Récupérer des informations géographiques basées sur l'adresse IP à l'aide de GeoIP2 Java API
1. Qu'est-ce que GeoIP2?
GeoIP2 est une bibliothèque de source ouverte Java. il offre gratuitement GeoLite2 qui est une base de données de localisation correspondant à l'adresse IP, et des API pour travailler avec cette base de données ainsi que le service web (Web service)qui fournit des données de localisation.
GeoLite2 est une base de données de localisation grauite, ses données sont mises à jours fréquemment le premier mardi (Tuesday) de chaque mois.
GeoLite2 est une base de données de localisation grauite, ses données sont mises à jours fréquemment le premier mardi (Tuesday) de chaque mois.
GeoIP2 soutient de plusieurs langages de la programmation tels que: C#, C, Java, Perl, PHP, Python,...
Il y a 2 manières de travailler avec GeoIP2 API:
- Téléchargez GeoLite2 avec des données de localisations et utilisez les comme une base de données locale. GeoIP2 API va récupérer des informations géographiques à partir de cette base de données.
- Utilisez GeoIP2 API pour connecter au service en ligne qui fournit des informations de locatisation via l'adresse IP, De cette façon, vous avez besoin d'une License_Key (vous devez l'acheter).
2. Download GeoIP2
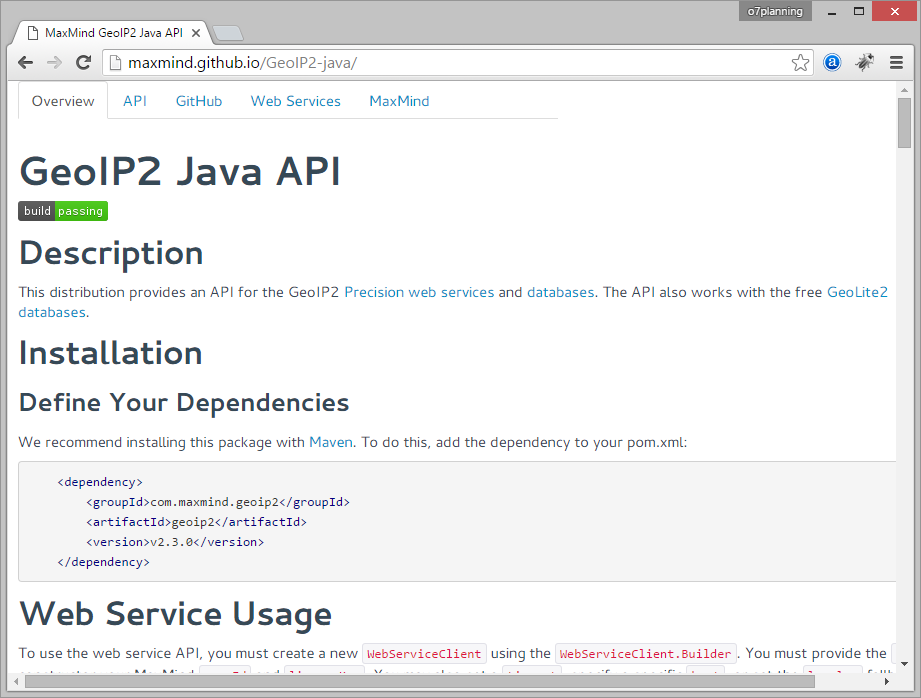
Si vous utilisez Maven:
<!-- http://mvnrepository.com/artifact/com.maxmind.geoip2/geoip2 -->
<dependency>
<groupId>com.maxmind.geoip2</groupId>
<artifactId>geoip2</artifactId>
<version>2.15.0</version>
</dependency>
ou téléchargez cette bibliothèque:
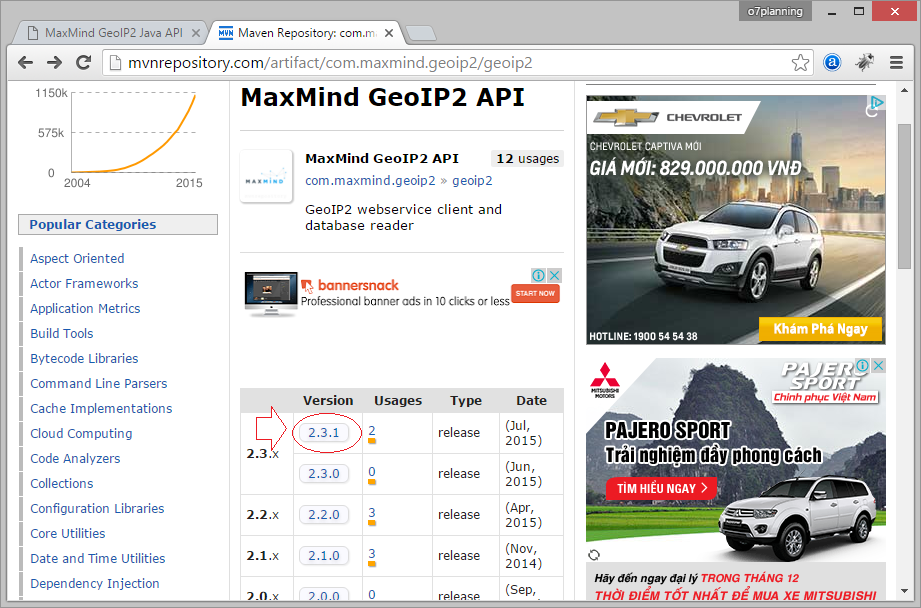
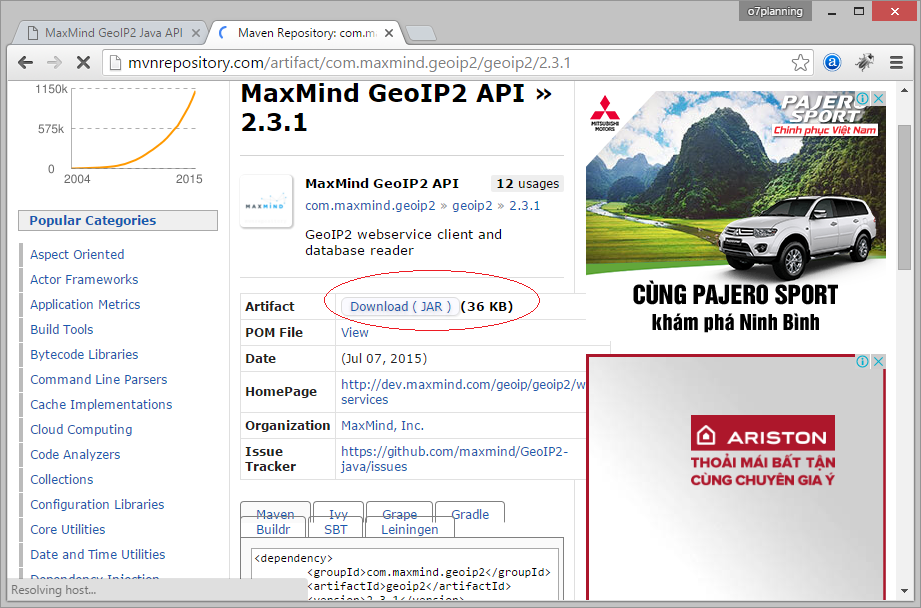
3. Download GeoLite2
To download GeoLite2 database you need to register for an account, which is completely free.
