Le Tutoriel de Java SWT Text
1. SWT Text
La classe Text est un control qui accepte et affiche l'entrée du texte. Elle fournit des possibilités de récupérer la saisie du texte du utilisateur.
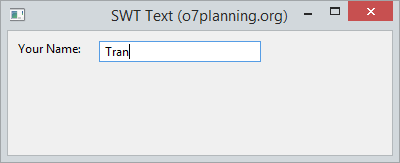
La classe Text permet de créer une saisie de texte qui permet aux utilisateurs de saisir sur une seule ligne. Il peut également créer une saisie de texte pour entrer sur plusieurs lignes ou pour créer un champ du mot de passe (Password field).
Voyez plus sur le champ du mot de passe (Password Field) dans SWT:
// Style ....
int style = SWT.BORDER;
int style = SWT.BORDER | SWT.PASSWORD;
Text text = new Text(parent, style);
Les styles (Style) peuvent être appliqués au Text:
Style | Description |
SWT.BORDER | Créer un texte avec une bordure (border). |
SWT.MULTI | Autoriser de saisir le contenu sur plusieurs lignes. |
SWT.PASSWORD | Pour afficher le Texte comme le champ du mot de passe. |
SWT.V_SCROLL | Afficher la barre de défilement verticale, habituellement utilisée avec SWT.MULTI. |
SWT.H_SCROLL | Afficher la barre de défilement horizontale, habituellement utilisée avec SWT.MULTI. |
SWT.WRAP | Enroulez (wrap) automatiquement si le texte est trop long. |
SWT.READ_ONLY | Ne pas autoriser l'édition, il est équivalent d'appeler la méthode text.setEditable(false); |
SWT.LEFT | Alignement du texte à gauche |
SWT.RIGHT | Aligner du texte à droite |
SWT.CENTER | Aligner du texte au centre |
Examinez quelques méthodes utiles que vous pouvez utiliser avec les champs de Text.
text.setEnabled(enabled);
text.setFont(font);
text.setForeground(color);
text.setEditable(editable);
text.setTextLimit(limit);
text.setToolTipText(string);
text.setTextDirection(textDirection);
text.addSegmentListener(listener);
2. Exemple avec SWT Text
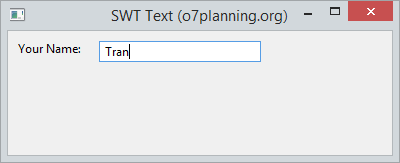
TextDemo.java
package org.o7planning.swt.text;
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.RowData;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
public class TextDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT Text (o7planning.org)");
RowLayout rowLayout = new RowLayout();
rowLayout.marginLeft = 10;
rowLayout.marginTop = 10;
rowLayout.spacing = 15;
shell.setLayout(rowLayout);
// Label
Label label = new Label(shell, SWT.NONE);
label.setText("Your Name: ");
// Text
Text text = new Text(shell, SWT.BORDER);
RowData layoutData = new RowData();
layoutData.width = 150;
text.setLayoutData(layoutData);
text.setText("Tran");
shell.setSize(400, 200);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
3. SWT Text et des Styles
L'exemple ci-dessous illustre la création de différent SWT Text avec des styles tels que SWT.BORDER, SWT.PASSWORD, SWT.MULTI, SWT.WRAP, SWT.READ_ONLY, ...
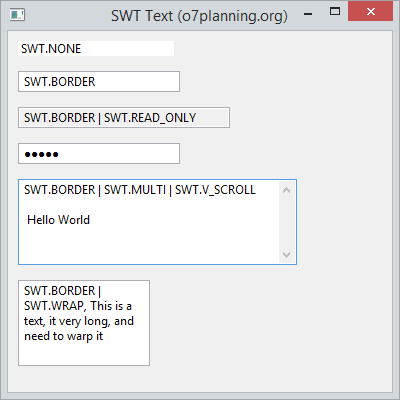
TextStylesDemo.java
package org.o7planning.swt.text;
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.RowData;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
public class TextStylesDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT Text (o7planning.org)");
RowLayout rowLayout = new RowLayout(SWT.VERTICAL);
rowLayout.marginLeft = 10;
rowLayout.marginTop = 10;
rowLayout.spacing = 15;
shell.setLayout(rowLayout);
// Text without border
Text text1 = new Text(shell, SWT.NONE);
text1.setText("SWT.NONE");
text1.setLayoutData(new RowData(150, SWT.DEFAULT));
// Text with border
Text text2 = new Text(shell, SWT.BORDER);
text2.setText("SWT.BORDER");
text2.setLayoutData(new RowData(150, SWT.DEFAULT));
// Text with border and readonly.
