Tutoriel Java RESTful Web Service pour débutant
1. Introduction
Le document est écrit sur la base de :
- Eclipse 4.6 (NEON).
- Jersey
Si vous êtes débutant avec RESTful Web Service, vous devez prendre 10 minutes pour savoir ce qui est RESTful Web service avant de commencer avec une application Java RESTful serivce.
Vous pouvez voir "Qu'est-ce que le service web RESTful" ici :
Vous pouvez voir "Qu'est-ce que le service web RESTful" ici :
2. Qu'est-ce que Jersey?
Jersey qui est une plate-forme Java de source ouverte vous permet de développer des applications RESTful Web service et les applications Client connexes. Jersey exécute les spécifications JSR 311.
Le Jersey fournit des bibliothèques de mettre en œuvre RESTful web service dans le conteneur de servlet (Servlet Container).
A côté serveur, Jersey fournit une implémentation de servlet qui analyse des classes prédéfinies pour identifier les ressources RESTful. Dans votre fichier de configuration web.xml, enregistrez cette servlet pour votre application web.
L'implémentation Jersey fournit également une bibliothèque client pour communiquer avec un service web RESTful.
A côté serveur, Jersey fournit une implémentation de servlet qui analyse des classes prédéfinies pour identifier les ressources RESTful. Dans votre fichier de configuration web.xml, enregistrez cette servlet pour votre application web.
L'implémentation Jersey fournit également une bibliothèque client pour communiquer avec un service web RESTful.
Voici la structure de liaison des ressources proposées par Jersey.

Dans le quel com.sun.jersey.spi.container.servlet.ServletContainer est un servlet fourni par JERSEY, et doit être déclaré dans web.xml.
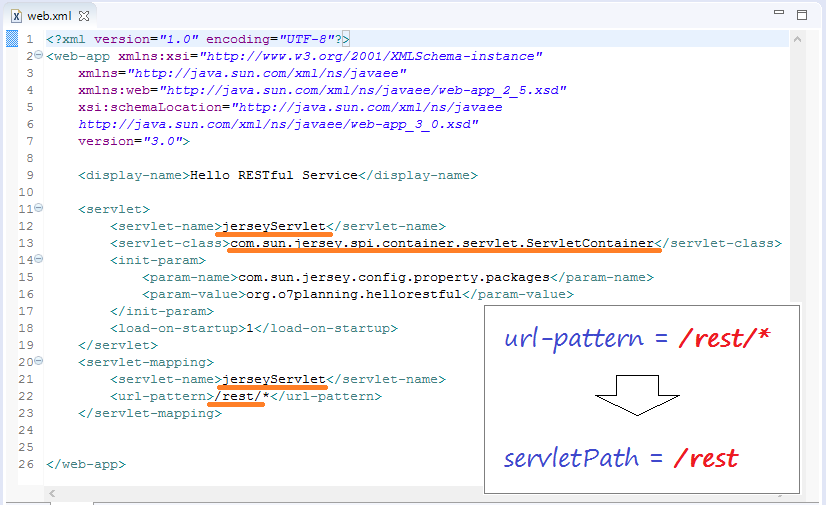
3. Créerr une application Maven
Sur Eclipse sélectionnez :
- File/New/Other..
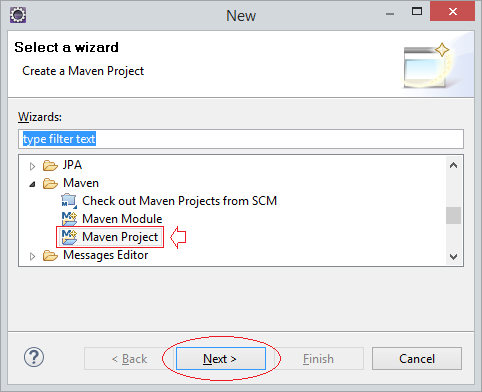
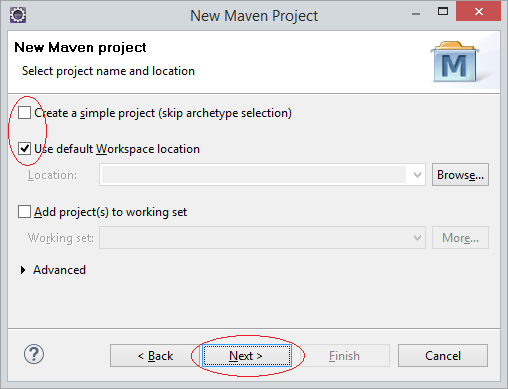
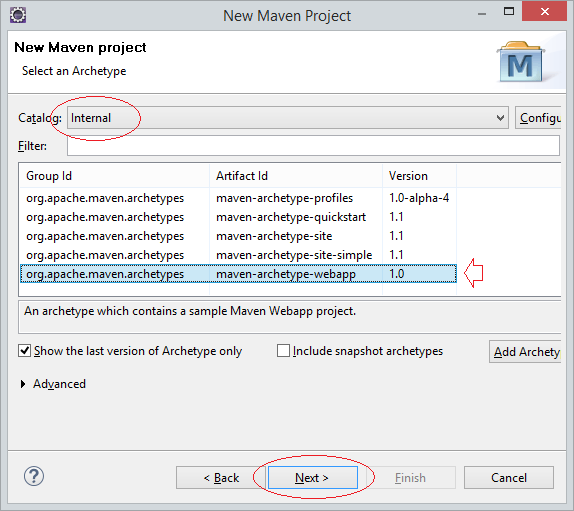
Saisissez :
- Group Id: org.o7planning
- Artifact Id: HelloRESTful
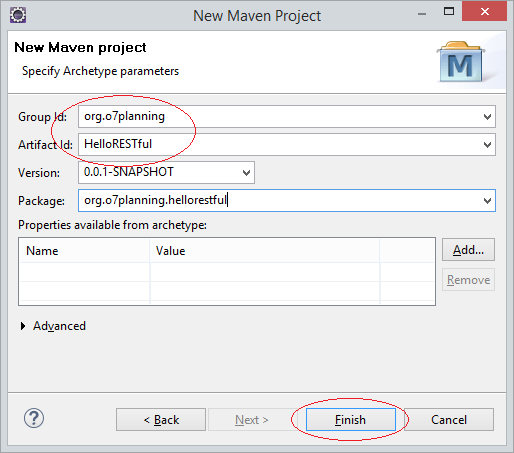
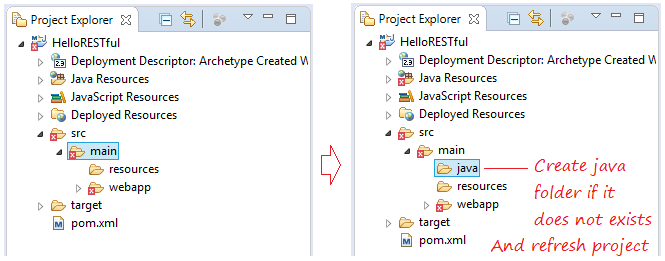
4. Déclarer maven et web.xml
Vous devez déclarer la bibliothèque JERSEY et Servlet dans le pom.xml :
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>HelloRESTful</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>HelloRESTful Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/javax.servlet/javax.servlet-api -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/asm/asm -->
