Le Tutoriel de Java GatheringByteChannel
1. GatheringByteChannel
Si vous êtes débutant avec Java NIO, il est utile de lire tout d'abord les articles suivants pour acquérir des connaissances de bases :
- Java Nio
Comme vous le savez, WritableByteChannel est une interface qui fournit une méthode pour écrire des bytes à partir d'un ByteBuffer. GatheringByteChannel est une extension de WritableByteChannel qui fournit des méthodes de collecte d'écriture. En un seul appel, les bytes de plusieurs ByteBuffer(s) seront écrits dans le GatheringByteChannel.
public interface GatheringByteChannel extends WritableByteChannel
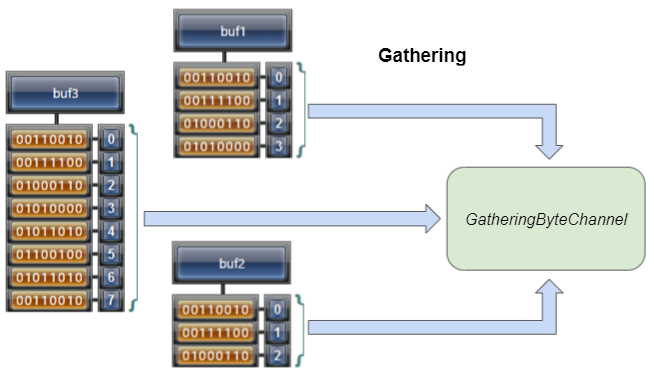
GatheringByteChannel channel =...; // (GatheringByteChannel)Channels.newChannel(fileOS);
ByteBuffer[] buffers = new ByteBuffer[]{buf1, buf2, buf3};
channel.write(buffers);
La hiérarchie des interfaces et des classes relatives à GatheringByteChannel :
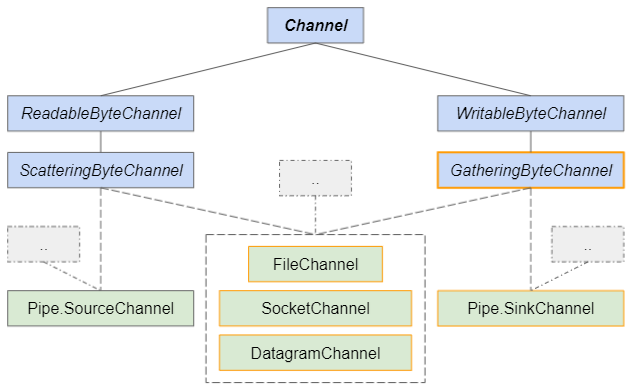
2. Methods
Les méthodes sont définies dans l'interface GatheringByteChannel :
public long write(ByteBuffer[] srcs, int offset, int length) throws IOException;
public long write(ByteBuffer[] srcs) throws IOException;
Les méthodes héritées de l'interface WritableByteChannel :
public int write(ByteBuffer src) throws IOException;
Les méthodes héritées de l'interface Channel :
public boolean isOpen();
public void close() throws IOException;
3. write(ByteBuffer[])
public long write(ByteBuffer[] srcs) throws IOException;
Écrit une séquence de bytes dans ce GatheringByteChannel à partir de plusieurs ByteBuffer(s) donné(s). La méthode renvoie le nombre de bytes écrits ou renvoie -1 si la fin du canal a été atteinte.
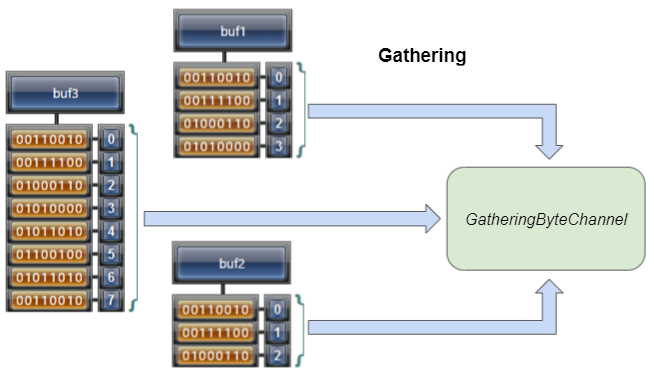
Convoquer cette méthode est l'équivalent de :
gatheringByteChannel.write(srcs, 0, srcs.length);
Une seule opération d'enregistrement sur le Channel peut avoir lieu à la fois. Cela signifie que si un thread initie une opération d'écriture sur un Channel, les autres threads ne peuvent pas écrire sur ce Channel, ils sont bloqués jusqu'à ce que l'opération soit terminée. D'autres opérations d'IO peuvent être effectuées au même moment que l'opération d'écriture en fonction du type de Channel.