Text text3 = new Text(shell, SWT.BORDER | SWT.READ_ONLY);
text3.setText("SWT.BORDER | SWT.READ_ONLY");
text3.setLayoutData(new RowData(200, SWT.DEFAULT));
// Password field.
Text text4 = new Text(shell, SWT.BORDER | SWT.PASSWORD);
text4.setText("12345");
text4.setLayoutData(new RowData(150, SWT.DEFAULT));
// Text with multi lines and show vertiacal scroll.
Text text5 = new Text(shell, SWT.BORDER | SWT.MULTI | SWT.V_SCROLL);
text5.setText("SWT.BORDER | SWT.MULTI | SWT.V_SCROLL \n\n Hello World");
text5.setLayoutData(new RowData(250, 80));
// Wrap
Text text6 = new Text(shell, SWT.BORDER | SWT.WRAP);
text6.setLayoutData(new RowData(120, 80));
text6.setText("SWT.BORDER | SWT.WRAP, This is a text, it very long, and need to warp it");
shell.setSize(400, 400);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
4. Des méthodes utiles
L'exemple ci-dessous illustre l'utilisation des méthodes telles que clear(),copy(), paste(), cut(), qui sont les méthodes utiles du TextField.
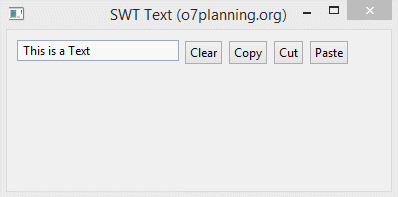
TextDemo2.java
package org.o7planning.swt.text;
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.layout.RowData;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
public class TextDemo2 {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT Text (o7planning.org)");
RowLayout rowLayout = new RowLayout();
rowLayout.marginLeft = 10;
rowLayout.marginTop = 10;
rowLayout.spacing = 5;
shell.setLayout(rowLayout);
// Text
Text text = new Text(shell, SWT.BORDER);
text.setLayoutData(new RowData(150, SWT.DEFAULT));
text.setText("This is a Text");
// Clear Button
Button clearButton = new Button(shell, SWT.PUSH);
clearButton.setText("Clear");
// Copy Button
Button copyButton = new Button(shell, SWT.PUSH);
copyButton.setText("Copy");
// Cut Button
Button cutButton = new Button(shell, SWT.PUSH);
cutButton.setText("Cut");
// Paste Button
Button pasteButton = new Button(shell, SWT.PUSH);
pasteButton.setText("Paste");
clearButton.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
text.setText("");
text.forceFocus();
}
});
copyButton.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
text.copy();
text.forceFocus();
}
});
cutButton.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
text.cut();
text.forceFocus();
}
});
pasteButton.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
text.paste();
text.forceFocus();
}
});
shell.setSize(400, 200);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
Tutoriels de programmation Java SWT
- Le Tutoriel de Java SWT FillLayout
- Le Tutoriel de Java SWT RowLayout
- Le Tutorial de Java SWT SashForm
- Le Tutoriel de Java SWT Label
- Le Tutoriel de Java SWT Button
- Le Tutoriel de Java SWT Toggle Button
- Le Tutoriel de Java SWT Radio Button
- Le Tutoriel de Java SWT Text
- Le Tutoriel de Java SWT Password Field
- Le Tutoriel de Java SWT Link
- Programmation de l'application Java Desktop à l'aide de SWT
- Le Tutoriel de Java SWT Combo
- Le Tutoriel de Java SWT Spinner
- Le Tutoriel de Java SWT Slider
- Le Tutoriel de Java SWT Scale
- Le Tutoriel de Java SWT ProgressBar
- Le Tutoriel de Java SWT TabFolder et CTabFolder
- Le Tutoriel de Java SWT List
Show More