<dependency>
<groupId>asm</groupId>
<artifactId>asm</artifactId>
<version>3.3.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.sun.jersey/jersey-bundle -->
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-bundle</artifactId>
<version>1.19.2</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.json/json -->
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20160810</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.sun.jersey/jersey-server -->
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-server</artifactId>
<version>1.19.2</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.sun.jersey/jersey-core -->
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-core</artifactId>
<version>1.19.2</version>
</dependency>
</dependencies>
<build>
<finalName>HelloRESTful</finalName>
<plugins>
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.2</version>
<configuration>
<path>/HelloRESTful</path>
<port>8080</port>
</configuration>
</plugin>
</plugins>
</build>
</project>
Déclarer RESTful Servlet - com.sun.jersey.spi.container.servlet.ServletContainer - Un Servlet est fourni par Jersey REST API.
Vous devez déclarer le package qui contient votre classe RESTful avec ce servlet via les paramètre com.sun.jersey.config.property.packages.
(Notez l'utilisation du servlet > = 3.0)
Vous devez déclarer le package qui contient votre classe RESTful avec ce servlet via les paramètre com.sun.jersey.config.property.packages.
(Notez l'utilisation du servlet > = 3.0)
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
version="3.0">
<display-name>Hello RESTful Service</display-name>
<servlet>
<servlet-name>jerseyServlet</servlet-name>
<servlet-class>com.sun.jersey.spi.container.servlet.ServletContainer</servlet-class>
<init-param>
<param-name>com.sun.jersey.config.property.packages</param-name>
<param-value>org.o7planning.hellorestful</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>jerseyServlet</servlet-name>
<url-pattern>/rest/*</url-pattern>
</servlet-mapping>
</web-app>
5. RESTful Service class
Dans l'étape suivante, vous devez créer votre classe de service RESTful. Par exemple, la classe WeatherRESTfulService ci-dessous est un service Web fournissant des informations météorologiques.
