Le Tutoriel de AngularJS Filter
1. AngularJS Filters
Dans AngularJS, Filter (Filtre) sert à formater les données affichées pour les utilisateurs.
Ci-dessous une syntaxe générale utilisant Filter :
Filters Usage
{{ expression [| filter_name[:parameter_value] ... ] }}
AngularJS a construit quelques Filter basiques et vous pouvez les utiliser.
Filter | Description |
lowercase | Format a string to lower case. |
uppercase | Format a string to upper case. |
number | Format a number to a string. |
currency | Format a number to a currency format. |
date | Format a date to a specified format. |
json | Format an object to a JSON string. |
filter | Select a subset of items from an array. |
limitTo | Limits an array/string, into a specified number of elements/characters. |
orderBy | Orders an array by an expression. |
2. uppercase/lowercase
upperlowercase-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.greeting = "Hello World";
});
upperlowercase-example
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="upperlowercase-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filters: uppercase/lowercase</h3>
<p>Origin String: {{ greeting }}</p>
<p>Uppercase: {{ greeting | uppercase }}</p>
<p>Lowercase: {{ greeting | lowercase }}</p>
</div>
</body>
</html>
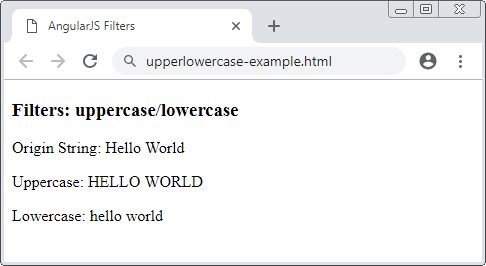
3. number
Filtre (filter) number aide à formater un numéro dans une chaine.
L'usage dans HTML :
<!-- fractionSize: Optional -->
{{ number_expression | number : fractionSize }}
L'usage dans Javascript :
// fractionSize: Optional
$filter('number')( aNumber , fractionSize )
Des paramètres :
aNumber |
number
string | un numéro à formater. |
fractionSize (Optionnel) | number
string | Nombre de décimales (decimal). Si vous ne fournissez pas des valeurs à ce paramètre, ses valeurs vont dépendre à locale. Dans le cas de locale par défaut, ses valeurs seront 3. |
number-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="number-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: number</h3>
<p><b>Origin Number:</b> {{ revenueAmount }}</p>
<p><b>Default Number Format:</b> {{ revenueAmount | number }}</p>
<p><b>Format with 2 decimal places:</b> {{ revenueAmount | number : 2 }}</p>
</div>
</body>
</html>
number-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.revenueAmount = 20011.2345;
});
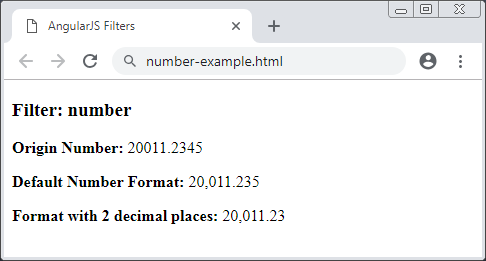
Par exemple, utilisez un filtre number dans Javascript :
number-example2.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope, $filter) {
var aNumber = 19001.2345;
// Use number filter in Javascript:
$scope.revenueAmountStr = $filter("number")(aNumber, 2);// ==> 19,001.23
});
4. currency
Le filtre (filter) currency est utilisé pour formater certaines fonctions de monnaie (par exemple $1,234.56). Fondamentalement, vous devez fournir un symbol de monnaie pour le formatage, au contraire, le symbol de monnaie locale actuel sera utilisé.
L'usage dans HTML :
{{ amount | currency : symbol : fractionSize}}
L'usage dans Javascript :
$filter('currency')(amount, symbol, fractionSize)
Des paramètres :
amount | number | un numéro. |
symbol (optional) | string | Symbol (symbol) de monnaie, ou quelque chose équivaut pour afficher. |
fractionSize (optional) | number | Nombre de décimales (decimal). Au cas où il n'est pas fournit, leur valeurs seront par défaut basées sur le locale courant du navigateur. |
Exemple :
currency-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.myAmount = 12345.678;
});
currency-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="currency-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: currency</h3>
Enter Amount:
<input type="number" ng-model="myAmount" />
<p>
<b ng-non-bindable>{{ myAmount | currency }}</b>:
<span>{{ myAmount | currency }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '$' : 2 }}</b>:
<span>{{ myAmount | currency : '$' : 2 }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '€' : 1 }}</b>:
<span>{{ myAmount | currency : '€' : 1 }}</span>
</p>
</div>
</body>
</html>
Normalement le symbol de monnai se trouve devant le montant (Par exemple €123.45), cela dépend à locale. Vous pouvez préciser un locale que vous voulez utiliser, par exemple locale_de-de, où vous recevrez le résultat de 123.45€.
