Le Tutoriel de Java Period
1. Period
La classe Period utilise des unités d'année, de mois et de jour pour représenter une période de temps. Par exemple "3 ans 2 mois 15 jours". Si vous souhaitez une durée basée sur les heures, les minutes et les secondes, utilisez la classe Duration.
Les valeurs de l'année, du mois et du jour de la Period sont indépendantes, ce qui signifie que si vous ajoutez ou soustrayez quelques jours de la Period, cela n'affectera pas l'année et le mois. La raison en est que la longueur du mois et de l'année n'est pas fixe.
public final class Period implements ChronoPeriod, Serializable
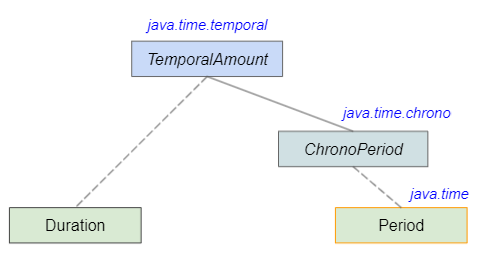
- ChronoPeriod
- Duration
- TemporalAmount
Par exemple : Le footballeur Cristiano Ronaldo est né le 5 février 1985. Le 29 septembre 2002, Ronaldo a fait ses débuts en Primeira Liga, il avait donc 17 ans, 7 mois 24 jours à l'époque.
Period_ronaldo_ex1.java
// 1985-02-05 (Ronaldo's birthday).
LocalDate startInclusiveDate = LocalDate.of(1985, 2, 5);
// 2002-09-29 (First time playing in Primeira Liga ).
LocalDate endExclusiveDate = LocalDate.of(2002, 9, 29);
Period period = Period.between(startInclusiveDate, endExclusiveDate);
System.out.println(period); // P17Y7M24D
System.out.println("Years: " + period.getYears()); // 17
System.out.println("Months: " + period.getMonths()); // 7
System.out.println("Days: " + period.getDays()); // 24
2. Static Factory Methods
Les méthodes de fabrique statiques:
public static final Period ZERO = new Period(0, 0, 0);
public static Period ofYears(int years)
public static Period ofMonths(int months)
public static Period ofWeeks(int weeks)
public static Period ofDays(int days)
public static Period of(int years, int months, int days)
public static Period from(TemporalAmount amount)
public static Period parse(CharSequence text)
public static Period between(LocalDate startDateInclusive, LocalDate endDateExclusive)
3. Other methods
Les autres méthodes:
// -----------------------------------------------------------------
// getX()
// -----------------------------------------------------------------
// Inherited from ChronoPeriod interface
public long get(TemporalUnit unit)
// Inherited from ChronoPeriod interface
public List<TemporalUnit> getUnits()
// Inherited from ChronoPeriod interface
public IsoChronology getChronology()
public int getYears()
public int getMonths()
public int getDays()
// -----------------------------------------------------------------
// withX()
// -----------------------------------------------------------------
public Period withYears(int years)
public Period withMonths(int months)
public Period withDays(int days)
// -----------------------------------------------------------------
// plusX()
// -----------------------------------------------------------------
// Inherited from ChronoPeriod interface
public Period plus(TemporalAmount amountToAdd)
public Period plusYears(long yearsToAdd)
public Period plusMonths(long monthsToAdd)
public Period plusDays(long daysToAdd)
// -----------------------------------------------------------------
// minusX()
// -----------------------------------------------------------------
// Inherited from ChronoPeriod interface
public Period minus(TemporalAmount amountToSubtract)
public Period minusYears(long yearsToSubtract)
public Period minusMonths(long monthsToSubtract)
public Period minusDays(long daysToSubtract)
// -----------------------------------------------------------------
public long toTotalMonths()
// -----------------------------------------------------------------
// Other method inherited from ChronoPeriod interface
// -----------------------------------------------------------------
public boolean isZero()
public boolean isNegative()
public Period multipliedBy(int scalar)
public Period negated()
public Period normalized()
public Temporal addTo(Temporal temporal)
public Temporal subtractFrom(Temporal temporal)
4. get(TemporalUnit)
Renvoyer la valeur de l'unité spécifiée.
// Inherited from ChronoPeriod interface
public long get(TemporalUnit unit) {
Seulement les trois unités standard suivantes sont prises en charge, les autres lèveront une UnsupportedTemporalTypeException.
