Le Tutoriel de Java Writer
1. Writer
Writer est une classe dans le package java.io, qui est une classe de base représentant un flux de caractères (stream of characters) qui a pour mission d'écrire les caractères sur une cible, un fichier par exemple.
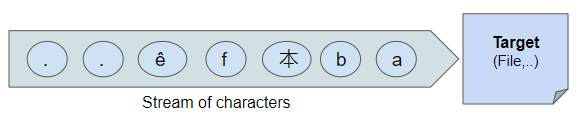
public abstract class Writer implements Appendable, Closeable, Flushable
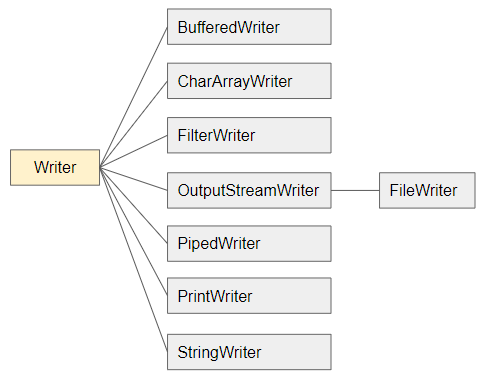
En règle générale, vous ne pouvez pas utiliser directement la classe Writer, car il s'agit d'une classe abstraite. Mais dans un cas particulier, vous pouvez utiliser l'une de ses sous-classes.
Observer un scénario d'écriture de caractères suivants dans un fichier avec un encodage UTF-8:
JP日本-八洲
Java utilise 2 bytes pour stocker un caractère, et ci-dessous une ilustration de bytes du texte susmentionné:
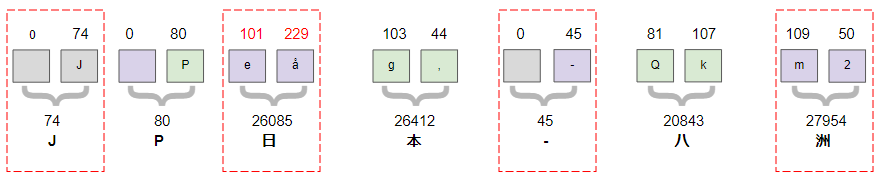
FileWriter est une sous-classe de Writer utilisée pour écrire les caractères sur un fichier texte. Chaque caractère dans Writer est de 2 bytes, mais lorsque vous les écrivez sur un fichier texte UTF-8, chaque caractère peut être stocké par 1, 2, 3 ou 4 bytes.
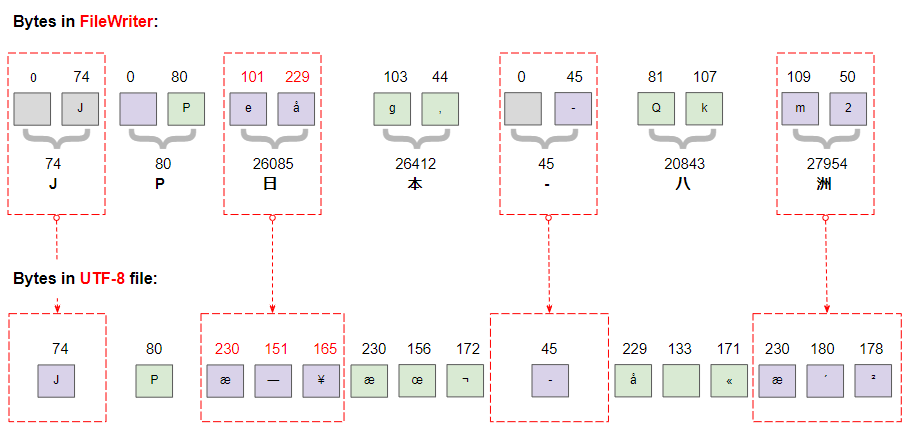
Voir aussi mon explication sur la manière dont Java convertit les caractères Java à ceux d'UTF-8:
Writer methods
public static Writer nullWriter()
public void write(int c) throws IOException
public void write(char cbuf[]) throws IOException
public void write(char cbuf[], int off, int len) throws IOException
public void write(String str) throws IOException
public void write(String str, int off, int len) throws IOException
public Writer append(CharSequence csq) throws IOException
public Writer append(CharSequence csq, int start, int end) throws IOException
public Writer append(char c) throws IOException
public void flush() throws IOException
public void close() throws IOException
2. write(int)
public void write(int chr) throws IOException
Ecrire un caractère sur Writer. Le paramètre chr, qui est le code du caractère, est un entier compris entre 0 et 65535. Si la valeur de chr se trouve à l'extérieur du champ susmentionné, elle sera ignorée.