WeatherRESTfulService.java
package org.o7planning.hellorestful;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Random;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.Produces;
@Path("/weather")
public class WeatherRESTfulService {
private static final DateFormat df = new SimpleDateFormat("yyyy-MM-dd");
//
// http://localhost:8080/contextPath/rest/weather/{location}/{date}
// Example:
// http://localhost:8080/contextPath/rest/weather/chicago/2016-09-27
// http://localhost:8080/contextPath/rest/weather/hanoi/2016-09-27
//
@Path("{location}/{date}")
@GET
@Produces("application/xml")
public String getWeather_XML(@PathParam("location") String location,//
@PathParam("date") String dateStr) {
Date date = null;
if (dateStr == null || dateStr.length() == 0) {
date = new Date();
} else {
try {
date = df.parse(dateStr);
} catch (ParseException e) {
date = new Date();
}
}
dateStr = df.format(date);
String[] weathers = new String[] { "Hot", "Rain", "Cold" };
int i = new Random().nextInt(3);
String weather = weathers[i];
return "<weather>"//
+ "<date>" + dateStr + "</date>"//
+ "<location>" + location + "</location>"//
+ "<info>" + weather + "</info>"//
+ "</weather>";
}
//
// http://localhost:8080/contextPath/rest/weather/{location}
// Example:
// http://localhost:8080/contextPath/rest/weather/chicago
// http://localhost:8080/contextPath/rest/weather/hanoi
//
@Path("{location}")
@GET
@Produces("application/xml")
public String getWeather_XML(@PathParam("location") String location) {
return getWeather_XML(location, null);
}
//
// http://localhost:8080/contextPath/rest/weather/{location}/{date}
// Example:
// http://localhost:8080/contextPath/rest/weather/chicago/2016-09-27
// http://localhost:8080/contextPath/rest/weather/hanoi/2016-09-27
//
@Path("{location}/{date}")
@GET
@Produces("application/json")
public String getWeather_JSON(@PathParam("location") String location,//
@PathParam("date") String dateStr) {
Date date = null;
if (dateStr == null || dateStr.length() == 0) {
date = new Date();
} else {
try {
date = df.parse(dateStr);
} catch (ParseException e) {
date = new Date();
}
}
dateStr = df.format(date);
String[] weathers = new String[] { "Hot", "Rain", "Cold" };
int i = new Random().nextInt(3);
String weather = weathers[i];
return "{" //
+ "'date': '" + dateStr + "'," //
+ "'location': '" + location + "'," //
+ "'info': '" + weather + "'" //
+ "}";
}
//
// http://localhost:8080/contextPath/rest/weather/{location}
// Example:
// http://localhost:8080/contextPath/rest/weather/chicago
// http://localhost:8080/contextPath/rest/weather/hanoi
//
@Path("{location}")
@GET
@Produces("application/json")
public String getWeather_JSON(@PathParam("location") String location) {
return getWeather_JSON(location, null);
}
}
7. Giải thích RESTful
Copiez l'URL ci-dessous et l'exécutez sur le navigateur :
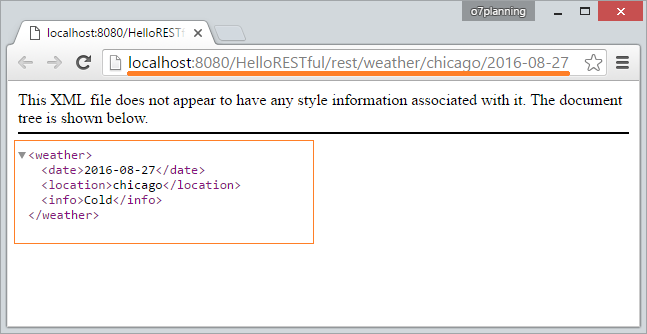
Lorsque vous exécutez l'URL ci-dessus, c'est la même chose que lorsque vous envoyez une requête au serveur :
GET /HelloRESTful/rest/weather/chicago/2016-08-27 HTTP/1.1
Host: localhost:8080
Si la requete ne spécifie pas le type de données de retour (type MINE), le type de données par défaut sera renvoyé par le service web.
Les requêtes peuvent spécifier le type de données de retour.