- ChronoUnit.YEARS
- ChronoUnit.MONTHS
- ChronoUnit.DAYS
Par exemple:
Period_get_unit_ex1.java
// 20 years 5 months 115 days.
Period period = Period.of(20, 5, 115);
long years = period.get(ChronoUnit.YEARS); // 20
long months = period.get(ChronoUnit.MONTHS); // 5
long days = period.get(ChronoUnit.DAYS); // 115
System.out.printf("%d years, %d months %d days", years, months, days);
5. getX() *
La méthode getDays() renvoie le nombre de jours représentés dans cette Period. Les valeurs du jour, du mois et de l'année dans Period sont indépendantes les unes des autres, elles ne peuvent pas être converties les unes aux autres car la longueur du mois et de l'année n'est pas fixe.
Les méthodes getMonths(), getYears() sont également interprétées comme leurs noms indiquent.
public int getYears()
public int getMonths()
public int getDays()
Par exemple : Le 20 juillet 1969, le vaisseau spatial Apollo 11 s'est posé sur la lune. On peut calculer depuis combien de temps cet événement a eu lieu par rapport à aujourd'hui :
Period_getX_ex1.java
// 1969-07-20
LocalDate apollo11Date = LocalDate.of(1969, 7, 20);
// Now
LocalDate now = LocalDate.now();
System.out.println("Now is " + now);
Period period = Period.between(apollo11Date, now);
System.out.println(period);
System.out.println("Years: " + period.getYears());
System.out.println("Months: " + period.getMonths());
System.out.println("Days: " + period.getDays());
Output:
Now is 2021-06-25
P51Y11M5D
Years: 51
Months: 11
Days: 5
En effet, pour calculer le nombre d'années, de mois, de semaines ou de jours entre 2 moments différents, vous pouvez également utiliser la classe ChronoUnit. Voir par exemple :
ChronoUnit_between_ex1.java
// 1969-07-20
LocalDate apollo11Date = LocalDate.of(1969, 7, 20);
// Now
LocalDate now = LocalDate.now();
System.out.println("Now is " + now);
long years = ChronoUnit.YEARS.between(apollo11Date, now);
long months = ChronoUnit.MONTHS.between(apollo11Date, now);
long weeks = ChronoUnit.WEEKS.between(apollo11Date, now);
long days = ChronoUnit.DAYS.between(apollo11Date, now);
System.out.println("Years: " + years);
System.out.println("Months: " + months);
System.out.println("Weeks: " + weeks);
System.out.println("Days:" + days);
Output:
Now is 2021-06-25
Years: 51
Months: 623
Weeks: 2709
Days:18968
6. getUnits()
Renvoyer la ou le(s) TemporalUnit(s) prise(s) en charge par cette Period. Seules les 3 TemporalUnit(s) standard suivantes sont prises en charge :
- ChronoUnit.YEARS
- ChronoUnit.MONTHS
- ChronoUnit.DAYS
// Inherited from ChronoPeriod interface
public List<TemporalUnit> getUnits()
Par exemple:
Period_getUnits_ex1.java
Period period = Period.of(2, 8, 0);
// ChronoUnit.YEARS, ChronoUnit.MONTHS, ChronoUnit.DAYS
List<TemporalUnit> units = period.getUnits();
for(TemporalUnit unit: units) {
System.out.println("Unit: " + unit.getClass().getName() + " - " + unit);
}
Output:
Unit: java.time.temporal.ChronoUnit - Years
Unit: java.time.temporal.ChronoUnit - Months
Unit: java.time.temporal.ChronoUnit - Days
7. getChronology()
Renvoyer la chronologie de cette Period, qui est le système de calandrier ISO (IsoChronology.INSTANCE).
// Inherited from ChronoPeriod interface
public IsoChronology getChronology()
Par exemple :
Period_getChronology_ex1.java
Period period = Period.of(20, 5, 10);
IsoChronology isoChronology = period.getChronology();
System.out.println("IsoChronology: " + isoChronology.getId()); // ISO.
- Le Tutoriel de Java IsoChronology
8. withX(..) *
La méthode withDays(days) renvoie une copie de cette Period avec le nombre de jours remplacé par le nombre de jours spécifié. L'année et le mois ne sont pas affectés par cette méthode.