Writer_write_ex1.java
package org.o7planning.writer.ex;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.Writer;
import java.nio.charset.StandardCharsets;
public class Writer_write_ex1 {
// Windows path: C:/Somedirectory/out-file.txt
private static final String file_path = "/Volumes/Data/test/out-file.txt";
public static void main(String[] args) throws IOException {
File file = new File(file_path);
// Create parent folder:
file.getParentFile().mkdirs();
// FileWriter constructor in Java 11.
Writer writer = new FileWriter(file, StandardCharsets.UTF_8);
writer.write((int)'J'); // 74
writer.write((int)'P'); // 80
writer.write((int)'日'); // 26085
writer.write(26412); // 本
writer.write((int)'-'); // 45
writer.write(20843); // 八
writer.write((int)'洲'); // 27954
writer.close();
}
}
Output:
out-file.txt
JP日本-八洲
3. write(char[])
public void write(char[] cbuf) throws IOException
Ecrire un tableau de caractère sur Writer.
Writer_write_ex2.java
package org.o7planning.writer.ex;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.Writer;
import java.nio.charset.StandardCharsets;
public class Writer_write_ex2 {
// Windows path: C:/Somedirectory/out-file.txt
private static final String file_path = "/Volumes/Data/test/out-file.txt";
public static void main(String[] args) throws IOException {
File file = new File(file_path);
// Create parent folder:
file.getParentFile().mkdirs();
// FileWriter constructor in Java 11.
Writer writer = new FileWriter(file, StandardCharsets.UTF_8);
writer.write((int)'J'); // 74
char[] cbuf = new char[] {'P', '日', '本', '-', '八', '洲'};
writer.write(cbuf);
writer.close();
}
}
Output:
out-file.txt
JP日本-八洲
4. write(char[], int, int)
public void write(char[] cbuf, int off, int len) throws IOException
Ecrire une partie du tableau de caractère sur Writer. Ecrire les caractères à partir de l'indice offset à l'indice offset+len.
Writer_write_ex3.java
package org.o7planning.writer.ex;
import java.io.IOException;
import java.io.StringWriter;
public class Writer_write_ex3 {
public static void main(String[] args) throws IOException {
// StringWriter is a subclass of Writer.
StringWriter stringWriter = new StringWriter();
char[] cbuf = new char[] {'0', '1', '2', '3', '4', '5', '6', '7', '8', '9'};
stringWriter.write(cbuf, 2, 5); // '2', ...'6'
String s = stringWriter.toString();
System.out.println(s);
stringWriter.close();
}
}
Output:
23456
5. close()
public void close() throws IOException
La méthode close() est utilisée pour fermer ce flux, la méthode flush() sera convoquée en premier. Lorsque le flux est fermé, les autres appels write() ou flush() entraînera la levée d'une IOException. La fermeture d'un flux précédemment fermé ne donne aucun effet.
public interface Closeable extends AutoCloseable
La classe Writer implémente l'interface Closeable. Si vous écrivez le code selon les règles d'AutoCloseable, le système fermera automatiquement le flux sans avoir à convoquer directement la méthode close().
Writer_close_ex1.java
package org.o7planning.writer.ex;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.Writer;
import java.nio.charset.StandardCharsets;
public class Writer_close_ex1 {
// Windows path: C:/Somedirectory/out-file.txt
private static final String file_path = "/Volumes/Data/test/out-file.txt";
public static void main(String[] args) throws IOException {
File file = new File(file_path);
// Create parent folder:
file.getParentFile().mkdirs();
try (Writer writer = new FileWriter(file, StandardCharsets.UTF_8)) {
writer.write((int) 'J'); // 74
writer.write((int) 'P'); // 80
writer.write((int) '日'); // 26085
writer.write(26412); // 本
writer.write((int) '-'); // 45
writer.write(20843); // 八
writer.write((int) '洲'); // 27954
}
}
}
- Le Tutoriel de Java Closeable
6. write(String)
public void write(String str) throws IOException
Ecrire une String sur Writer.
