Le Tutoriel de Flutter CircleAvatar
1. CircleAvatar
CircleAvatar est tout simplement un cercle dans lequel on peut ajouter une couleur d'arrière-plan, une image d'arrière-plan ou du texte. Il représente généralement un utilisateur avec son image ou ses initiales. Bien que l'on puisse créer un widget similaire à partir de zéro, ce widget est utile dans le développement rapide d'une application.
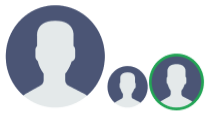
CircleAvatar Constructor
const CircleAvatar(
{Key key,
Widget child,
Color backgroundColor,
ImageProvider<Object> backgroundImage,
void onBackgroundImageError(
dynamic exception,
StackTrace stackTrace
),
Color foregroundColor,
double radius,
double minRadius,
double maxRadius}
)
On commence par un CircleAvatar simple composé d'une image d'arrière-plan donnée et d'une couleur d'arrière-plan par défaut.
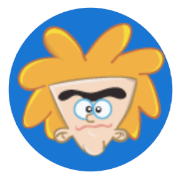
(ex1)
CircleAvatar(
radius: 100,
backgroundImage: NetworkImage("https://s3.o7planning.com/images/boy-128.png"),
)
En règle générale, CircleAvatar ne fournit pas de propriété pour définir les bordures. Cependant, vous pouvez l'envelopper dans un CircleAvatar différent avec un rayon plus grand et une couleur d'arrière-plan différente pour créer quelque chose de similaire à la bordure.
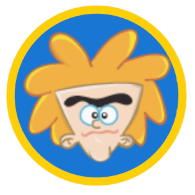
(ex2)
CircleAvatar(
radius: 110,
backgroundColor: Color(0xffFDCF09),
child: CircleAvatar(
radius: 100,
backgroundImage: NetworkImage("https://s3.o7planning.com/images/boy-128.png"),
)
)
Par exemple: Un CircleAvatar avec les initiales de l'utilisateur (user's initials).
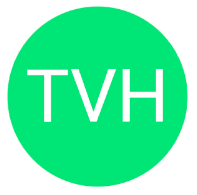
(ex3)
CircleAvatar(
radius: 110,
backgroundColor: Colors.greenAccent[400],
child: Text(
'TVH',
style: TextStyle(
fontSize: 90,
color: Colors.white
),
),
)
3. backgroundColor
backgroundColor - La couleur d'arrière-plan de CircleAvatar.
La valeur par défaut de backgroundColor est ThemeData.primaryColorLight si foregroundColor (couleur de lettres) est sombre, et est ThemeData.primaryColorDark si foregroundColor est claire.
Color backgroundColor
Par exemple:
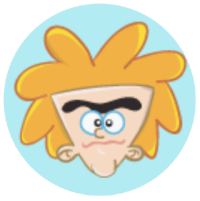
backgroundColor (ex1)
CircleAvatar(
radius: 110,
backgroundImage: NetworkImage("https://s3.o7planning.com/images/boy-128.png"),
backgroundColor: Colors.cyan[100],
)
4. backgroundImage
backgroundImage - L'image d'arrière-plan de CircleAvatar, qui est l'avatar de l'utilisateur.
Aux fins d'afficher les initiales de l'utilisateur sur CircleAvatar, il faut utiliser la propriété child.
ImageProvider<Object> backgroundImage
- Le Tutoriel de Flutter ImageProvider
backgroundImage (ex1)
CircleAvatar(
radius: 110,
backgroundImage: NetworkImage("https://s3.o7planning.com/images/boy-128.png"),
)
5. foregroundColor
foregroundColor - La couleur par défaut du texte dans CircleAvatar.
La valeur par défaut de foregroundColor est ThemeData.primaryColorLight si backgroundColor (couleur d'arrière-plan) est sombre, et est ThemeData.primaryColorDark si backgroundColor est claire.
Color foregroundColor
Par exemple:
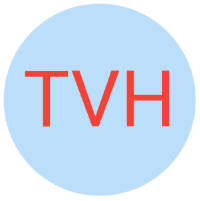
foregroundColor (ex1)
CircleAvatar(
radius: 110,
foregroundColor: Colors.red,
child: Text(
'TVH',
style: TextStyle(
fontSize: 90
),
),
)
6. radius
radius - Le rayon de CircleAvatar.