GET /HelloRESTful/rest/weather/chicago/2016-08-27 HTTP/1.1
Host: localhost:8080
Accept: application/xml
Ou :
GET /HelloRESTful/rest/weather/chicago/2016-08-27 HTTP/1.1
Host: localhost:8080
Accept: application/json
Dans l'exemple ci-dessus, si requête spécifie le type de retour comme application/json, vous obtiendrez un résultat comme ci-dessous :
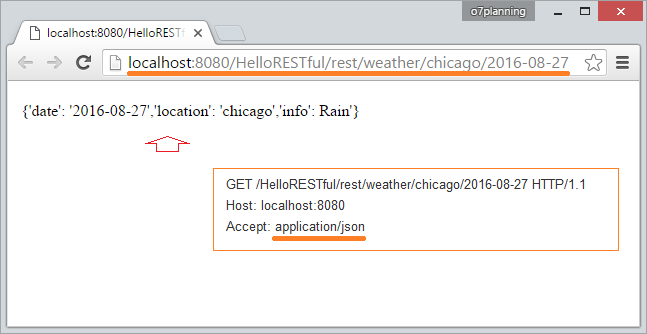
Pour spécifier le type de données renvoyées, vous devez rédiger une application Client pour former des Requêtes (Request) personnalisées et les envoyer au service web. Toutefois, vous pouvez également utiliser les AddOns du navigateur pour ajuster les requêtes avant de les envoyer au service RESTful.
RESTClient AddOns est un tel plugin, vous pouvez le configurer sur Firefox ou Chrome. Il vous aide à tester les services web RESTful dans le processus de développement d'applications.
Comment fonctionne Jersey RESTful ?
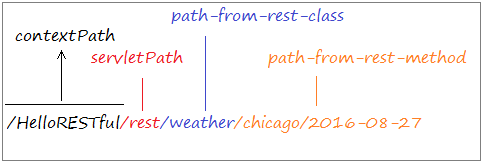
contextPath:
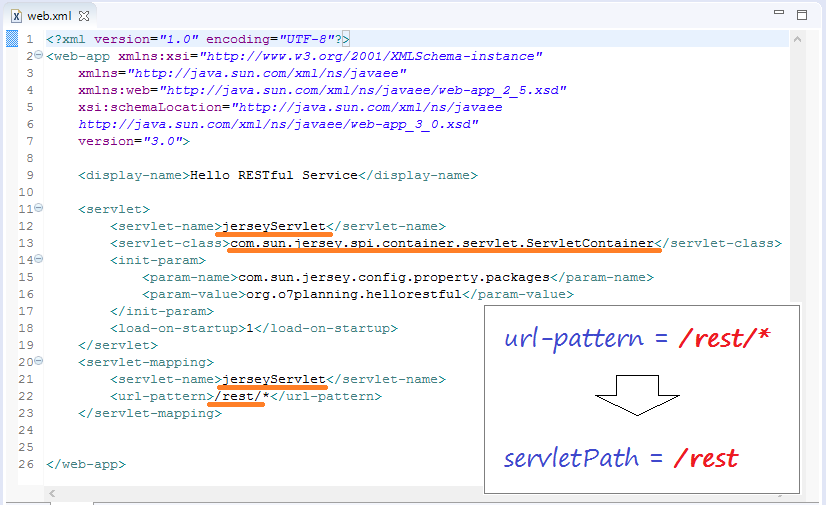
REST Path:
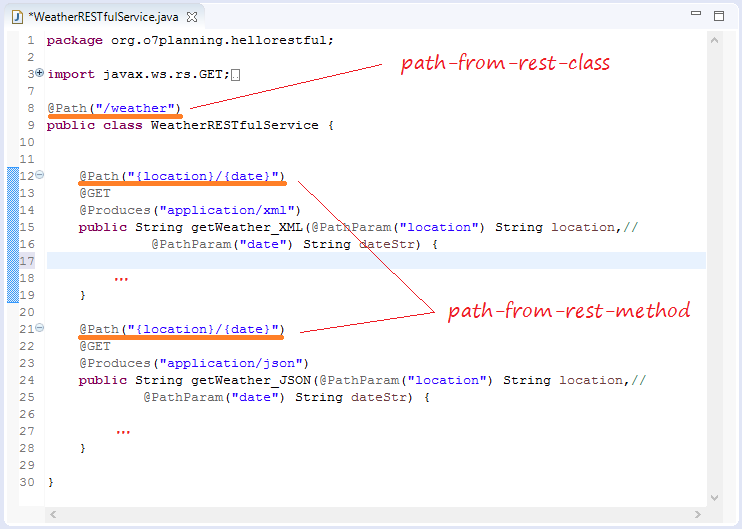
Tutoriels Java Web Service
- Qu'est-ce que RESTful Web Service?
- Tutoriel Java RESTful Web Service pour débutant
- Exemple CRUD simple avec Java RESTful Web Service
- Créer Java RESTful Client avec Jersey Client
- RESTClient Un débogueur pour RESTful Web Service
- Exemple de CRUD simple avec Spring MVC RESTful Web Service
- Exemple CRUD Restful WebService avec Spring Boot
- Exemple Spring Boot Restful Client avec RestTemplate
- Sécurité Spring RESTful Service utilisant Basic Authentication
Show More