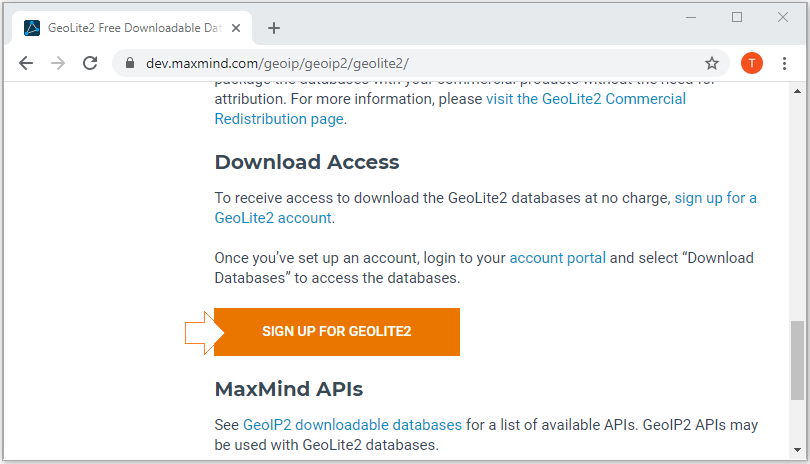
Then log in with your account:
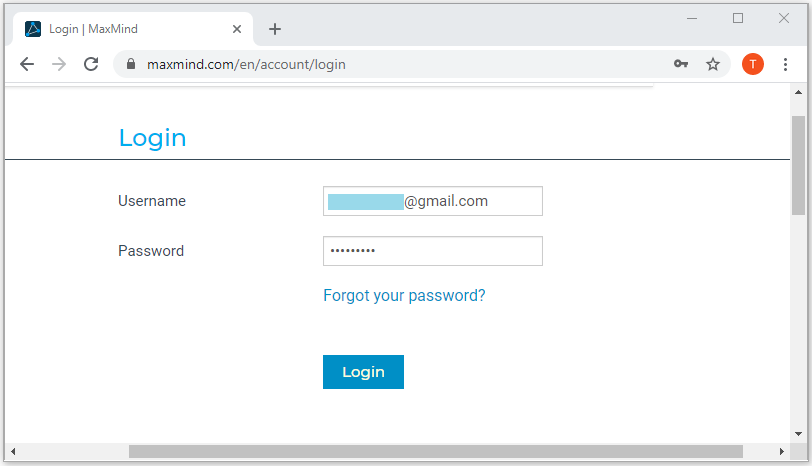
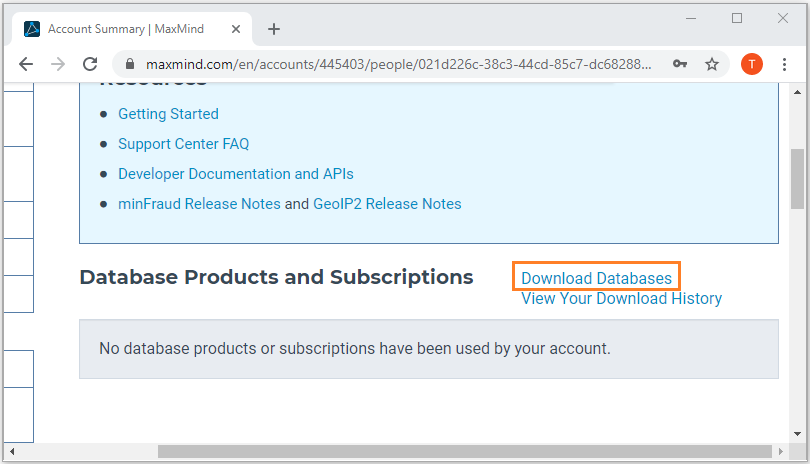
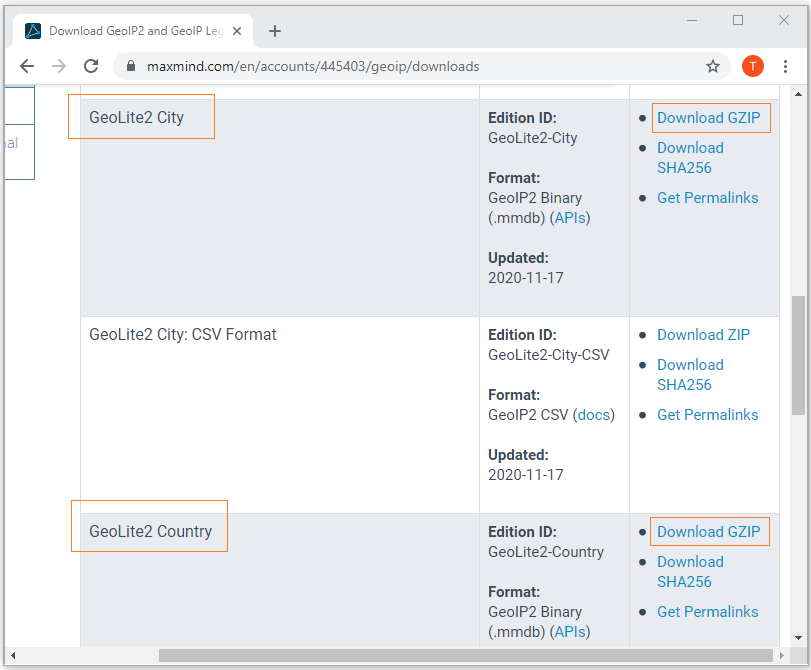
Les résultats sont ci- dessous:
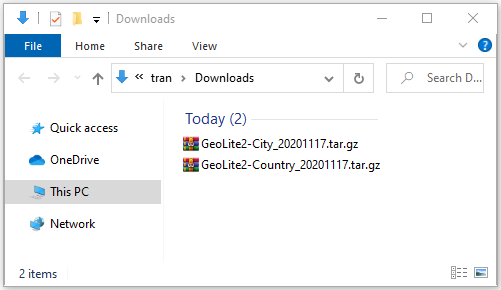
Compressez 2 fichiers:
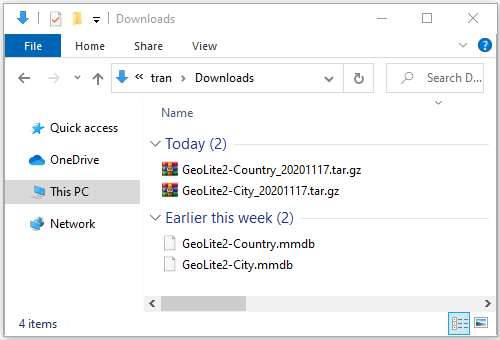
4. Créer rapidement un projet pour travailler avec GeoIP2
Créez un nouveau projet Maven.
- File/New/Other...
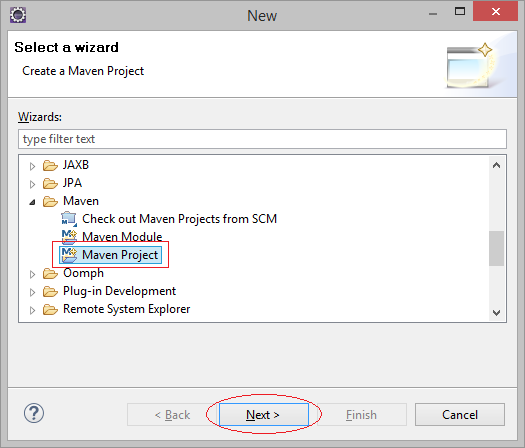
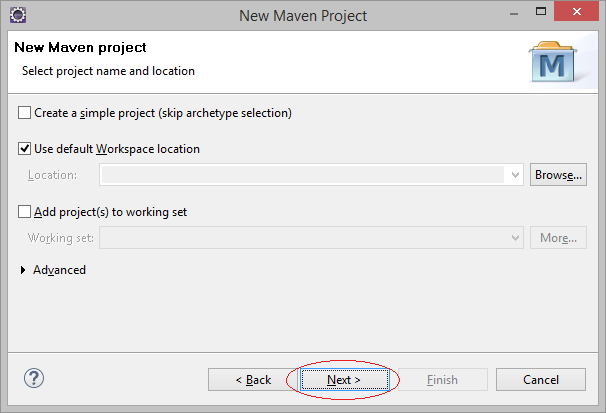
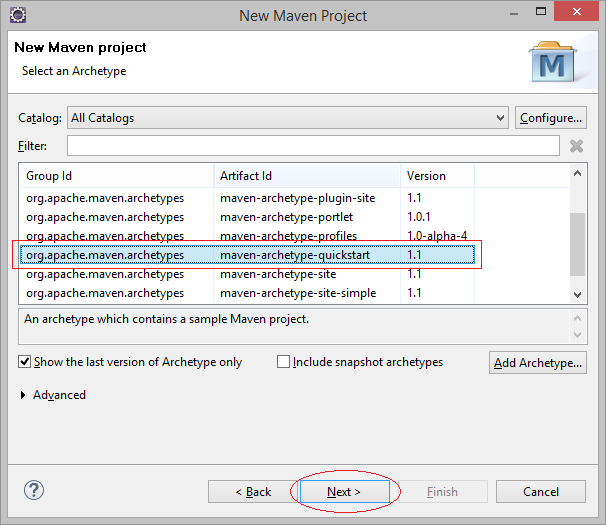
Saisissez:
- Group Id: org.o7planning
- Artifact Id: geoip2tutorial
- Package: org.o7planning.geoip2tutorial
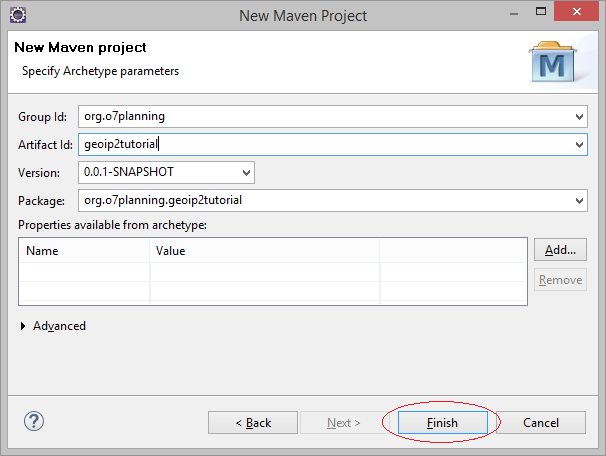
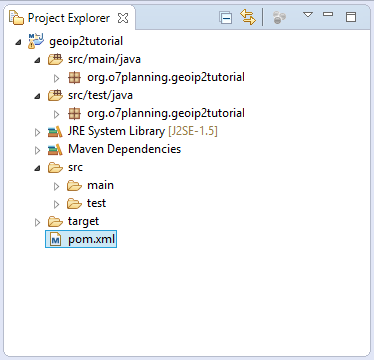