4. write(ByteBuffer[], int, int)
public long write(ByteBuffer[] srcs, int offset, int length) throws IOException;
Ecrire une séquence de bytes dans ce GatheringByteChannel à partir d'un tableau de ByteBuffer(s), de l'index offset à offset+length-1. La méthode renvoie le nombre de bytes écrits ou renvoie -1 si la fin du canal a été atteinte.
Convoquer cette méthode est l'équivalent de :
ByteBuffer[] srcs2 = new ByteBuffer[] {srcs[offset], srcs[offset+1], .., srcs[offset+length-1]};
gatheringByteChannel.write(srcs2);
Une seule opération d'enregistrement sur le Channel peut avoir lieu à la fois. Cela signifie que si un thread initie une opération d'écriture sur un Channel, les autres threads ne peuvent pas écrire sur ce Channel, ils sont bloqués jusqu'à ce que l'opération soit terminée. D'autres opérations d'IO peuvent être effectuées au même moment que l'opération d'écriture en fonction du type de Channel.
5. Example 1
Exemple : Ecrire un programme qui copie le fichier, utilise ScatteringByteChannel pour lire le fichier d'entrée et utilise GatheringByteChannel pour écrire le fichier.
GatheringByteChannel_ex1.java
package org.o7planning.gatheringbytechannel.ex;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.ByteBuffer;
import java.nio.channels.Channels;
import java.nio.channels.GatheringByteChannel;
import java.nio.channels.ScatteringByteChannel;
public class GatheringByteChannel_ex1 {
// Windows: C:/somepath/test-file.txt
private static final String inputFilePath = "/Volumes/Data/test/in-file.txt";
private static final String outputFilePath = "/Volumes/Data/test/out-file.txt";
public static void main(String[] args) throws IOException {
try (// Input:
FileInputStream fis = new FileInputStream(inputFilePath);
ScatteringByteChannel inChannel = (ScatteringByteChannel) Channels.newChannel(fis);
// Output:
FileOutputStream fos = new FileOutputStream(outputFilePath);
GatheringByteChannel outChannel = (GatheringByteChannel) Channels.newChannel(fos);) { // try
ByteBuffer buf1 = ByteBuffer.allocate(10);
ByteBuffer buf2 = ByteBuffer.allocate(15);
ByteBuffer buf3 = ByteBuffer.allocate(10);
ByteBuffer[] buffers = new ByteBuffer[] { buf1, buf2, buf3 };
long bytesRead = -1;
while ((bytesRead = inChannel.read(buffers)) != -1) {
for (int i = 0; i < buffers.length; i++) {
System.out.println(" --- buffer[" + i + "] ---");
ByteBuffer buffer = buffers[i];
// Set limit = current position; position = 0;
buffer.flip();
outChannel.write(buffer);
buffer.clear();
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
- ScatteringByteChannel
- FileInputStream
- FileOutputStream
- Channels
Tutoriels de Java New IO
- Le Tutoriel de Java ReadableByteChannel
- Le Tutoriel de Java WritableByteChannel
- Le Tutoriel de Java Pipe.SinkChannel
- Le Tutoriel de Java Pipe.SourceChannel
- Le Tutoriel de Java ScatteringByteChannel
- Le Tutoriel de Java GatheringByteChannel
- Le Tutoriel de Java Buffer
- Le Tutoriel de Java DatagramChannel
- Le Tutoriel de Java Channel
Show More