Boucles dans Thymeleaf
1. Boucle (Loop)
Thymeleaf vous fournit boucle 'each', et vous pouvez l'utiliser à travers l'attribut (attribue) th:each. C'est la seule boucle supportée dans Thymeleaf.
Cette boucle accepte certains types de données tels que :
- Les objets implémentent (implements) l'interface java.util.Iterable.
- Les objets implémentent (implements) l'interface java.util.Map.
- Des tableaux (Arrays)
La syntaxe la plus simple de th:each :
<someHtmlTag th:each="item : ${items}">
....
</someHtmlTag>
L'étiquette <th:block> est une étiquette virtuelle dans Thymeleaf, elle ne correspond à aucune étiquette HTML, mais elle est utile dans de nombreux cas, par exemple, vous pouvez définir l'attribut (attribute) th:each dans cette étiquette.
<th:block th:each="item : ${items}">
....
</th:block>
La syntaxe la plus simple de la boucle th:each :
(Java Spring)
@RequestMapping("/loop-simple-example")
public String loopExample1(Model model) {
String[] flowers = new String[] { "Rose", "Lily", "Tulip", "Carnation", "Hyacinth" };
model.addAttribute("flowers", flowers);
return "loop-simple-example";
}
loop-simple-example.html (Template)
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Loop</title>
</head>
<body>
<h1>th:each</h1>
<ul>
<th:block th:each="flower : ${flowers}">
<li th:utext="${flower}">..</li>
</th:block>
</ul>
</body>
</html>
Sortie (Output) :
(Output)
<!DOCTYPE HTML>
<html>
<head>
<meta charset="UTF-8" />
<title>Loop</title>
</head>
<body>
<h1>th:each</h1>
<ul>
<li>Rose</li>
<li>Lily</li>
<li>Tulip</li>
<li>Carnation</li>
<li>Hyacinth</li>
</ul>
</body>
</html>
La syntaxe complète de th:each comprend deux variables, variable item (item variable) et variable d'état (state variable).
<someHtmlTag th:each="item, iState : ${items}">
.....
</someHtmlTag>
<!-- OR: -->
<th:block th:each="item, iState : ${items}">
.....
</th:block>
Variable d'état (State variable) est un objet utile. Elle contient les informations vous indiquant l'état actuel de la boucle, par exemple, le nombre d'éléments de la boucle, l'index actuel de la boucle, ....
Vous trouverez ci-dessous une liste des propriétés (property) de la variable d'état (state variable) :
Propriété | Description |
index | L'indice d'itération (iteration) actuel, commençant par 0 (zéro) |
count | Le nombre d'éléments traités jusqu'à présent |
size | Le nombre total d'éléments de la liste |
even/odd | Vérifie si l'indice (index) d'itération (iteration) actuel est pair ou impair. |
first | Vérifie si l'itération en cours est la première |
last | Vérifie si l'itération en cours est la dernière |
Exemple de th:each et variable d'état (state variable) :
(Java Spring)
@RequestMapping("/loop-example")
public String loopExample(Model model) {
String[] flowers = new String[] { "Rose", "Lily", "Tulip", "Carnation", "Hyacinth" };
model.addAttribute("flowers", flowers);
return "loop-example";
}
loop-example.html (Template)
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Loop</title>
<style>table th, table td {padding: 5px;}</style>
</head>
<body>
<h1>th:each</h1>
<table border="1">
<tr>
<th>index</th>
<th>count</th>
<th>size</th>
<th>even</th>
<th>odd</th>
<th>first</th>
<th>last</th>
<th>Flower Name</th>
</tr>
<tr th:each="flower, state : ${flowers}">
<td th:utext="${state.index}">index</td>
<td th:utext="${state.count}">count</td>
<td th:utext="${state.size}">size</td>
<td th:utext="${state.even}">even</td>
<td th:utext="${state.odd}">odd</td>
<td th:utext="${state.first}">first</td>
<td th:utext="${state.last}">last</td>
<td th:utext="${flower}">Flower Name</td>
</tr>
</table>
</body>
</html>
Résultat :
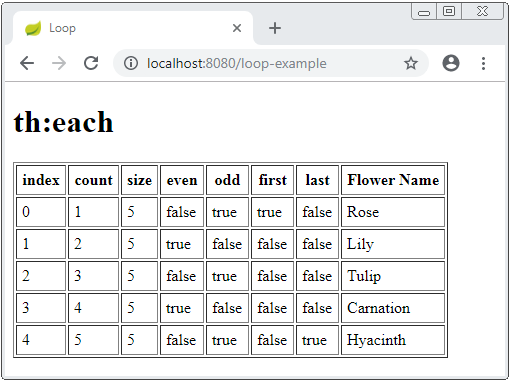
2. Exemple avec la boucle th:each
Quelques autres exemples pour vous aider à mieux comprendre la boucle de Thymeleaf :
- Exemple d'utilisation d'une boucle avec objet List.
- Exemple d'utilisation d'une boucle avec objet Map.
- Créer un tableau directement sur Thymeleaf Template et utiliser la boucle pour cet objet.