La dépendance maven:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>geoip2tutorial</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>geoip2tutorial</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- http://mvnrepository.com/artifact/com.maxmind.geoip2/geoip2 -->
<dependency>
<groupId>com.maxmind.geoip2</groupId>
<artifactId>geoip2</artifactId>
<version>2.15.0</version>
</dependency>
</dependencies>
</project>
5. L'exemple de GeoIP2
Vous avez téléchargé la base de données GeoLite2 et la mettre dans un fichier local. Par exemple, l'utilisation GeoIP2 Java API pour récupérer des informations géographiques correspondant à une adresse IP précise.
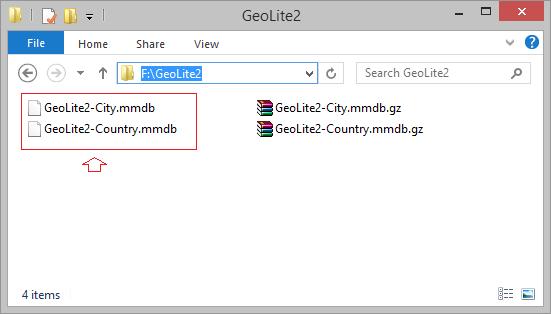
MyConstants.java
package org.o7planning.geoip2tutorial;
public class MyConstants {
// Country Data.
public static final String DATABASE_COUNTRY_PATH = "F:/GeoLite2/GeoLite2-Country.mmdb";
// City Data.
public static final String DATABASE_CITY_PATH = "F:/GeoLite2/GeoLite2-City.mmdb";
public static final int MY_USER_ID = 42;
public static final String MY_LICENSE_KEY = "license_key";
}
HelloGeoIP2.java
package org.o7planning.geoip2tutorial;
import java.io.File;
import java.io.IOException;
import java.net.InetAddress;
import com.maxmind.geoip2.DatabaseReader;
import com.maxmind.geoip2.exception.GeoIp2Exception;
import com.maxmind.geoip2.model.CityResponse;
import com.maxmind.geoip2.record.City;
import com.maxmind.geoip2.record.Country;
import com.maxmind.geoip2.record.Location;
import com.maxmind.geoip2.record.Postal;
import com.maxmind.geoip2.record.Subdivision;
public class HelloGeoIP2 {
public static void main(String[] args) throws IOException, GeoIp2Exception {
// A File object pointing to your GeoLite2 database
File dbFile = new File(MyConstants.DATABASE_CITY_PATH);
// This creates the DatabaseReader object,
// which should be reused across lookups.
