Variable dans Thymeleaf
1. Biến (Variable)
Vous n’êtes pas étrange au concept de variable dans le langage Java, mais qu’en est-il dans Thymeleaf? Certainement, Thymeleaf a également le concept de variables.
Un attribut (attribute) de l'objet org.springframework.ui.Model, ou un attribut (attribute) de l'objet HttpServletRequest est une variable de Thymeleaf. Cette variable peut être utilisée partout dans Template.
(Java Spring)
@RequestMapping("/variable-example1")
public String variableExample1(Model model, HttpServletRequest request) {
// variable1
model.addAttribute("variable1", "Value of variable1!");
// variable2
request.setAttribute("variable2", "Value of variable2!");
return "variable-example1";
}
variable-example1.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Variable</title>
</head>
<body>
<h1>Variables</h1>
<h4>${variable1}</h4>
<span th:utext="${variable1}"></span>
<h4>${variable2}</h4>
<span th:utext="${variable2}"></span>
</body>
</html>
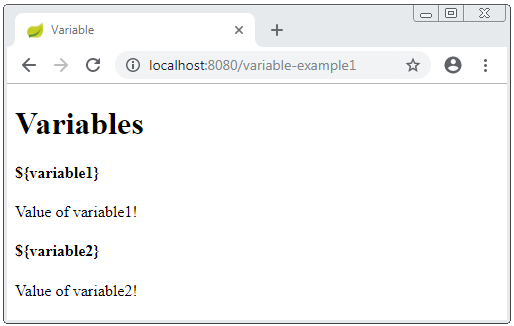
Local Variables
Vous pouvez définir des variables dans Template. Ce sont des variables locales. Elles existent seulement et sont disponibles dans une section Template.
Dans cet exemple, deux variables flower, iter seulement existent et sont disponibles dans la boucle qui les a déclarées.
(Java Spring)
@RequestMapping("/variable-in-loop")
public String objectServletContext(Model model, HttpServletRequest request) {
String[] flowers = new String[] {"Rose","Lily", "Tulip", "Carnation", "Hyacinth" };
model.addAttribute("flowers", flowers);
return "variable-in-loop";
}
variable-in-loop.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Variable</title>
</head>
<body>
<h1>Variable: flower, iter</h1>
<table border="1">
<tr>
<th>No</th>
<th>Flower Name</th>
</tr>
<!--
Local Variable: flower
Local Variable: iter (Iterator).
-->
<tr th:each="flower, iter : ${flowers}">
<td th:utext="${iter.count}">No</td>
<td th:utext="${flower}">Flower Name</td>
</tr>
</table>
</body>
</html>
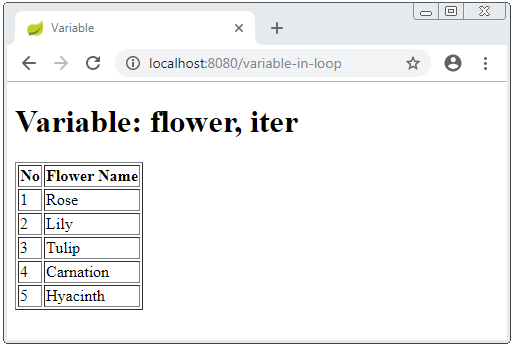
th:with
Vous pouvez également créer une ou plusieurs variables locales via l'attribut (attribute) th:with. Sa syntaxe est comme une "expression d'attribution de valeur" régulière.
(Java Spring)
@RequestMapping("/variable-example3")
public String variableExample3(Model model) {
String[] flowers = new String[] {"Rose","Lily", "Tulip", "Carnation", "Hyacinth" };
model.addAttribute("flowers", flowers);
return "variable-example3";
}
variable-example3.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Variable</title>
</head>
<body>
<h1>th:with</h1>
<!-- Local variable: flower0 -->
<div th:with="flower0 = ${flowers[0]}">
<h4>${flower0}</h4>
<span th:utext="${flower0}"></span>
</div>
<!-- Local variable: flower1, flower2 -->
<div th:with="flower1 = ${flowers[1]}, flower2 = ${flowers[2]}">
<h4>${flower1}, ${flower2}</h4>
<span th:utext="${flower1}"></span>
<br/>
<span th:utext="${flower2}"></span>
</div>
<hr>
<!-- Local variable: firstName, lastName, fullName -->
<div th:with="firstName = 'James', lastName = 'Smith', fullName = ${firstName} +' ' + ${lastName}">
First Name: <span th:utext="${firstName}"></span>
<br>
Last Name: <span th:utext="${lastName}"></span>
<br>
Full Name: <span th:utext="${fullName}"></span>
</div>
</body>
</html>
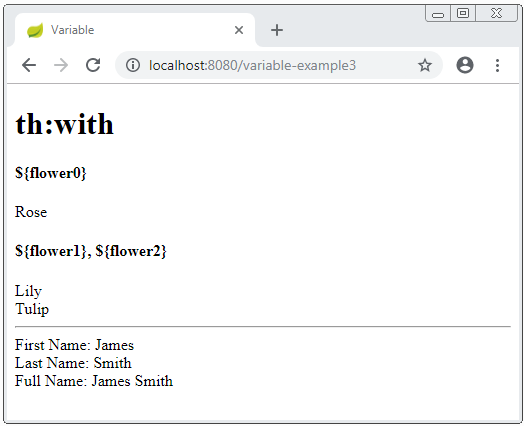
Exemple de la création d'un tableau (array) dans Thymeleaf:
variable-array-example.html (Template)
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Variable</title>
</head>
<body>
<h1>th:with (Array)</h1>
<!-- Create an Array: -->
<th:block th:with="flowers = ${ {'Rose', 'Lily', 'Tulip'} }">
<table border="1">
<tr>
<th>No</th>
<th>Flower</th>
</tr>
<tr th:each="flower, state : ${flowers}">
<td th:utext="${state.count}">No</td>
<td th:utext="${flower}">Flower</td>
</tr>
</table>
</th:block>
</body>
</html>
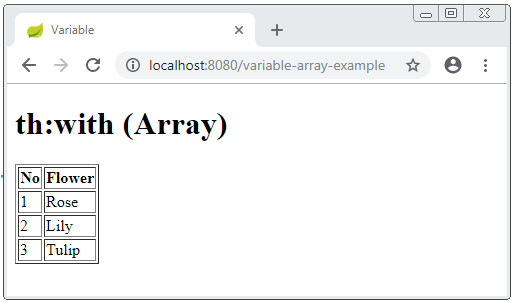
Tutoriels Thymeleaf
- Opérateur Elvis dans Thymeleaf
- Boucles dans Thymeleaf
- Instructions conditionnelles If, unless, switch dans Thymeleaf
- Objets prédéfinis dans Thymeleaf
- Utiliser Thymeleaf th:class, th:classappend, th:style, th:styleappend
- Introduction à Thymeleaf
- Variable dans Thymeleaf
- Utiliser Fragments dans Thymeleaf
- Utiliser Layout dans Thymeleaf
- Utiliser Thymeleaf th:object et syntaxe Asterisk *{ }
- Exemple de Thymeleaf Form Select option
Show More