Cherchez les bibliothèques locale de AngularJS :
currency-locale-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.myAmount = 12345.678;
});
currency-locale-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<!-- Use locale_de-de -->
<!-- https://cdnjs.com/libraries/angular-i18n -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/angular-i18n/1.7.4/angular-locale_de-de.js"></script>
<script src="currency-locale-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: currency (LOCALE: de-de)</h3>
Enter Amount:
<input type="number" ng-model="myAmount" />
<p>
<b ng-non-bindable>{{ myAmount | currency }}</b>:
<span>{{ myAmount | currency }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '$' : 2 }}</b>:
<span>{{ myAmount | currency : '$' : 2 }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '€' : 1 }}</b>:
<span>{{ myAmount | currency : '€' : 1 }}</span>
</p>
</div>
</body>
</html>
5. date
Le filtre date est utilisé pour formater un objet Date en une chaine.
L'usage du filtre date dans HTML :
{{ date_expression | aDate : format : timezone}}
L'usage du filtre date dans Javascript :
$filter('date')(aDate, format, timezone)
Des paramètres :
TODO
Exemple :
date-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.myDate = new Date();
});
date-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="date-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: date</h3>
<p>
<!-- $scope.myDate = new Date() -->
<b ng-non-bindable>{{ myDate | date: 'yyyy-MM-dd HH:mm'}}</b>:
<span>{{ myDate | date: 'yyyy-MM-dd HH:mm'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:'medium'}}</b>:
<span>{{1288323623006 | date:'medium'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:'yyyy-MM-dd HH:mm:ss Z'}}</b>:
<span>{{1288323623006 | date:'yyyy-MM-dd HH:mm:ss Z'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:'MM/dd/yyyy @ h:mma'}}</b>:
<span>{{'1288323623006' | date:'MM/dd/yyyy @ h:mma'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:"MM/dd/yyyy 'at' h:mma"}}</b>:
<span>{{'1288323623006' | date:"MM/dd/yyyy 'at' h:mma"}}</span>
</p>
</div>
</body>
</html>
6. json
Le filtre json sert à convertir un objet (object) en une chaine du format JSON.
L'usage dans le HTML :
{{ json_expression | json : spacing}}
L'usage dans Javascript :
$filter('json')(object, spacing)
Des paramètres :
object | * | Un objet JavaScript quelconque (y compris des tableaux et des types primatifs (primitive) ) |
spacing (optional) | number | Nombre d'espaces à utiliser par indentation, la valeur par défaut est 2. |
Exemple :
json-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.department = {
id : 1,
name: "Sales",
employees : [
{id: 1, fullName: "Tom", gender: "Male"},
{id: 2, fullName: "Jerry", gender: "Male"}
]
};
});
json-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="json-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: json</h3>
<p>
<b ng-non-bindable>{{ department | json : 5 }}</b>:
<pre>{{ department | json : 5}}</pre>
</p>
<p>
<b ng-non-bindable>{{ department | json }}</b>:
<pre>{{ department | json }}</pre>
</p>
</div>
</body>
</html>
7. limitTo
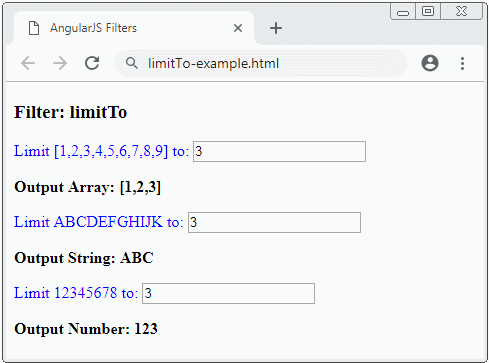
L'usage dans le HTML :
{{ input | limitTo : limit : begin}}
L'usage dans le Javascript :
$filter('limitTo')(input, limitLength, startIndex)
Des paramètres :
Exemple :
limitTo-example.js
var app = angular.module("myApp", [ ]);
app.controller("myCtrl", function($scope) {
$scope.anArray = [1,2,3,4,5,6,7,8,9];
$scope.aString = "ABCDEFGHIJK";
$scope.aNumber = 12345678;
$scope.arrayLimit = 3;
$scope.stringLimit = 3;
$scope.numberLimit = 3;
});
limitTo-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="limitTo-example.js"></script>
<style>
p {color: blue;}
</style>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: limitTo</h3>
<p>
Limit {{anArray}} to:
<input type="number" step="1" ng-model="arrayLimit">
</p>
<b>Output Array: {{ anArray | limitTo: arrayLimit }}</b>
<p>
Limit {{aString}} to:
<input type="number" step="1" ng-model="stringLimit">
</p>
<b>Output String: {{ aString | limitTo: stringLimit }}</b>
<p>
Limit {{aNumber}} to:
<input type="number" step="1" ng-model="numberLimit">
</p>
<b>Output Number: {{ aNumber | limitTo: numberLimit }}</b>
</div>
</body>
</html>
8. filter
Le filtre filter sert à sélectionner un sous-ensemble (subset) dans un tableau et renvoie un nouveau tableau.
L'usage dans le HTML :
{{ inputArray | filter : expression : comparator : anyPropertyKey}}
L'usage dans le Javascript :
$filter('filter')(inputArray, expression, comparator, anyPropertyKey)