Les méthodes withYears(years), withMonths(months) sont également interprétées comme leurs noms indiquent.
public Period withYears(int years)
public Period withMonths(int months)
public Period withDays(int days)
Par exemple:
Period_withX_ex1.java
Period period = Period.of(20, 3, 15);
period = period.withDays(100);
System.out.println(period);
System.out.println("Years: " + period.getYears()); // 20
System.out.println("Months: " + period.getMonths()); // 3
System.out.println("Days: " + period.getDays()); // 100
9. plus(TemporalAmount)
Renvoyer une copie de cette Period avec une quantité de temps ajoutée.
public Period plus(TemporalAmount amountToAdd)
Les valeurs du jour, du mois et de l'année sont indépendantes les unes des autres, aucune normalisation ne sera effectuée. Tel que:
- "2 years 10 months 110 days" + "3 years 5 months 10 days" --> "5 years 15 months 120 days".
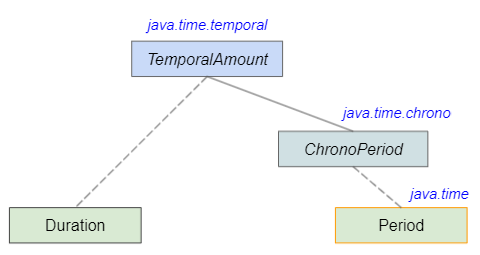
Par exemple:
Period_plus_amount_ex1.java
// 20 years 3 months 15 days.
Period period = Period.of(20, 3, 15);
System.out.println("period: " + period); // P20Y3M15D
TemporalAmount amount = Period.ofMonths(15); // 15 months
Period period2 = period.plus(amount);
System.out.println("period2: " + period2); // P20Y18M15D (20 years, 18 months, 15 days)
10. plusX(..) *
La méthode plusDays(daysToAdd) renvoie une copie de cette Period avec le nombre de jours spécifié ajouté, ce qui n'affecte pas les valeurs de l'année et du mois.
Les méthodes plusYears(yearsToAdd) et plusMonths(monthsToAdd) sont également interprétées comme leurs noms indiquent.
public Period plusYears(long yearsToAdd)
public Period plusMonths(long monthsToAdd)
public Period plusDays(long daysToAdd)
Par exemple :
Period_plusX_ex1.java
Period period = Period.of(20, 3, 15);
// period1
Period period1 = period.plusDays(100);
System.out.println("period1.getYears(): " + period1.getYears()); // 20
System.out.println("period1.getMonths(): " + period1.getMonths()); // 3
System.out.println("period1.getDays(): " + period1.getDays()); // 115
System.out.println(" ------- \n");
// period2
Period period2 = period.plusDays(-100);
System.out.println("period2.getYears(): " + period2.getYears()); // 20
System.out.println("period2.getMonths(): " + period2.getMonths()); // 3
System.out.println("period2.getDays(): " + period2.getDays()); // -85
System.out.println(" ------- \n");
// period3
Period period3 = period.plusYears(-30);
System.out.println("period3.getYears(): " + period3.getYears()); // -10
System.out.println("period3.getMonths(): " + period3.getMonths()); // 3
System.out.println("period3.getDays(): " + period3.getDays()); // 15
Output:
period1.getYears(): 20
period1.getMonths(): 3
period1.getDays(): 115
-------
period2.getYears(): 20
period2.getMonths(): 3
period2.getDays(): -85
-------
period3.getYears(): -10
period3.getMonths(): 3
period3.getDays(): 15
11. minus(TemporalAmount)
Renvoyer une copie de cette Period avec la quantité de temps spécifiée soustraite.
public Period minus(TemporalAmount amountToSubtract)
Les valeurs du jour, du mois et de l'année sont indépendantes les unes des autres, aucune normalisation ne sera effectuée. Tel que:
- "2 years 10 months 110 days" - "3 years 5 months 10 days" --> "-1 years 10 months 90 days".
Par exemple:
Period_minus_amount_ex1.java
// 20 years 3 months 15 days.
Period period = Period.of(20, 3, 15);
System.out.println("period: " + period); // P20Y3M15D
TemporalAmount amount = Period.ofMonths(5); // 5 months
Period period2 = period.minus(amount);
System.out.println("period2: " + period2); // P20Y-2M15D (20 years, -2 months, 15 days)
- Le Tutoriel de Java TemporalAmount
12. minusX(..) *
La méthode minusDays(daysToSubtract) renvoie une copie de cette Period avec le nombre de jours spécifié soustrait, ce qui n'affecte pas les deux autres champs, année et mois.