Par exemple:
writer.write("Some String");
7. write(String, int, int)
public void write(String str, int offset, int len) throws IOException
Ecrire une partie d'une String sur Writer. Ecrire les caractères à partir de l'indice offset à celui d'offset+len sur Writer.
Par exemple:
writer.write("0123456789", 2, 5); // 23456
8. append(CharSequence)
public Writer append(CharSequence csq) throws IOException
Ajouter les caractères de CharSequence à Writer. Cette méthode renvoie l'actuel objet Writer, ce qui vous permet de convoquer une autre méthode de Writer plutôt que de terminer par un point-virgule (;).
Writer_append_ex1.java
package org.o7planning.writer.ex;
import java.io.IOException;
import java.io.StringWriter;
import java.io.Writer;
public class Writer_append_ex1 {
public static void main(String[] args) throws IOException {
// StringWriter is a subclass of Writer.
Writer writer = new StringWriter();
writer.append("01234").append("5678").write("9");
System.out.println(writer.toString()); // 0123456789
writer.close();
}
}
9. append(CharSequence, int , int)
public Writer append(CharSequence csq, int start, int end) throws IOException
Ajouter une partie de CharSequence à Writer. Cette méthode renvoie l'actuel objet Writer.
10. append(char)
public Writer append(char chr) throws IOException
Ajouter un caractère à Writer. Cette méthode renvoie l'actuel objet Writer, ce qui vous permet de convoquer une autre méthode de Writer plutôt que de terminer par un point-virgule (;).
Writer_append_ex2.java
package org.o7planning.writer.ex;
import java.io.IOException;
import java.io.StringWriter;
import java.io.Writer;
public class Writer_append_ex2 {
public static void main(String[] args) throws IOException {
Writer writer = new StringWriter();
writer.append('J').append('P').append('日').append('本');
System.out.println(writer.toString()); // JP日本
writer.close();
}
}
11. flush()
public void flush() throws IOException
Les données que vous écrivez sur Writer sont parfois stockées temporairement dans une mémoire tampon. La méthode flush() est utilisée pour vider (flush) toutes les données de la mémoire tampon dans la cible.
Vous pouvez lire l'article ci-dessous sur BufferedWriter pour en savoir plus sur la méthode flush().
Si la destination prévue de ce Writer est une abstraction fournie par le système d'exploitation sous-jacent, un fichier par exemple, le vidage du flux ne garantit que les caractères précédemment écrits dans le flux sont transmis au système d'exploitation pour l'écriture; cela ne garantit pas qu'ils sont réellement écrits sur un périphérique physique tel qu'un lecteur de disque.
Tutoriels Java IO
- Le Tutoriel de Java CharArrayWriter
- Le Tutoriel de Java FilterReader
- Le Tutoriel de Java FilterWriter
- Le Tutoriel de Java PrintStream
- Le Tutoriel de Java BufferedReader
- Le Tutoriel de Java BufferedWriter
- Le Tutoriel de Java StringReader
- Le Tutoriel de Java StringWriter
- Le Tutoriel de Java PipedReader
- Le Tutoriel de Java LineNumberReader
- Le Tutoriel de Java PushbackReader
- Le Tutoriel de Java PrintWriter
- Tutoriel sur les flux binaires Java IO
- Le Tutoriel de Java IO Character Streams
- Le Tutoriel de Java BufferedOutputStream
- Le Tutoriel de Java ByteArrayOutputStream
- Le Tutoriel de Java DataOutputStream
- Le Tutoriel de Java PipedInputStream
- Le Tutoriel de Java OutputStream
- Le Tutoriel de Java ObjectOutputStream
- Le Tutoriel de Java PushbackInputStream
- Le Tutoriel de Java SequenceInputStream
- Le Tutoriel de Java BufferedInputStream
- Le Tutoriel de Java Reader
- Le Tutoriel de Java Writer
- Le Tutoriel de Java FileReader
- Le Tutoriel de Java FileWriter
- Le Tutoriel de Java CharArrayReader
- Le Tutoriel de Java ByteArrayInputStream
- Le Tutoriel de Java DataInputStream
- Le Tutoriel de Java ObjectInputStream
- Le Tutoriel de Java InputStreamReader
- Le Tutoriel de Java OutputStreamWriter
- Le Tutoriel de Java InputStream
- Le Tutoriel de Java FileInputStream
Show More