufferedReader
- Le Tutoriel de Java BufferedWriter
- Le Tutoriel de Java StringReader
- Le Tutoriel de Java StringWriter
- Le Tutoriel de Java PipedReader
- Le Tutoriel de Java LineNumberReader
- Le Tutoriel de Java PushbackReader
- Le Tutoriel de Java PrintWriter
- Tutoriel sur les flux binaires Java IO
- Le Tutoriel de Java IO Character Streams
- Le Tutoriel de Java BufferedOutputStream
- Le Tutoriel de Java ByteArrayOutputStream
- Le Tutoriel de Java DataOutputStream
- Le Tutoriel de Java PipedInputStream
- Le Tutoriel de Java OutputStream
- Le Tutoriel de Java ObjectOutputStream
- Le Tutoriel de Java PushbackInputStream
- Le Tutoriel de Java SequenceInputStream
- Le Tutoriel de Java BufferedInputStream
- Le Tutoriel de Java Reader
- Le Tutoriel de Java Writer
- Le Tutoriel de Java FileReader
- Le Tutoriel de Java FileWriter
- Le Tutoriel de Java CharArrayReader
- Le Tutoriel de Java ByteArrayInputStream
- Le Tutoriel de Java DataInputStream
- Le Tutoriel de Java ObjectInputStream
- Le Tutoriel de Java InputStreamReader
- Le Tutoriel de Java OutputStreamWriter
- Le Tutoriel de Java InputStream
- Le Tutoriel de Java FileInputStream
Show More