Les méthodes minusYears(yearsToSubtract) et minusMonths(monthsToSubtract) sont également interprétées comme leurs noms indiquent.
public Period minusYears(long yearsToSubtract)
public Period minusMonths(long monthsToSubtract)
public Period minusDays(long daysToSubtract)
Par exemple:
Period_minusX_ex1.java
Period period = Period.of(20, 3, 15);
// period1
Period period1 = period.minusDays(100);
System.out.println("period1.getYears(): " + period1.getYears()); // 20
System.out.println("period1.getMonths(): " + period1.getMonths()); // 3
System.out.println("period1.getDays(): " + period1.getDays()); // -85
System.out.println(" ------- \n");
// period2
Period period2 = period.minusDays(-100);
System.out.println("period2.getYears(): " + period2.getYears()); // 20
System.out.println("period2.getMonths(): " + period2.getMonths()); // 3
System.out.println("period2.getDays(): " + period2.getDays()); // 115
System.out.println(" ------- \n");
// period3
Period period3 = period.minusYears(30);
System.out.println("period3.getYears(): " + period3.getYears()); // -10
System.out.println("period3.getMonths(): " + period3.getMonths()); // 3
System.out.println("period3.getDays(): " + period3.getDays()); // 15
Output:
period1.getYears(): 20
period1.getMonths(): 3
period1.getDays(): -85
-------
period2.getYears(): 20
period2.getMonths(): 3
period2.getDays(): 115
-------
period3.getYears(): -10
period3.getMonths(): 3
period3.getDays(): 15
13. isZero()
Renvoyer true si les 3 valeurs du jour, du mois et de l'année dans cette Period sont 0, sinon renvoyer false.
// Inherited from ChronoPeriod interface
public boolean isZero()
Par exemple:
Period_isZero_ex1.java
Period period1 = Period.of(0, -1, 30);
System.out.println("period1.isZero()? " + period1.isZero()); // false
Period period2 = Period.of(-1, 12, 0);
System.out.println("period2.isZero()? " + period2.isZero()); // false
Period period3 = Period.of(0, 0, 0);
System.out.println("period3.isZero()? " + period3.isZero()); // true
14. isNegative()
Renvoyer true si un jour, un mois ou une année de cette Period est négatif, sinon renvoie false.
// Inherited from ChronoPeriod interface
public boolean isNegative()
Par exemple:
Period_isNegative_ex1.java
Period period1 = Period.of(10, -1, 30);
System.out.println("period1.isNegative()? " + period1.isNegative()); // true
Period period2 = Period.of(1, -12, -15);
System.out.println("period2.isNegative()? " + period2.isNegative()); // true
Period period3 = Period.of(0, 0, 0);
System.out.println("period3.isNegative()? " + period3.isNegative()); // false
Period period4 = Period.of(5, 10, 15);
System.out.println("period4.isNegative()? " + period4.isNegative()); // false
15. multipliedBy(int)
Multiplier cette Period par une valeur spécifiée et renvoyer une nouvelle Period.
// Inherited from ChronoPeriod interface
public Period multipliedBy(int scalar)
Les valeurs du jour, du mois et de l'année sont indépendantes les unes des autres, aucune normalisation ne sera effectuée.
Par exemple:
Period_multipliedBy_ex1.java
// 20 years 10 months 35 days.
Period period = Period.of(20, 10, 35);
Period result = period.multipliedBy(2);
long years = result.get(ChronoUnit.YEARS); // 40
long months = result.get(ChronoUnit.MONTHS); // 20
long days = result.get(ChronoUnit.DAYS); // 70
System.out.printf("%d years, %d months %d days", years, months, days);
16. negated()
// Inherited from ChronoPeriod interface
public Period negated()
Par exemple:
Period_negated_ex1.java
// 20 years 3 months 15 days.
Period period = Period.of(20, 3, 15);
System.out.println("period: " + period); // P20Y3M15D
// period2 = 0 - period1
Period period2 = period.negated();
System.out.println("period2: " + period2); // P-20Y-3M-15D (-20 Years, -3 months, -15 days)
17. normalized()
Renvoyer une copie de cette Period avec l'année et le mois normalisés. Une année est considérée comme égale à 12 mois, après la normalisation, la valeur du mois sera comprise entre -11 et 11.