Person.java
package org.o7planning.thymeleaf.model;
public class Person {
private Long id;
private String fullName;
private String email;
public Person() {
}
public Person(Long id, String fullName, String email) {
this.id = id;
this.fullName = fullName;
this.email = email;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getFullName() {
return fullName;
}
public void setFullName(String fullName) {
this.fullName = fullName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
th:each & List
Exemple avec th:each et List :
(Java Spring)
@RequestMapping("/loop-list-example")
public String loopListExample(Model model) {
Person tom = new Person(1L, "Tom", "tom@waltdisney.com");
Person jerry = new Person(2L, "Jerry", "jerry@waltdisney.com");
Person donald = new Person(3L, "Donald", "donald@waltdisney.com");
List<Person> list = new ArrayList<Person>();
list.add(tom);
list.add(jerry);
list.add(donald);
model.addAttribute("people", list);
return "loop-list-example";
}
loop-list-example.html (Template)
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Loop</title>
<style>
table th, table td {padding: 5px;}
.row {
font-style: italic;
}
.even-row {
color: black;
}
.odd-row {
color: blue;
}
</style>
</head>
<body>
<h1>th:each</h1>
<table border="1">
<tr>
<th>No</th>
<th>Full Name</th>
<th>Email</th>
</tr>
<tr th:each="person, state : ${people}"
class="row" th:classappend="${state.odd} ? 'odd-row' : 'even-row'">
<td th:utext="${state.count}">No</td>
<td th:utext="${person.fullName}">Full Name</td>
<td th:utext="${person.email}">Email</td>
</tr>
</table>
</body>
</html>
Résultat :
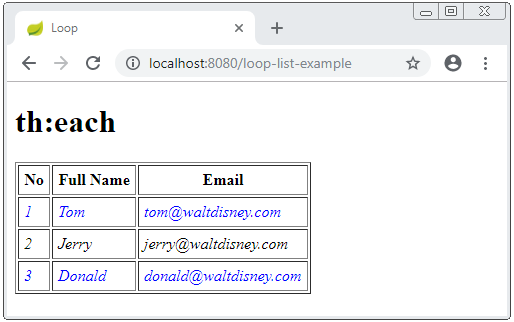
th:each & Map
Exemple de th:each et Map :
(Java Spring)
@RequestMapping("/loop-map-example")
public String loopMapExample(Model model) {
Person tom = new Person(1L, "Tom", "tom@waltdisney.com");
Person jerry = new Person(2L, "Jerry", "jerry@waltdisney.com");
Person donald = new Person(3L, "Donald", "donald@waltdisney.com");
// String: Phone Number.
Map<String, Person> contacts = new HashMap<String, Person>();
contacts.put("110033", tom);
contacts.put("110055", jerry);
contacts.put("110077", donald);
model.addAttribute("contacts", contacts);
return "loop-map-example";
}
loop-map-example.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Loop</title>
<style>
table th, table td {padding: 5px;}
.row {
font-style: italic;
}
.even-row {
color: black;
}
.odd-row {
color: blue;
}
</style>
</head>
<body>
<h1>th:each</h1>
<table border="1">
<tr>
<th>No</th>
<th>Phone</th>
<th>Full Name</th>
<th>Email</th>
</tr>
<tr th:each="mapItem, state : ${contacts}"
class="row" th:classappend="${state.odd} ? 'odd-row' : 'even-row'">
<td th:utext="${state.count}">No</td>
<td th:utext="${mapItem.key}">Phone Number</td>
<td th:utext="${mapItem.value.fullName}">Email</td>
<td th:utext="${mapItem.value.email}">Email</td>
</tr>
</table>
</body>
</html>
Résultat :
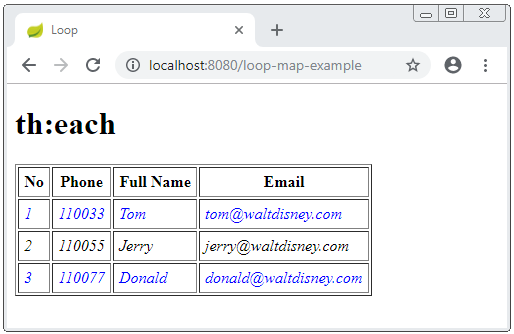
Other example:
Exxemple : Créer un tableau directement sur Thymeleaf Template et utiliser une boucle.
loop-other-example.html (Template)
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Loop</title>
<style>
table th, table td {
padding: 5px;
}
</style>
</head>
<body>
<h1>th:each</h1>
<!-- Create an Array: -->
<th:block th:with="flowers = ${ {'Rose', 'Lily', 'Tulip'} }">
<table border="1">
<tr>
<th>No</th>
<th>Flower</th>
</tr>
<tr th:each="flower, state : ${flowers}">
<td th:utext="${state.count}">No</td>
<td th:utext="${flower}">Flower</td>
</tr>
</table>
</th:block>
</body>
</html>
Résultat :
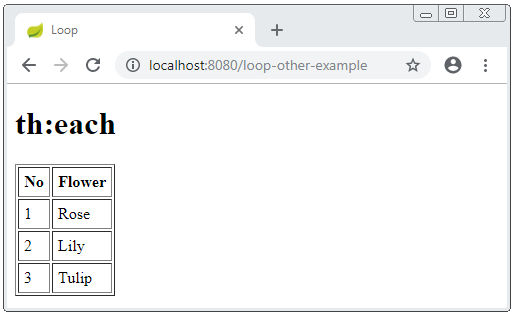
Tutoriels Thymeleaf
- Opérateur Elvis dans Thymeleaf
- Boucles dans Thymeleaf
- Instructions conditionnelles If, unless, switch dans Thymeleaf
- Objets prédéfinis dans Thymeleaf
- Utiliser Thymeleaf th:class, th:classappend, th:style, th:styleappend
- Introduction à Thymeleaf
- Variable dans Thymeleaf
- Utiliser Fragments dans Thymeleaf
- Utiliser Layout dans Thymeleaf
- Utiliser Thymeleaf th:object et syntaxe Asterisk *{ }
- Exemple de Thymeleaf Form Select option
Show More