DatabaseReader reader = new DatabaseReader.Builder(dbFile).build();
// A IP Address
InetAddress ipAddress = InetAddress.getByName("128.101.101.101");
// Get City info
CityResponse response = reader.city(ipAddress);
// Country Info
Country country = response.getCountry();
System.out.println("Country IsoCode: "+ country.getIsoCode()); // 'US'
System.out.println("Country Name: "+ country.getName()); // 'United States'
System.out.println(country.getNames().get("zh-CN")); // '美国'
Subdivision subdivision = response.getMostSpecificSubdivision();
System.out.println("Subdivision Name: " +subdivision.getName()); // 'Minnesota'
System.out.println("Subdivision IsoCode: "+subdivision.getIsoCode()); // 'MN'
// City Info.
City city = response.getCity();
System.out.println("City Name: "+ city.getName()); // 'Minneapolis'
// Postal info
Postal postal = response.getPostal();
System.out.println(postal.getCode()); // '55455'
// Geo Location info.
Location location = response.getLocation();
// Latitude
System.out.println("Latitude: "+ location.getLatitude()); // 44.9733
// Longitude
System.out.println("Longitude: "+ location.getLongitude()); // -93.2323
}
}
Exécutez l'exemple:
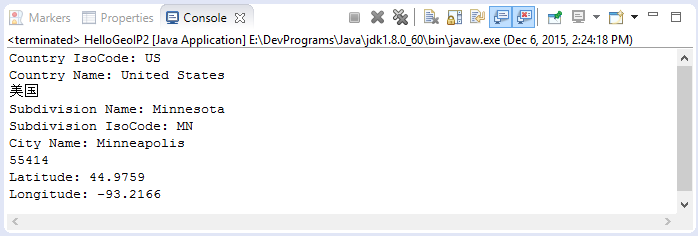
6. L'exemple de service web GeoIP2
Au lieu de connexion à une base de données locale pour récupérer des informations géographiques correspondant à l'adresse IP, vous pouvez utiliser un service en ligne (Web service) qui fournit ces données. Vous devez acheter une LICENSE_KEY. Par exemple, l'exemple des instructions ci-dessous vous indique comment obtenir des informations géographiques correspondant à l'adresse IP à travers le service web.
HelloGeoIP2Service.java
package org.o7planning.geoip2tutorial;
import java.io.IOException;
import java.net.InetAddress;
import com.maxmind.geoip2.WebServiceClient;
import com.maxmind.geoip2.exception.GeoIp2Exception;
import com.maxmind.geoip2.model.CountryResponse;
import com.maxmind.geoip2.record.Country;
public class HelloGeoIP2Service {
public static void main(String[] args) throws IOException, GeoIp2Exception {
WebServiceClient.Builder builder
= new WebServiceClient.Builder(MyConstants.MY_USER_ID, MyConstants.MY_LICENSE_KEY);
WebServiceClient client = builder.build();
// IP Address
InetAddress ipAddress = InetAddress.getByName("128.101.101.101");
// Do the lookup
CountryResponse response = client.country(ipAddress);
// Country Info .
Country country = response.getCountry();
System.out.println("Country Iso Code: "+ country.getIsoCode()); // 'US'
System.out.println("Country Name: "+ country.getName()); // 'United States'
System.out.println(country.getNames().get("zh-CN")); // '美国'
}
}
Tutoriels Java Open Source Libraries
- Le Tutoriel de Java JSON Processing API (JSONP)
- Utilisation de l'API Java Scribe OAuth avec Google OAuth2
- Obtenir des informations sur le matériel dans l'application Java
- Restfb Java API pour Facebook
- Créer Credentials pour Google Drive API
- Le Tutoriel de Java JDOM2
- Le Tutoriel de Java XStream
- Utiliser Java Jsoup pour analyser HTML
- Récupérer des informations géographiques basées sur l'adresse IP à l'aide de GeoIP2 Java API
- Lire et écrire un fichier Excel en Java à l'aide d'Apache POI
- Explorer le Facebook Graph API
- Manipulation de fichiers et de dossiers sur Google Drive à l'aide de Java
Show More