// Inherited from ChronoPeriod interface
public Period normalized()
Par exemple :
- "1 years -25 months 50 days" --> "-1 year -1 months 50 days".
Period_normalized_ex1.java
// 1 years -25 months 50 days.
Period period = Period.of(1, -25, 50);
Period result = period.normalized();
long years = result.get(ChronoUnit.YEARS); // -1
long months = result.get(ChronoUnit.MONTHS); // -1
long days = result.get(ChronoUnit.DAYS); // 50
System.out.printf("%d years, %d months %d days", years, months, days);
18. toTotalMonths()
Renvoyer le nombre total de mois de cette Period en multipliant le nombre d'années par 12 et en ajoutant le nombre de mois. Le nombre de jours sera ignoré.
public long toTotalMonths()
Par exemple :
Period_toTotalMonths_ex1.java
// 1 years 3 months 145 days.
Period period = Period.of(1, 3, 145);
System.out.println("period: " + period); // P1Y3M145D
long months = period.toTotalMonths();
System.out.println("period.toTotalMonths(): " + months); // 15
19. addTo(Temporal)
Ajouter cette Period à un objet Temporal spécifié et renvoyer un nouvel objet Temporal.
// Inherited from ChronoPeriod interface
public Temporal addTo(Temporal temporal)
Cette méthode équivaut à utiliser la méthode Temporal.plus(TemporalAmount).
// Defined in Temporal interface.
public Temporal plus(TemporalAmount amount)
Par exemple :
Period_addTo_ex1.java
// Create Temporal object.
LocalDateTime localDateTime = LocalDateTime.of(2000, 1, 10, 23, 30, 0);
System.out.println("localDateTime: " + localDateTime); // 2000-01-10T23:30
// 1 Year 1 month 25 days.
Period period = Period.of(1, 1, 25);
LocalDateTime newLocalDateTime = (LocalDateTime) period.addTo(localDateTime);
System.out.println("newLocalDateTime: " + newLocalDateTime); // 2001-03-07T23:30
20. subtractFrom(Temporal)
Soustraire cette Period d'un objet Temporal spécifié et renvoyer un nouvel objet Temporal.
// Inherited from ChronoPeriod interface
public Temporal subtractFrom(Temporal temporal)
Cette méthode équivaut à utiliser la méthode Temporal.minus(TemporalAmount).
// Defined in Temporal interface.
public Temporal minus(TemporalAmount amount)
Par exemple :
Period_subtractFrom_ex1.java
// Create Temporal object.
LocalDateTime localDateTime = LocalDateTime.of(2000, 1, 10, 23, 30, 0);
System.out.println("localDateTime: " + localDateTime); // 2000-01-10T23:30
// 1 Year 1 month 25 days.
Period period = Period.of(1, 1, 25);
LocalDateTime newLocalDateTime = (LocalDateTime) period.subtractFrom(localDateTime);
System.out.println("newLocalDateTime: " + newLocalDateTime); // 1998-11-15T23:30
Tutoriels Java Date Time
- Le Tutoriel de Java ZoneId
- Le Tutoriel de Java Temporal
- Le Tutoriel de Java Period
- Le Tutoriel de Java TemporalAdjusters
- Le Tutoriel de Java MinguoDate
- Le Tutoriel de Java TemporalAccessor
- Le Tutoriel de Java JapaneseEra
- Le Tutoriel de Java HijrahDate
- Le Tutoriel de Java Date, Time
- Qu'est-ce que l'heure d'été (DST)?
- Le Tutoriel de Java LocalDate
- Le Tutoriel de Java LocalTime
- Le Tutoriel de Java LocalDateTime
- Le Tutoriel de Java ZonedDateTime
- Le Tutoriel de Java JapaneseDate
- Le Tutoriel de Java Duration
- Le Tutoriel de Java TemporalQuery
- Le Tutoriel de Java TemporalAdjuster
- Le Tutoriel de Java ChronoUnit
- Le Tutoriel de Java TemporalQueries
Show More
- Tutoriels Java Web Service
- Tutoriels de programmation Java Servlet/JSP
- Tutoriels de JavaFX
- Tutoriels de programmation Java SWT
- Tutoriels Java Oracle ADF
- Java Basic
- Tutoriels de Java Collections Framework
- Tutoriels Java IO
- Tutoriels Struts2
- Tutoriels Spring Boot
- Tutoriels Spring Cloud
- Tutoriels Maven
- Tutoriels Gradle