Si radius est spécificié, donc minRadius et maxRadius ne sont pas spécifiés. Spécificier radius est l'équivalent de spécificier les valeurs pour minRadius et maxRadius, qui ont tous la même valeur.
double radius
7. minRadius
minRadius - Le rayon minimum de CircleAvatar.
Si minRadius est spécifié, donc radius ne sera pas spécifié. Flutter sera automatiquement calculé pour s'approprier à la taille de CircleAvatar en fonction de l'espace disponible.
double minRadius
8. maxRadius
maxRadius - Le rayon maximum de CircleAvatar.
Si maxRadius est spécificié, donc radius ne sera pas spécificié. Flutter sera automatiquement calculé pour s'approprier à la taille de CircleAvatar en fonction de l'espace disponible.
double maxRadius
9. onBackgroundImageError
onBackgroundImageError - une fonction callback facultative, qui est convoquée lorsqu'une erreur de chargement d'image se produit pour backgroundImage.
void onBackgroundImageError(
dynamic exception,
StackTrace stackTrace
)
Par exemple: Un CircleAvatar essaie d'afficher l'avatar de l'utilisateur. Si une erreur se produit au cours du chargement de l'image, un texte d'erreur s'affichera.
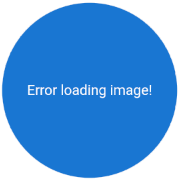
onBackgroundImageError (ex1)
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning.org',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
State<StatefulWidget> createState() {
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
// String imageUrl = "https://s3.o7planning.com/images/boy-128.png";
String imageUrl = "https://file-not-found";
bool _loadImageError = false;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Flutter CircleAvatar Example")
),
body: Center (
child: CircleAvatar(
radius: 100,
backgroundImage: this._loadImageError? null: NetworkImage(this.imageUrl),
onBackgroundImageError: this._loadImageError? null:
(dynamic exception, StackTrace stackTrace) {
print("Error loading image! " + exception.toString());
this.setState(() {
this._loadImageError = true;
});
},
child: this._loadImageError? Text("Error loading image!") : null
)
)
);
}
}
Tutoriels de programmation Flutter
- Installer Flutter SDK sur Windows
- Installer Flutter Plugin pour Android Studio
- Créez votre première application Flutter - Hello Flutter
- Le Tutoriel de Flutter Scaffold
- Le Tutoriel de Flutter AppBar
- Le Tutoriel de Flutter BottomAppBar
- Le Tutoriel de Flutter TabBar
- Le Tutoriel de Flutter Banner
- Le Tutoriel de Flutter SplashScreen
- Le Tutoriel de Flutter BottomNavigationBar
- Le Tutoriel de Flutter FancyBottomNavigation
- Le Tutoriel de CircularProgressIndicator
- Le Tutoriel de Flutter LinearProgressIndicator
- Le Tutoriel de Flutter Container
- Le Tutoriel de Flutter Center
- Le Tutoriel de Flutter Align
- Le Tutoriel de Flutter Row
- Le Tutoriel de Flutter Column
- Le Tutoriel de Flutter Stack
- Le Tutoriel de Flutter IndexedStack
- Le Tutoriel de Flutter Spacer
- Le Tutoriel de Flutter Expanded
- Le Tutoriel de Flutter SizedBox
- Le Tutoriel de Flutter RotatedBox
- Le Tutoriel de Flutter Card
- Le Tutoriel de Flutter CircleAvatar
- Le Tutoriel de Flutter IconButton
- Le Tutoriel de Flutter FlatButton
- Le Tutoriel de Flutter TextButton
- Le Tutoriel de Flutter ElevatedButton
- Le Tutoriel de Flutter SnackBar
- Le Tutoriel de Flutter Tween
- Le Tutoriel de Flutter SimpleDialog
- Le Tutoriel de Flutter AlertDialog
- Navigation et Routing dans Flutter
- Le Tutoriel de Flutter Border
- Le Tutoriel de Flutter ContinuousRectangleBorder
- Le Tutoriel de Flutter RoundedRectangleBorder
- Le Tutoriel de Flutter CircleBorder
- Le Tutoriel de Flutter StadiumBorder
- Le Tutoriel de Flutter EdgeInsetsGeometry
- Le Tutoriel de Flutter EdgeInsets
- Le Tutoriel de Flutter Alignment
- Le Tutoriel de Flutter Positioned
Show More